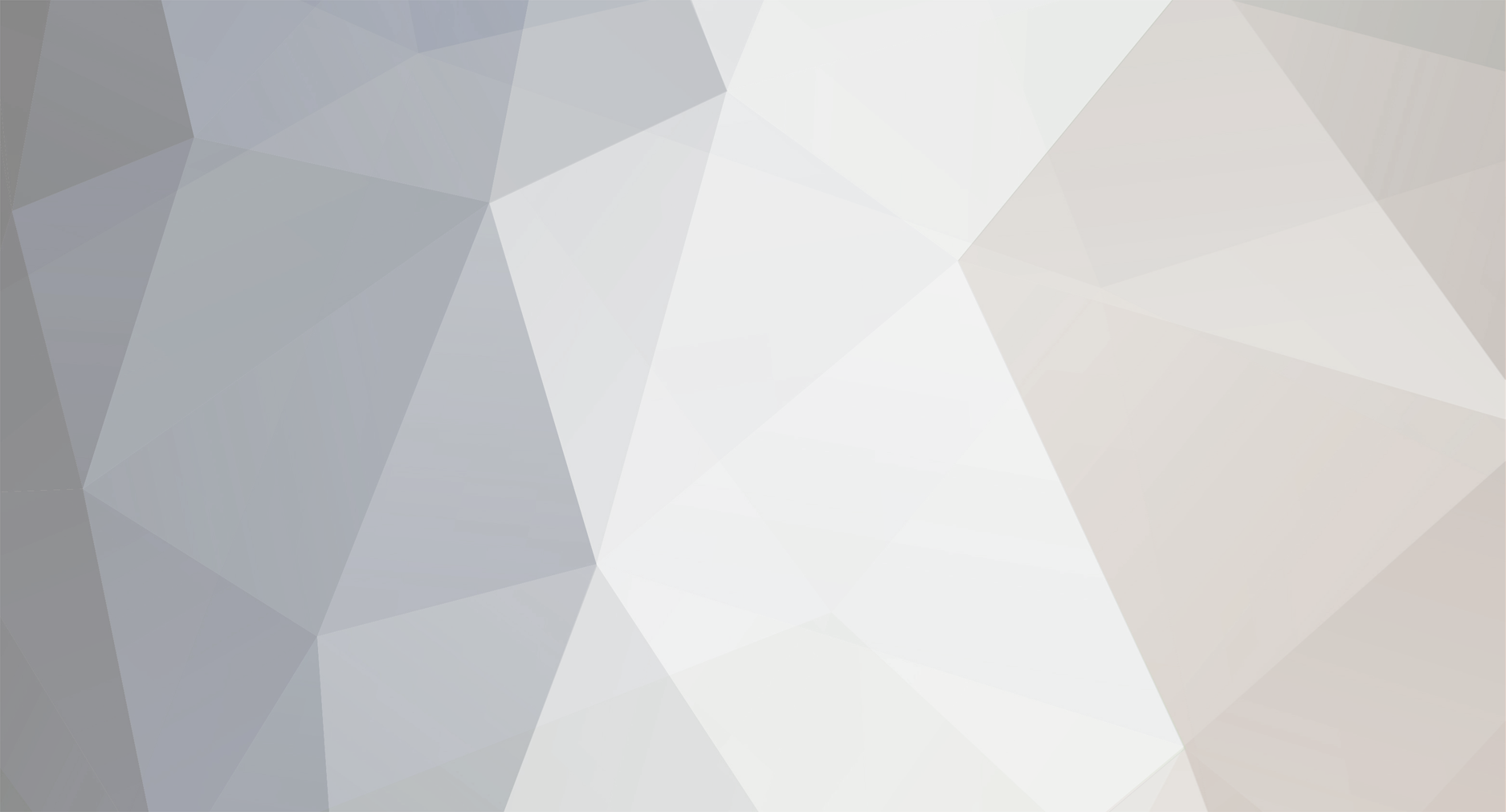
Synergic
-
Posts
21 -
Joined
-
Last visited
Never
Posts posted by Synergic
-
-
anyone? been stuck on it for days....
-
I have a web service which i want to consume via PHP.
Whenever i attempt to communicate with it it comes up with:
"Server was unable to process request. --> Object reference not set to an instance of an object."
Googled it and got the following:
http://php.net/soap_soapclient_soapcall
Apparently this code should work to fix the above problem:
<?php class Test { public $account; public $password; } $parameters = new Test; $parameters -> account = $username; $parameters -> password = $password; try { $client = new SoapClient ("https://www.somewebsite.com/Serv ice.asmx?wsdl", array('classmap' => array('CheckUser' => 'Test'))); $client -> CheckUser ($parameters); echo "Valid Credentials!"; } catch (Exception $e) { echo "Error!<br />"; echo $e -> getMessage (); } ?>
I modified it and my version as follows:
<? class Book { public $author = "1"; public $title = "2"; public $publisher= "3"; public $loc= "4"; } $bookC = new Book; $sc = new SoapClient("URLTOWEBSERVICE?wsdl", array('classmap' => array('bookC ' => "Book"))); try { print_r($sc->getBook($bookC)); } catch (Exception $e) { echo $e->getMessage(); } WSDL as follows: - <s:complexType name="Book"> - <s:sequence> <s:element minOccurs="0" maxOccurs="1" name="author" type="s:string" /> <s:element minOccurs="0" maxOccurs="1" name="title" type="s:string" /> <s:element minOccurs="0" maxOccurs="1" name="publisher" type="s:string" /> <s:element minOccurs="0" maxOccurs="1" name="loc" type="s:string" /> </s:sequence> </s:complexType>
Been stuck on it for a while, any ideas?
I've also tried using nuSoap with the same result. :|
-
$months[01] = "Jan"; $months[02] = "Feb"; $months[03] = "Mar"; $i = 02; // also tried "02" echo $months[$i];
The above code has me stumped (echos nothing). Coming from a Java background I've had no such troubles substituting array indices with variables. When i change the $i (in between the brackets) with 02 it appears to work. Any ideas?
-
thanks alpine,
i figured it out from your code. -
I have a drop down menu, i also have a value so when the form is loaded i want to select the drop down menu item based on the string. I tried all combinations and i just can't get it to auto select the drop down menu item based on the string (they're EXACTLY the same) any ideas?
-
heh whoops yes it works now:
[code]
$a = 10/100;
$b = 1000;
$b = $a*$b;
echo $b;
[/code] -
i don't know why the following isn't working. I'm trying to calculate 10% of a value?
[code]
$a = 10/100;
$b = 0;
$b = $a*$b;
echo $b;
[/code]
it just gives me 0 :|
-
ok solution was to:
[code]
$sql = "INSERT INTO userinfo VALUES ('','".$user->getUserName()."')";
[/code]
??? -
and if i pass a $user object with a username, password i can print it within the function, it works. However if i use it in the insert statement i get undefined variable:
$sql = "INSERT INTO birthdays (name, birthday) VALUES ('$user->getUserName()', '$birthday')"; -
actually i still want to use OO concepts. i still can't figure out why the original code doesn't work. If i remove the variables and insert values instead it works no problems. The funny thing is if i declare the variable locally and use it, it works.
-
i haven't figured why i'm getting unidentified variable with the following insert statements. i mean using values work fine but as soon as i stick a variable inside the insert clause i get unidentified variable:
[code]
<?php
include_once 'DatabaseClass.php';
class BookStoreClass extends DatabaseClass {
var $name = 'hello';
var $birthday = 'hello';
function registerUser($user)
{
parent::connect();
mysql_query ("INSERT INTO birthdays (name, birthday) VALUES ('$name','$birthday')");
parent::close();
}
}
?>
[/code]
Notice: Undefined variable: name in
Notice: Undefined variable: birthday in -
As part of making my code more elegant and following an mvc pattern i want to store a query result inside a variable where i can invoke it else where besides the presentation layer.
I've tried:
[code]
$result = mysql_fetch_array($quer);
while($result) {
}
ends up being an endless loop. Do i need to continue to update the variable as i scroll through the rows?
[/code] -
nerver mind, my bad :) thanks for the help.
-
[quote author=ToonMariner link=topic=104209.msg415452#msg415452 date=1155565618]
Don't!
Just have a table of artist and a field for their publisher
[/quote]
for simplicity i have kept things in one table. i will fix the structure once i've figured this problem. -
Ok i've got a CD catalog in a database. I have a cd table in the cd table i have a column for the artist, song and publisher. What i want to do is list each artist under their publisher so something like
Sony/BMG
Artist 1
Artist 2
Universal
Artist 1
Artist 2
I'm just having trouble approaching the problem of printing it out. How do i populate each artist under their respective publisher? -
ok fixed, the problem was 'cart' was an object i got confused with 'it' being an array. I accessed the array inside [i] cart [/i] which is [i] items [/i] and recieve a 0 :)
-
[code]
<? if (isset($_SESSION['cart']))
{
echo 'Array is set.';
} ?>
<?echo '<pre>';
print_r( $_SESSION );
echo '</pre>';?>
[/code]
result:
[code]
Array is set
Array
(
[cart] => ShoppingCartClass Object
(
[items] => Array
(
)
[totalPrice] =>
)
)
[/code]
what gives? :-\ -
yeah but if i add something to the array it gives me 1. It's hard to tell if its really empty or not...
-
why do both these functions:
sizeof -- Alias of count()
count -- Count elements in an array, or properties in an object
give an array size of 1 even though there's nothing inside it?
I then add something to it and it gives me 1 still then 2. what is in index 0? i need to do some manipulation and have to check if an array is empty. -
I'm creating a simple, non-presistent shopping cart. Currently it allows a product ID and a qty. What i want is without re-writing the code below a simple way of adding a third variable such as a description. Any ideas? Since the array only accepts one associated field inside it i can't seem to figure how to add a third attribute.
[code] <?php
class ShoppingCart {
var $items;
function add_items($product_id, $qty, $desc)
{
$this->items[$product_id]=$qty;
}
function update_items($product_id, $qty)
{
if(array_key_exists($product_id, $this->items))
{
if($this->items[$product_id]>$qty)
{
$this->items[$product_id]-=($this->items[$product_id]-$qty);
}
if($this->items[product_id]<$qty)
{
$this->items[$product_id]+=abs($this->items[$product_id]-$qty);
}
if($qty==0)
{
unset($this->items[product_id]);
}
}
}
function remove_item($product_id)
{
if(array_key_exists($product_id, $this->items))
{
unset($this->items[$product_id]);
}
}
function show_cart()
{
return $this->items;
}
}
$cart = new ShoppingCart;
$cart->add_items("test", "2", "3");
//$cart->remove_item("Apples");
$cart_items=$cart->show_cart();
foreach($cart_items as $key=>$value)
{
echo "$key $value<br>";
}
?>[/code]
PHP/SOAP -> SoapClient
in PHP Coding Help
Posted
btw if i change getBook to getBookX i get invalid method, so i know i can connect to the web service.