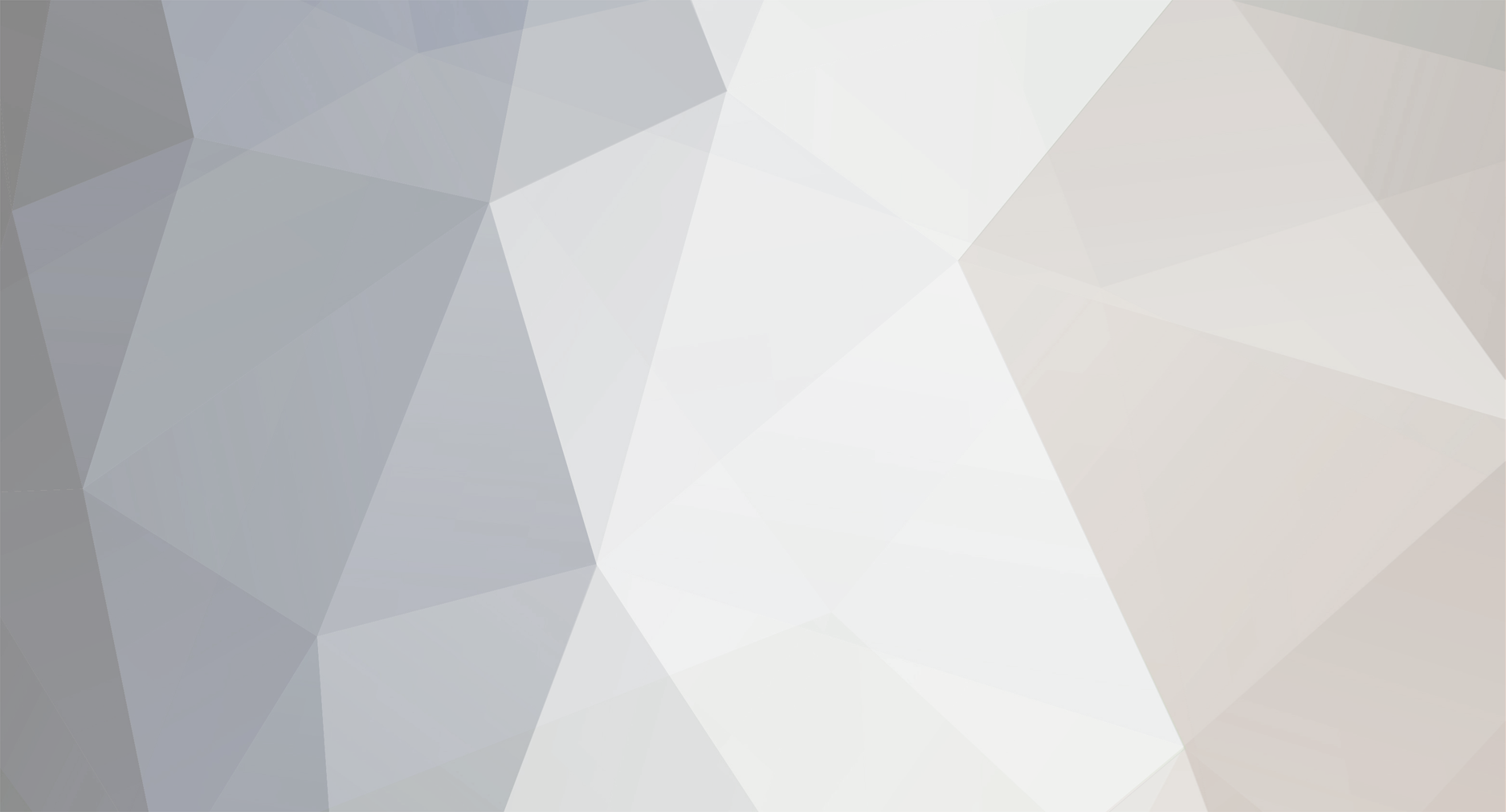
bschultz
Members-
Posts
486 -
Joined
-
Last visited
Everything posted by bschultz
-
Thanks for the help! After re-thinking this, I need to make sure that the mp3gain command only runs once per hour. I think I'll have to store the count in a flat file...and compare the count. Then, I won't have to compare the timestamp... Thanks again, though!
-
I need to check a file's timestamp (last modified) and if it's BEFORE 15 minutes past the current hour (xx:15:00) then run a shell command. Here's the code <?php ///////// start of time constants ///////// date_default_timezone_set('US/Central'); $fullyear = date('Y'); $year = date('y'); $month = date('m'); $day = date('d'); $now = date('Y-m-d-h'); $hour = date('g'); $hourplus1 = date('g', strtotime("Now +1 hour")); $hourminus1 = date('g', strtotime("Now -1 hour")); $ampm = date('A'); $rename_date = date('mdg'); $nowis = date('m'); $copy1 = "/home/rpbroadcasting/audio/S$rename_date.mp3"; if (file_exists($copy1)) { echo system('normalize-mp3 --mp3 '.escapeshellcmd($copy1)); $stamp1 = date ("n-j-Y g:i:s A.", filemtime($copy1)); $fifteenpastthishour = mktime(date("n"), date("j"), date("y"), date("g"), 15); if ($stamp1 <= $fifteenpastthishour) { echo system(escapeshellcmd("mp3gain -g 3 $copy1")); } } $copy2 = "/home/rpbroadcasting/audio/N$rename_date.mp3"; if (file_exists($copy2)) { echo system('normalize-mp3 --mp3 '.escapeshellcmd($copy2)); $stamp2 = date ("n-j-Y g:i:s A.", filemtime($copy2)); $fifteenpastthishour = mktime(date("n"), date("j"), date("y"), date("g"), 15); if ($stamp2 <= $fifteenpastthishour) { echo system(escapeshellcmd("mp3gain -g 1 $copy2")); } } $copy3 = "/home/rpbroadcasting/audio/W$rename_date.mp3"; if (file_exists($copy3)) { echo system('normalize-mp3 --mp3 '.escapeshellcmd($copy3)); $stamp3 = date ("n-j-Y g:i:s A.", filemtime($copy3)); $fifteenpastthishour = mktime(date("n"), date("j"), date("y"), date("g"), 15); if ($stamp3 <= $fifteenpastthishour) { echo system(escapeshellcmd("mp3gain -g 1 $copy3")); } } ?> The problem is, it's ALWAYS running the mp3gamin shell command...no matter what the timestamp of the file is. Where am I going wrong with this? Thanks. Brian
-
That did it...thanks!
-
I'm trying to print the results of an array inside an email message. It's working...accept that it prints the word Array before the values of the array. <?php $sql8 = "SELECT first_name, last_name, ump_id FROM ump_names WHERE `ump_id` IN (SELECT ump_id FROM scheduled_umps WHERE `game_id` = '$_SESSION[game_id_number]')"; //echo "$sql8<br />"; $rs8 = mysql_query($sql8,$dbc); $umps[] = array(); //while($row8 = mysql_fetch_array($rs8)) //{ //$umps[] = "$row8[first_name] $row8[last_name]."; //} while($row8 = mysql_fetch_array($rs8)){ $umps[] = "$row8[first_name]" . " " . "$row8[last_name]"; } $valuesgdf = implode(', ', $umps); // this sets the headers and other info for the email $headers = "From: $_SESSION[association_name] \n"; $headers .= "Reply-To: $_SESSION[association_name] \n"; $subject = "There has been a change in your $_SESSION[association_name] Schedule"; $message = "Here is the info for this date: \n\n Date - $day \n Game - $visitor at $home \n Start Time - $start \n GAME NOTES - $notes \n SCHEDULED OFFICIALS - $valuesgdf"; //this sends"SCHEDULED OFFICIALS - Array name1, name2, name3 etc" in the email... ?> How do I get "Array" to not show up prior to $valuesgdf? Thanks.
-
I have a page that has two forms. Form 1: - list baseball game information (who is playing, what time is the game, etc) Form 2: - lists umpires scheduled to work that game I would like to combine this to one form...to edit the info in the database (edit the start time, date etc...AND to edit the umpires scheduled to work the game...ON ONE FORM. Here's what I currently have: <?php $conn1 = mysql_connect($host, $username, $password); if (!$conn1) { echo "Unable to connect to DB: " . mysql_error(); exit; } if (!mysql_select_db($db, $conn1)) { echo "Unable to select mydbname: " . mysql_error(); exit; } $sql = "SELECT * from games WHERE `game_id` = '$_GET[game]' AND `association_id` = '$_SESSION[association_id]'"; $rs = mysql_query($sql,$conn1); $matches = 0; while ($row = mysql_fetch_assoc($rs)) { $matches++; ?> <form method="POST" action="edit_db_1_game.php"> <p> <input name="game_id" type="hidden" id="game_id" size="10" maxlength="10" value="<?php echo $row[game_id]; ?>" /> <br /> <strong><br /> THIS SECTION OF THE PAGE WILL EDIT THE GAME INFO...IF YOU WANT TO EDIT OFFICIALS, DO SO BELOW.</strong><br /> <br /> Date of game - <input name="day" type="text" id="day" size="10" maxlength="10" value="<?php echo $row[day]; ?>" /> <br /> <br /> Sport - <input name="sport" type="text" id="sport" size="20" maxlength="20" value="<?php echo $row[sport]; ?>" /> <br /> <br /> Visitor <input name="visitor" type="text" id="visitor" size="50" maxlength="50" value="<?php echo $row[visitor]; ?>" /> <br /> <br /> Home <input name="home" type="text" id="home" size="50" maxlength="50" value="<?php echo $row[home]; ?>" /> <br /> <br /> Start <input name="start" type="text" id="start" size="10" maxlength="30" value="<?php echo $row[start]; ?>" /> <br /> <br /> Notes <input name="notes" type="text" id="notes" size="50" maxlength="200" value="<?php echo $row[notes]; ?>" /> <?php echo "<br /><br />Send Email? <label><input type='checkbox' name='send_email' id='send_email' value='1' /></label><br /><br />"; ?> <input name="submit" type="submit" value="Submit" /> </form> <?php } if (! $matches) { echo ("<br /><br />There are no matches for this game ID"); } ?> <br /> <br /> -----------------------------------------------------------------------------------------------<br /> </p> <p><strong>THIS SECTION OF THE PAGE WILL EDIT THE SCHEDULED OFFICIALS...IF YOU WANT TO EDIT GAME INFO, DO SO ABOVE.<br /> <br /> </strong>The officials scheduled for this game are: <br /><br /> <?php $dbc = mysql_pconnect($host, $username, $password); mysql_select_db($db,$dbc); $sql = "SELECT first_name, last_name, ump_id FROM ump_names WHERE `ump_id` IN (SELECT ump_id, row AS thisrow FROM scheduled_umps WHERE `game_id` = '$_GET[game]')"; //echo "$sql<br />"; $rs = mysql_query($sql,$dbc); $num_rows = mysql_num_rows($rs); echo "<form method='POST' action=''>"; $r = 1; while ($r <= $num_rows) { while($row = mysql_fetch_array($rs)) { echo "<label><input type='radio' name='tba[$r]' id='tba[$r]' value='1' /></label> Set <b>$row[first_name] $row[last_name]</b> to TBA<br />"; echo "<label><input type='hidden' name='tbarow[$r]' id='tbarow[$r]' value='thisrow' /></label>"; echo "<label><input type='radio' name='delete[$r]' id='delete[$r]' value='1' /></label> Delete the \"slot\" for <b>$row[first_name] $row[last_name]</b> permanently - (IE you need one fewer official than you thought)<br /><br />"; echo "<label><input type='hidden' name='deleterow[$r]' id='deleterow[$r]' value='thisrow' /></label>"; $r++; } } ?> This works...but it's on two forms (which makes the "update db" script kinda clumsy). I've never done a table join to anything like it (which I'm assuming is what will make this work better)...but how can I combine this to one form...and add an "update db" section to the code? Thanks.
-
ok...thanks
-
It's working just fine with number_rows...I was just curious as to why it returned an empty set the other way.
-
Is it returning an empty set because of LIKE? If it were asking for an absolute match, would it throw an error?
-
MySQL returned an empty result set (i.e. zero rows). ( Query took 0.0005 sec ) if (mysql_num_rows($rs)==0) { echo "There are no matches for this letter..."; }...worked...thanks!
-
The following code isn't working...and I don't know why... <?php $dbc = mysql_pconnect($host, $username, $password); mysql_select_db($db,$dbc); $sql = "SELECT * FROM ump_names WHERE `association_id` = '$_SESSION[association_id]' AND `is_active` = '1' AND `last_name` LIKE '$_GET[first]%' order by last_name ASC"; //echo "$sql<br />"; $rs = mysql_query($sql,$dbc); if (!$rs) { echo "There are no matches for this letter..."; } while($row = mysql_fetch_array($rs)) { echo "<div class='style5'><strong>$row[first_name] $row[last_name]</strong><br />"; echo "$row[address]<br />"; echo "$row[city], $row[state] $row[zip]<br />"; echo "Home - $row[home_number]<br />"; echo "Cell - $row[cell_number]<br />"; echo "Work - $row[work_number]<br />"; echo "<a href='mailto:$row[email]'>$row[email]</a></div><br />------------------------<br />"; } ?> If there are matches with $_GET[first], everything works great...if there are no matches, there's no error (error reporting is turned on ) and the line There are no matches for this letter... never is echoed. Any help on what I'm doing wrong...???
-
I've now been asked by my bosses to add a feature! Why can't they ever think of EVERYTHING at once? So, the code above has this order: song commercial song commercial etc... Now, they want this: song song song feature (this is new) commercial song song song feature (this is new) commercial etc... How can I add more rotations of songs...without messing with the order of the commercials and AND features?
-
Is join faster? I did find the problem... $values2 = implode(', ', $priority_array); should be $values2 = implode(',', $priority_array); //remove space after comma I'll redo the code if it will run better.
-
I stumbled across find_in_set...which still isn't working. <?php ////////////get priorities $sql = "SELECT ump_id FROM ump_priority WHERE `ump_id` IN ($values) ORDER BY priority DESC"; echo "priorities select = $sql<br />"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_assoc($rs)) { $priority_array[] = $row['ump_id']; } print_r ($priority_array); $values2 = implode(', ', $priority_array); $sqlx = "SELECT ump_id, first_name, last_name FROM ump_names WHERE `ump_id` IN ($values2) ORDER BY find_in_set(ump_id, '$values2')"; echo "get names select = $sqlx<br />"; $rsx = mysql_query($sqlx,$dbc); echo "<br /><br /><form id='form1' name='form1' method='post' action='edit_officials_1_game.php'>"; echo "<label><select name='officials' id='officials'>"; while($rowx = mysql_fetch_array($rsx)) { $full_name = "$rowx[first_name]" . " $rowx[last_name]"; echo "<option value='$rowx[ump_id]'>$full_name</option>"; } echo "</select></label><br /><br /><label><input type='submit' name='button' id='button' value='Submit' /></label></form>"; ?> The order of the array after priorities select = is correct...but when the get_names_select results come back, they are ordered by ump_id...1, 2, 4, 5, 6, 10, 14, 15, 18.
-
Thanks for the help with the extra quotes. That got me all the results that it should have. So, how can I order the final drop box by the priority...which is in a prior query?
-
Thanks again for the help... The next part of this code is to take that list of id's...sort them by priority, and spit out a drop box. <?php $sql = "SELECT ump_id, priority FROM ump_priority WHERE `ump_id` IN ('$values') ORDER BY priority DESC"; echo "priorities select = $sql<br />"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_array($rs)) { $priority_array[] = $row['ump_id']; } $values2 = implode(', ', $priority_array); $sqlx = "SELECT ump_id, first_name, last_name FROM ump_names WHERE `ump_id` IN ('$values2')"; echo "get names select = $sqlx<br />"; $rsx = mysql_query($sqlx,$dbc); echo "<br /><br /><form id='form1' name='form1' method='post' action='edit_officials_1_game.php'>"; while($rowx = mysql_fetch_array($rsx)) { $full_name = "$rowx[first_name]" . " $rowx[last_name]"; echo "<label><select name='officials' id='officials'>"; echo "<option value='$rowx[ump_id]'>$full_name</option>"; } echo "</select></label><br /><br /><label><input type='submit' name='button' id='button' value='Submit' /></label></form>"; ?> This returns... Two problems...the highest priority is id #4...so why isn't 4 showing up first...and why is it only returning 1 row...instead of the 9 that it should?
-
Cool...thanks!
-
I'm trying to write a subquery (my first)...and I can't get it to work right. <?php session_start(); if( !isset( $_SESSION['is_admin']) ){ echo "You don't have access to this page, or you're not logged in...<meta http-equiv=Refresh content=0;url='/secure/index.php'>"; } //include "../config.php"; putenv("TZ=US/Central"); //connect to database $dbc = mysql_pconnect($host, $username, $password); mysql_select_db($db,$dbc); //get info of game $sql = "SELECT sport,home,day,game_id FROM games WHERE `game_id` = '$_GET[game]'"; //echo "$sql<br />"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_array($rs)) { $what_sport = $row['sport']; $home_team = $row['home']; $day_of_game = $row['day']; $weekday = date('w', strtotime($day_of_game)); $game_id = $row['game_id']; } //get all officials working today $sql = "SELECT day,game_id FROM games WHERE `day` = '$day_of_game'"; //echo "$sql<br />"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_assoc($rs)) { $array_of_date[] = $row['game_id']; } $values = implode(', ', $array_of_date); $sql = "SELECT ump_id FROM ump_names WHERE `$what_sport` = '1' AND ump_id NOT IN (SELECT ump_id, sport, school FROM bad_school WHERE `sport` = '$what_sport' AND `school` = '$home_team') AND ump_id NOT IN (SELECT ump_id, day, dow FROM days_off WHERE `day` = '$day_of_game') AND ump_id NOT IN (SELECT ump_id, game_id FROM scheduled_umps WHERE `game_id` = '$values') "; echo "$sql<br />"; $rs = mysql_query($sql,$dbc); if ($rs){ echo "This worked"; } else{ echo mysql_errno().": ".mysql_error()."<BR>"; } while($row = mysql_fetch_assoc($rs)) { $available_umps_array[] = $row['ump_id']; } $values = implode(', ', $available_umps_array); echo "$values<br />"; ?> Here is the error What is 1241: Operand should contain 1 column(s)...and how do I fix it.
-
people around this board are smart! Thanks!
-
I'm trying to run a query based on matches of an array (that I've put in a foreach loop). Here's the code... <?php foreach($step_three as $step_three_final) //$step_three is an array { //echo $step_three_final . "<br />"; $sqlx = "SELECT ump_id, priority FROM ump_priority WHERE `ump_id` = '$step_three_final' ORDER BY priority DESC"; echo $sqlx; $rsx = mysql_query($sqlx,$dbc); while($rowx = mysql_fetch_array($rsx)) { echo "$rowx[ump_id]<br />"; } } ?> This results is this This would answer my problem...why isn't this ordering by the priority properly?...of course it's because it's running 9 queries. What is the right way to run this query...so that it will have all the records in one query...so that I can order by the priority? Thanks.
-
thanks...that worked!
-
no difference...still doesn't echo anything
-
I'm trying to come up with some code...and need to echo certain things based on which query failed. There are several query's and while loops nested together. I'm trying to use this... <?php $rs = mysql_query($sql,$dbc); if (! $rs) { echo "You aren't scheduled to work in the next seven days...but check back often as the schedule is adjusted often."; } $matches = 0; while ($row = mysql_fetch_assoc($rs)) { $matches++; //rest of code here...which work when there are matches... ?> With no matches, it isn't echoing anything...instead of "You aren't scheduled to work in the next seven days...but check back often as the schedule is adjusted often." If I echo out $rs, I get RESOURCE ID #6 Any ideas? Thanks!
-
By the way...here's the echo of the $sql: As far as I can tell...that looks right to me.
-
I'm having a problem with a multiple table select. <?php //get who is already working list $sql = "SELECT ump_id.scheduled_umps, game_id.games, game_id.scheduled_umps, day.games FROM scheduled_umps, games WHERE `day.games` = '$game_day_array' AND `game_id.scheduled_umps` = '$game_id_array'"; echo $sql; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_array($rs)) { echo $row['ump_id']; $already_working_array[] = $row['ump_id.scheduled_umps']; } if (!$rs) { echo "i found nothing here"; } ?> This returns [quote] Warning: mysql_fetch_array(): supplied argument is not a valid MySQL result resource in /home/briansch/public_html/bemidjiumps.com/secure/pages/schedule_game.php on line 69 i found nothing here [/quote] AND [quote] Warning: array_diff() [function.array-diff]: Argument #2 is not an array in /home/briansch/public_html/bemidjiumps.com/secure/pages/schedule_game.php on line 83 [/quote] The table scheduled_umps has the game_id and the ump_id for that game. The table games has the game_id and the day. So, I need the day (defined earlier in the code...and working...) of ONE game that day. Then, I need to find ALL the game_id's of that day (all from the games tables). Then, I need to compare the game_id's of that day, to the scheduled_ump table, to see which ump_id is already scheduled that day. Make sense? Any idea's what I'm doing wrong? Thanks!
-
I need to compare several arrays...to narrow down a bunch of mysql selects. I can't figure out which of the array_diff, array_unique, array_merge options there are. I need to get an array of umpires (baseball), then compare that to the array of umpires that can work a given level, then compare that to the array of umpires that can work at that school, and finally compare that to the array of umpires who have asked for the day off. In the code below, all of the selects work...the echo's that are commented out printed out what I expected them to. I'm having a problem with the array comparisons at the end of this code: <?php //connect to database $dbc = mysql_pconnect($host, $username, $password); mysql_select_db($db,$dbc); //get info of game $sql = "SELECT sport,home,day FROM games WHERE `game_id` = $_GET[game]"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_array($rs)) { $game_array = $row['sport']; $home_team_array = $row['home']; $game_day_array = $row['day']; } //list of all umpires in association $sql = "SELECT ump_id FROM ump_names WHERE '$_SESSION[association_id]' = `association_id`"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_array($rs)) { $name_array[] = $row['ump_id']; // echo "<br /><br />All Ump ID's Array = "; // foreach($name_array as $name_array_final) { // echo $name_array_final . "<br />"; // } } //get bad schools list $sql = "SELECT ump_id FROM bad_school WHERE '$_SESSION[association_id]' = `association_id` AND `school` = '$home_team_array' and `sport` = '$game_array'"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_array($rs)) { $bad_school_array[] = $row['ump_id']; // echo "<br /><br />Bad School Array = "; // foreach($bad_school_array as $bad_school_array_final) { // echo $bad_school_array_final . ", "; // } } //get official list at this game's level $sql = "SELECT ump_id FROM ump_names WHERE `association_id` = '$_SESSION[association_id]' AND `$game_array` = '1'"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_array($rs)) { $official_level_array[] = $row['ump_id']; // echo "<br /><br />Officials that can work this level of game = "; // foreach($official_level_array as $official_level_array_final) { // echo $official_level_array_final . ", "; // } } //get vacation list $sql = "SELECT ump_id FROM days_off WHERE '$_SESSION[association_id]' = `association_id` AND `day` = '$game_day_array'"; $rs = mysql_query($sql,$dbc); while($row = mysql_fetch_array($rs)) { $days_off_array[] = $row['ump_id']; // echo "<br /><br />Officials that are on vacation this day = "; // foreach($days_off_array as $days_off_array_final) { // echo $days_off_array_final . ", "; // } } //$first_step = array_merge($official_level_array, $name_array); $first_step = array_merge($official_level_array, $name_array); $first_step_part_2=array_unique($first_step); //$second_step = array_merge($bad_school_array, $first_step); $second_step = array_merge($bad_school_array, $first_step_part_2); $second_step_part_2=array_unique($second_step); //$third_step = array_merge($days_off_array, $second_step); $third_step = array_merge($days_off_array, $second_step_part_2); $third_step_part_2=array_unique($third_step); echo "These umpires are available for this game:<br />"; foreach ($third_step as $iamavailable) { echo "$iamavailable<br />"; } ?> This prints out: It should only print out 4, 5, 6, 10, 14, 15. What I want to do is: compare $official_level_array and $name_array...only keep those that are in $official_level_array. Then, compare that result with $bad_school_array...removing those in the $bad_school_array...and keeping the ones that are left. Then, compare that result, with $days_off_array...removing those in the $days_off_array...and keeping the ones that are left. Print out what's left... Where am I going wrong?