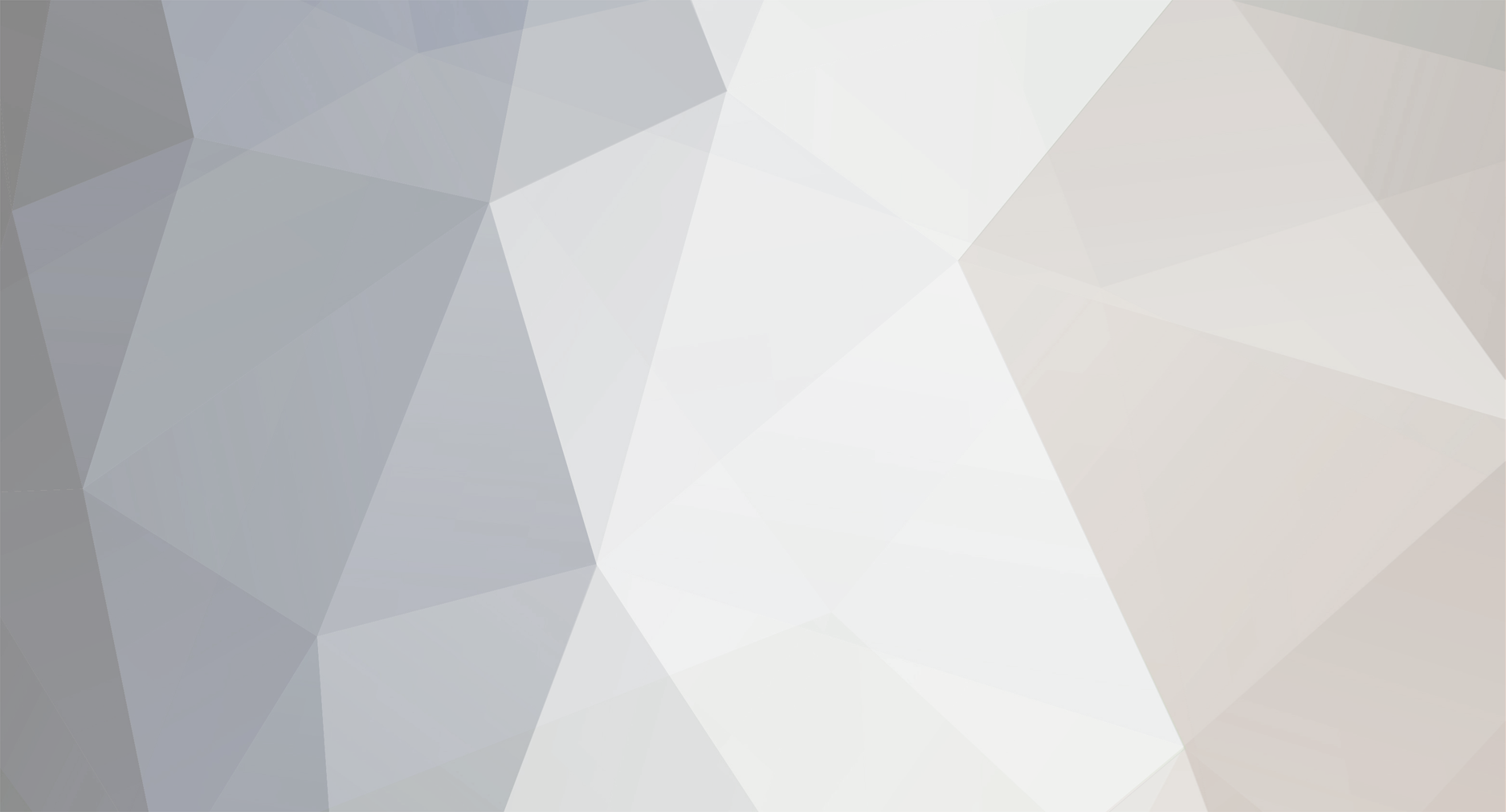
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
[SOLVED] onclick insert values on input boxes and then fill an area
Psycho replied to katerina's topic in Javascript Help
Add an ID to the span around the text: <span style="font-size: 1.5em;" id="outputText">Hello World</span><br> Then add the new code at the bottom of the function function setRGB(inputObj) { //Get the value for the object's background color and create array of RGB values var colorsAry = inputObj.style.backgroundColor.replace(/[^\d,]/g, '').split(','); //Populate the appropriate fields with the RGB values document.getElementById(inputObj.name+'R').value = colorsAry[0]; document.getElementById(inputObj.name+'G').value = colorsAry[1]; document.getElementById(inputObj.name+'B').value = colorsAry[2]; //Change the text color accordingly if (inputObj.name=='foreColor') { document.getElementById('outputText').style.color = inputObj.style.backgroundColor; } else { document.getElementById('outputText').style.backgroundColor = inputObj.style.backgroundColor; } return; } -
[SOLVED] onclick insert values on input boxes and then fill an area
Psycho replied to katerina's topic in Javascript Help
Note: there are some changes in your provided code: <html> <head> <script type="text/javascript"> function setRGB(inputObj) { var colorsAry = inputObj.style.backgroundColor.replace(/[^\d,]/g, '').split(','); document.getElementById(inputObj.name+'R').value = colorsAry[0]; document.getElementById(inputObj.name+'G').value = colorsAry[1]; document.getElementById(inputObj.name+'B').value = colorsAry[2]; return; } </script> </head> <body> <div id="color"> <div id="col"> <h2>Foreground/h2> <p> <a style="background-color:rgb(0, 0, 0);" title="#000000" name="foreColor" onclick="setRGB(this);"> <img src="images/px.gif" alt="#000000" class="px"></a> <a style="background-color:rgb(0, 0, 192);" title="#0000C0" name="foreColor" onclick="setRGB(this);"> <img src="images/px.gif" alt="#0000C0" class="px"></a> <a style="background-color:rgb(73, 108, 143);" title="#496C8F" name="foreColor" onclick="setRGB(this);"> <img src="images/px.gif" alt="#496C8F" class="px"></a> <a style="background-color:rgb(127, 0, 127);" title="#7F007F" name="foreColor" onclick="setRGB(this);"> <img src="images/px.gif" alt="#7F007F" class="px"></a> <br> <a style="background-color:rgb(255, 255, 255);" title="#FFFFFF" name="foreColor" onclick="setRGB(this);"> <img src="images/px.gif" alt="#FFFFFF" class="px"></a> </p> <p> <b> R : </b><input name="color11" id="foreColorR" maxlength="3" size="8" value="000" type="text"><br> <b> G : </b><input name="color12" id="foreColorG" maxlength="3" size="8" value="000" type="text"><br> <b> B : </b><input name="color13" id="foreColorB" maxlength="3" size="8" value="000" type="text"> </p> </div> <div id="bg"> <h2>Background</h2> <p> <a style="background-color:rgb(73, 108, 143);" title="#496C8F" name="backColor" onclick="setRGB(this);"> <img src="images/px.gif" alt="#496C8F" class="px"></a> <a style="background-color:rgb(96, 132, 164);" title="#6084A4" name="backColor" onclick="setRGB(this);"> <img src="images/px.gif" alt="#6084A4" class="px"></a> <a style="background-color:rgb(169, 192, 213);" title="#A9C0D5" name="backColor" onclick="setRGB(this);"> <img src="images/px.gif" alt="#A9C0D5" class="px"></a> <a style="background-color:rgb(255, 255, 255);" title="#FFFFFF" name="backColor" onclick="setRGB(this);"> <img src="images/px.gif" alt="#FFFFFF" class="px"></a> </p><br> <p> <b> R : </b><input name="" id="backColorR" maxlength="3" size="8" value="000" type="text"><br> <b> G : </b><input name="" id="backColorG" maxlength="3" size="8" value="000" type="text"><br> <b> B : </b><input name="" id="backColorB" maxlength="3" size="8" value="000" type="text"> </p> </div> <div id="cont"> <h2>SHOW</h2> <div id="plaisio" name="plaisio" style="background: rgb(255, 255, 255) none repeat scroll 0% 0%; color: rgb(0, 0, 0); -moz-background-clip: -moz-initial; -moz-background-origin: -moz-initial; -moz-background-inline-policy: -moz-initial;"> <p style="padding: 1.5em 0em 2em;"><br> <span style="font-size: 1.5em;">Hello World</span><br> </p> </div> </div> <p style="text-align: center; clear: both;"> <input value="check" type="submit"></p> <h2 style="clear: both;"> </h2> <p> </p> </div> </body> </html> -
Can I pass a parameter when I call a Javascript file
Psycho replied to garry27's topic in Javascript Help
Or, if you are using some type of server-side language such as PHP, then you could do something like what you proposed, you would just call a PHP page instead of a js file Example <script type='text/javascript' src='filename.php?paramname=paramvalue'></script> Then your PHP JavaScript file could look something like this: <?php echo "var paramename = {$_GET['paramname']};\n"; ?> // continue javascript code normall here ... -
Forgot to add that you will also need to do a check to ONLY do the redirect if data was submitted if (isset($_POST['submit'])) { header('Location: '.$_SERVER['PHP_SELF']); } But you should be doing that check to determine if you should process the data anyway, so you could simply add it at the end of the processing phase.
-
Well, if you want help it is extremely helpful if you 1) use code tags when posting and 2) have properly indented code to visually display the structure. In any event, the solution is still the same as I stated above. Since, you are displaying the form again after a submission you need to just reload the page WITHOUT the post data using a header() function. You will need to move all of your processing code above any output to the browser. Then after the processing is done (e.g. Inserts) and before you output anything to the page add this command: header('Location: '.$_SERVER['PHP_SELF']); The page will then reload as if no data was submitted. So the user can hit refresh as much as they like and no duplicates will be entered.
-
When the page is submitted tot he processing page, have the processing page insert the record and then immediately redirect to a confirmation page. If the user hits refresh he/she will just see the confirmation page.
-
You need to provide the code for the writelog function if you want some help with it. As to special characters to be allowed. Do you want to allow the heart symbol (♥), the inifinity symbol (∞), sigma (Σ) or how about the control characters such as new line, page break, etc. All of those (and many, many more) might be causing your problems. Without knowing exactly what the input is that is breaking the function it is impossible to know what the problem is - or what other characters might break it. Basically if you fix it for the known problem characters now, there is no guarantee there are not others that will break it.
-
Trying to create a blacklist of unsupported characters is not a good approach, IMHO, as there is always something you forget about. I think a better approach is to have a white list of supported characters. You can then strip out all unsupported characters with a simple regex expression.
-
<form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="POST"> Of course there will be more to it than that. I do not have enough knowledge of your overall process to give any specific advice. But, I typically have my forms POST to themselves so if there is an error in the submitted data I can redisplay the form to the user with the form filled with their previous data. If you have a multiple page form then I assume you are saving data in SESSION. So, have the page submit to itself. If validation fails, redisplay the form with the submitted data. If validation passes, save the data to SESSION and then do a redirect tot he next page.
-
Before submision? With PHP? You could do that with AJAX, but that wouldn't make sense. If you want to do it before submission you will want to use JavaScript, but you will also need to do it server-side in PHP in case the user has JavaScript disabled. And in that case it is typically easiest if you have your form page submit to itself for processing. Here's the JS part <html> <head> <script type="text/javascript"> function setTotal(formObj) { var total = parseInt(formObj.body.value) + parseInt(formObj.mind.value) + parseInt(formObj.soul.value); formObj.total.value = total; formObj.submit.disabled = (total!=12); var error = false; if (total==12) { error = ''; } else if (total>12) { error = " (Over by +" + (total-12) + ")"; } else if (total<12) { error = " (Under by -" + (12-total) + ")"; } document.getElementById('errorSpan').innerHTML = error; return (error==''); } </script> </head> <body> <form action="insert#.php" method="post"> <table> <tr> <td><strong>Body:</strong></td> <td><SELECT NAME="body" SIZE="1" onchange="setTotal(this.form);"> <OPTION value="1">1</OPTION> <OPTION value="2">2</OPTION> <OPTION value="3">3</OPTION> <OPTION value="4">4</OPTION> <OPTION value="5">5</OPTION> <OPTION value="6">6</OPTION> <OPTION value="7">7</OPTION> <OPTION value="8">8</OPTION> <OPTION value="9">9</OPTION> <OPTION value="10">10</OPTION> <OPTION value="11">11</OPTION> <OPTION value="12">12</OPTION> </SELECT> </td></tr> <tr> <td><strong>Mind:</strong></td> <td><SELECT NAME="mind" SIZE="1" onchange="setTotal(this.form);"> <OPTION value="1">1</OPTION> <OPTION value="2">2</OPTION> <OPTION value="3">3</OPTION> <OPTION value="4">4</OPTION> <OPTION value="5">5</OPTION> <OPTION value="6">6</OPTION> <OPTION value="7">7</OPTION> <OPTION value="8">8</OPTION> <OPTION value="9">9</OPTION> <OPTION value="10">10</OPTION> <OPTION value="11">11</OPTION> <OPTION value="12">12</OPTION> </SELECT> </td></tr> <tr> <td><strong>Soul:</strong></td> <td><SELECT NAME="soul" SIZE="1" onchange="setTotal(this.form);"> <OPTION value="1">1</OPTION> <OPTION value="2">2</OPTION> <OPTION value="3">3</OPTION> <OPTION value="4">4</OPTION> <OPTION value="5">5</OPTION> <OPTION value="6">6</OPTION> <OPTION value="7">7</OPTION> <OPTION value="8">8</OPTION> <OPTION value="9">9</OPTION> <OPTION value="10">10</OPTION> <OPTION value="11">11</OPTION> <OPTION value="12">12</OPTION> </SELECT> </td></tr> <tr><td><strong>Total:</strong></td> <td><input type="text" name="total" size="5" maxlength="2" /><span id="errorSpan"> (Under by -12)</span></td></tr> </table> <input type="submit" name="submit" value="Submit Stats" disabled="disabled" /> <input type="reset" name="reset" value="reset" /> </form> </body> </html>
-
[SOLVED] Use Javascript to replace/strip out character
Psycho replied to Jason28's topic in Javascript Help
Personally I think JavaScript and PHP are VERY similar. The function simply accepts a form field object. The function then replaces the value of that form field with the same value run through a bit of RegEx code. A PHP implementation would look similar: (not tested) $cleanValue = preg_replace('(/\*{2,}/', '*', $_POST['fieldname']); ' In fact, it is fine to have this functionality in JavaScript, but you should really be doing it on the server-side (i.e. in PHP) as users may have JavaScript disabled. -
[SOLVED] Use Javascript to replace/strip out character
Psycho replied to Jason28's topic in Javascript Help
You don't state what should happen if there are three or more asterisks in a row! I will assume that any multiple succession of asterisks (2 or more) should be replaced with a single asterisk. Here's a sample script: <html> <head> <script type="text/javascript"> function removeAst(fieldObj) { fieldObj.value = fieldObj.value.replace(/\*{2,}/g, '*'); } </script> </head> <body> Enter a value and tab out:<br> <input type="text" name="theField" onchange="removeAst(this);" /> </body> </html> -
problem in Passing arduments in javascript function
Psycho replied to ammu412's topic in Javascript Help
The problem is in your "creation" of the onChange event. The code will produce a trigger that looks something like this: onChange = 'change_host(1,host);' Where 15 is the value of the variable of num and host is the value of the variable hostF. Passing a number like that is fine. But, if 'host' is supposed to be a string then it needs to be properly formatted as such. Try this: function change_host(number,host){ alert(number+"---"+host) } function test(){ var num=1; var hostF="host"; var htmlCode = '<div id="er_clone"'+num+'" style="display:inline;font-weight:bold;color:#FF2323;font-size:15px;"></div>'; htmlCode += '<center><table class="tblheaderbg" width="200"><tr>'; htmlCode += '<th>Server Name</th>'; htmlCode += '<th>VM Machine Name</th>'; htmlCode += '<th>Vm Domain Name</th>'; htmlCode += '<th>VM IP</th>'; htmlCode += '<th>VM Net Mask</th>'; htmlCode += '<th>VM Gateway</th>'; htmlCode += '<th>VM DNS</th>'; htmlCode += '<th>Clone from template</th>'; htmlCode += '</tr><tr>'; htmlCode += '<td><select name="host_name'+num+'"'; htmlCode += ' id="host_name'+num+'"'; htmlCode += ' onChange="change_host('+num+', \''+hostF+'\');">'; newin.innerHTML = htmlCode; } -
What he (Crayon Violent) said. There is absolutely, positively no way to prevent someone from "stealing" your images. Any image a user sees in their browser has already been downloaded to their machine. It's just sitting in their cache waiting for the user to find it. Every method to prevent this has a fairly simple workaround. And, when all else fails, the user can simply press the "Print Screen" key and capture a screenshot. The best you can do is to use watermarking to prevent others from reusing your photos as trying to pass them off as their own. Just be sure to use watermarking correctly. Don't put it around the edges where it can be easily cropped. You need to ensure it is in the subject of the image and strike a balance between making the watermaking impossible (or very hard) to erradicate and not taking away from the subject of the photo.
-
Or you could simply create an array of all the values - duplicates and all - then use array_unique() $carriers = array(); foreach($response['ServiceInfo'] as $shipment) { $carriers[] = $shipment['Carrier']; } array_unique($carriers);
-
He said "unique" values: $carriers = array(); foreach($response['ServiceInfo'] as $shipment) { if($shipment['Carrier'] && !in_array($shipment['Carrier'], $carriers)) { $carriers[] = $shipment['Carrier']; } }
-
using images as radio buttons + swapping images with onClick
Psycho replied to coder1's topic in Javascript Help
1. Well, it typically helps if you put your JavaScript code within SCRIPT tags! 2. Your image names are not consistent (e.g. 'US' vs. 'USA'). 3. You are using document.images but trying to reference the id of the images instead of their names. 4. Make your image names and the values of the image tags consistent 5. make your life easier and make the images all the same type Using the code below you can add as many "options" as you want to the list and you do not need to edit the JavaScript code - just make sure the images match the value of the option. (Note I used jpg for the images as I was testing). <html> <head> <script type="text/javascript"> function checkOption(optionValue){ var cntryList = document.getElementById('countryOptions').childNodes; for (i=0; i<cntryList.length; i++) { if (cntryList[i].value) { countryID = cntryList[i].value; imgSrc = (optionValue==countryID)?'images/'+countryID+'.jpg':'images/'+countryID+'fade.jpg'; cntryList[i].src = imgSrc; } } } </script> </head> <body> <div id="countryOptions"> <img src="images/AED.jpg" name='img1' value="AED" onClick='checkOption(this.value)'> <img src="images/GBPfade.jpg" name='img1' value="GBP" onClick='checkOption(this.value)'> <img src="images/EURfade.jpg" name='img1' value="EUR" onClick='checkOption(this.value)'> <img src="images/USAfade.jpg" name='img1' value="USA" onClick='checkOption(this.value)'> </div> </body> </html> -
When I run that code, $dodge equals zero after the if statement, as it should (100 - 150 = -50, thus the if sets it to 0). Are you saying it has a null value for you?
-
var sqrFt = "6050 SQFT"; alert(sqrFt.replace(/[a-z ]/gi, '')); //Output = '6050' Although, if you ONLY want numbers then you would use something like this sqrFt.replace(/[^0-9]/g, '') Or this if you want to allow a decimal (however you would need an additional step to ensure there are not two decimals) sqrFt.replace(/[^0-9\.]/g, '')
-
difference between different page loading method.
Psycho replied to toprashantjha's topic in Javascript Help
1. history will change the page to one of the URLs in, you guessed it, your history. You can't direct the user to just any url - it has to be one in their history. 2. reload, does just that - reloads the current page 3. replace will direct the browser to the selected URL, but I believe it also replaces the current page with the new page in the browsers history, which is the opposite of #4 4. Location directs the browser to a new URL and ADDs it to the history. Example: user is on page one.htm and then clicks a link to go to page two.htm. Then on page two.htm javascriptuses replace to direct the user to page three.htm. If the user clicks the back button they will go to page one.htm. If documnet.location was used, then the back button would take the user to two.htm Again, not 100% sure on all of this (never had a need to use replace), but a quick search gave me that info. A quick test could verify. -
That doesn't make sense to me. I would think the purchase table would have a foreign key for clients, but not a foreign key for products. The products table should have a foreign key for purchases. A single purchase may have multiple products, correct? how are you storing the product id's in a single purchase record? I would think the query should look something like this SELECT * FROM clients LEFT JOIN purchases ON clients.client.id = purchases.clientid LEFT JOIN products ON purchases.purchaseid = products.purchaseid
-
Yeah, well so I made a mistake with a copy paste. So sue me.
-
Edit: neil.johnson beat me to it, but only because I was writing some code. I'm assuming the 'Image' value from the query is returning a null or empty value. So, file_exists() is returning true because the FOLDER '/images/userprofiles/' exists! While($row = mysql_fetch_assoc($Get)) { if ($row['Image']) //Ensure a value was returned { if (file_exists('/images/userprofiles/'.$row['Image'])) { echo "<img src=\"/images/userprofiles/<?php echo $Image;?>\" width=\"100px\" height=\"150px\">\n"; echo "<br>TEST<br>\n"; } else { $query = "DELETE FROM userimages WHERE RecordID='{$row['RecordID']}'"; mysql_query($query) or die(mysql_error()); } } } FYI: This makes no sense $DELETE = mysql_query("DELETE FROM userimages WHERE RecordID='$RecordID'") What do you plant to do with the variable $DELETE? Unless the query is returning data, there is no need to assign a variable to the query call. Also, I changed the script to reduce some of the variables created. In most instances it makes no sense to create a variable just to use it only once or twice (e.g. $Image & $RecordID)
-
Fine, make me think a little harder. Here's a probably better approach. When the form is submitted to the processing page, go through the normal validation procedures and if everything passes take whatever action is required (save data to DB, send email, etc.). THEN, do a header redirect to a confirmation page (e.g. "Thank you for your submission"). Then if the user refreshes the page they will just keep seing the confirmation page and the submission won't be processed again.