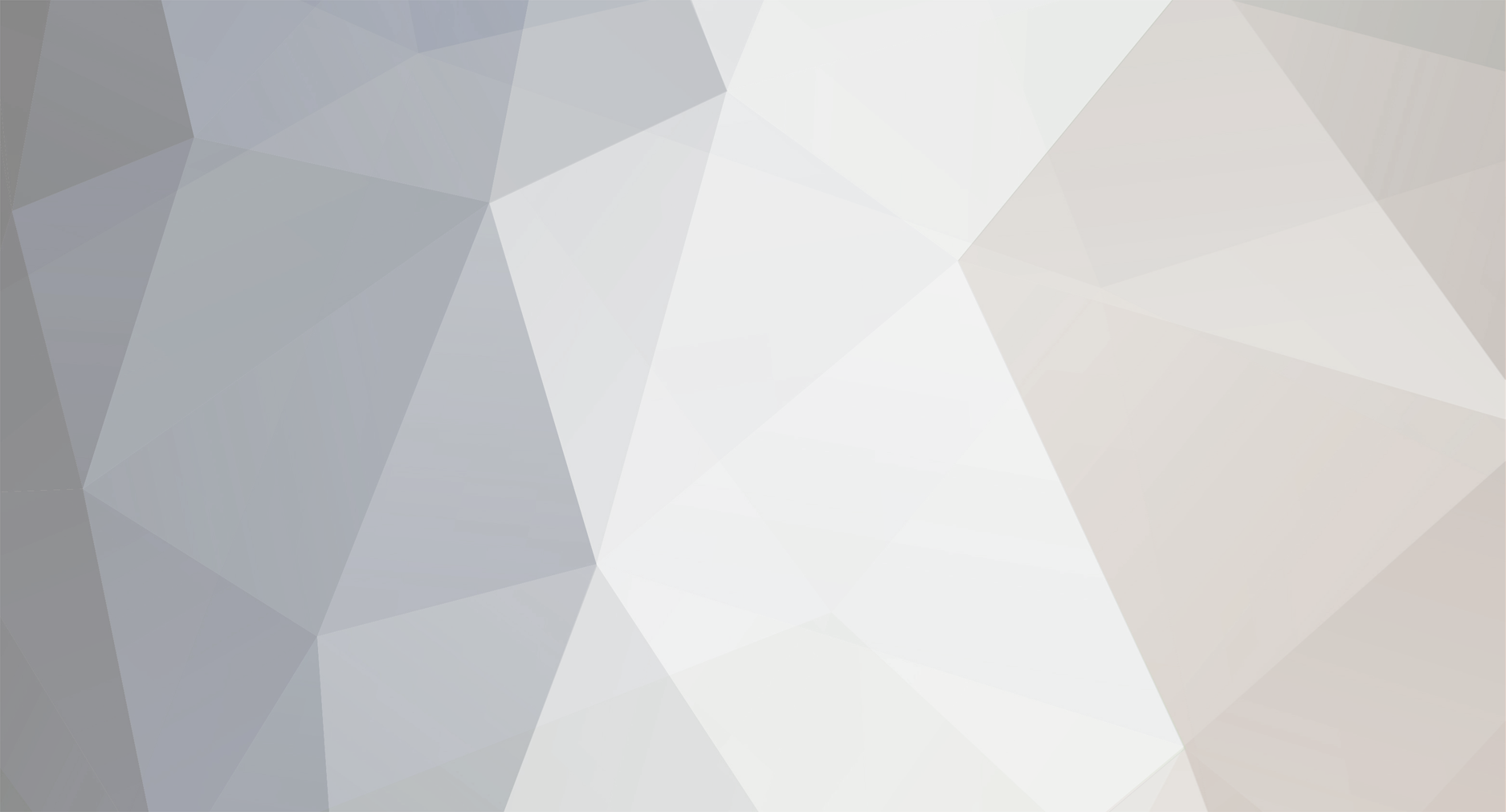
Popple3
-
Posts
8 -
Joined
-
Last visited
Never
Posts posted by Popple3
-
-
Hi,
I wrote a quick script that *should* check my friends timeline on twitter and dump certain info into an SQL database.
The idea is that the script will check if a status exists in my SQL, and if it doesn't, add it, and send an SMS to me using a class my friend wrote (SMS isn't in the script yet, I just want SQL working first). I will then do a cron job on this to check from time to time.
Here is the code (without certain, obvious data):
<?php //------CONNECT TO DATABASE----- $db = mysql_connect("$sql_server", "$sql_user", "$sql_pass") or die("Connection Failure to Database"); mysql_select_db("$sql_db",$db); //------------------------------ //------GET FRIEND STATUSES----- $ch = curl_init(); curl_setopt ($ch, CURLOPT_URL, 'http://user:pass@twitter.com/statuses/friends_timeline.xml'); curl_setopt ($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt ($ch, CURLOPT_CONNECTTIMEOUT, 5); $xmldata = curl_exec($ch); curl_close($ch); //------------------------------ $xml = new SimpleXMLElement($xmldata); //parse friend statuses function checkStatus($text) { //Check if status is in SQL database $checksql = "SELECT * FROM tweets WHERE status='$text'"; $checkresult = mysql_query($checksql); if ($checkresult) { return true; } else { return false; } } function insertStatus($id, $user, $text, $time) { //Insert status into database $insertsql = "INSERT INTO tweets (id, user, status, time) VALUES ('$id', '$user', '$text', $time')"; $insertresult = mysql_query($insertsql); } //-----GO THROUGH EACH STATUS----- foreach($xml->status as $status) { $tweetid = $status->id; $tweettime = $status->created_at; $tweettext = $status->text; $tweetuser = $status->user->screen_name; $check = checkStatus($tweettext); //returns true or false if ($check=false) { insertStatus($tweetid, $tweetuser, $tweettext, $tweettime); //inserts into SQL database echo $tweetuser.": ".$tweettext."\n"; } } //-------------------------------- ?>
Any ideas why it's not working? I'm stumped!
-
Well, first of all, why? ???
Second, use height:100%
-
Shouldnt it be :: ?
I'm new to objects so I'm not sure.
When I do that I get
Parse error: parse error, unexpected T_PAAMAYIM_NEKUDOTAYIM in /home/www/popple3.com/picasa.php on line 13
-
Yeah, I meant to actually include it
<?php include('xmlparser.php'); //Get the XML document loaded into a variable $xml = file_get_contents('http://picasaweb.google.com/data/feed/api/user/popple3?kind=album'); //Set up the parser object $parser = new XMLParser($xml); //Work the magic... $parser->Parse(); //Echo the plot of each <movie> foreach($parser->document->entry as $entry) { $imgurl = $entry->media:group->media:thumbnail->tagAttrs['url']; echo $entry->title[0]->tagData; echo "<img src=\"" . $imgurl . "\">"; } ?>
And then that just returns:
Parse error: parse error, unexpected ':' in /home/www/popple3.com/picasa.php on line 14
-
Sadly, "\:" doesn't escape colons...
-
I'm parsing XML, but many of the tags in the XML file (which I have no control over) have colons ( : ) in them, and as a result i get an "Unexpected ':'" parse error. Is there a way of escaping these colons, or removing them from the XML before it is parsed? I'm using PHP4...
Thanks in advance
-
I can get the information from tags, however there are two tags in the XML (that I have no control over) called media:group and media:thumbnail. When entered (as in the code below), i get an "unexpected ':'" parse error. I'm looking for a simple solution. I'm not very familiar with PHP, so examples would be helpful. Thanks in advace
<?php include('xmlparser.php'); //Get the XML document loaded into a variable $xml = file_get_contents('http://picasaweb.google.com/data/feed/api/user/popple3?kind=album'); //Set up the parser object $parser = new XMLParser($xml); //Work the magic... $parser->Parse(); //Echo the title and image of each <entry> foreach($parser->document->entry as $entry) { $imgurl = $entry->media:group->media:thumbnail->tagAttrs['url']; echo $entry->title[0]->tagData; echo "<img src=\"" . $imgurl . "\">"; } ?>
Putting data from XML into SQL using PHP
in PHP Coding Help
Posted
Hmmm.... Neither of them displays anything... Which is weird...