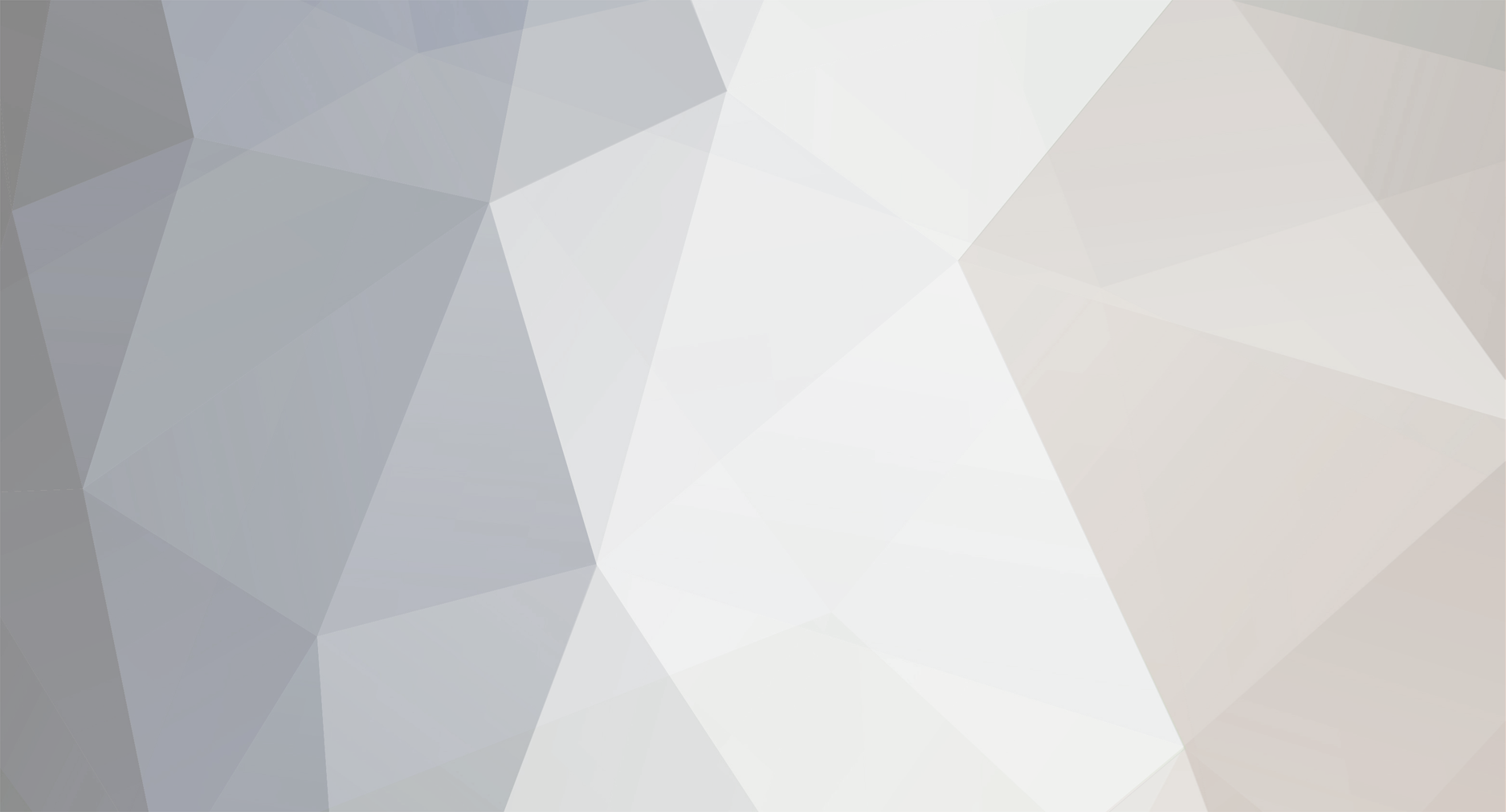
dennismonsewicz
Members-
Posts
1,136 -
Joined
-
Last visited
Everything posted by dennismonsewicz
-
[SOLVED] Session problems still
dennismonsewicz replied to dennismonsewicz's topic in PHP Coding Help
can anyone help? -
[SOLVED] Session problems still
dennismonsewicz replied to dennismonsewicz's topic in PHP Coding Help
bump -
I have this code: <?php session_start(); ob_start(); include "sql.php"; $action = $_GET['action']; $login_error = '<h2 style="color: red">Login Failed, Try Again</h2> <form action="index.php?action=login" method="post"> <label>Username:</label> <input type="text" name="username" id="username" /> <label style="margin-top: 5px;">Password: </label> <input type="password" name="password" id="password" /> <label><input type="submit" value="Login" style="margin-top: 5px;" /></label> </form>'; $login = '<h2>Login</h2> <form action="index.php?action=login" method="post"> <label>Username:</label> <input type="text" name="username" id="username" /> <label style="margin-top: 5px;">Password: </label> <input type="password" name="password" id="password" /> <label><input type="submit" value="Login" style="margin-top: 5px;" /></label> </form>'; if($_POST) { if($_POST['username'] || $_POST['password']) { $_SESSION['username'] = stripslashes($_POST['username']); $_SESSION['password'] = stripslashes($_POST['password']); } } switch($action) { case "login": $result = mysql_query("SELECT * FROM users WHERE username = '".mysql_real_escape_string($_SESSION['username'])."' AND password = '".mysql_real_escape_string($_SESSION['password'])."'") or die(mysql_error()); $num_rows = mysql_num_rows($result); if($num_rows == 0) { echo $login_error; } else { header("location:index.php"); } break; case "logout": session_destroy(); ob_end_flush(); echo $login; header("location:index.php"); break; } $sql = mysql_query("SELECT * FROM users WHERE username = '".mysql_real_escape_string($_SESSION['username'])."' AND password = '".mysql_real_escape_string($_SESSION['password'])."'") or die(mysql_error()); $results = mysql_fetch_object($sql); $username = $results->username; if(!$_SESSION['username']) { echo $login; } else { echo '<h2>Recent Projects</h2>'; echo '<ul>'; $query = mysql_query("SELECT * FROM added_projects ORDER BY id DESC LIMIT 0,10") or die(mysql_error()); while($results = mysql_fetch_object($query)) { echo '<li><a href="tools.php?action=view&id=' . $results->id . '">' . $results->project . '</a></li>'; } echo '</ul>'; } ?> The above code is in a file called rightnav.php and is included on the individual pages I also duplicated the session_start code in the included header file (that is also included on every page): session_start(); ob_start(); When I login using a wrong username and/or password (for testing) I receive the $login_error var but the While statement displays results and if you refresh the page the session begins! Any ideas on how to fix this?
-
$action is being set above the $login_error var $action = $_GET['action']; And no the pw is not encrypted. I will worry about that a little later I am just trying to get the dern thing working to full capacity
-
anyone have any ideas?
-
try changing this line: $body .= chunk_split(base64_encode($return_html),68,"\n"); to $body .= $return_html; Try sending the mail without encoding the output
-
why are you encoding the email?
-
the session_start() and ob_start() are located in the header file: $session = $_GET['session_start']; switch($session) { case "yes": session_start(); ob_start(); break; }
-
I have a login system that checks to see if the username and password are there and if they are the script is supposed to then login you in and off you go on your marry way Well here is my code: $login_error = '<h2 style="color: red">Login Failed, Try Again</h2> <form action="index.php?action=login" method="post"> <label>Username:</label> <input type="text" name="username" id="username" /> <label style="margin-top: 5px;">Password: </label> <input type="password" name="password" id="password" /> <label><input type="submit" value="Login" style="margin-top: 5px;" /></label> </form>'; if($_POST) { if($_POST['username'] || $_POST['password']) { $_SESSION['username'] = stripslashes($_POST['username']); $_SESSION['password'] = stripslashes($_POST['password']); } } switch($action) { case "login": $result = mysql_query("SELECT * FROM users WHERE username = '".mysql_real_escape_string($_SESSION['username'])."' AND password = '".mysql_real_escape_string($_SESSION['password'])."'") or die(mysql_error()); $num_rows = mysql_num_rows($result); if($num_rows == 0) { echo $login_error; } else { header("location:index.php?session_start=yes"); } break; case "logout": session_destroy(); ob_end_flush(); header("location:index.php"); break; } $login = '<h2>Login</h2> <form action="index.php?action=login" method="post"> <label>Username:</label> <input type="text" name="username" id="username" /> <label style="margin-top: 5px;">Password: </label> <input type="password" name="password" id="password" /> <label><input type="submit" value="Login" style="margin-top: 5px;" /></label> </form>'; if(!$_SESSION) { echo $login; } else { echo '<h2>Recent Projects</h2>'; echo '<ul>'; $query = mysql_query("SELECT * FROM added_projects ORDER BY id DESC LIMIT 0,10") or die(mysql_error()); while($results = mysql_fetch_object($query)) { echo '<li><a href="tools.php?action=view&id=' . $results->id . '">' . $results->project . '</a></li>'; } echo '</ul>'; } When you login, even if the login is correct, the script displays the $login_error var and also displays the unordered list in the while statement Any ideas?
-
thanks for the help... I was able to locate the address for our SMTP server and adjust the php.ini file accordingly
-
I am trying to use the mail() function on my server and it doesn't work. I tried it on another server and it works fine, but not on the current server. Code: <?php $Name = "Da Duder"; //senders name $email = "dennismonsewicz@gmail.com"; //senders e-mail adress $recipient = "dennismonsewicz@gmail.com"; //recipient $mail_body = "The text for the mail..."; //mail body $subject = "testing"; //subject $header = "From: ". $Name . " <" . $email . ">\r\n"; //optional headerfields $headers .= "MIME-Version: 1.0\n"; $headers .= "Content-type: text/html; charset=iso-8859-1\n"; if(mail($recipient, $subject, $mail_body, $header)) { echo '<p>Mail sent successfully...</p>'; } else { echo '<p>Mail delivery impossible!</p>'; } //mail command ?> I receive the "Mail delivery impossible" when I try running the page on one server and the "Mail sent successfully..." on another server Any ideas?
-
ok I have fixed the syntax error, but the results are only displaying one result when there are 4 results in the tested DB that are exactly the same. Any ideas? Updated code: $result = mysql_query("SELECT * FROM added_projects WHERE project LIKE '%" . $project_name . "%'") or die(mysql_error()); $rows = mysql_num_rows($result); if ($rows > 0) { while ($result = mysql_fetch_object($result)) { echo '<p>Here is a list of other projects that have similar Project Names:</p>'; echo '<p><a href="tools.php?action=view&id=' . $result->id . '">' . $result->project . '</a></p>'; } }
-
Ok so i have this script: $exists_query = mysql_num_rows(mysql_query("SELECT project FROM added_projects WHERE project LIKE %" . $project_name . "%'")); if($project_name == $exists_query) { while($results = mysql_fetch_object($exists_query)) { echo '<p>Here is a list of other projects that have similar Project Names:</p>'; echo $results->project; } } The code is supposed to loop through the db and find like project names and then display the results. But its not happening Any ideas?
-
alright, thanks for everyones replies i was just doing some thinking about structuring of a sql table.
-
To allow a SQL column to have a NULL value?
-
yes wildteen's example works! <?php $animal = $_GET['animal']; switch($animal) { case 'dog': case 'cat': // etc echo 'You requested : '. $animal; break; default: echo "none"; } ?> I modified the $animal var to GET the animal from the URL, but it works like a charm! Thanks again!
-
Well the problem with "unique hit counters" is that they are never a true unique counter. You seem to be on your way by calculating the information using a DB but you may have to get into capturing IP addresses as well...
-
you don't have your mailform.php FORM tag pointing to mail.php its pointing back to iself. Also I would use $_POST or $_GET not $_REQUEST
-
refreshing a page after updating database
dennismonsewicz replied to saco721's topic in PHP Coding Help
I wouldn't use meta tags I would use PHPs header or JS window.reload -
Is there anyway to stack cases in PHP like you can in Javascript? IE: case "dog": case "cat": case "bird": DO SOMETHING Where the programming will say if its all three of those cases then do the statement at the end
-
I am trying to send an email on my server using PHP and i keep getting the error message if the mail fails Here is my code: <?PHP $to = "dennismonsewicz@gmail.com"; $subject = "Hi!"; $body = "Hi,\n\nHow are you?"; if (mail($to, $subject, $body)) { echo("<p>Message successfully sent!</p>"); } else { echo("<p>Message delivery failed...</p>"); } ?> Is there any place to check to see if mail is running?
-
"imagestick" made me ROFL! I needed that... been a rough day
-
thanks... I knew i could do that.. I was just wondering if there was any more dynamic way to solve this problem. Thanks though, problem solved
-
in if statements, can a variable == a variable?
dennismonsewicz replied to Hoppus's topic in PHP Coding Help
if you are referring to something like this if($var1 == $var2) { echo "some code"; } then yes -
I have the following html select echo '<select name="company">'; echo '<option value="" selected="selected">' . $results->company . '</option>'; echo '<option value="Health Resources">company1</option>'; echo '<option value="True Health">company2</option>'; echo '</select>'; The DB is going to house company 1 or company 2 depending upon the company selected at the time the user was creating this entry. Well I am wanting to make it where if company1 is in the DB then it doesn't repeat itself in the select list. And vice versa for company2. Any ideas?