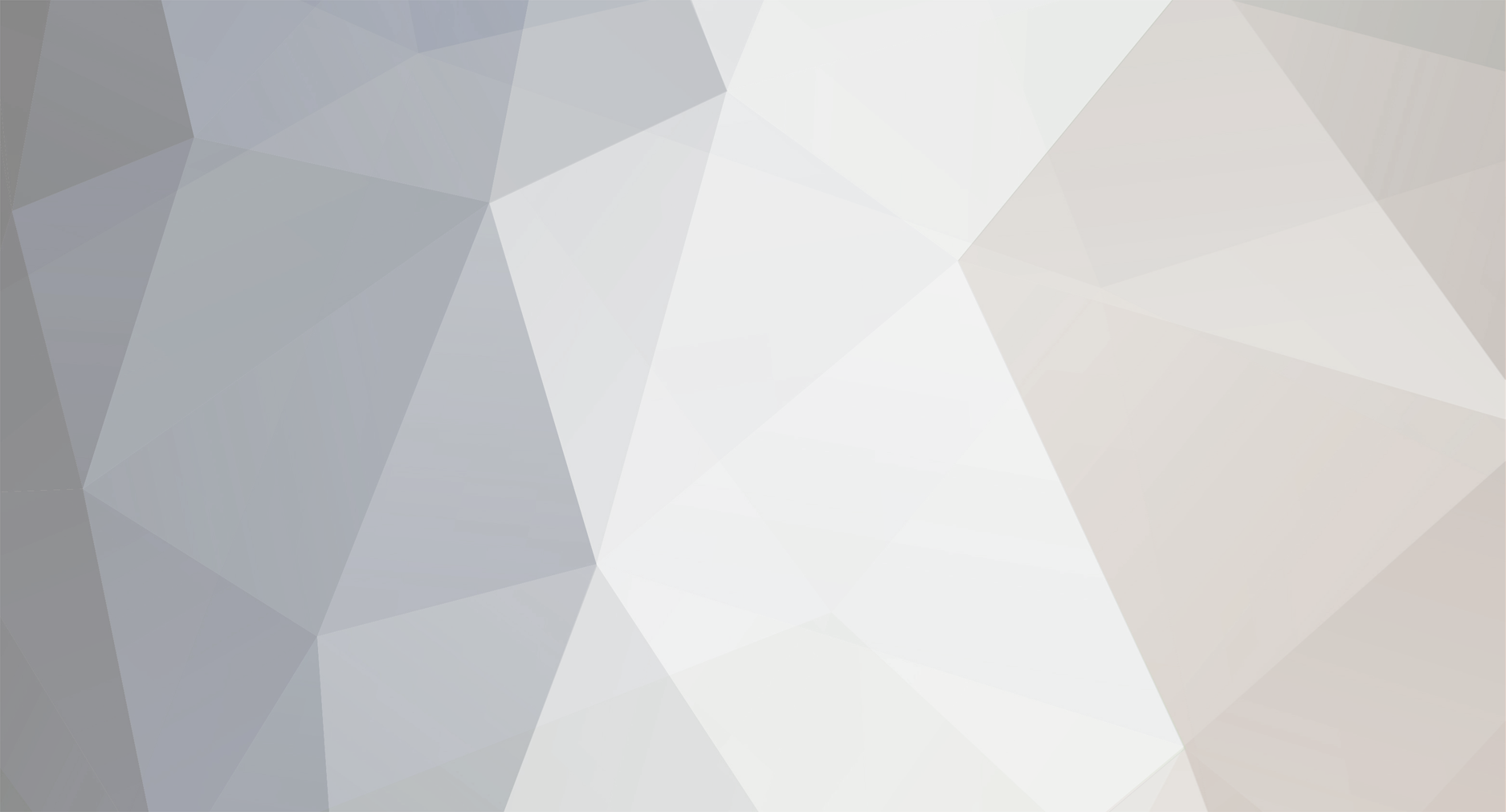
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
remove ?a=blah from link after whatever needs to be done is done?
Psycho replied to jBooker's topic in PHP Coding Help
Use the header() function with a Location reference to a success page. Example: test.php <?php if ($_GET['test']=='true') { //Do some process header('Location: http://localhost/test/success.php'); } else { echo "Click this <a href=\"http://localhost/test/test.php?test=true\">link</a> to reload the page with the paramater on the URL."; } ?> success.php <?php echo "Process succeeded"; ?> -
You should never, never use loops for doing queries like that. It is a big performance hit and there are better ways to do it. I can't really make sense of the first block of code you posted as tehre are multiple instances of PHP closing tags in succession - it looks like you left out the code for displaying the results. Instead of looping through the IDs, just create a single query: $query = "SELECT * FROM sublink_2 WHERE id IN (" . implode(',', $id) . ") and cat='$cat' ORDER BY id"; $result = mysql_query($query) or die(mysql_error()); if (!mysql_num_rows($result)) { echo "nothing for display"; } else { //display the results } The second block of code makes little sense to me as well. It looks like you created looping structures that are not properly nested. The Loop using "foreach($qua as $quant)" is used to make the inserts into the database, but the values it uses are created in the "while($query2 = mysql_fetch_array($query1))". That loop ends before the $qua loop begins, so the same values will be inserted into the database based upon how many records there are in the first query results. (properly nesting your code will help prevent this type of error). I took a shot at rewriting it more efficiently, but I'm not 100% sure of the result/logic you want. (I'm sure there are typos as well as I could not test it) if($mode=="add_all_$listcart") { $cat = htmlspecialchars($_POST['cat']); $id = $_POST['id']; $qua = $_POST['qua']; mklog($username_2,"Add items to shopping cart -- selecting",$_SESSION['permission']); //Run query to get records in sublink_2 for list of IDs //where there are no matching records in cart $query = "select * FROM sublink_2 RIGHT JOIN cart ON sublink_2.id = cart.item_id WHERE sublink_2.id IN (" . implode(',', $id) . ") AND sublink_2.cat='$cat' AND cart.item_id IS NULL ORDER BY id"; $result = mysql_query($query) or die (mysql_error()); if (mysql_num_rows($result)) { //Create insert records from the results while($record = mysql_fetch_array($result)) { //Get the corresponding quantity for the id if(array_search($record['id'], $id) && count($qua) > array_search($record['id'], $id)) { $quant = $qua[array_search($record['id'], $id)]; $insert_records[] = "('{$record['title']}', '{$record['id']}', "; . "'{$quant}', '{$username_2}', '{$record['price']}', " . "' $total_ps', '0', '0', '$date', '0')\n"; } } //Creat the combined insert query and run $query = "INSERT INTO cart (title,item_id,quantity,username,price,total,read_r,payed,date,approve)\n " . "VALUES (" . implode(',', $insert_records) . ")"; $result = mysql_query($query) or die (mysql_error()); } } noerror("$title_linkS Added Successfully To your Shopping Cart"); redirection("?mode=cart_show","4500");
-
Sorry, I forgot you were working with a serialized array - and I had some typos. Here is a working piece of code using the value you posted in the beginning: <?php //Create the array of selected values $selected_values_serial = 'a:4:{i:0;s:31:"Soldiers, marines, paratroopers";i:1;s:37:"Sailors, coast guard, merchant marine";i:2;s:14:"Airmen, pilots";i:3;s:28:"Policewomen, security people";}'; $selected_values = unserialize($selected_values_serial); $checkOptions = array ( 'Soldiers, marines, paratroopers', 'Sailors, coast guard, merchant marine', 'Airmen, pilots', 'Policewomen, security people', 'Truck drivers', 'Firemen', 'Executioners', 'Nurses, doctors', 'Prostitutes, tramps', 'Cruel/abusive women', 'Cowgirl', 'Surfers, life guards', 'Biker chicks', 'Warrior/Wrestler', 'Royalty, nobility', 'Business people. managers, the boss', 'Divas', 'Teachers', 'Daddy', 'Mommy' ); foreach ($checkOptions as $option) { $selected = (in_array($option, $selected_values))?' checked="checked"':''; echo "<input type=\"checkbox\" name=\"Archetypes[]\" value=\"$option\"$selected />$option<br />\n"; } ?> However, based on the large piece of code you posted previously I would make this into a function that takes two parameters. The array of all values and the array of selected values.
-
Let's make your life easier and put all the options in an array as well: <?php $checkOptions = array ( 'Soldiers, marines, paratroopers', 'Sailors, coast guard, merchant marine', 'Airmen, pilots', 'Policewomen, security people', 'Truck drivers', 'Firemen', 'Executioners', 'Nurses, doctors', 'Prostitutes, tramps', 'Cruel/abusive women', 'Cowgirl', 'Surfers, life guards', 'Biker chicks', 'Warrior/Wrestler', 'Royalty, nobility', 'Business people. managers, the boss', 'Divas', 'Teachers', 'Daddy', 'Mommy' ); foreach ($checkOptions as $option) { $selected = (in_array($option, $row->Archetypes)) ? ' selected="selected"' : ''; echo "<input type=\"checkbox\" name=\"Archetypes[]\" value=\"$option\"$selected />$option<br />\n"; } ?>
-
If all of your events end with the word 'Cup' then what Effigy posted will work - If not, then you need to determine what rule to apply - perhaps removing ' Round *' or ' Qualifying *' from all of the values. However the foreach loop is not necessary. You can use an array with preg_replace(). I would suggest the following that would do the same thing with one line: $rounds = array( 'FA Cup', 'FA Cup Qualifying Round 1', 'FA Cup Qualifying Round 2', 'FA Cup Qualifying Round 3', 'FA Cup Round 1', 'FA Cup Round 2', 'Carling Cup', 'Carling Cup Round 1', 'Carling Cup Round 2', ); $events = array_unique(preg_replace('/( Qualifying)? Round(.)*/', '', $rounds)); echo "<pre>"; print_r($events); echo "</pre>";
-
You are going to need a 3rd party app/tool to do this. There is no internal functions within PHP that can do this.
-
Yes, some people would probably build the script to just programatically go through every field in the form, but that can create a problem when you decide to have a field that is not required.
-
To make things easy set the valueof select options with the text "---select---" to an empty value. <html> <head> <script type="text/javascript"> var requiredFields = ['select1', 'select2', 'input1', 'input2']; function validate_form(formObj) { for (var i=0; i<requiredFields.length; i++) { if (!formObj[requiredFields[i]].value) { alert('Please fill all the fields.'); return false; } } return true; } </script> </head> <body> <form name="test" onsubmit="return validate_form(this)"> Select 1: <select name="select1"> <option value="">---Select---</option> <option value="1">One</option> <option value="2">Two</option> <option value="3">Three</option> </select><br> Select 2: <select name="select2"> <option value="">---Select---</option> <option value="1">One</option> <option value="2">Two</option> <option value="3">Three</option> </select><br> Input 1: <input type="text" name="input1"><br> Input 2: <input type="text" name="input2"><br> <br> <button type="submit">Submit</button> </body> </html>
-
My Own Working Coding for Get Sum Total in Javascript
Psycho replied to djbuddhi's topic in Javascript Help
If someone asked for this solution, you should have posted it in that thread. -
how to convert radio button to checkbox - code included
Psycho replied to nandwana_vikas's topic in PHP Coding Help
Hmm, I'm a little pressed for time to be reading through all of that to make sense of it. But, basically you just need to use checkboxes instead of a radio group. Give each checkbox the same name in the format name="something[]" with the brackets so the results will be received as an array. Only "checked" values will be returned. I would suggest two columns of checkboxes (one for approval and one for rejection). you could use one checkbox for each, but then you have to use a flag or some other means to track which records you were just reviewing (in case another record was added while you were reviewing). It also allows you to NOT review some of the questions and save for later. So, if you have two sets of checkboxes with the names rejected[] and approved[] you could just run two queries. Something like: //Set approved to false for rejected records $query = "UPDATE table SET approved=0 WHERE id IN (" . implode(',', $_POST['rejected'] . ")"; //Set approved to true for approved records $query = "UPDATE table SET approved=1 WHERE id IN (" . implode(',', $_POST['approved'] . ")"; -
That question would require way too much detail to answer than would be appropriate for a forum post. There are plenty of tutorials out there on PHP and databases. I suggest you look up a couple. Before you start coding you will want to design your database.
-
My Own Working Coding for Get Sum Total in Javascript
Psycho replied to djbuddhi's topic in Javascript Help
Did you have a question? If you are posting your code as an example of how that should be done, then I would sieriously disagree with that methodology. There's no validation and the results are improperly displayed in some instances. -
[SOLVED] dynamic table (for canda and u.s. shipping info)
Psycho replied to M.O.S. Studios's topic in Javascript Help
<html> <head> <script type="text/javascript"> var ship_pro=new Array() ship_pro[0]='' ship_pro[1]=['Ontario|ON', 'Quebec|QC', 'Nova Scotia|NS', 'New Brunswick|NB', 'Manitoba|MB', 'British Columbia|BC', 'Prince Edward Island|PE', 'Saskatchewan|SK', 'Alberta|AB', 'Newfoundland and Labrador|NU', 'Yukon|YT'] ship_pro[2]=['state1|state1', 'state2|state2'] function updateship_pro(selectedship_pro) { var ship_prolist=document.shipform.ship_pro; ship_prolist.options.length = 0; for (var i=0; i<ship_pro[selectedship_pro].length; i++) { option = ship_pro[selectedship_pro][i].split('|'); ship_prolist.options[ship_prolist.options.length] = new Option(option[0], option[1]); } document.getElementById('address_fields').style.visibility = (selectedship_pro==0) ? 'hidden' : ''; document.getElementById('addr_3').style.visibility = (selectedship_pro!=2) ? 'hidden' : ''; } </script> </head> <body> <form name="shipform" action="test.php" method="GET"> <select name="ship_con" onChange="updateship_pro(this.selectedIndex)"> <option value="">Please select</option> <option value="Canada">Canada</option> <option value="U.S.A.">USA</option> </select><br> <span id="address_fields" style="visibility:hidden;"> <select name="ship_pro"></select><br> Address Line 1: <input type="text" name="line1"><br> Address Line 2: <input type="text" name="line2"><br> <span id="addr_3" style="visibility:hidden;">Address Line 3: <input type="text" name="line3"></span><br> </span> <input type="submit" Value="test"> </form> </body> </html> -
How is that more functinoal? It only shows the error. By echoing the query to the page along with the eorror it is much more informative.
-
Quite simply, your query is failing. Add some error handling to find out why: $query = "SELECT about FROM `profile` WHERE `accountid` = '{$_SESSION['login_id']}'"; $sql = mysql_query($query) or die ("Query:<br>$query<br>Error:<br>".mysql_error());
-
I really don't see a reason for validating a radio group - just set one value as selected by default and the user can only select a different value - he cannot unselect all the values. There should be server-side validation as well. But if you really want/need to do it client side here is some sample code. I'm not going to try to interpret what you are doing above. You can just implement this as you need it: <html> <head> <script type="text/javascript"> function validate(formObj) { radioGrp = formObj.color; for(i=0; i<radioGrp.length; i++) { if (radioGrp[i].checked) { return true; } } return false; } </script> </head> <body> <form name="theform" onsubmit="return validate(this);"> What is your favorite color:<br> <input type="radio" name="color" value="blue">Blue<br> <input type="radio" name="color" value="red">Red<br> <input type="radio" name="color" value="green">Green<br> <input type="radio" name="color" value="yellow">Yellow<br> <input type="radio" name="color" value="purplpe">Purple<br> <button type="submit">Submit</button> <form> </body> </html>
-
I'm not going to fix your particular problem because I think your code is way overblown. instead I'll post cleaner code that will accomplish what you want. The process you are taking also makes no sense. You are artificially creating the current time from a server-side script and then trying to keep that time current. Why not just use JavaScript to keep the current time? If you need to show the server time, then just echo the server time to the page and use that to determine the correct offset - and still use JS to keep the time. <html> <head> <script type="text/javascript"> var daysAry = ['Sunday', 'Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday', 'Saturday']; var mnthAry = ['January', 'February', 'March', 'April', 'May', 'June', 'July', 'August', 'September', 'October', 'November', 'December']; function padVal(numInt) { var padVal = '0' + numInt.toString(); return padVal.substr(padVal.length-2, 2); } function live_clock() { var today = new Date(); var hours = (today.getHours()<=12) ? padVal(today.getHours()) : padVal(today.getHours()-12); var ampm = (today.getHours()<=12) ? 'AM' : 'PM'; var htmlOutput = ''; htmlOutput += hours + ':' + padVal(today.getMinutes()) + ':' + padVal(today.getSeconds()); htmlOutput += ' ' + ampm + '<br>' + daysAry[today.getDay()] + ', '; htmlOutput += today.getDate() + ' ' + mnthAry[today.getMonth()] + ' ' + today.getFullYear(); document.getElementById('live_clock').innerHTML = htmlOutput; return; } setInterval('live_clock()', 1000); window.onload = live_clock; </script> </head> <body> <div id="live_clock" align="center" class="PositiveMoney" style="background-color:#b4cbe8;max-width:150px;"> </div> </body> </html>
-
Did you try the line of code I posted? The way you had it previously you were not using the return values of the function - you were simply calling the function and the results were being thrown away. The example you just posted appear to be that the function is called within an echo statemetn so the results would be echo'd. Example: function output () { return "Hello World!"; } echo output(); //Result: Hello World output(); //Result: --NOTHING-- use the line of code I posted in my first response and I think it will work how you want it.
-
Well, if something IS being returned from that function, the code you have will do nothing with it. Are the results of that function supposed to be added to $categories->content if(isset($sub_category)){ $categories->content .= get_products($sub_category); }
-
Also, when you return back tothe index page, you might be viewign a cached page. Try refreshing to see what happens.
-
Read the manual:session_destroy In order to kill the session altogether, like to log the user out, the session id must also be unset. The very first example explains a method of how to properly destroy a session.
-
How do I assign a form drop down a variable onCHANGE
Psycho replied to kpetsche20's topic in Javascript Help
I think you are confusing server-side code (PHP) and client-side code (JavaScript). JavaScript cannot change the value of $_POST unless you submit the form or do an AJAX request. I've reread your request a couple of times and still not sure what you are trying to accomplish. When the user changes the option in the select list the value is already updated. S9o, just run the function that you want to run onchange() of that field. I *think* you may be wanting to add multiple sets of input fields based upon the select value (since the title is number of players to add). Provide more specifics for better help. -
This will ensure a properly formatted email function validEmail($emailStr) { $format_test = '/^[\w\+-]+([\.]?[\w\+-])*@[a-zA-Z0-9]{2,}([\.-]?[a-zA-Z0-9]{2,})*\.[a-zA-Z]{2,4}$/'; $length_test = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($format_test,$emailStr) && preg_match($length_test,$emailStr)); }
-
Nice find on the .hash property - wasn't aware of that one. however, that assumes you are dealing with an object with that parameter value. If the URL is just a text object, it will not have a hash property.
-
function getURLParam(URLstr) { return URLstr.replace(/.*#/, ''); } @CroNiX: This is the JavaScript forum. You posted PHP code.