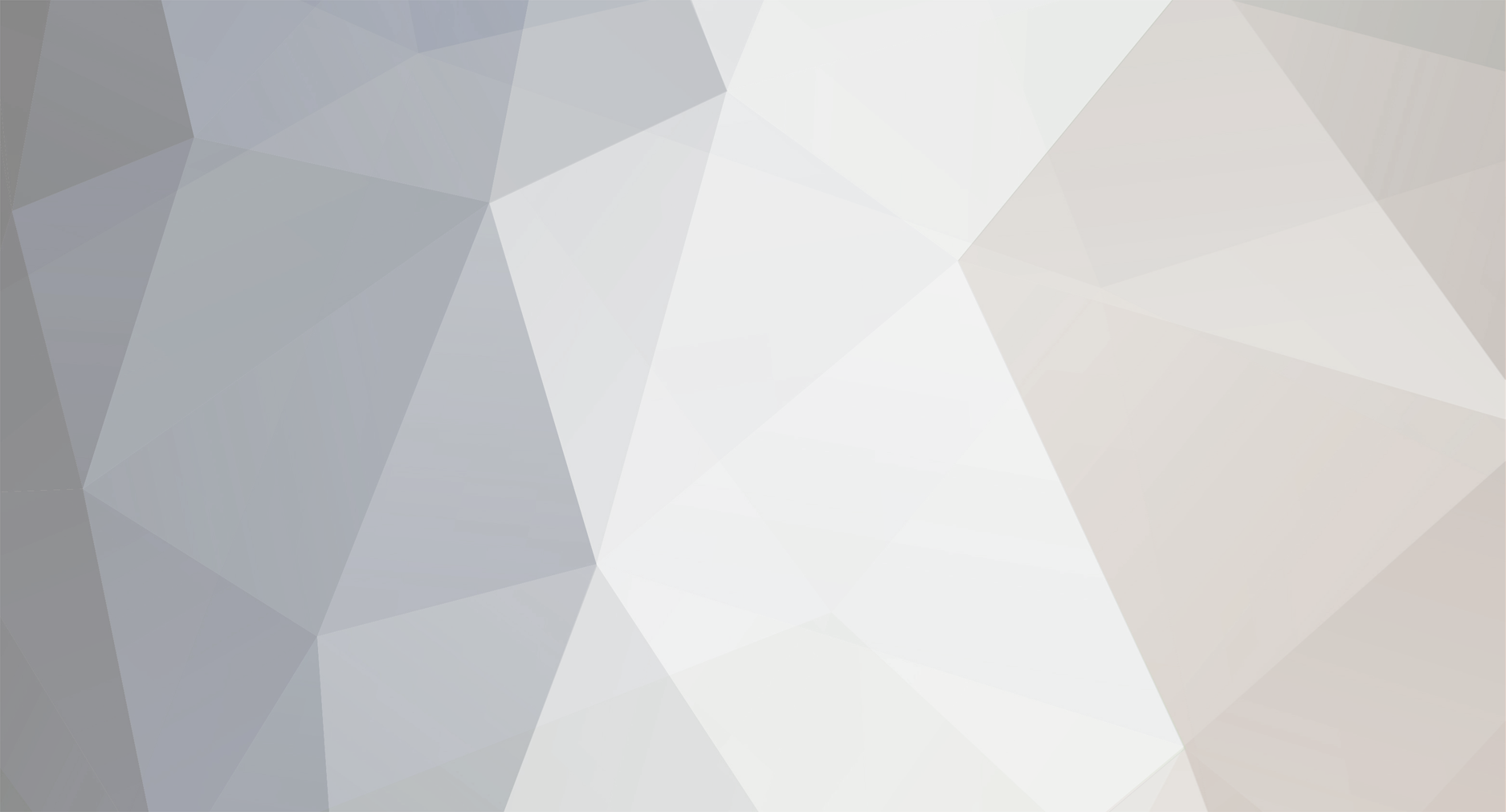
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
To match records that do not exist in another table: SELECT * FROM tableA LEFT JOIN tableB ON (tableA.joincol = tableB.joincol) WHERE tableB.joincol IS NULL
-
Please post your code, along with any warning or error messages.
-
I think you want array_search() .. eg <?php $main_nav = array( "names" => array("Home", "Pictures", "Templates", "Website Coding"), "pages" => array("index.php", "pictures.php", "templates.php", "website_coding.php") ); $find = 'Templates'; $index = array_search($find, $main_nav['names']); print "$find uses the script {$main_nav['pages'][$index]}\n"; ?>
-
A quicker approach may be to use file() to read the page into an array of lines, find the line containing "scrobbled" and process only that line.
-
What exactly are you integrating here? We have a set V of vertexes, E of edges (equal weighted). We can find sums of paths, sure. And we can think about directions, since we're on a grid. Where does integration come into that? The direct distance from one point to another is always sqrt(abs(x1-x2)^2 + abs(y1-y2)^2), the high school algorithm. The actual distance is what we are calculating. No shortcuts there.
-
An infinite number of points? How many computers do you know that can handle an infinite number of points? I'm sure that those algorithms are intended for finite graphs only.
-
Can you show the code you have so far, and give a clearer explanation of what you want to do? An example would be great.
-
To search for that key as a value: if (in_array($key, $array)) To search for that key as a key: if (array_key_exists($key, $array))
-
That sounds like Dijkstra's algorithm to me. Or perhaps A* (Dijkstra's algorithm augmented with heuristic evaluations for each point).
-
Other solutions on : How to modify and remove some parts of an array()????
btherl replied to andz's topic in PHP Coding Help
I typically use a loop like this: $array = array(1, 2, 3, 4, 5); # Array after explode(), if you start with '1~2~3~4~5' foreach ($array as $key => $value) { if ($value == 2) unset($array[$key]); } var_dump($array); This will delete the "2" from the array. Note that it won't update the array indices, so be careful if you rely on these. -
[SOLVED] Shorten Variables Longer Than $x Characters
btherl replied to Trium918's topic in PHP Coding Help
Overlib can do that -
What about this case? |X|-| |-|O| |-|-| |-|-| |-| |-|-| |-| |-|-|-| |-|-|-|-|-| All points now are connected to at least 2 other points. The dead end is there, but expanded.
-
Hmm.. so you are checking if there is any x for which there is only one valid y, and vice versa? That check will be quite fast to do. I can imagine situations where the check will not succeed even though there is only one path, but that can't be helped. Eg. |X|-| |-|O| |-| |-|-| |-| |-|-|-| |-|-|-|-|-| Here there is a choke point at the bottom, but it can't be detected by a simple check, because there are 2 valid values of y for each value of x. Why must we? I still disagree with this assumption I think we should forget about checking all paths, and go for an algorithm which gives a "reasonably good" path.
-
The only answer there is to benchmark it. But I would go for convenience first if I were you. The $lang[] approach seems much more convenient to me, because: - You can list all the translations easily. - You don't pollute the namespace with the defines.
-
Thanks, that makes much more sense But please, paragraphs! Paragraphs! Paragraphs! It may not always be necessary to check all possible paths for two reasons - We may be able to accept an approximate solution. - In the case of a grid with equal weighted edges, any "direct" path is a shortest path (none exists in the example above though). A "direct" path is any path where all steps are in the direction of the "general directional derivative" from point X to O. In this example, all steps would have to be up and right, and no direct path exists. What is the algorithm by which you find this point "&" ? I agree that it exists, but I don't see any efficient method of finding it.
-
Here you go: $st = 'hello'; $command = '/usr/bin/php -r \'echo "'.$st.'";\''; print "About to execute $command\n"; $test = system($command); echo $test; Much better to print out the command before executing, so you can visually inspect it. Dealing with shell escaping is a hassle though, for any more complicated code.
-
cooldude, I'm a pure mathematician and even I am confused by your posts ??? Please use paragraphs, and give examples where possible. Regarding the "always move closer" idea, it will need to have backtracking (in some form) to handle dead ends. An efficient way to do this might be to mark a map location as "dead end", and eliminate it from all further processing. Then it will never be considered in any further paths. Then you take a step back, and continue with the same algorithm, but disregarding the locations now marked as "dead end". In this way, you progressively eliminate dead end locations without having to process each dead end location more than once. Of course there will be some maps for which this performs terribly.. so a lot depends on what the maps actually look like. Arguably, path finding should not be implemented for mazes, because the user should be solving the maze himself So in practice I imagine the maps will be simpler than a maze?
-
If your map is as simple as a grid (with equal weights on every edge), then you could use some heuristics to improve your path finding algorithm. For example, if you find a path which never moves away from the destination at any step, then you have a shortest path. This is not true in general, only for the example map you showed. Similarly, you might want to check a straight line first, hoping that there is no need to run a full path-finding algorithm Once you have different costs associated with particular steps (such as different terrain), all bets are off. But you may be ok with a "shortest path" which is not necessarily the fastest path.
-
[SOLVED] Incrementing backwards through an array
btherl replied to ninedoors's topic in PHP Coding Help
prev() is a different way of doing things to $array[$i]. It has no effect here. Just do this: <?php $array = array(2, 2, 5, 8, 21, 15, 16); $len = count($array); for($i = $len - 1; $i >= 0; --$i) { $h = $array[$i]; echo $h . ", "; } echo "\n"; ?> Note that this only works when your array is indexed by consecutive integers. An alternative is to use array_reverse(), and foreach(). -
[SOLVED] ODBC and committing a transaction? Help!
btherl replied to leesiulung's topic in PHP Coding Help
I would test it with: odbc_autocommit($conn, false); # do an insert odbc_rollback($conn); If the insert is visible, then something is wrong. Then try the same with odbc_commit(). The insert should be visible, but only after the commit. You might want to add a sleep(10) before the commit (and check the db in another window), so you can verify that the insert only becomes visible after committed. -
Ah, that would make sense. I don't know much about SELinux, so I'll leave that to you to figure out But it sounds like it can deny access to apache for making tcp connections. Regarding php installations, after compiling php you have several ways to install it. You can install it as an apache module (something like /usr/lib/php4/apache/php4.so), as a CLI program (/usr/bin/php), or both. Normally it will run as a module under apache, as that is more efficient and more powerful. I suspect that the difference is more that the user Apache runs under does not have permission to make arbitrary outgoing tcp connections, but your user account does. So when run from the command line, php can connect, but not when run through apache.
-
How about compiling a command line installation of php in a local directory, and see if that works. If it does, then you've narrowed it down 100% to the php configuration. And if that works, then you know that installing your own php in apache could fix the problem. The message of "permission denied" for a tcp connection is very strange indeed. It doesn't make much sense to me either.
-
Before fetching data from a result with mysql_fetch_assoc(), you must first do a query with mysql_query(). Otherwise you will get the error in your original post.
-
You have a problem there in that the code in double quotes will have variable substitution done BEFORE being passed to php4. Try enclosing it in single quotes instead. You will need to escape single quotes within the string also. Eg. $test = exec('/usr/bin/php -r \'$ffmpegObj = new ffmpeg_movie($filetmp);$duration = $ffmpegObj->getDuration();\'');
-
It's saying that $result is not a mysql result. Check back through your code to where $result comes from. You may need to test it after calling mysql_query(), as the query may have failed.