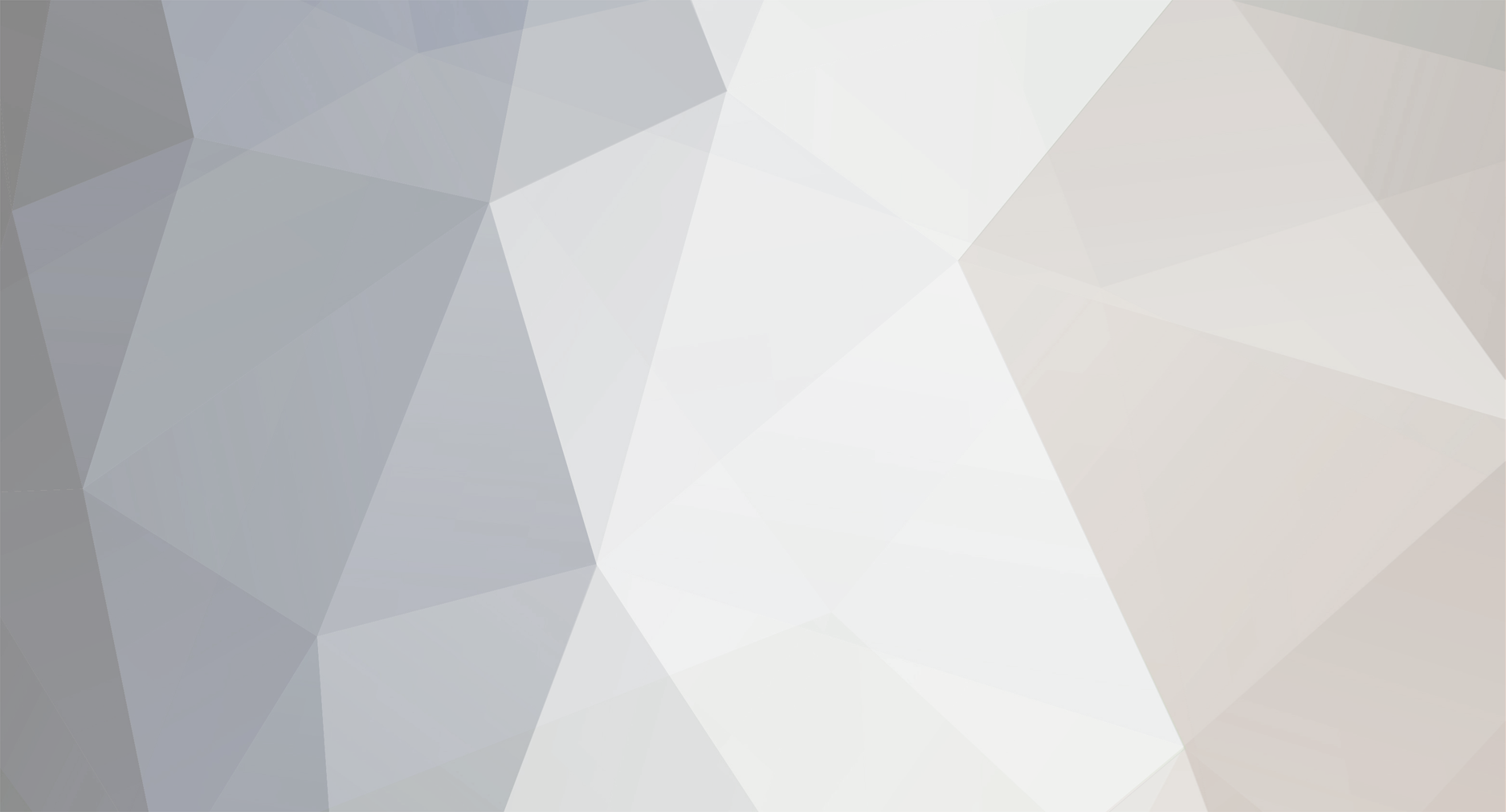
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
[SOLVED] mysql query/insert/update sequence rules?
btherl replied to dsaba's topic in PHP Coding Help
Can you post your script (or a minimized script which demonstrates the problem)? -
How do I use $_REQUEST to get a list of variables?
btherl replied to php_joe's topic in PHP Coding Help
Try the following to investigate: var_dump($_REQUEST); print_r($_REQUEST); foreach ($_REQUEST as $name => $value) { print "$name has value $value\n"; } Then call your script with some arguments, for example http://domain.com/script.php?something=this&another=somethingelse $_REQUEST will also include posted variables and cookies, in addition to GET variables (which is what is used in the example url above) -
search for files in directory by a like filename...
btherl replied to simple_man_11's topic in PHP Coding Help
The simple way is with glob() $files = glob("{$path}*{$like}*"); That should give you the file "./uploads/specialcode.pdf". You may want to strip off the path afterwards, using basename() -
Just add "ORDER BY numbers" or "ORDER BY numbers DESC" to the very end of the query.
-
It's possible you have a space or empty line before your "<?php". I don't see anything in your code that I would expect to generate output.
-
How about SELECT id, name, numbers FROM table WHERE id IN ( SELECT max(id) FROM numbers GROUP BY name ); HAVING won't work because the condition is based on both the actual id and max id. HAVING can only deal with aggregated data, not individual row data.
-
You're using $result for both the inner and outer query. Use different variable names and you should be fine
-
To fix it, you need to tell us what algorithm you are implementing. Right now we are guessing your algorithm based on your code. And obviously your code does not implement the algorithm you want it to
-
Can you post the rest of your script?
-
gaurentee a "unique" image is uploaded; should use md5()?
btherl replied to arianhojat's topic in PHP Coding Help
Yes, your code encrypts the actual data. Strictly speaking it creates a "hash" of the data. md5() is appropriate for this (although it won't detect similar images of course). GUID isn't appropriate, as it's randomly generated rather than derived from the file itself. As for which is faster, run each a thousand times and measure it -
Example: $strsql = "SELECT ".$bywhat." , COUNT(*) as num FROM database ORDER BY ".$bywhat." DESC"; $ressql = mysql_query($strsql) or die("Error in $strsql\n" . mysql_error());
-
The first thing to do is replace $dbconn = @mysql_connect("localhost","username","password"); @mysql_select_db("database"); with $dbconn = mysql_connect("localhost","username","password") or die("Couldn't connect to database\n"); mysql_select_db("database") or die("Couldn't select database\n"); Similarly with every call to mysql_query(). You should add 'or die("Query failed: " . mysql_error());' to each one. But DON'T add it to mysql_num_rows(), or mysql_fetch_row() or any of the result fetching functions. @ will ignore errors. die() will tell you when there is an error.
-
Howto stop long Url wrapping in email at 989 chars?
btherl replied to ryel01's topic in PHP Coding Help
If you need more information, you can store it on the server and put a reference to that information in the url. For example http://www.site.com/?id=12345 Then, id 12345 will be associated with the full data on your server. -
I don't trust php 5's memory_get_usage(). It doesn't always give sensible values. You might want to print out the memory usage at various stages throughout your program and track it, and see what's going on.
-
If you finish a function, memory for variable accessible ONLY in that function will be reused automatically. Variables accessible outside the function will not be freed automatically. If variables are still in scope and you want to reuse their memory, you should use unset($var). Edit: And if the script finishes, then all memory used by it will be freed (in theory, there's some bugs related to that in php4, I think they are fixed in php5).
-
Aha, it is possible. You need this function: http://sg.php.net/manual/en/function.preg-replace-callback.php Your callback will need to see which string was matched (eg rep1, rep2) and return the appropriate variable. It could be as simple as: function my_callback($matches) { return $$matches[0]; } The variables must be available within my_callback(), so you may need to declare them as global. Or you can return $_GLOBALS[$matches[0]] instead. Then echo preg_replace_callback("/(@{2})(.{1,10})(@{2})/","my_callback",$text); No guarantees on this untested code. Note that to use preg instead of ereg, you usually just need to add "/" to the start and end of the pattern, and escape any "/" which appears within the pattern.
-
How about: SELECT * FROM message WHERE recipient_id IN (1, 2) ORDER BY message_id DESC LIMIT 1 Or, order by time_sent, which should match message_id in ordering I presume. Then you can check if the message was to 1 or 2 and display the appropriate output. Is that what you wanted?
-
To be safe, I would use mysql_real_escape_string() before inserting the data to mysql. Then you can be sure that ANY character will be correctly stored (any that is supported by the underlying data type that is. Binary data will need mysql's binary data type, which I forgot the name of. But \n is still ok for text/varchar data).
-
You can output headers with Header() as long as you have not yet produced any other output. Same with cookies. If it's not working, you need to find where the output has already been produced. You haven't explained what you are trying to do and what is going wrong.
-
For that example, yes you must create an instance of SQL, because the method refers to $this. But in general, you can just use SQL::query($var);
-
You definitely can't do it like that. There's bizarre extensions in perl which allow you to do that within a regexp, but I would do it outside to maintain sanity. The simplest and cleanest way I can think of is to use one ereg_replace() for each variable you are replacing. Then there's no need to be so flexible with the regexp. If you're planning to do some heavy duty replacing (too much to use individual ereg_replace()), I would use Smarty or another existing template system. And finally, preg_replace() is faster
-
Assuming you want all wars between your_id and target_id: $result = dbquery("SELECT war_id FROM fusion_war WHERE (a_id='".$your_id."' AND d_id='".$target_id."' ) OR ( d_id='".$your_id."' AND a_id='".$target_id."' )");
-
What error do you get when you try to validate?
-
No.. you will need to remove the extra "WHERE". Apart from that it's fine. Are you wanting to check two "AND" conditions with an "OR" between? Rather than all "OR" ?
-
The manual is pretty unambiguous .. it doesn't mention any settings that can be altered. If it's a once-off import, why not just do it the easy way? I don't quite get what you mean by "subtract 100 years for anyone born before today", but it's simple enough to insert "19" in front of each year before calling str_to_date().