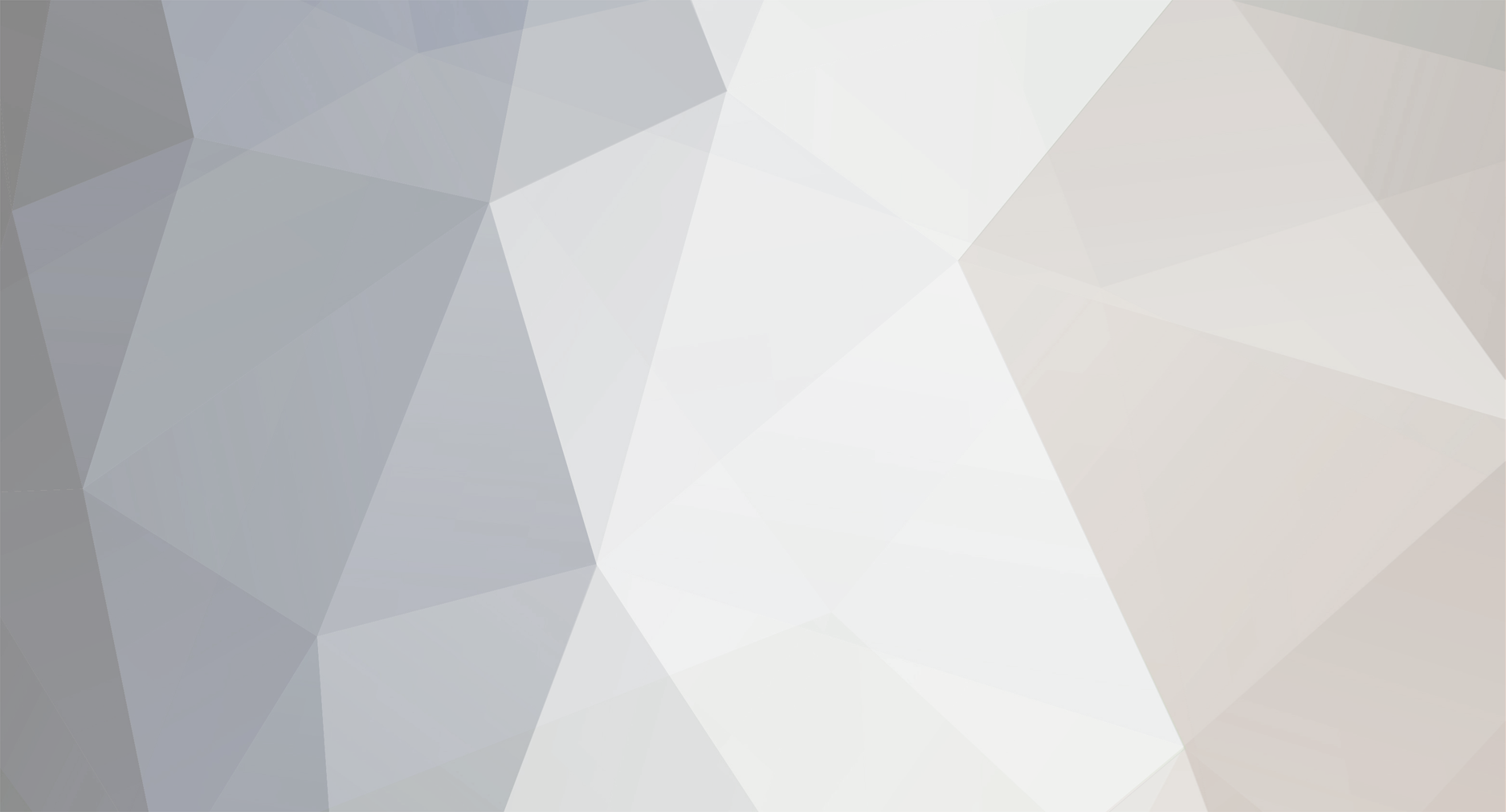
Psycho
-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Community Answers
-
Psycho's post in Date inserts 00 was marked as the answer
This 'array' makes no sense. At least I have never seen assignments used within an array like that. Since you would be overriding the assignments on each iteration of the loop it makes even less sense why you would have assignments (since they are not used).
$stmt->execute(array( $pastorname = $_POST['pastorsname'][$i], $ministryname = $_POST['ministriesname'][$i], $startdate = "'".date('Y-m-d H:i:s', strtotime(str_replace('-', '/', $_POST['xstartdate'][$i])))."'", $clientsname = $_POST['clientname'][$i], $video_url = $_POST['url'][$i], )); But, part of your problem is that you are appending a single quote mark to the beginning and end of the date string. You don't quote the values that will be used in prepared statements. Try this:
for ($i = 0; $i < count($_POST['pastorsname']); $i++) { //Create date string $startdate = date('Y-m-d H:i:s', strtotime(str_replace('-', '/', $_POST['xstartdate'][$i]))); echo "Input: {$_POST['xstartdate'][$i]}, Start date: {$startdate}<br>\n"; //For debugging //Create values array $values = array( $_POST['pastorsname'][$i], $_POST['ministriesname'][$i], $startdate, $_POST['clientname'][$i], $_POST['url'][$i] ); //Execute statement $stmt->execute($values); } Does it work (without the extra quotes)? If not, what does the output show?
By the way, you should create your fields something like this
name="record[1][pastorsname]" Then, in your code you would just do
foreach($_POST['record'] as $record) { //Fields are then referenced like this //$record['pastorsname'] //$record['ministriesname'] } -
Psycho's post in complex php project was marked as the answer
Um, 'complex' is a subjective term. When I think 'complex', I am thinking about projects that require a team developers, DBAs, QA analysts, business analysts, etc. etc. I'm guessing that is not the type of 'complex' project you are wanting to do.
My best advice is find something to do in an area that you enjoy. If you do that, it will help you stay motivated. If you pick something you have no interest in, you aren't going to be too interested in writing the code for it either. Some examples:
If you are into computer gaming, build a site that gathers data from servers that run a particular game to show metrics, player standings, or other data of interest to people that play that game. Or perhaps, allow players to sign up with the game id so they can show metrics between the players.
Do you like photography? Build a site that allows a person to upload their geotagged photos and provide a world map (using Google's APIs) with markers where the different photo's were taken. User can click a location and see the photos the person took. Actually, this would be cool for people that travel a lot.
Are you into sports? Find a feed with data for a particular sport and present the data in different ways that users would like or maybe add some prediction logic. E.g. probability of a player hitting a home run based on results of his last x games.
Etc. Etc.
-
Psycho's post in Prepare statement query not executing was marked as the answer
Running loops and doing a select statement each time [especially with rand()] is a bad idea. here is what I think is a better approach.
1. Run ONE SELECT query to get all of the vtext values from the rsample table for the groupname
2. Populate those results into an array
3. User array_shuffle() to randomize the results of the array
4. Run the loops for $i & $j like you have now, but create a simple INSERT statement like this
INSERT INTO decks (id, dnumber, vtext) VALUES (:id, :dnumber, :vtext) 5. Define the vtext value on each loop using array_shift() which will remove and return the first value in the array
$stmt -> bindValue(':vtext', array_shit($vtextValuesAry)); -
Psycho's post in Display an image a certain number of times depending on a value in database was marked as the answer
Instead of creating a loop, a more elegant approach might be to use the str_repeat() function.
Create a variable to hold the content for a star image element and then just use str_repeat() with that variable and the rating number to create the correct output.
So, somewhere before the loop to create the output for the records define the image
$starImg = "<img src='images/star.jpg'>"; Then within the loop for the records, use a single line to create the correct number of images using the rating number from the query results
echo str_repeat($starImg, $game_rs['rating_number']); -
Psycho's post in calculation in a query was marked as the answer
Your table structure appears to be malformed. The last column appears to be the player name which is associated with the player id in the first column. Plus, the gme column appears to be the "name" of a particular match.
First, the Player info should be in its own table and you would then only reference the player ID in the table for the match results info. Second, the matches should also be in their own table with unique IDs as well. Then you would reference the match ID - not the name in the match results table (what would happen in your current structure if the same two teams played each other twice?). Third, you should also have another table to store the teams as separate entities.
Here is a sample structure:
Teams TeamID | TeamName ---------------------- 1 CRYSTAL SA 2 Sebowa FC Players PlayerID | PlayerName ------------------------ 1 Sam Lukoye 3 Mulan Babu 13 Doughlas Doughlas 9 Owori Clinton 5 Zimbe Zimbe Matches MatchID | HomeTeamID | VisitingTeamID | MatchDate -------------------------------------------------- 2 1 2 2017-08-14 MatchResults
MatchID | PlayerID | Goals -------------------------- 2 1 4 2 3 3 2 13 0 2 9 0 2 5 0
With your current structure (which I don't advise) you can get both the total goals for a match as well as the individual totals/percentage with a single query by using the "ROLLUP" modifier. It will create a subtotal after each GROUP BY parameter.
Query
SELECT r1.gme, r1.player_id, SUM(r1.goals) as player_goals, SUM(r1.goals) / (SELECT SUM(goals) FROM results r2 WHERE r1.gme = r2.gme) as player_perc FROM results r1 GROUP BY r1.gme, r1.player_id WITH ROLLUP
Example Output
gme | player_id | player_goals | player_perc ----------------------------------------------------------------- CRYSTAL SA Vs Sebowa FC 1 4 .57 CRYSTAL SA Vs Sebowa FC 3 3 .43 CRYSTAL SA Vs Sebowa FC 13 0 0 CRYSTAL SA Vs Sebowa FC 9 0 0 CRYSTAL SA Vs Sebowa FC 5 0 0 . . . NULL NULL 7 1 -
Psycho's post in Best way to do this... was marked as the answer
Here's one solution:
First create a query using GROUP_CONCAT() to derive the device combinations for each user ID. Then use that query as a sub-query source to determine the count of users that have each unique device configuration.
SELECT COUNT(UserID) as UserCount, ConfigType FROM ( SELECT UserID, GROUP_CONCAT(`DeviceType`) as ConfigType FROM votes GROUP BY UserID ) as DerivedTable GROUP BY ConfigType -
Psycho's post in Multiple drop down was marked as the answer
You will want to implement an AJAX solution. This is sometimes called a "chained select" implementation. You can google that for some possible solutions. Here are the basics.
Implement JavaScript triggers on the Select lists that are parents for the onChange event Whenever a parent select list is changed call a function to: Pass the currently selected value via AJAX to get the list of applicable options for the immediate child select list Empty out the options from the child and grandchildren select lists, then replace the options for the immediate child element You need to decide whether you want a "select one" as the default option when populating the options of a child element (I would) or if you want to default to the first valid value. If you do the latter, you need to run the same process on that child element, and the next (recursively), until all parent/child elements are run.
-
Psycho's post in how to group results was marked as the answer
Use GROUP BY. You need to group by all the unique fields - i.e. those that are not included in aggregate functions such as SUM()
SELECT houseid as P_id, ShortName, logo, SeasonName, SUM(Apps) as Apps1, SUM(Gl) as Gls FROM tble_stats GROUP BY houseid, ShortName, logo, SeasonName
You state you want the values for each houseid and Shortname. But, you also have logo and SeasonName - so you would need to group by those as well in case they can be different for the same houseid/Shortname values. If they cannot be different - then your structure is wrong.
-
Psycho's post in Use last login as a condition - how? was marked as the answer
My guess is that you are making things more difficult than they need to be. I'm curious what value you are changing and why. In the vast majority of instances business rules can be performed in "real time" without needing to reset data. As to your question, just put a caluse on the update query to not perform the change to users based on thier login date
UPDATE table_name SET some_field = :new_value WHERE last_login > DATE_SUB(NOW(), INTERVAL 10 DAY) -
Psycho's post in Creating a complicated registrationsystem - how? was marked as the answer
rand() and str_pad().
Use rand() to generate a random number between 1 and 9999 (or 999999) then use str_pad() to add 0's to the left if the result is less than 4 (or 6) characters.
-
Psycho's post in Array to string conversion error when retrieving data from DB(MySQL) was marked as the answer
$statement->execute([$userID]); What IS the variable $userID? If it is already an array, then putting it in [] (brackets) would make it a multi-dimensional array (and possibly cause an array to string conversion error)
What does this output?
var_dump([$userID); -
Psycho's post in Is there a way to use multiple countdown counters on same page? was marked as the answer
<html>
<head>
<script>
//Create object with the list of due dates
//The 'name' will correspond to the field ID to populate the results
var dueDates = {
'date1':'2017-03-03 02:16:02',
'date2':'2017-05-05 19:01:58',
'date3':'2017-06-05 05:32:33'
};
var timer = setInterval(function() {
//Instantiate variables
var dueDate, distance, days, hours, minutes, seconds, output;
//Set flag to repeat function
var repeat = false;
// Get todays date and time
var now = new Date().getTime();
//Iterate through the due dates
for (var dueDateId in dueDates)
{
//Get the due date for this record
dueDate = new Date(dueDates[dueDateId]);
// Find the distance between now an the due date
distance = dueDate - now;
// Time calculations for days, hours, minutes and seconds
days = Math.floor(distance / (1000 * 60 * 60 * 24));
hours = Math.floor((distance % (1000 * 60 * 60 * 24)) / (1000 * 60 * 60));
minutes = Math.floor((distance % (1000 * 60 * 60)) / (1000 * 60));
seconds = Math.floor((distance % (1000 * 60)) / 1000);
//Determine the output and populate the corresponding field
output = "OVERDUE";
if (distance > 0)
{
output = days + "d " + hours + "h " + minutes + "m " + seconds + "s";
repeat = true; //If any record is not expired, set flag to repeat
}
document.getElementById(dueDateId).innerHTML = output;
//If flag to repeat is false, clear event
if(!repeat)
{
clearInterval(timer);
}
}
}, 1000);
</script>
</head>
<body>
Date 1: <div id="date1"> </div><br>
Date 2: <div id="date2"> </div><br>
Date 3: <div id="date3"> </div><br>
</body>
</html>
-
Psycho's post in How to extract date from UTC? was marked as the answer
Where are you getting the value '2015-11-17T00:00:00+00:00' from? That's not the format I would expect from a DB query or a timestamp generated from PHP.
Anyway, give this a try
//Create new datetime object as a UTC time value $date = new DateTime('2015-11-17T00:00:00+00:00', new DateTimeZone('UTC')); //Set the timezone for the output and format it $dateOuptut = $date->setTimezone(new DateTimeZone('Europe/London'))->format('j M Y'); //Output the value echo $dateOuptut; NOTE: I added a time to the output to test and for "America/Chicago" it showed the value that I would expect (UTC - 6 hours), but from what I found London should be UTC + 1 and I was not getting those results. You could always artificially add an hour to the input time.
-
Psycho's post in CSS Active Dropdown was marked as the answer
OK, I gave you a lot of information, so let's take it one step at a time.
1. Your main problem was that the function Active() was either applying the 'dropdown' class OR the 'active' class. The dropdown class is the one that applied the styles which create the dropdown affect. So, if you only apply the 'Active' class you lose the dropdown affect. You can apply multiple classes to the same element by separating them with spaces. So, you want to always apply the 'dropdown' class to all of the relevant element and add the 'active' class only to the one where it applies.
2. Even if you were to properly apply the classes per #1 (Inactive class="dropdown" or Active class="dropdown active"), the definition for the 'active' class would do nothing because it is applied to the <li> element. The background colors you are seeing are due to styles applied to the anchor tags (<a></a>) not the <li> tags. That can be easily fixed, by changing the definition for active in the stylesheet to:
li.active a { background-color: #990000; } What that means is for any <li> elements with the 'active' class, find any anchors (<a></a>) and apply the formatting.
Those two things alone will directly fix your problem. But, your HTML follows a predictable format. Rather than copy/paste code and change labels, hrefs, etc. - create the content with a function. That way you know you won't have any copy/paste errors. This is especially helpful when you need to make changes to the format. So, I suggest creating an array that defines the menu (the parent links and the child links), then creating a function to produce the HTML output. This way you can easily add/edit/modify the menu links by just changing the array - which you can use as an include file. You would not have to modify core code to change the menu. Or, if you want to change the menu structure you just need to change the function. So, I provided an array for the current menu items you had as well as a function to create the menu - as well as fix the above issues.
Here is a working script with all the pieces
<?php //hardcoded $webpage value for testing purposes $webpage = "drivers.php"; //definition of available menu $menuLinks = array( 'Home' => array( 'href' => 'menu.php' ), 'Territory Manager' => array( 'href' => 'tms.php', 'children' => array ( 'Add TM' => '#', 'Search TM' => 'search.php?search=TM' ), ), 'Sales' => array( 'href' => 'sales.php', 'children' => array ( 'Add Sales Person' => '#', 'Search Sales' => 'search.php?search=sales' ), ), 'Drivers' => array( 'href' => 'drivers.php', 'children' => array ( 'Add Driver' => '#', 'Search Drivers' => 'drivers.php' ), ), 'Passengers' => array( 'href' => 'passengers.php', 'children' => array ( 'Add Passenger' => '#', 'Search Passengers' => 'search.php?search=passengers' ) ) ); //Function to create html content for menu function createMenu($menuLinks, $webpage=false) { $menuLinksHtml = ''; foreach($menuLinks as $parentLabel => $parentLink) { //Determine link if parent is active or not $active = ($webpage == $parentLink['href']) ? ' active' : ''; $menuLinksHtml .= "<li class=\"dropdown{$active}\">\n"; //Determine if parent link has children if(!isset($parentLink['children'])) { //No child elements $menuLinksHtml .= "<a href='{$parentLink['href']}'>{$parentLabel}</a>\n"; } else { //Has child elements $menuLinksHtml .= "<a href=\"javascript:void(0)\" class=\"dropbtn\">{$parentLabel}</a>"; $menuLinksHtml .= "<div class=\"dropdown-content\">\n"; foreach($parentLink['children'] as $childLabel => $childLink) { $menuLinksHtml .= "<a href=\"{$childLink}\">{$childLabel}</a>\n"; } $menuLinksHtml .= "</div>\n"; } $menuLinksHtml .= "</li>\n"; } //Return HTML content return $menuLinksHtml; } ?> <html> <head> <style> /***** Begin Menu CSS *****/ ul { width: 100%; list-style-type: none; margin: 0; padding: 0; overflow: hidden; background-color: #333; } li { float: left; } li a, .dropbtn{ display: inline-block; font-size: 15px; color: white; text-align: center; padding: 14px 16px; text-decoration: none; border-right: 1px solid #bbb; } /* Color of the main menu text when hovering */ li a:hover { background-color: red; } /* Once the mouse has moved off the main menu button and is now highlighting a sub menu button, this defines what that main menu button color is */ .dropdown:hover{ background-color: red; } /* Color of main menu button when not selected */ .dropbtn { background-color: 333; } li .dropdown { position:relative; display: inline-block; } li:last-child { border-right: none; } .dropdown-content{ display: none; position: absolute; background-color: #f9f9f9; min-width: 160px; box-shadow: 5px 7px 5px 0px rgba(0,0,0,0.2); z-index: 1; } /* Links inside the dropdown */ .dropdown-content a{ color: black; padding: 12px 16px; text-decoration: none; display: block; text-align: left; background-color: #f6f6f6; /* Sets background color of the drop down menu (not selected) */ } /* Change color of dropdown links on hover */ .dropdown-content a:hover {background-color: #ccc} .dropdown:hover .dropdown-content{ display: block; } /* I have no idea what this does as it appears nothing... li a:hover:not(.active) { background-color: #blue; } */ li.active a { background-color: #990000; } .active dropdown-content{ display: none; position: absolute; min-width: 160px; box-shadow: 5px 7px 5px 0px rgba(0,0,0,0.2); z-index: 1; } .active dropdown-content a{ color: black; padding: 12px 16px; text-decoration: none; display: block; text-align: left; } /***** End Menu CSS *****/ </style> </head> <body> <ul> <?php echo createMenu($menuLinks, $webpage); ?> </ul> </body> </html> EDIT: Removed the Active() function that is not used in my code.
-
Psycho's post in Queries equal same amount of rows but should not was marked as the answer
I'm making some assumptions here, but I would think that a vote should indicate a vote for or against the issue. But, your logic seems to imply that votes are only to indicate a "for" vote and all users must for for in order for it to pass. That seems like odd logic. Even if a unanimous decision is required, you should have some allowance for when someone doesn't cast a vote.
Plus, you can easily determine 1) How many voters there are, 2) How many users have votes, and 3) The results of the vote with a single query. You don't state whether the votes table has an identifier for the user who casts a vote - but it should.
Query
SELECT COUNT(u.user_id) as users, COUNT(v.vote_id) as votes, (COUNT(u.user_id)=COUNT(v.vote_id)) as passed FROM votes v RIGHT JOIN users u ON v.user_id = u.user_id AND v.motion_id = :motion_id Results would look something like this (3 of 5 users have voted)
users | votes | passed 5 3 0 Or this (5 of 5 users have voted)
users | votes | passed 5 5 1 -
Psycho's post in substitute array keys was marked as the answer
Change
echo "$row<br>"; To
echo "{$d[$row][0]}<br>"; -
Psycho's post in Math From Filesize was marked as the answer
Here's a class to get the duration of an MP3 file from the header information.
http://www.zedwood.com/article/php-calculate-duration-of-mp3
-
Psycho's post in input select field not working was marked as the answer
What "problem" are you having - exactly. What IS getting saved in the database? Or, are you getting errors? If so, what are they?
Here are a few things you should verify:
1. Do a View Source on the form page and inspect the Select Options are properly formatted and that their values are the IDs that you expect.
2. Run this in the code the handles the form submission to verify the complete and correct data is being submitted
echo "<pre>".print_r($_POST, 1)."</pre>";
3. echo the query tot he page to ensure it is correct. Also, you can copy/paste the derived query into your database management console (e.g. PHPMyAdmin) to verify it is valid and see if there are any errors. Pro tip: Create and test your queries in a management console first (with hard coded data). Once you have them working as you want - then put them in your code replacing with the dynamic values.
NOTE 1: Your code is wide open to SQL injection. You should be using prepared statements.
NOTE 2: Who are you to say what characters may be in a person's name? What about a person with a hyphentated name "Julie Brown-Smith" or what about someone with diacritic characters in their name: "Robert Muñoz"
-
Psycho's post in Trying to add days to a date, failing miserably.. was marked as the answer
I don't think this will work:
$del_tim_holder_1[count($del_tim_holder_1)] Assuming a zero based index, an array with a count of '3' would have indexes of '0', '1', & '2'. There would be no value with an index of '3'. You could instead use array_pop or, instead of having to use split/explode first to create an array, just use string functions (see example below).
Also, rather than only put in the logic for the interval in the function, I would suggest just passing the $del_tim_holder_0 value and have the function do all the logic to return the applicable date string.
private function calculateDate($intervalStr) { //Get value following last ':' and ensure a number $inervalWeeks = intval(substr(strrchr($intervalStr, ":"), 1)); //Convert number to weeks $intervalDays = $inervalWeeks * 7; //Create a date intervale $interval = new DateInterval("P{$intervalDays}D"); //Create current date object $currDate = new DateTime(date("d.m.Y")); //Apply the interval and convert to string $newDate = $currDate->add($interval)->format('d.m.Y'); return $newDate; } $del_tim_holder_0 = "15:18:21"; $this->date = $this->calculateDate($del_tim_holder_0); // $this->date = '13.07.2017' -
Psycho's post in Form Validation not working was marked as the answer
I'll just say that what you are doing is probably not needed and (if you don't have similar validation on the back end) completely useless.
However, your problem is that your current "test" is trying to see if the value contains the entire string of "!\"·$%&/()=?¿@#¬". You need to create a regular expression patter to look for any character.
Plus, I suspect one of your other lines in the failure scenario is failing, probably this one
document.signup.char_name.focus();
You should pass the form element by reference to the function instead of using getElementById()
Also, you should use the onchange event - otherwise the logic will get caught in an infinite loop. When the alert is displayed, the act of the user clicking the OK will initiate another onblur event.
function checkInput(inputObj) { var user_input = inputObj.value; var notgood = /[!"·$%&\/()=?¿@#¬]/; if ( !notgood.test(user_input) ) { document.getElementById('submitlink').removeAttribute('disabled'); document.getElementById('badInput').innerHTML = ''; return true; } else { document.getElementById('submitlink').setAttribute('disabled','disabled'); document.getElementById('badInput').innerHTML = 'Bad Input'; alert('Cannot use that character'); inputObj.focus(); return false; } }
<input type="text" name="char_name" value="" id="char_name" required="" onchange="checkInput(this);"/> -
Psycho's post in Send multiple rows to one email was marked as the answer
1. Why are you using LEFT JOIN? Are there bills with no associated users? If so, there would be no email address to send anyway. Don't use LEFT/RIGHT JOINs unless you need to. They are inefficient.
2. No need to create a prepared query if there are no variables to be applied
3. There is a "class" used in one of the divs. I don't see how it would have a purpose in an email
4. $row_sent has no purpose
5. Don't use SELECT * - only select the data you need
6. You have invalid HTML in the output. The following exists between TR elements
<h3>Yours<br> Coy Name</h3> There's a random "</h4>" tag
There's opening tags without corresponding closing tags
Etc.
Pro Tip: Create organized code that separates different logic so it is easy to maintain.
##--------------------------## ## Prepare the mailer class ## ##--------------------------## //$message = htmlspecialchars($mess); $mail = new PHPMailer; //$mail->SMTPDebug = 3; // Enable verbose debug output $mail->isSMTP(); // Set mailer to use SMTP $mail->Host = 'localhost'; // Specify main and backup SMTP servers $mail->SMTPAuth = true; // Enable SMTP authentication $mail->Username = 'mails@mail.com'; // SMTP username $mail->Password = 'pasword'; // SMTP password $mail->SMTPSecure = 'ssl'; // Enable TLS encryption, `ssl` also accepted $mail->Port = 465; // TCP port to connect to $mail->From = 'no_reply@noreply.com'; $mail->FromName = 'Coy Name'; $mail->WordWrap = 587; // Set word wrap to 50 characters $mail->AddEmbeddedImage("../img/logo.png", "logo"); $mail->isHTML(true); // Set email format to HTML $mail->Subject = 'Notice'; ##----------------------## ## Create and run query ## ##----------------------## $bill_status = "OVERDUE"; $query = "SELECT username, trans_ref, due_date, service_provider, service_type, amount_paid, bill_status, recurring, DATEDIFF(NOW(), due_date) AS days_diff FROM bills JOIN login_details ON bills.user_id = login_details.user_id WHERE bills.bill_status = ?" $st = $pdo->prepare($query); $st->execute([$bill_status]); ##--------------------------------------------------## ## Loop through records and create structured array ## ##--------------------------------------------------## while($row = $st->fetch(PDO::FETCH_ASSOC)) { $records[$rows['username']][] = $row; } ##-----------------------------------------------------## ## Iterate over records sending one email to each user ## ##-----------------------------------------------------## foreach($records as $username => $userRecords) { //Create message header $message = " <div class='messages'> <h3><img src='cid:my_logo'></h3><br> <div style='font-size:15px;'>Email Notification on Bill Due for Payment <p>Dear: Customer</p> <p>The following bills are due for payment:</p> <table width='80%' border='0' cellspacing='0' cellpadding='0'> <tr style='font-weight:bold;'> <td>Trans Ref</td> <td>Due Date</td> <td>Days Overdue</td> <td>Service Provider</td> <td>Service Type</td> <td>Amount Paid</td> <td>Bill Status</td> <td>Recurring</td> </tr>"; //Iterate over all records for the user foreach($userRecords as $record) { $message .= "<tr>"; $message .= " <td>".$rows['trans_ref']."</td>"; $message .= " <td>".$rows['due_date']."</td>"; $message .= " <td>".$rows['days_diff']."</td>"; $message .= " <td>".$rows['service_provider']."</td>"; $message .= " <td>".$rows['service_type']."</td>"; $message .= " <td>".$rows['amount_paid']."</td>"; $message .= " <td>".$rows['bill_status']."</td>"; $message .= " <td>".$rows['recurring']."</td>"; $message .= "</tr>"; } //Add message closing tags $message .= "</table>"; $message .= "</div>"; $message .= "</div>"; //Send the email to the current user $mail->addAddress($username); $mail->Body = $message; $mail->send(); $mail->ClearAddresses(); } -
Psycho's post in Change background color based off value returned was marked as the answer
Create classes for the different colors you want. Then have the logic determine which class to use. Or, you can make this much easier by just creating styles named for the brands and then use the dynamic name in defining the class to use. No additional coding needed to set the color:
<?php //Test values $row_rsStockDynoFk['vs_brand'] = "Honda"; $row_rsStockDynoFk['vs_yrmodsize'] = "Crx"; ?> <html> <head> <style> .brandHonda { background-color: #FFD9D5; } .brandHusky { background-color: #FED6F5; } .brandKawasaki { background-color: #D6F5D3; } .brandKTM { background-color: #FFE4CA; } .brandSuzuki { background-color: #FFFFD5; } .brandTM { background-color: #D5FFFF; } .brandYamaha { background-color: #DFDFFF; } </style> </head> <body> <div class="lvl1-4-5 brand<?php echo $row_rsStockDynoFk['vs_brand']?>"><?php echo $row_rsStockDynoFk['vs_brand']; ?></div> <div class="lvl1-4-6"><?php echo $row_rsStockDynoFk['vs_yrmodsize']; ?></div> </body> </html> -
Psycho's post in Error when checkbox is checked. was marked as the answer
You need to give your form fields meaningful names. "checkbox" is not a good name for a form field.
One glaring problem is that you have multiple fields with the same name
-
Psycho's post in get 10 records from each category was marked as the answer
I did say that code was not tested. I don't have your database or other required files to run that code. I expect you to check for any typos I made and fix them or provide the errors and I can assist.
Well, your current query is sorting by p.id. If you want things to be grouped by category then you need to put logic in place to do that. The logic in the code I provided previously would do that. But, even once we get that working, you have another issue. If you want all the categories to be returned with only 10 products per category, you are going to need a somewhat more complex query. Give me some time. If you can provide a dump of the relevant tables, then I could actually test the code I provide.
-
Psycho's post in Pictures rotating 90 degrees was marked as the answer
Have you tried displaying BOTH the original image and the resized images? My guess is that the original image will display with the correct orientation, but the resized image will not because the original image has the required EXIF data that the browser is applying while the resized image does not. The user manual for imagecreatefromjpeg() has the following user contributed note:
Even though an image displays correctly on the iPhone does not mean it is really saved that way. The camera has a specific orientation and I suspect all images are saved with that default orientation. All the rotation implementation is likely just software based.
For a PHP application, I would not rely upon EXIF information to determine how an image is displayed. I would instead convert the image (as the example with that note would do) so it does not depend on rotation. The reason is if you need to do any logic on the size of the image it would be more complicated. For example, if you will create the output differently based on the width of an image you would have to first check the EXIF data to determine if you should check the "width" or the "height" of the image based on what is the real width when displayed
Since you already have a process to resize the original image go ahead and rotate the new image accordingly. If you also save the original image, then you should replace it with one that is not rotated as well.