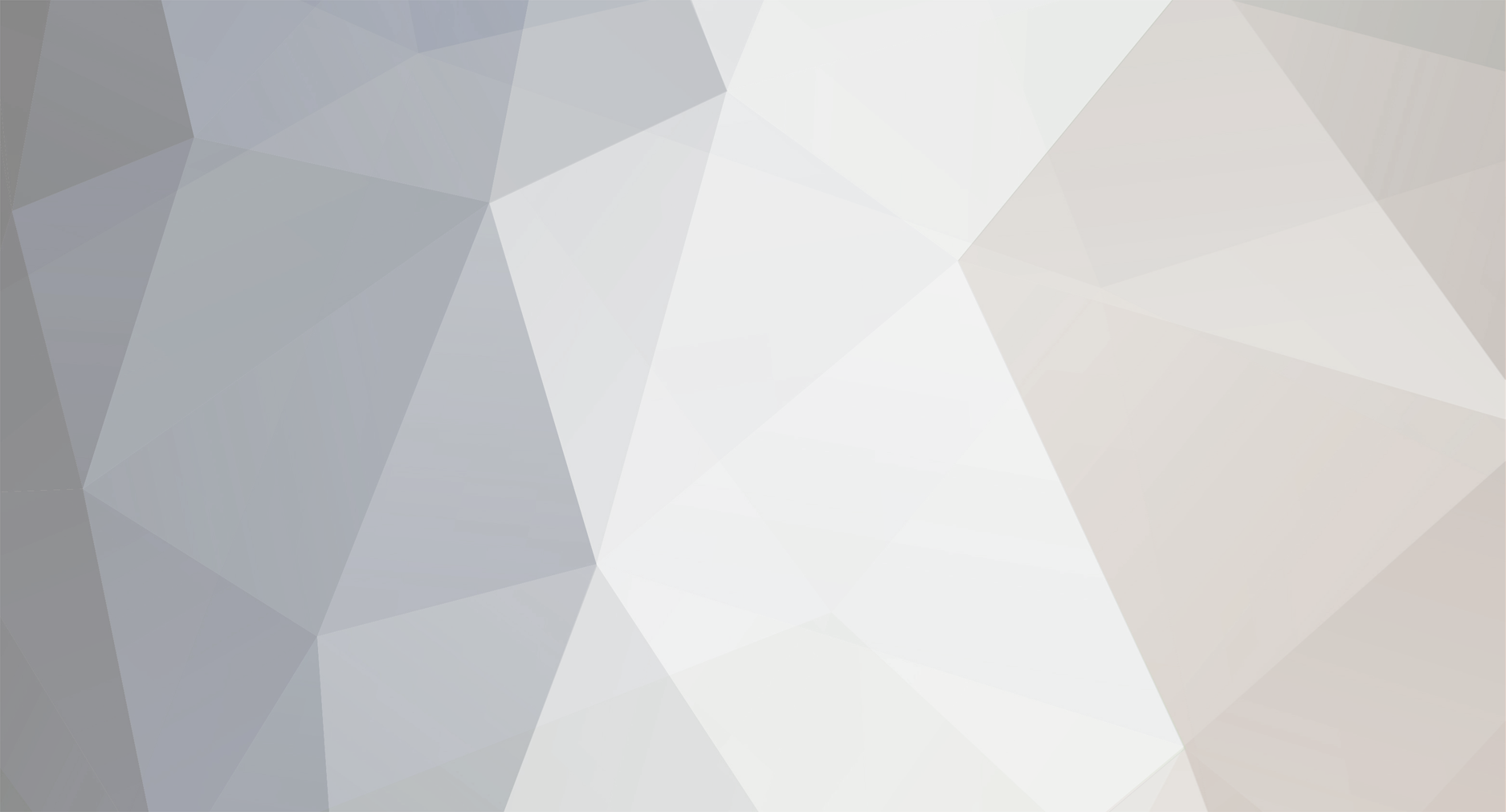
rhodesa
-
Posts
5,253 -
Joined
-
Last visited
Posts posted by rhodesa
-
-
If you have logins, I assume you have a database to track meta data about the user? Aka username, password, etc. Just add a new column for logins, and each time the login, increase the number.
-
you can't output anything before using header(). what is the rest of the code for page2.php?
-
whats <<<EOD and EOD; ?
Dont you want quotes?
edit: cool didnt know about Heredoc.. neat
personally...i don't like that way of outputting...i think it's messy...i'd rather just close and re-open the php tags
-
-
no problems...it will keep following the redirects
-
there is nothing wrong with the script that i can see
-
Last Name:<input type="text" size="12" name="lname" value="<?php echo substr($_GET['pt'],0,strpos($_GET['pt'],','));?>"/>
-
what do you mean by "it won't pull when I try from php"? no rows are returned? are you sure you are connected to the right database? try updating this line:
$query2 = mysql_query("SELECT * FROM photog, shoot WHERE photog.actual=shoot.photog ORDER BY id Asc") or die(mysql_error());
-
is there a field in the DB you can sort by? like an auto_increment id? assuming it's called script_id, you would just do:
<select name="script" id="script"> <option value="" selected>- Script -</option> <?php //Include connection include("conn.php"); $sql = "SELECT DISTINCT(script) FROM scripts ORDER BY script_id DESC"; $result = mysql_query($sql); while($row = mysql_fetch_array($result)) { ?> <option value="<? echo $row["script"]; ?>"><? echo $row["script"]; ?></option> <? } ?> </select>
-
how about:
<select name="script" id="script"> <option value="" selected>- Script -</option> <?php //Include connection include("conn.php"); $sql = "SELECT DISTINCT(script) FROM scripts"; $result = mysql_query($sql); while($row = mysql_fetch_array($result)) { ?> <option value="<? echo $row["script"]; ?>"><? echo $row["script"]; ?></option> <? } ?> </select>
-
yes, that is what i would do
-
So, there is no problem then? This was the easiest problem EVER!
-
What is the entire code...my guess is you change the value of $d between this line:
$d = implode(',', $clean);
and this one:
<META HTTP-EQUIV="refresh" content="0;URL=http://localhost/td/ref/deny.php?d=$d">
-
$i = strrpos($file,'-'); $time = substr($file,0,$i+1);
or
list($time) = explode('-',$file);
-
use either this:
$r = pg_fetch_array($pull, NULL, PGSQL_NUM);
or
$r = pg_fetch_row($pull);
-
to elaborate...the parent would look like:
<input type="button" value="Open Child" onclick="child_win = window.open('child.html');"> <input type="button" value="Alter Child" onclick="child_win.document.getElementById('child_ele').innerHTML = 'Here is the new text';">
and the child like this:
<p id="child_ele">Here is some text before</p>
-
you are defining all the code in a function, but never executing the function...it should be more like this:
//check total size of directory and set variable $totalsize function dir_size($dir) { $totalsize=0; if ($dirstream = @opendir($dir)) { while (false !== ($filename = readdir($dirstream))) { if ($filename!="." && $filename!="..") { if (is_file($dir."/".$filename)) $totalsize+=filesize($dir."/".$filename); if (is_dir($dir."/".$filename)) $totalsize+=dir_size($dir."/".$filename); } } } closedir($dirstream); return $totalsize; } $totalsize = dir_size($dir);
-
yeah.....unchecked checkboxes don't get passed by the form
-
Help
in MySQL Help
that's right...can't do that...try this:
http://dev.mysql.com/doc/refman/5.0/en/outer-join-simplification.html
-
ok...couple of things....
first: you don't set $totalsize...it's in your function, but not in your script
second: after any header() call, you should always have an exit; to make sure the script doesn't keep working:
if(($_FILES["user_file"]["size"] > 2097153)) { header ('Location: upload_errorsize.php'); exit; }
...do that for all your header()s
third: make sure you sql is working by changing this:
$result2 = mysql_query($query,$link);
to this:
$result2 = mysql_query($query,$link) or die (mysql_error($link));
fourth: these lines are redundant and should be removed since it already happens at the top:
$link=mysql_connect($hostname, $adminuser, $adminpass) or die("couldnt connect to database"); mysql_select_db("$database") or die("Could not select database");
-
Help
in MySQL Help
Use OUTER JOIN instead
-
mysqli classes are available if the module is enabled:
http://us.php.net/manual/en/mysqli.installation.php
The method query() in the mysqli object just returns an object. The code inside query() creates a MySQLi Result Instance and then just returns it
-
change this:
$result = mysqli_query($cxn, $query);
to
$result = mysqli_query($cxn, $query) or die(mysqli_error($cxn));
-
If I remember right...it was just:
Step 1: http://us.php.net/manual/en/mssql.requirements.php
Step 2: In your php install dir, in the ext folder, make sure there is php_mssql.dll
Step 3: Enabled it in your php.ini file
Step 4: Restart webserver and confirm it's working with a phpinfo() page
but I did it with 5.2, not 5.3
PHP - Get HTML Source and read elements
in PHP Coding Help
Posted
Also be aware, for this to work, the HTML content needs to be 100% valid. The majority of webpages on the internet are NOT valid HTML.