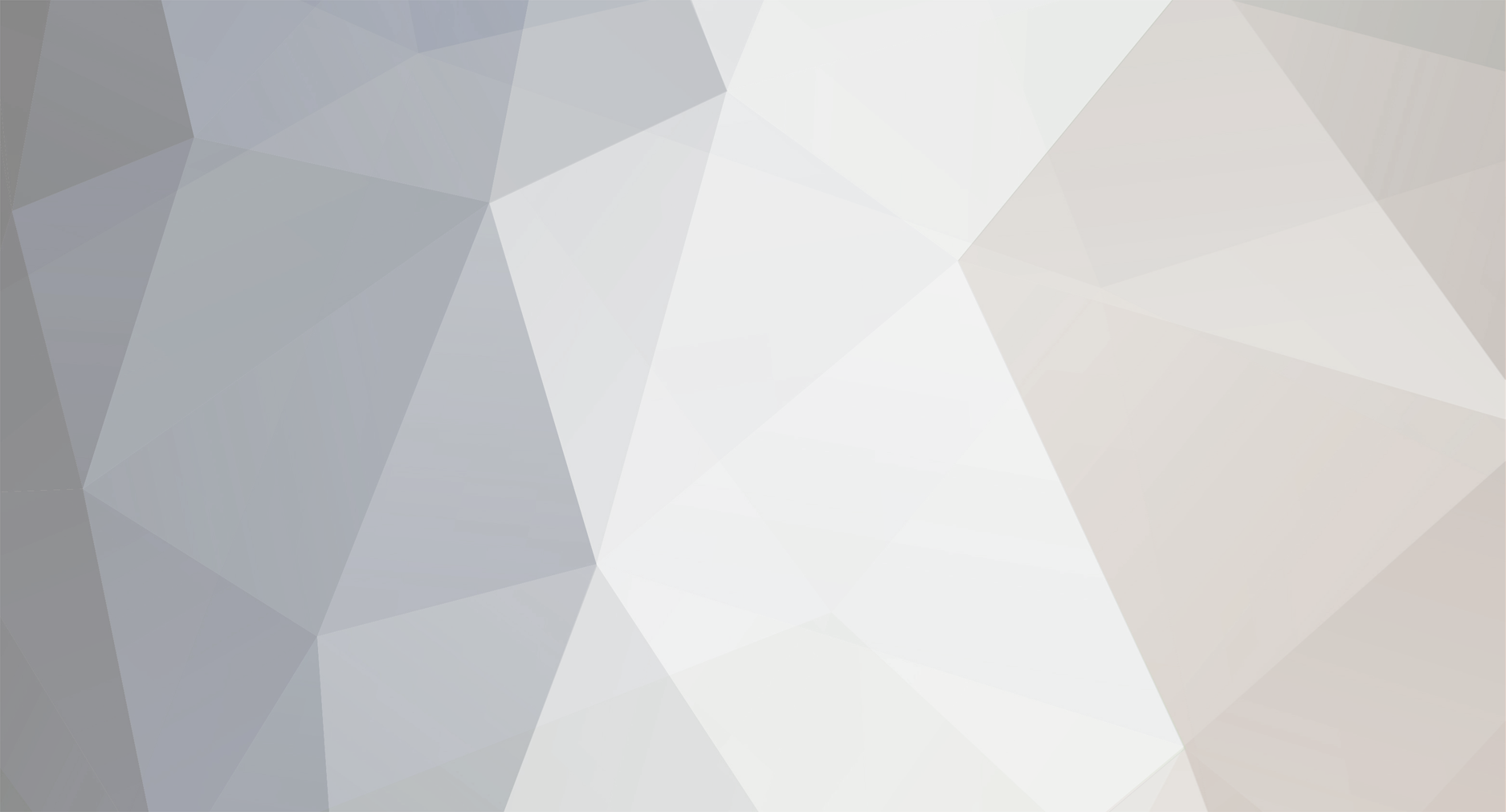
electricshoe
Members-
Posts
27 -
Joined
-
Last visited
Never
Everything posted by electricshoe
-
Advanced String Find/Replace/Regex Question
electricshoe replied to electricshoe's topic in PHP Coding Help
Modified code to handle between, less than, more than, and equals. This provides a summary of the query beautifully, although there are many more fields than just the 5 I have below. Thank you both again <?php $human = array( 'make_id' => 'Make', 'model_id' => 'Model', 'year' => 'Year', 'bodystyle' => 'Body Style', 'mileage' => 'Mileage' ); $string = $searchstring; if(preg_match_all('/(\w+)\s*=\s*\'(.+?)\'/',$string,$matches)) { foreach(array_keys($matches[0]) as $n) { print "{$human[$matches[1][$n]]}: {$matches[2][$n]}<br>"; } } if(preg_match_all('/([^ ]*)[ ]*>[ ]*([^ ]*)/',$string,$matches)) { foreach(array_keys($matches[0]) as $n) { print "{$human[$matches[1][$n]]}: More Than {$matches[2][$n]}<br>"; } } if(preg_match_all('/([^ ]*)[ ]*<[ ]*([^ ]*)/',$string,$matches)) { foreach(array_keys($matches[0]) as $n) { print "{$human[$matches[1][$n]]}: Less Than {$matches[2][$n]}<br>"; } } if(preg_match_all('/([^ ]*)[ ]*BETWEEN \']*([^ ]*)[\' AND \']*([^ ]*)[\']/',$string,$matches)) { foreach(array_keys($matches[0]) as $n) { print "{$human[$matches[1][$n]]}: ".number_format($matches[2][$n])."-".number_format($matches[3][$n])."<br>"; } } ?> Edit: How do you make the code colorful? -
Advanced String Find/Replace/Regex Question
electricshoe replied to electricshoe's topic in PHP Coding Help
Thank you both! You're both beautiful people! Rhodesa's code works great since I have a lot more field names than just those 3 and I like being able to redo the text for them. But sasa's regex was useful in covering the other search conditions (Less Than, More Than, Between). preg_match_all() was the function I was missing and I thank you both for enlightening me to it. Now the fun part is coming up in which I have to interpret a rather long join query into human readable text as well, but this puts me well on my way. I'll post the basic result I end up with for further knowledge and then close this thread out. Thanks again for the help -
I have a query and I want to parse the WHERE conditions to a human readable format. The conditions are already seperated and they look like this: make_id = 'Ford' AND model_id = 'Focus' AND year = '2005' And I want to parse it and make it look like this: Make: Ford Model: Focus Year: 2005 Now what I'm working with is a rather messy set of explodes and strpos that breaks way too easily. What I want to do is use regex to capture the innerpart of the quotes, is there an easy way to use regex to pull that part of the string out? It would be matching between the text and pulling out the value between the quotes. Any help is greatly appreciated, Thank you many moons in advance:)
-
Is there a no leading zero minute variable hidden somewhere in magicland? I'm assuming no since there isn't one in the manual. If not is there an easy way for me to parse it in the date() function instead of writing something complicated? Thanks ten million billion in advance
-
Anyone? Please help, this problem has had me stumped all weekend. Thanks 1million times in advance:)
-
Okay so the bits to get the URL of a page, simple and effective function selfURL() { $s = empty($_SERVER["HTTPS"]) ? '' : ($_SERVER["HTTPS"] == "on") ? "s" : ""; $protocol = strleft(strtolower($_SERVER["SERVER_PROTOCOL"]), "/").$s; return $protocol."://".$_SERVER['SERVER_NAME'].$_SERVER['REQUEST_URI']; } function strleft($s1, $s2) { return substr($s1, 0, strpos($s1, $s2)); } print(selfURL()); BUT, using mod rewrites makes it all funky as you can imagine. So if I'm using RewriteRule ^([^/]+)/$ index.php?variable=$1 Then I do domain.com/thispage/ but the above function will return domain.com/index.php?variable=thispage. How do I get the first part without writing a reverse rewrite, or is that what I have to do?
-
Reposted since I put it in the wrong forum! So I use a function to create multiple requests in an array so I can use multiple ajax requests at the same time. This works great until it comes time to get the response text request_array[i].onreadystatechange = function() { update_target(i,target) } function update_target(request_array_key,target_to_update) { if (request_array[request_array_key].readyState == 4) { if (request_array[request_array_key].status == 200) { document.getElementById(target_to_update).className = ''; document.getElementById(target_to_update).innerHTML = request_array[request_array_key].responseText; } } } If I create 3 requests it will run all 3 successfully, and I get all 3 returns in firebug, but it only updates the innerHTML on the last one. Why is this? It's got to be some kind of order of operations thing. Thanks in advance!
-
So I use a function to create multiple requests in an array so I can use multiple ajax requests at the same time. This works great until it comes time to get the response text request_array[i].onreadystatechange = function() { update_target(i,target) } function update_target(request_array_key,target_to_update) { if (request_array[request_array_key].readyState == 4) { if (request_array[request_array_key].status == 200) { document.getElementById(target_to_update).className = ''; document.getElementById(target_to_update).innerHTML = request_array[request_array_key].responseText; } } } If I create 3 requests it will run all 3 successfully, and I get all 3 returns in firebug, but it only updates the innerHTML on the last one. Why is this? It's got to be some kind of order of operations thing.
-
Using PHP/Session Variables to secure queries through AJAX
electricshoe replied to electricshoe's topic in PHP Coding Help
Thanks for the reply, the problem with that solution is that all of the queries are generated automatically on 2 seperate levels, and several of my pages have the potential to need several hundred different queries (Which is why I'm switching to ajax, to only run the ones I need) -
I have a php application already built and I'm trying to add AJAX to it (This is mainly a php question though) I need to be able to secure query information that I send between files. Can't just create a file thats like query.php?q="INSERT INTO table (Whatever you want!) VALUES ('ruin my database') and pass that info to it through GET, nor through POST. I understand I could set a session variable with the query string inside it, and pass the session id through ajax, as well as the names of the session variables to call. And by calling those variables the PHP file would then do what it needed as per what variables it was provided. How secure would this be? I've been reading about session hijacking, but it doesn't seem possible. There isn't much you can do with a plain text Session ID to harm my server right? Or with a plain text Session ID, and the session variable Names, but not values. There's no way for a user to set a session variable value short of hacking my server and uploading their own php right? Thanks a lot in advance, I'm trying to become less of a session noob, and more of a session pro!
-
[SOLVED] PHP Form Security - A Few Questions
electricshoe replied to electricshoe's topic in PHP Coding Help
Thanks, but I've been reading fervently on session stuff to become less of a noob at it. What I had before was this: function draw_process($table, $type='insert', $conditions='') { echo '<input type="hidden" name="form_table" value="'.$table.'" >'; echo '<input type="hidden" name="form_process" value="'.$type.'" >'; echo '<input type="hidden" name="form_conditions" value="'.$conditions.'" >'; } Now I've changed it to this function draw_process($table, $type='insert', $conditions='') { $_SESSION[$GLOBALS['form_name'].'_'.$GLOBALS['form_id'].'_form_table'] = $table; $_SESSION[$GLOBALS['form_name'].'_'.$GLOBALS['form_id'].'_form_process'] = $type; $_SESSION[$GLOBALS['form_name'].'_'.$GLOBALS['form_id'].'_form_conditions'] = $conditions; } The globals are generated each time I run a draw_form() function, to ensure that each form on the page has unique variables. Now the fun problem is I need to pass ${$GLOBALS['form_name'].'_'.$GLOBALS['form_id']}, which is the name attribute of the form I'm submitting, but unluckily I can't simply pull in the name of the form being submitted through post. So now I need to be able to recognize what form has been submitted in the processor without using $_GET or a hidden field, I suppose a hidden field that has the same name as the form? and then I operate of its name and ignore its value? -
[SOLVED] PHP Form Security - A Few Questions
electricshoe replied to electricshoe's topic in PHP Coding Help
My specific problem is that I'm using hidden fields to tell my form processor what to do (update, delete, insert, etc). I'm kind of a noob with sessions, but if I set it (and display an SID in the url is out of the question for the application), would that put a cookie on their machine with the values? I could just encrypt that and then decrypt it back on the server though right? That sounds awfully complicated for just passing a few variables that are independent to each form. My system is currently setup so that I can generate multiple forms on the same page each with validation and everything else, so if possible I'd just like to pass the variables to the form processor, do I need to create a whole thing to pass it through the http header when it goes to the post? And my big question is, is this more secure or would it still allow tampering. I'm configuring how each form behaves separately and then passing it to a universal processor that executes it. There cannot be anyway to alter that behavior data or else I have a BIG problem. So whatever way that allows me to pass it without any accessibility to the user is what I need. Thanks! -
Question 1: I just noticed that with developer extension in firefox you can change the values of hidden fields to whatever you want and then submit the form. This is pretty much fatal to anyone using paypal's easy form code to process simple transactions (I don't use this, but just an example, since you could edit in your own price or anything else you wanted) Is there anything php can do to stop this? Do I need to write a much more advanced poster so I can store the variables in php and then have them posted with http headers, or is that just as vulnerable? Question 2: Is there something I can put in my form processor to make certain that it doesn't process post information that originated outside my server? Is checking the HTTP_REFERRER good enough to do that? I couldn't find much clear documentation about this, specifically the dev extension problem, but it fundamentally breaks hidden inputs. My code is secured against SQL injections and everything else very well, but I don't know how to address these issues, and I don't like to take chances with security. So your help is very much appreciated!
-
Figured it out, it wasnt an explicitly a java problem. Just had to use multiple escapes per level, and in the php generator I had to use escapes for the escapes to get it in so in php the quote was escaped by \\\' and in java the output was \'
-
I'm using php to generate html, that has a javascript event, that calls a function that builds a string of java functions that is eval'd by java. The problem is this creates triple nested quotes and I have no idea how to do it. The problem looks like this: <a onclick="java_function_1('variable_1','java_function_2("variable_2","variable_3","etc")')" What should I do for quotes for the variables in java_function_2? This is something that has bugged me for a while but I've never had to deal with it until now. Thanks 10 million in advance:)
-
Serverside time is correct, vbulletin pulls in the correct time as well. But whenever I run the date() function it returns a time 12 hours in the future. I'm running the latest version of zendcore and I can't seem to find a place to just set a date offset, do I have to do it all with the latitude and longitude stuff? Thanks 10 million times in advance:)
-
[SOLVED] Function Names stored in Array
electricshoe replied to electricshoe's topic in PHP Coding Help
Beautiful, thanks very much. foreach($java as $var) { if function_exists($var); { eval($var); } else { echo $var.' '; } } I'm building html elements with functions. I'm doing form fields right now I'm loading in java functions, but some of the java functions are built through php functions (Depending on if they need ajax stuff or not). -
I'm storing java and php functions in one array. The java functions are just added to a string that is echoed in. I need the php functions to be executed if they are php functions. Is there anyway to check if a variable is a php function and then execute that function if it is? Thanks very much in advance!
-
[SOLVED] Insert Into Middle of Array
electricshoe replied to electricshoe's topic in PHP Coding Help
Derp, thank you very much! ;D I forgot that setting zero on splice inserted the value. Unfortunately I wound up setting it up a different way since the way I was including it broke the arrays relation to their dynamic variable children:( But it works, and thank you very much for the info, hats off to you! -
I've read over all of the array functions at php.net. The closest thing to what I'm looking for is array_push(). But I can't figure out how to use push to put data in at a specific point in the array, just the beginning. I want to insert data in the middle of my arrays while preserving/pushing back all the values behind it: array(0,1,2,3,4); insert_into_array at 2(value1,value2,value3) so then my array is 0,1,2,value1,value2,value3,3,4 How do you do this? I'm feeling befuddled, maybe I should get more sleep (I really don't get enough sleep:() Thanks for your help in advance
-
I'm modifying a copy of oscommerce to work with a template system I'm building. This template system basically just includes a list of files in the order they are specified in the database and sets their permissions etc. It includes the files through a function, which is limiting the included files from referencing objects/classes/anything. This works: include("store_session.php"); include("../includes/header.php"); include("index.php"); This Does Not Work: function includeThis() {include("store_session.php");} function includeThat() {include("../includes/header.php");} function includeThisToo() {include("index.php");} includeThis(); includeThat(); includeThisToo(); It returns call to non-object errors on all of the classes. My code is a bit more complex than this, but I have tested the above code and it doesn't work. How can I use includes inside a function and have their class structure still work? Do I need to rethink how the program works? I hope I don't. Here's the page builder function: function pagebuilder() { //Load the current page info query("thispage","pages","id","=",$page,'','',''); queryData("thispage"); //echo 'This Page ID:'.$GLOBALS['thispage']['id'].'<br>This template id: '.$GLOBALS['thispage']['template'].'<br>'; //Load the current page template query("template","templates","id","=",$GLOBALS['thispage']['template'],'','',''); queryData("template"); //Load module stack - This builds the whole page query("mod","templatemodules","templateid","=",$GLOBALS['template']['id'],"position","ASC",0); while ($GLOBALS['modrows'] > 0) { queryData("mod"); //echo '<br><p><b>Module Function Run</b></p>'; moduleBuilder($GLOBALS['mod']['id']); } } Basically I get the page id -> get the template id -> get all the 'modules' with that template id and then use the moduleBuilder() function to load all the modules. How can I do this and not get non object errors? Thanks soooo much in advance, I'm completely in the dark on this one ???
-
Class Includes returning Non-Objects (File Structure Problem)
electricshoe replied to electricshoe's topic in PHP Coding Help
I figured out exactly what the problem is, the file includes are coming in through functions and this is destroying their ability to do classes. I'm gonna close this one and ask in the general php forum. Feel free to delete this post. -
I'm building an application that pulls in other applications in the document, and I'm having a bit of a file structure problem calling in classes with includes. I'm a huge noob with file structure and using classes. The problem exactly is that oscommerce is in a separate directory than the main script. The index builds out a file, which includes all of the oscommerce header stuff, then custom template things, and then includes the body of the oscommerce. When I run a simplified version of the script in the root folder of the oscommerce installation it works fine. But when I run oscommerce in a different folder and include the files into the script it breaks and gives me a "Call to a member function display_price() on a non-object in filepath/file.php on line 33" Is there anyway to get around this? Because I'm going to be cramming a lot of applications together in this script and I want each one to be in a separate folder and I include them into the script that runs in the root directory. Currently the file structure looks like this: root :: mainscript root/modules :: custom modules root/modules/include :: custom includes root/modules/store/ :: oscommerce root/modules/anyotherapp/ :: any other application i may want to include so the script references files in different folders and includes them all into the main page builder. What can I do, I'm completely clueless here and having a hard time pinning down a tutorial that covers this. Thanks in advance, I appreciate your help A LOT
-
[SOLVED] Dynamic Variables in $GLOBALS
electricshoe replied to electricshoe's topic in PHP Coding Help
You're beautiful. $GLOBALS['customRowName']['id'] loves me and I'm a noob with for statements so I have been educated. If I were a girl and hot I would kiss you -
Heya I'm trying to set a dynamic variable as a global in a function. It's a simple function that takes a $result from a mysql query and gets the array with a custom variable name. function queryData($data) { $GLOBALS[$data] = mysql_fetch_array($GLOBALS['result']); } queryData("customRowName"); echo $customRowName['id']; This code doesn't work. The query is fine, I can do print_r($GLOBALS[$data]) and it returns my array. I've tried $GLOBALS[${$data}] and it doesn't work either. I'm getting a notice that the variable is unset for both of them Notice: Undefined variable: customRowName in ---- on line 20 Any help would be greatly appreciated, thanks:)