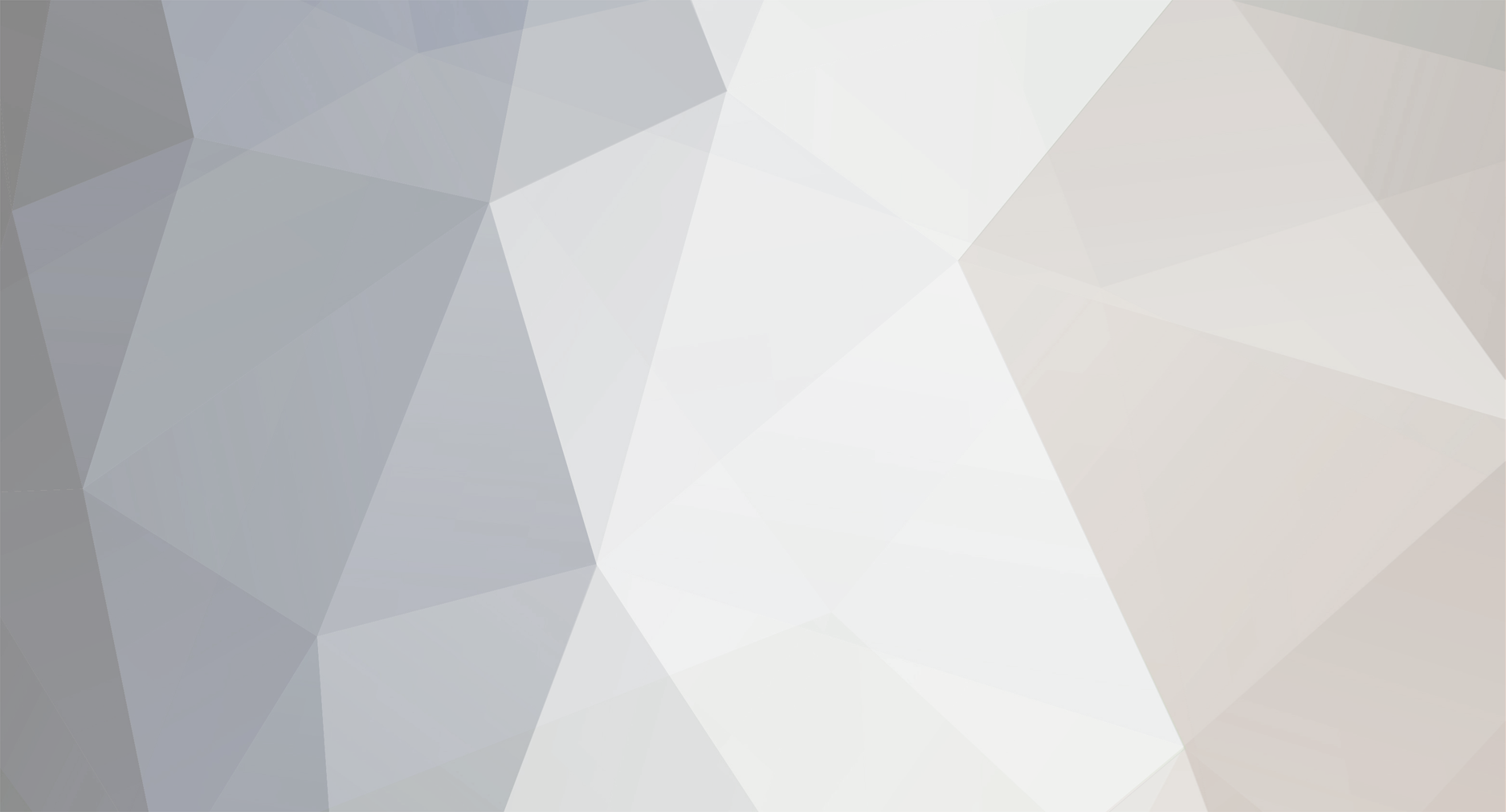
HuggieBear
Members-
Posts
1,899 -
Joined
-
Last visited
Everything posted by HuggieBear
-
What columns are in your table? It's always a good idea to have an auto-incrementing ID column of some kind in there. e.g. [b]Match_News[/b] Article_ID (unique, auto-increment) Article_Title Article_Date Article_Contents Then your sql could look like this: [code] <div align="justify"> <?php $query = "SELECT * FROM `Match_News` ORDER BY Article_ID DESC LIMIT 5"; $result = mysql_query($query); while (mysql_fetch_array($result)) { echo nl2br($row["article"]); echo '<br /><hr><br />'; } ?> </div> [/code] Regards Huggie
-
OK, do you have phpMyAdmin installed? If not I'd strongly advise getting it. Then you can test the sql works before testing the php. As it is, there's nothing wrong with the php. It's all the '.' you have. Just get rid of them and insert the following: [code] <?php $sql = "CREATE TABLE $producer ( `model` varchar(60) NOT NULL default 0, `serial` varchar(60) NOT NULL default 0, `InTime` date NOT NULL default 0, `provider` varchar(60) NOT NULL default 0, `aviz` varchar(60) NOT NULL default 0, `OutTime` date NOT NULL default 0, `add1` varchar(60) NOT NULL default 0, `add2` varchar(60) NOT NULL default 0, `add3` varchar(60) NOT NULL default 0, `buyer` varchar(60) NOT NULL default 0)"; ?> [/code] Regards Huggie
-
OK, to order by date, you must have the date in the database field somewhere. Assuming this is the case and the database field is called article_date, try this: [code] <div align="justify"> <?php $query = "SELECT * FROM `match_news` ORDER BY article_date DESC LIMIT 5"; $result = mysql_query($query); while (mysql_fetch_array($result)) { echo nl2br($row["article"]); echo '<br /><hr><br />'; } ?> </div> [/code] There's no point in limiting the loop to 5 iterations if you know your SQL array only contains 5 rows. You've made the restriction in MySQL. Regards Huggie
-
What errors are being output to the browser? Regards Huggie
-
I'd stick it in a variable... [code] <?php if ($row['hotelRating'] == 3){ $star = "star.gif"; } else if ($row['hotelRating'] == 4){ $star = "star2.gif"; } else if ($row['hotelRating'] == 5){ $star = "star3.gif"; } // List the hotels echo "<div class=\"homebar2\"><h1>".$name."</h1></div><div class=\"hotel\">".$image."</div><div class=\"hotelcontent\">".$description."</div><h3><img src=\"".$star."\" hspace=\"2\"></h3> ?> [/code] Regards Huggie
-
If you're passing an array into ValidateInput, which it looks as though you are. You probably need to carry out your actions on each element of the array, not the whole array. I'd have thought you're in need of a loop somewhere. Don't hold me to this, but maybe something like this inside the function. [code] <?php function ValidateInput($value) { $BBCode = array( "<b>" => "[b]", "</b>" => "[/b]", "<u>" => "[u]", "</u>" => "[/u]", ); foreach ($value as $k => $v){ $value[$k] = str_replace(array_keys($BBCode), array_values($BBCode), $v); $value[$k] = mysql_real_escape_string(trim(strip_tags($v))); } return $value; } ?>[/code] Regards Huggie
-
[quote] You could sort by rating. Maybe have 5 images for effect But the next line needs changing. from.....[/quote] No it doesn't, there's nothing wrong with that code. regionId is an integer, meaning no single quotes are required and as the $id is inside double quotes, the variable will be read, not a literal string. Regards Huggie
-
You'd be wise to use a regular expression here. Have a look at the [url=http://www.php.net/manual/en/ref.pcre.php]RegEx[/url] section in the manual. Regards Huggie
-
There's a function just for this... It's called [url=http://www.php.net/manual/en/function.file-get-contents.php]file_get_contents[/url] [quote] I would use cURL for this task. Make sure you have it installed. [/quote] cURL is overkill for what greenba wants. That is of course if his/her description is correct :) Quote from the manual... [quote] file_get_contents() is the preferred way to read the contents of a file into a string. It will use memory mapping techniques if supported by your OS to enhance performance. [/quote] Regards Huggie
-
Ordering Records in Columns rather than rows *solved*
HuggieBear replied to stualk's topic in PHP Coding Help
The following works, obviously you'll need to change the sql and variables accordingly. [code]<?php include('connect.php'); $result = mysql_query('SELECT count(countryName) FROM tblCountry'); $num_records = mysql_result($result,0,0); $max_num_rows = 2; $max_num_columns = 3; $per_page = $max_num_columns * $max_num_rows; $total_pages = ceil($num_records / $per_page); if (isset($_GET['page'])) $page = $_GET['page']; else $page = 1; $start = ($page - 1) * $per_page; $result = mysql_query("SELECT countryName FROM tblCountry ORDER BY countryName LIMIT $start, $per_page"); $num_columns = ceil(mysql_num_rows($result)/$max_num_rows); $num_rows = min($max_num_rows, mysql_num_rows($result)); echo "<table border=\"2\">\n"; for ($r = 0; $r < $num_rows; $r++){ echo "<tr>\n"; for ($c = 0; $c < $num_columns; $c++){ $x = $c * $num_rows + $r; if ($x < $num_records) $y = mysql_result($result, $x, 0); else $y = ' '; echo "<td>$y</td>"; } echo "</tr>\n"; } echo "</table>\n"; for ($i=1;$i <= $total_pages;$i++) { if ($i == $page) echo " $i "; else echo " <a href=\"?page=$i\">$i</a> "; } ?>[/code] [size=8pt][color=red][b]Edit:[/b][/color][/size] Just realised that this example includes pagination too. You can remove that if not required. Regards Huggie -
That's what I thought, I'm not a fan of doing it inline either ;) Huggie
-
Is the tracking field in the database unique? You have this line: [code=php:0]SELECT $filename,$filetype,$filesize,$filecontent FROM fileuploads WHERE tracking='$id' [/code] $id is coming from the variable $_GET['id'] and you're using WHERE with EQUALS instead of IN, so I was assuming there'd be only one id passed to the sql statement, but more than one row in the database with a 'tracking' value of $id. [color=red][size=8pt][b]EDIT:[/b][/size][/color] I've just had a thought. In you're loop, you're outputting a header and then you echo content, then you loop again. I'm not sure you can send headers more than once can you? Regards Huggie
-
[quote] Whats the correct syntax to merge [/quote] I'm not sure I understand the question. What do you have and what do you want to achieve? You want to say if $data1['maincat_id'] is equal to $maincat then to make it the selected option? Regards Huggie
-
You need to remove the pagination that you just put in... It shouldn't be too difficult to achieve. Regards Huggie
-
I'm guessing this is for printing purposes, otherwise, why would you need a page break. If so, then the answer is no, you'd have to work out how many rows you have that fit on a page and pad out with <br/> tags accordingly. Regards Huggie
-
Rodin, care to share? Huggie
-
Jenk, He only wants to keep parts of the [b]<font>[/b] tag, not all of it... It helps to read the post ;D Having said that, there is a good example on the manual page that a user has posted on how to achieve what you want. Regards Huggie
-
I'd imagine there's a bigger processing overhead with the MySQL solution than with Barand's. If that's a consideration then you might want to take another look at his suggestion. Regards Huggie
-
Good luck with this one... Not only is your post rude and demanding, but you've posted it in a forum that helps coders with specific problems, not provides prebuilt scripts for lazy students. Try http://www.google.com If you have attempted to code something, then post it here with the problems you're having and we'll happily look at it for you. Regards Huggie
-
Assuming that $hisname is greater than or equal to one, the code you've just posted will print... [color=red]s and Dave Lawson[/color] Probably not quite what you want... Regards Huggie
-
I'd use substr() to get the last two characters (individually)... $penultimate = substr($row['anumber'], -2, 1); $last = substr($row['anumber'], -1, 1); As to what you do with them after that, I'm not sure. You could put all the letters into an array and refer to them via their index... So if you wanted the letter Z (26th letter of the alphabet) you could use $letter[25]; (remember that an array index starts at 0, not 1). Regards Huggie
-
A session variable is a way to pass information between pages. An example would be... your user logs in with their username and password, your php code checks their username and password against the values stored in the database and then, assuming they authenticate, returns their nickname. You can then assign this info to a session variable, the code looks something a little like this: [code=php:0]$_SESSION['name'] = "Huggie"; [/code] You can then refer to that variable on any of your pages. So you could have this at the top of every page... [code]<?php echo "You are logged in $_SESSION['name']"; ?>[/code] Which would return [color=green]You are logged in Huggie[/color]. This information is active all the time you're still in the same session. There is other information that you need to include, such as session_start() but you can find this out by looking at the relevant pages in the [url=http://uk.php.net/manual/en/ref.session.php]PHP Manual[/url] Regards Huggie
-
When the user logs in, you could add their id into a session variable, maybe called id :) and then when they select their livingtype, you can insert into the table with something like... [code=php:0] sql = "INSERT INTO option (user_id, livingtype) VALUES ('$_SESSION[id']', '$type')"; [/code] Regards Huggie