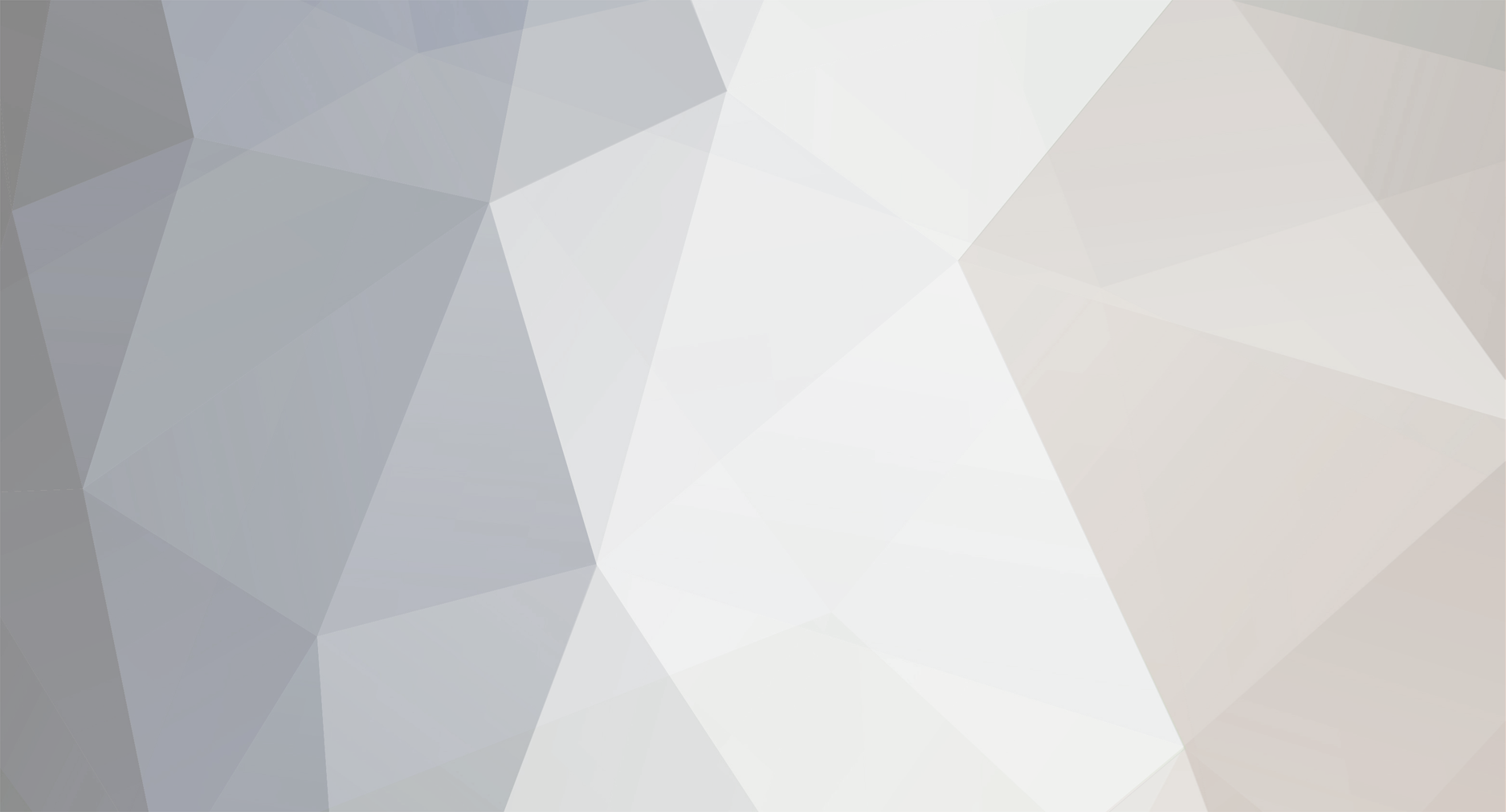
herghost
-
Posts
699 -
Joined
-
Last visited
Posts posted by herghost
-
-
Hi,
The page is just called from the browser directly, it then gets the details from one table (echo's here correctly) gets passed to the function to insert new post (echo s here correctly as well!) Then gets sent to the database in the WordPress insert_post function, this is where I am sure something dodgy is happening :-)
The tables all have the same encoding
-
It's just the question mark in replace of the ô which is the issue, there are no HTML declarations as its a php script. Utf8_bin and general should cover these characters as they are fine in one table, its just when it's moved, they are both utf8.
-
Thanks Kicken :-)
-
Hi all
I am having problems with french characters when using a custom function to upload posts from a database into wordpress
Firstly here is my code:
//This gets the values that is sent to the class to create post
<?php require_once('wp-content/plugins/PostAdder/index.php'); try { $host = 'localhost'; //$dbname = 'wordpress'; $dbname = 'HotelWifi'; $user = 'root'; $pass = ''; $DBH = new PDO("mysql:host=$host;dbname=$dbname", $user, $pass, array(PDO::ATTR_ERRMODE => PDO::ERRMODE_WARNING, PDO::MYSQL_ATTR_INIT_COMMAND => 'SET NAMES utf8') ); } catch(PDOException $e) { echo $e->getMessage(); } $STH = $DBH->query('select * from activepropertylist'); $STH->setFetchMode(PDO::FETCH_ASSOC); while($row = $STH->fetch()) { $hotelname = ucwords(utf8_decode($row['Name'])); $city = $row['City']; $STH1 = $DBH->query('select * from wp_terms WHERE name = "' . $city . '"'); $STH1->setFetchMode(PDO::FETCH_ASSOC); while($row1 = $STH1->fetch()) { $cat = $row1['term_id']; } $tcat = array($cat); post_a_newhotel($hotelname, $tcat) ; } ?>
// this is the function that fills the class and calls the wp_insert_post function
<?php /* Plugin Name: POstHotel Plugin URI: http://www.hotelwifiscore.com Description: Creates post from supplied data Version: 1 Author: davegrix Author URI: http://www.hotelwifiscore.com */ require_once('wp-load.php'); class wm_mypost { var $post_title; var $post_category; } function post_a_newhotel($title, $post_cat) { if (class_exists('wm_mypost')) { $myclass = new wm_mypost(); } else { class wm_mypost { var $post_title; var $post_category; } } // initialize post object $wm_mypost = new wm_mypost(); // fill object $wm_mypost->post_title = utf8_decode($title); $wm_mypost->post_category = $post_cat; $wm_mypost->post_status = 'publish'; array_walk_recursive($wm_mypost, function (&$value) { $value = htmlspecialchars(html_entity_decode($value, ENT_QUOTES, 'UTF-8'), ENT_QUOTES, 'UTF-8'); } ); wp_insert_post($wm_mypost); } ?>
The string I am having problems with is 'Best Western Premier Hôtel L'Aristocrate'
The result stored using the above is: Best Western Premier H?tel L'Aristocrate
database is set to utf8_general_ci
Can anyone shine any light on what to do?
-
Can you post your real code? The full and complete version that can actually run?
Apologies, some of it appears to have been stripped, please see below:
<?PHP ini_set('display_errors',1); ini_set('display_startup_errors',1); error_reporting(-1); //use latest minorRev 14 $url ='http://api.ean.com/ean-services/rs/hotel/v3/list?minorRev=14'; $url .= 'removed'; $url .= '&cid=55505'; $url .= '&locale=en_US&city=Dallas&stateProvinceCode=TX&countryCode=US&numberOfResults=3'; $url .= '&searchRadius=50'; //using the cache returns results much faster $url .= '&supplierCacheTolerance=MED_ENHANCED'; //dates and occupancy //$url .='&arrivalDate=19/02/2014&departureDate=09/05/2012&room1=2'; $header[] = "Accept: application/json"; $header[] = "Accept-Encoding: gzip"; $ch = curl_init(); curl_setopt( $ch, CURLOPT_HTTPHEADER, $header ); curl_setopt($ch,CURLOPT_ENCODING , "gzip"); curl_setopt($ch, CURLOPT_CUSTOMREQUEST, 'GET'); curl_setopt( $ch, CURLOPT_URL, $url ); curl_setopt( $ch, CURLOPT_RETURNTRANSFER, true ); $response = json_decode(curl_exec($ch)); $var = '@activePropertyCount'; //foreach($response as $data) // { // $mycount = $data->HotelList->$var; // // } $mycount = 1; foreach($response as $data) { try { $host = 'localhost'; $dbname = 'HotelWifi'; $user = 'root'; $pass = 'removed'; # MySQL with PDO_MYSQL $DBH = new PDO("mysql:host=$host;dbname=$dbname", $user, $pass); } catch(PDOException $e) { echo $e->getMessage(); } //print_r($data->HotelList->HotelSummary[$mycount-1]->hotelId); //print_r($data->HotelList->HotelSummary[$mycount-1]); $STH = $DBH->prepare("INSERT INTO HotelData (HotelID, HotelName, HotelCity, HotelCountry, LowRate, Long, Lat) values (:id,:name,:city,:country,:low,:long,:lat)"); $HotelID = $data->HotelList->HotelSummary[$mycount-1]->hotelId; $HotelName = $data->HotelList->HotelSummary[$mycount-1]->name; $HotelCity = $data->HotelList->HotelSummary[$mycount-1]->city; $HotelCountry = $data->HotelList->HotelSummary[$mycount-1]->countryCode; $LowRate = $data->HotelList->HotelSummary[$mycount-1]->lowRate; $Long = $data->HotelList->HotelSummary[$mycount-1]->longitude; $Lat = $data->HotelList->HotelSummary[$mycount-1]->latitude; $STH->bindParam(":id", $HotelID); $STH->bindParam(":name", $HotelName); $STH->bindParam(":city", $HotelCity); $STH->bindParam(":country", $HotelCountry); $STH->bindParam(":low", $LowRate); $STH->bindParam(":long", $Long); $STH->bindParam(":lat", $Lat); echo $HotelID . " " . $HotelName . " " . $HotelCity . " " . $HotelCountry . " " . $LowRate . " " . $Long . " " . $Lat; try { $STH->execute(); print_r($STH); } catch(PDOException $e) { echo $e->getMessage(); } } ?> </body> </html>
-
Hi Guys
Any ideas why nothing is being stored in my database? I have echoed out all the variables and they appear fine, likewise I am not getting any PDO errors from the try/catch, just nothing is being stored!
<?PHP
//for ($x=0; $x <=9; $x++)
//{
// print_r($url);
// print_r("
");
// print_r("");
// print_r ($response);
// print_r("");
//}
$var = '@activePropertyCount';
foreach($response as $data)
{
$mycount = $data->HotelList->$var;
}
// while( $mycount > -1)
// {
$mycount = 1;
foreach($response as $data)
{
try {
$host = 'localhost';
$dbname = 'HotelWifi';
$user = 'removed';
$pass = 'removed';
# MySQL with PDO_MYSQL
$DBH = new PDO("mysql:host=$host;dbname=$dbname", $user, $pass);
}
catch(PDOException $e) {
echo $e->getMessage();
}
//print_r($data->HotelList->HotelSummary[$mycount-1]->hotelId);
//print_r($data->HotelList->HotelSummary[$mycount-1]);
$STH = $DBH->prepare("INSERT INTO HotelData (HotelID, HotelName, HotelCity, HotelCountry, LowRate, Long, Lat) values (:id,:name,:city,:country,:low,:long,:lat)");
$HotelID = $data->HotelList->HotelSummary[$mycount-1]->hotelId;
$HotelName = $data->HotelList->HotelSummary[$mycount-1]->name;
$HotelCity = $data->HotelList->HotelSummary[$mycount-1]->city;
$HotelCountry = $data->HotelList->HotelSummary[$mycount-1]->countryCode;
$LowRate = $data->HotelList->HotelSummary[$mycount-1]->lowRate;
$Long = $data->HotelList->HotelSummary[$mycount-1]->longitude;
$Lat = $data->HotelList->HotelSummary[$mycount-1]->latitude;
$STH->bindParam(":id", $HotelID);
$STH->bindParam(":name", $HotelName);
$STH->bindParam(":city", $HotelCity);
$STH->bindParam(":country", $HotelCountry);
$STH->bindParam(":low", $LowRate);
$STH->bindParam(":long", $Long);
$STH->bindParam(":lat", $Lat);
echo $HotelID . " " . $HotelName . " " . $HotelCity . " " . $HotelCountry . " " . $LowRate . " " . $Long . " " . $Lat;
try
{
$STH->execute();
print_r($STH);
}
catch(PDOException $e) {
echo $e->getMessage();
}
}
//
//
//
//
//
// print_r($data->HotelList);
//
//
// $i = $i + 1;
?> -
HI guys
I am having some issues getting the results I want from a query.
Basically I have two tables. To keep things short and sweet ill use made up values
table a contains the field ID,Week
table b contains ID and type
Type can be either a 1 or a 0 and and the ID will match table A.
Table b can contain more than one entry for the same ID on table A with different types.
What I am trying to do is return all the ID's in table B that only contain a type of 0, for a specific week in table a. So if table B contains:
ID Type
1 1
1 0
2 0
2 0
3 1
3 1
I would only need to return ID 2
I have tried something like this
select a.id, b.id from b inner join a on b.id = a.id where a.week = '22' and b.type = 0
This issue is that it will return ID 1 and 2 as ID 1 contains a 0?
I hope this is clear enough!
Thanks for any pointers or help
-
Hi everyone
I am having some problems with a query and I am wondering if it is even possible with sql!
Basically I have two tables, a and b, which contain columns 1,2 & 3
What I am trying to do is copy values 2 and 3 from table 2 into table 1 columns 2 and 3 and set my own variable for column 1.
Is this possible or is select/insert only possible if you are copying an exact match from one table to another?
-
Hi Guys
I am trying to implement the rpxnow / janrain social login into my site.
I am having issues in returning the providername when the user logs in with facebook. Basically the results from janrain are returned as an array.
to get the providerid from Google I use this:
foreach ($auth_info as $key) { } $provider = $key['providerName'];
which correctly returns "Google", however when the user try's to login with facebook the result is "f" where the array output is "Facebook".
Using the demo file for outputting the array, the structure looks like this for google:
auth_info: array(2) { ["stat"]=> string(2) "ok" ["profile"]=> array(10) { ["url"]=> string(45) "https://plus.google.com/*deleted*" ["preferredUsername"]=> string(10) "*deleted*" ["email"]=> string(20) "*deleted*" ["name"]=> array(3) { ["givenName"]=> string(4) "Dave" ["familyName"]=> string(4) "*deleted*" ["formatted"]=> string(9) "*deleted*" } ["photo"]=> string(169) "http://www.google.com/ig/c/photos/public/*deleted*" ["displayName"]=> string(9) "*deleted*" ["identifier"]=> string(53) "https://www.google.com/profiles/*deleted*" ["verifiedEmail"]=> string(20) "*deleted*" ["providerName"]=> string(6) "Google" ["googleUserId"]=> string(21) "*deleted*" } }
and like this for facebook
auth_info: array(3) { ["stat"]=> string(2) "ok" ["profile"]=> array(12) { ["providerName"]=> string( "Facebook" ["identifier"]=> string(48) "http://www.facebook.com/profile.php?id=*deleted*" ["preferredUsername"]=> string( "*deleted*" ["displayName"]=> string(9) "*deleted*" ["email"]=> string(20) "*deleted*" ["name"]=> array(3) { ["formatted"]=> string(9) "*deleted*" ["givenName"]=> string(4) "Dave" ["familyName"]=> string(4) "*deleted*" } ["url"]=> string(33) "http://www.facebook.com/*deleted*" ["photo"]=> string(55) "https://graph.facebook.com/*deleted*/picture?type=large" ["utcOffset"]=> string(5) "01:00" ["address"]=> array(2) { ["formatted"]=> string(16) "*deleted*" ["type"]=> string(15) "currentLocation" } ["gender"]=> string(4) "male" ["verifiedEmail"]=> string(20) "*deleted*" } ["limited_data"]=> string(5) "false" }
I also tried to use $provider = ['profile']['providerName'] however this returns an error :
PHP Fatal error: Cannot use string offset as an array
How can I correctly return "Facebook"
Thanks
-
usernamechecker.php - is this file in the same directory as the one in your URL?
You should probably use the full URL just to be safe.
Install Firebug and you can easily debug this stuff.
Hi Jesirose
Many thanks for your response, firebug is fantastic!
fixed script - I was forgetting to update my html content for the span div
<script type="text/javascript"> $(document).ready(function() { $("#username").change(function() { var username = $("#username").val(); var msgbox = $("#status"); if(username.length < 6) { $("#status").html('<img src="img/cancel.png" /> Username must be at least 6 characters'); $('#username').css('background-image', 'url("../forms/img/cancel.png")'); document.getElementById("registerNew").disabled=true; } if(username.length > 5) { $("#status").html('<img src="img/loading.gif" /> ...Checking Username'); $('#username').css('background-image', 'url("../forms/img/asterisk_orange.png")'); document.getElementById("registerNew").disabled=true; jQuery.ajax({ type: "POST", url: "http://localhost/logtrav/forms/usernamechecker.php", data: 'username='+ username, cache: false, success: function(response) { if(response == 0) { $('#username').css('background-image', 'url("../forms/img/tick.png")'); document.getElementById("registerNew").disabled=false; $("#status").html(''); } else{ $('#username').css('background-image', 'url("../forms/img/cancel.png")'); $("#status").html('Username already in use'); } } }); } }); }); </script>
-
Hi Guys
This is my first attempt at an ajax script, so please be gentle!
Basically my script is meant to check a db to see if the username exists, however it just hangs on the loading.gif
<script type="text/javascript"> $(document).ready(function() { $("#username").change(function() { var username = $("#username").val(); var msgbox = $("#status"); if(username.length < 6) { $("#status").html('<img src="img/cancel.png" /> Username must be at least 6 characters'); $('#username').css('background-image', 'url("../forms/img/cancel.png")'); document.getElementById("registerNew").disabled=true; } if(username.length > 5) { $("#status").html('<img src="img/loading.gif" /> ...Checking Username'); $('#username').css('background-image', 'url("../forms/img/asterisk_orange.png")'); document.getElementById("registerNew").disabled=true; jQuery.ajax({ type: "POST", url: "usernamechecker.php", data: 'username='+ username, cache: false, success: function(response) { if(response = 0) { $('#username').css('background-image', 'url("../forms/img/tick.png")'); document.getElementById("registerNew").disabled=false; } else{ $('#username').css('background-image', 'url("../forms/img/cancel.png") Username already in use'); } } }); } }); }); </script>
usernamechecker.php
<?php include('../class/class.dbconnect.inc.php'); $username = trim(strtolower($_POST['username'])); $username = mysql_real_escape_string($username); $db = new dbconnect(); $db->connect(); $db->select('tbl_users','username','username="'.$username.'"'); $amount = count($db->getResult()); echo $amount; ?>
If I set a variable username in the checker manually and call the page I get the correct response from the database.
My table (tbl_users)
id, email, username, password
1, test@test.com, testuser, sha1pass
Can anyone point me in the direction of what I am doing wrong?
-
It's GET, not POST. Does that explain it?
Wow, thats 3 hours wasted ;-)
Many Thanks
-
Hi Guys
Firstly apologies if this is in the wrong section, my required output is php!
Basically I have the following jQuery which once a submit button is clicked my form opens in a fancybox window.
What I am trying to achieve is basically just got the POST values of two input boxes, name & email
<script type="text/javascript"> $(document).ready(function(){ $('#register').submit(function() { var url = $(this).attr("action") + "?" + $(this).serialize(); $.fancybox({ href: url, centerOnScroll: true, width: 450, 'type': 'iframe' }); return false; }); });
I have had a read through the .serialize docs but dont really understand how to get the results out into php variables.
-
Hi Guys
Thanks for all your responses, the data is coming from an API into the minecraft extension bukkit, my actual results from query display as:
"result": "success", "source": "getDirectory", "success": ["plugins/Essentials.jar", "plugins/Essentials/", "plugins/Essentials/config.yml", "plugins/Essentials/items.csv", "plugins/Essentials/upgrades-done.yml", "plugins/Essentials/warps/", "plugins/Essentials/worth.yml", "plugins/JSONAPI.jar", "plugins/JSONAPI/", "plugins/JSONAPI/config.yml", "plugins/JSONAPI/config_rtk.yml", "plugins/JSONAPI/methods.json", "plugins/JSONAPI/methods/", "plugins/JSONAPI/methods/permissions.json", "plugins/JSONAPI/methods/readme.txt", "plugins/JSONAPI/methods/remotetoolkit.json", "plugins/JSONAPI/methods/system.json", "plugins/JSONAPI/methods/world.json", "plugins/MinecraftRKitPlugin.jar", "plugins/PluginMetrics/", "plugins/PluginMetrics/config.yml"] }
So all I am trying to do is extract the results that end in .jar and ignore the rest so I can have a list of installed plugins.
-
Hi guys
I was wondering if anyone could point me in the right direction with this.
I am using JSON to return the contents of a directory and then using a foreach loop to print each one to a new row in a table.
Is there anyway to filter the results? Basically I just want to show the files that end in a .jar extension? Currently all sub directories and files are shown, however all the files I need to pull will be in the main directory.
This is what I am using:
<?php foreach ($installedplugins1['success'] as $v) { echo "<tr><td>".$v."</td>"; echo "<td><a href='index.php?dp=".$v."'>Disable Plugin</a></td>"; } ?>
Any hints or reading material?
Cheers
-
Thanks for your reply
the following displays all files and folders for /
$sym_dir = "/"; echo getcwd()."\n"; chdir ($sym_dir); echo shell_exec("ls -a");
However if I change $sym_dir back to /root/ then I just get the contents of the www folder displayed?
-
Hi Guys
I am struggling with a shell_exec command in the long run, but I am trying to work down the root cause.
The error I am getting with shell_exec is a file not found error, so I thought I would start with getting to the right folder.
I have this
$sym_dir = "/root/"; $cwd = getcwd(); echo getcwd()."\n"; chdir ($sym_dir); echo getcwd()."\n";
Which in theory should 1st display '/var/www' as it does and then display the contents of root, at least that is my understanding!
However my return is this /var/www /var/www
Why cannot I move outside the web root?
Cheers
Dave
-
Hi Guys
I cant seem to get unzip to work via php shell_exec
this is what I have:
$rworld = $_GET['restore']; $connect->call('remotetoolkit.stopServer',Array()); sleep(12); $nrworld = substr($rworld, 0,-15); $sftp = new Net_SFTP($host); $sftp->login($root_user, $root_pass); $sftp->delete('/root/minecraft/'.$nrworld.'/', true); echo shell_exec("unzip -jo /root/minecraft/saves/$rworld -d /root/minecraft/$nrworld"); sleep(5); $connect->call('remotetoolkit.startServer',Array());
Nothing is returned, it doesn't echo and the command is not executed. If I execute via terminal and change the $variables to the actual names it works fine, and I can ls with shell_exec successfully?
Any ideas?
-
Hi Guys,
What I am doing is making a backup of a folder by zipping it on a server which is outside of web root, downloading a local copy to a web accessible place and then uploading the zip file back to the non web folder. I am using phpseclib's sftp to achieve this.
The error I have is that when the file is uploaded to the non web area, the file is 0 bytes and cannot be used, the copy stored on the web accesible area is fine?
The code I am using:
<?php if(isset($_GET['backup'])) { $worldname = $_GET['backup']; //checks to see if upload if(!is_dir("backups")) //create backup directory if not exist { mkdir("backups", 0777); } mkdir("backups/".$worldname, 0777); //make filename directory $main_dest = "backups/".$worldname.""; //set backup destination $delpath = "backups/"; //path which will be deleted later $sftp = new Net_SFTP($host); $sftp->login($root_user, $root_pass); //login to sftp $get = "/root/minecraft/".$worldname."/"; //get correct directory for backup get_folder($sftp, $get, $main_dest ); //execute command Zip('backups/'.$worldname.'','backups/'.$worldname.''.date("dmy-H:i").'.zip'); //make zip of folder $sftp->put('/root/minecraft/backups/'.$worldname.''.date("dmy-H:i").'.zip','backups/'.$worldname.' '.date("dmy-H:i").'.zip',NET_SFTP_LOCAL_FILE); //put zip file from web folder to root/backup folder rrmdir($delpath); //delete old folder (function below) //unlink('backups/'.$worldname.''.date("dmy-H:i").'.zip'); deletes web copy header('Location: index.php'); }
The line which is causing the issue is:
$sftp->put('/root/minecraft/backups/'.$worldname.''.date("dmy-H:i").'.zip','backups/'.$worldname.' '.date("dmy-H:i").'.zip',NET_SFTP_LOCAL_FILE);The function is basically $remotefile, $data, $mode
Can anyone take a guess at what is causing the issue? PHP max upload size is 200mb, the files are around 5mb, the timeout is 360 seconds?
Cheers
Dave[/code]
-
Hi all, Its been a while since I looked at PHP and I have forgotten some basics
What I am trying to achieve is display category names, sub categories and results into a list
<ul id="nav"> <li><a href="#">Main Cat</a> <ul> <li><a href="#">Sub Cat 1</a> <ul> <li><a href="#">result</a></li> <li><a href="#">result</a></li> </ul> </li> <li><a href="#">Sub Cat 2</a> <ul> <li><a href="#">result</a></li> <li><a href="#">result</a></li> </ul> </ul> </li>
What would be the best way to organise my database? Have everything in one entry like:
main_id / sub_id / listing_id / listing_name
or separate them into a table for cats, one for sub cats and what parent it belongs to, then a table for the listings linking to the sub cat it belongs to?
I have confused myself no end on how then to display the results, so any help with would be gratefully received!
Thanks
Dave
-
Hi all
I am having some problems with the logic behind a simple idea.
Basically I have a list which returns the names of players from a json request, what I simply want to do is for the user to click on the name which then activates it, so basically they can then run a command directed at that name, so something like
Names a b c[onlick select] d Action Ban
Thanks
-
Hi all,
How do I get the following paths from the array separately in a foreach loop?
level.dat
level.dat_old
session.lock
uid.data
If I use
foreach($outer_dir as $key=>$val) [code] then I get a warning because its returning the folder as 'Array'. The files still upload, as well as a single file called Array. Can i just strip these from the foreach? [code] Array ( [data] => Array ( ) [level.dat] => level.dat [level.dat_old] => level.dat_old [players] => Array ( [herghost.dat] => herghost.dat ) [region] => Array ( [r.-1.0.mcr] => r.-1.0.mcr [r.0.0.mcr] => r.0.0.mcr ) [session.lock] => session.lock [uid.dat] => uid.dat ) herghost.dat
Many thanks from a confused user
-
Thanks, however I am getting an Unsupported operand types error on the union array line? I am guessing files() is empty and its trying to divide by 0?
-
Your are already writing in php so why are you opening another php block? try removing the <?php & ?> in the middle of your code!
wordpress, wp_insert_post and utf8_decode help
in PHP Coding Help
Posted
Got it!