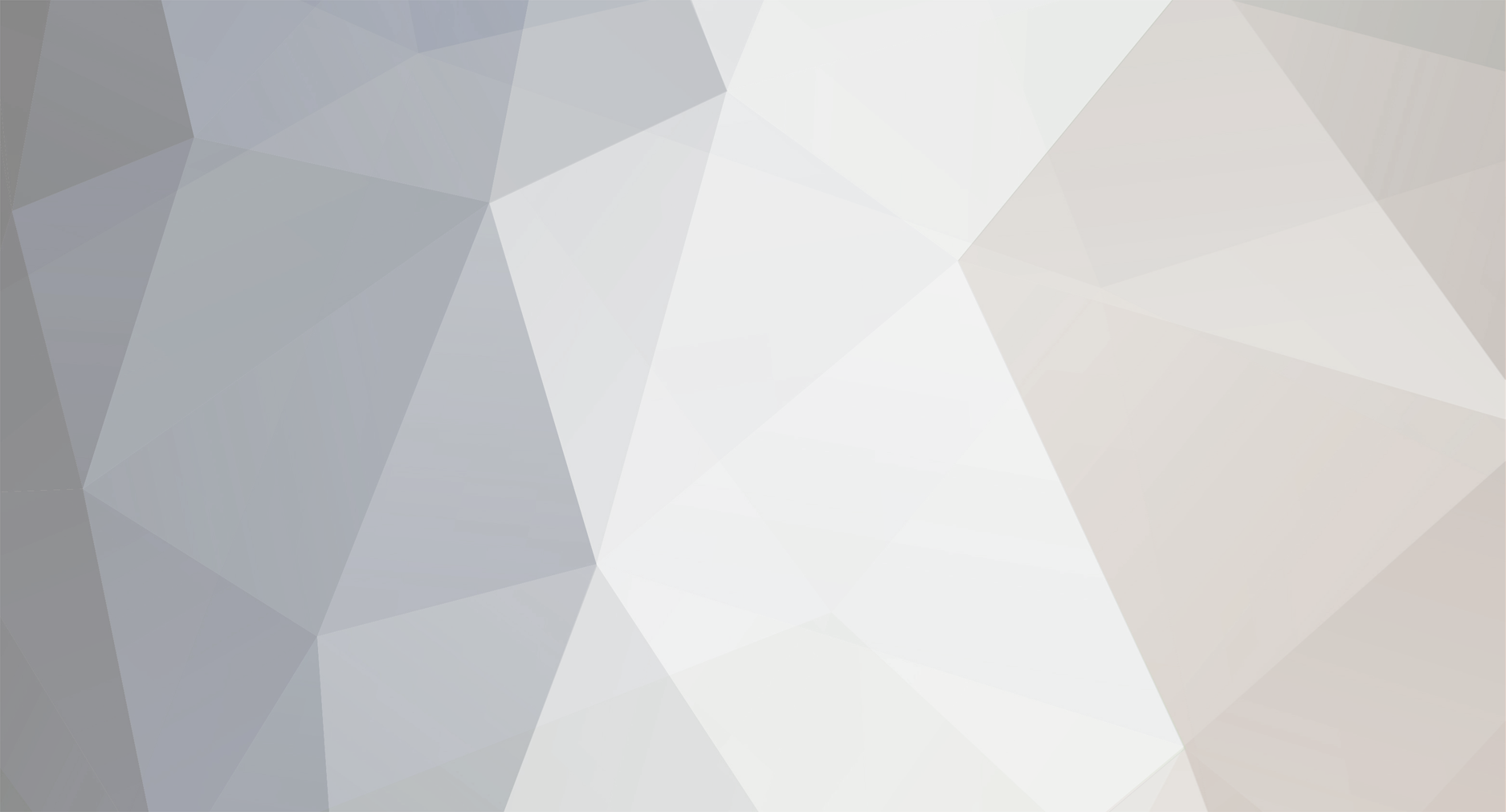
markjoe
Members-
Posts
226 -
Joined
-
Last visited
Never
Everything posted by markjoe
-
[SOLVED] Problem using responseText in different <div>s
markjoe replied to Michan's topic in Javascript Help
Wrong: xmlHttp.onreadystatechange=stateChanged(div); That line is assigning the return value of stateChanged(div), not assigning the function to the handler. Right: xmlHttp.onreadystatechange=stateChanged; Notice the problem? You cannot pass variables this way. Try this: xmlHttp.onreadystatechange=function(){ if (xmlHttp.readyState==4){ document.getElementById(div).innerHTML=xmlHttp.responseText; } }; This way you are assigning the code itself as a function type property (or something like that) and still within the scope of the selectcomic function. -
req.onreadystatechange = function() {ajaxDone();}; should be: req.onreadystatechange = ajaxDone; Does document have an innerHTML property? Here's a quick and dirty that seems to work. <script type='text/javascript'> timerID = setInterval("chatRefresh('m.php')", 2000); function ajaxDone() { if (req.readyState == 4 && req.responseText) { document.getElementById('TA').innerHTML=req.responseText; } } function chatRefresh(url){ var RN=Math.round(Math.random()*1000); if (window.XMLHttpRequest) { req = new XMLHttpRequest(); req.onreadystatechange = ajaxDone; req.open("GET", url+'?rn='+RN); req.send(null); // IE/Windows ActiveX version } else if (window.ActiveXObject) { req = new ActiveXObject("Microsoft.XMLDOM"); if (req) { req.onreadystatechange = ajaxDone; req.open("GET", url); req.send(null); } } } </script> <textarea id='TA'></textarea> I didn't really bother with the IE part, testing on Firefox. This is not at all how I would do this, but it works and is closest to your original code. Oh, yea... that random number thing is the best way I've seen to prevent caching.
-
I want to setup custom file extension for php files on IIS. I have done this (long time ago) on Apache, but I am not sure where to do it on IIS. I think I just need to set a new MIME type right? But what do I refer to the PHP interpreter as?
-
Hopefully this is enough to go on. PHP 5.2.5 connecting to SQL Server 2000. I have a fairly large union query that runs fine in SQL Query Analyzer in 1 minute 19 seconds. Run from PHP it fails with the last message being "Changed database context to 'DB NAME'". If I shorten the date range to a single day, it runs in PHP fine. This is how I am checking the date range: where datecol >= 17373 and datecol <= 17375 (date is integer date stamp in DB) I echoed the full query from the PHP script and pasted it in SQL Query Analyzer.
-
Would it be acceptable to round the number and just take the integer part of it? floor(12.3) gets converted to 12 Or you can test it as a string with strpos().
-
problem using recursion, trying to get info from nested arrays
markjoe replied to d_rock's topic in PHP Coding Help
downloading php file) I believe the only cause for this is your apache not being told what to do with a .php file. In your httpd.conf file there shoud be a line something like: AddType php application/x-httpd-php .php At least thats all my memory is coming up with at the moment. recursing multi-dimensional array) in the function that reads the array you need to check if the element is an array ( is_array() ) if it is (and only if) you call the function again. function parseArray($A){ foreach($A as $E){ if(is_array($E)){ parseArray($E) } } } ...thats what I have off the top of my head, hope it helps. -
I always wonder what people are looking at when they refer to a language being "powerfull". The number of built-in function ( I hope not)? The type of data access, SQL, binary files, network sockets, etc? The processing efficiency? I have a few PHP scripts that parse thousands of text files and directory listings (600-20000 files) in as short as a couple seconds. One of these days I want to write the equivalent in C, for a side-by-side. To the original point, I don't see why anybody would have a problem with a language that can literally show results in minutes. For data parsing, I would put PHP up against any other language complied or interpreted (development time included). That doesn't mean it will win them all, but I think it would show that PHP is no "toy". Full disclosure: it may come as a shock to some that I am not formally educated in programming. (in other words I may just be talking out of the 'other' end)
-
There are MANY different ways to make a calendar, there is no way to know where to start without more info. As always, your first step is google. http://www.google.com/search?q=php+calendar
-
I am rather confused by your code and feeling too lazy to put the time into it right now. But, I made a slider for a volume control recently and my test page was a drag-able button. Even though my slider is 1D, the test page is 2D. Here is the code, maybe it can help. Oh, and I did save the page and I see what you are talking about. It is nothing short of weird. <script type="text/javascript"> var F=null; var S=null; function grab(e,scope){ e.target.style.position='absolute'; S=scope; F=e.target } function letGo(){ F=null; } function drag(e){ var el=document.getElementById('div1'); el.innerHTML=e.clientX+' x '+e.clientY; if(F){ if(S=='h'){ F.style.left=e.clientX+'px'; } else if(S=='v'){ F.style.top=e.clientY+'px'; } else{ F.style.left=e.clientX+'px'; F.style.top=e.clientY+'px'; } } } document.onmousemove=drag; </script> <body> <button onmousedown="grab(event);" onmouseup="letGo();">Drag Me</button><br /> <div id="div1" style="font-size:9px;"></div> </body>
-
I took a quick look with Safari 2.0. No matter what I entered, it always said the Minimum Age was more than the Max Age (looking for tab).
-
Looks like setInterval('if(position>=duration){element.width=element.width+1;position++}else{updateUI();}',1000) will work. It's just a matter of fine tuning now. I'll probably end up around 2-3 seconds. with the option to disable it completely. I was mostly worried about slowly killing the browser the longer it played. Guess I'll just have to find out.
-
That's what I think I am going to have to do. Like with the conditional in the setInterval. I am just not quite sure how best to do it. I worry a little about if the functions keep calling each other back and forth without ever returning, is that going to keep eating more memory as it runs? I'll do some more testing with that idea and see what happens.
-
Here is another version that should play better in a real page. It gets the offsetTop for the element and all parent elements, ending up with the total offsetTop value of the element relative to the page. <script type='text/javascript'> var buttonClicked=false; function clickButton(){ if(buttonClicked==false){ document.form1.button1.style.top=(getOffsetTop(document.form1.button1)+30)+'px'; buttonClicked=true; } } function getOffsetTop(theElement){ theOffset=0; while(theElement!=null){theOffset+=theElement.offsetTop;theElement=theElement.offsetParent} return theOffset; } </script> <body> <form name='form1'> <input type='button' name='button1' value='button' style='position:absolute;top:0px;' onclick='clickButton();'> </form> </body>
-
As it turns out (had to check the book), offsetTop and offsetLeft are read-only properties. You will need to use styles. Here's what I worked out: <script type='text/javascript'> //set the global variable to track if button has been clicked var buttonClicked=false; function clickButton(){ if(buttonClicked==false){ //set the top attribute of the elements style property to its offset + 30 pixels document.form1.button1.style.top=(document.form1.button1.offsetTop+30)+'px'; //set global variable, so function will not moved button again buttonClicked=true; } } </script> <body> <form name='form1'> <input type='button' name='button1' value='button' style='position:absolute;' onclick='clickButton();'> </form> </body> It now occurs to me that offsetTop is relative to the parent element (like a table cell), so it may be better off to set the top position in the style tag. you can use document.form1.button1.style.top to get the value instead of document.form1.button1.offsetTop. But style.top will return with the "px" atached so you would need to remove that before doing any math.
-
I am building an iTunes Web interface (yes, I know there are quite a few out there, but all I have found are either incomplete or I just didn't like them). I did a setTimeout to wait the duration of the song to then update the current song info when the next song starts playing.(working fine so far) I would also like to use setInterval to update the interface once a second to scroll the position indicator as you would expect in any media player. example: setInterval('element.width=element.width+1',1000) Is there a way to combine them do do both at the same time? Maybe a conditional in the setInterval? setInterval('if(position>=duration){element.width=element.width+1;position++}else{updateUI();}',1000) I believe then updateUI() would have to clearInterval and re-call it with the new duration?
-
Well, I think i get what your asking for. <?php $height=10;$width=10; echo "<table border='1'><tr>"; for($i=0;$i<=$width;$i++){echo "<td>$i</td>";} echo "</tr>"; for ($i = 1; $i <= $height; $i++) { echo "<tr><td>$i</td>"; for ($j = 1; $j <= $width; $j++) { echo "<td>x</td>"; } echo "</tr>"; } echo "</table>"; ?> Outputs: 0 1 2 3 4 5 6 7 8 9 10 1 x x x x x x x x x x 2 x x x x x x x x x x 3 x x x x x x x x x x 4 x x x x x x x x x x 5 x x x x x x x x x x 6 x x x x x x x x x x 7 x x x x x x x x x x 8 x x x x x x x x x x 9 x x x x x x x x x x 10 x x x x x x x x x x
-
global variables or points or something i can modify
markjoe replied to optikalefx's topic in Javascript Help
(non-expert alert) What about making it a (pseudo)class? I made my first one only a few days ago. I have had global variable problems like this in the past. I have not, however, had any issue refering to "this.variable" in a method. Or, sometimes I fall back on a hidden input in palce of a global variable, ugly way of doing things, but it works. -
I'm no expert, but here's my guess: document.formname.B1.offsetTop=(document.formname.B1.offsetTop+30); Something like that should work to move the button, but it may differ slightly for different browsers. To make it only happen once you could set a hidden input value. if(document.formname.inputname.value!='1'){ document.formname.B1.offsetTop=(document.formname.B1.offsetTop+30); document.formname.inputname.value='1'; } That would require this in the HTML of the page within the named form: <input type='hidden' name='inputname' value=''> Side note: I would like to stress that Java and javascript are completly different things. I imaginge that you didn't mean Java in the middle of your post. I just feel the need to point that out sometimes.
-
I had a BAT file reading in and ftp script to "mget" some files by ftp. I am replacing this with a CLI PHP script to eliminate user interaction (changing date to match in file name), and make it more flexible. Trouble: everything I try in ftp_raw() returns "command not understood". $curdate3digit is returning the correct value. $ftpc=ftp_connect("123.45.67.89"); $ftpl=ftp_login($ftpc, "username", "Pass"); if($ftpl){ $ftpout[]=ftp_raw($ftpc, 'lcd D:/); $ftpout[]=ftp_raw($ftpc, 'prompt off'); $ftpout[]=ftp_raw($ftpc, 'bin'); $ftpout[]=ftp_raw($ftpc, 'cd /remote/dir/togetfrom'); $ftpout[]=ftp_raw($ftpc, "mget $curdate3digit*.sch"); }else{ echo "ftp login failed\n"; } ftp_close($ftpc);
-
PHP can definetly not get the MAC address. I was hoping there was some weird way for Javascript to get it. If I really want to do the automated free trial, I may have to require the user to run an app on their machine to request the free trial access. The client app would get some unique info, including but maybe not limited to the MAC address, and send it in the request. From the user POV, I wouldn't like that at all. Or, possibly, work it off email, emails include all kinds of info in the headers. Or, most likely to happen, handle free trail requests manually, if I offer them at all.
-
Thank you for confirming that for me. I will just have to go without the automated free trial.
-
This can be under PHP and Javascript, but, double posting is a no-no. So it is here only. I am looking for a way to uniquely identify a client machine either through PHP, or JavaScript or a combination of the two, other than IP and cookies. The point is a free trial with a limited number of uses. I don't see it as possible, but people here have proven me wrong in the past.
-
pass an ampersand to a database through AJAX......
markjoe replied to scarhand's topic in Javascript Help
I've never run into this problem, but it occurs to me that you could use the ascii code to make the transit and then back to the character to make the comparison. Just a thought, seems simple enough. 2 PHP functions of interest: ord() chr() -
I have 2 AJAX functions that populate drop down menus in the same form, from a database, they end up running at the same time. Each has it's own pair of functions, one to call the php script, and one to handle the response. (same PHP script), it works just fine.
-
I have recently had troubles with getting AJAX going for the first time. I dropped the check for http.status == 200. I only wait for http.readystate to reach 4. Then it worked for me. In the code you posted, I see where you are creating the XMLHttpRequest object, but I don't see you ever send the request. The function to send the request is there, but it doesn't get called. What are function are you calling from the page?