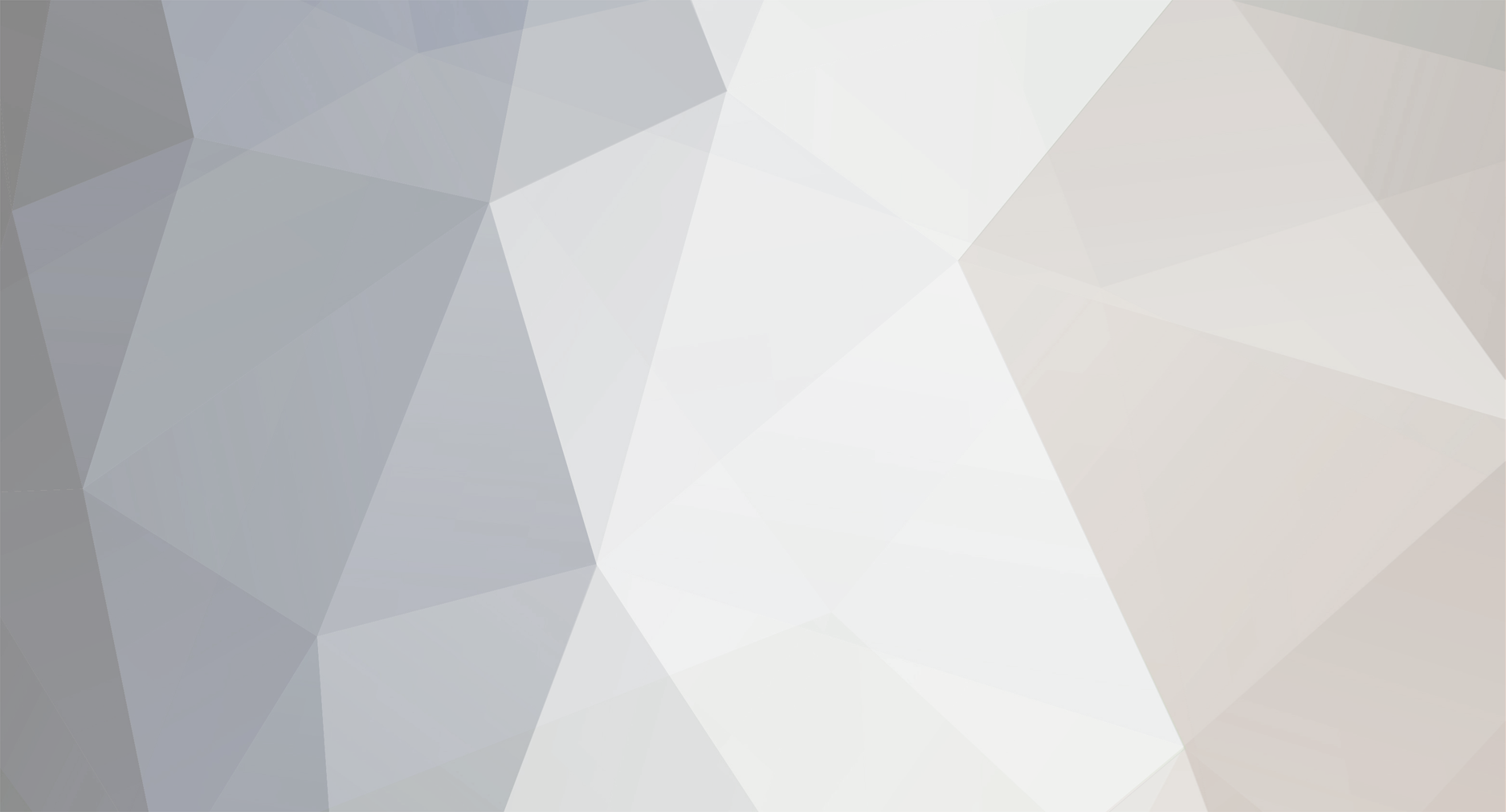
laffin
Members-
Posts
1,200 -
Joined
-
Last visited
Everything posted by laffin
-
Exporting an extremely large, poorly designed database
laffin replied to SchweppesAle's topic in PHP Coding Help
you shouldnt be storing all the results in php, and waiting to exhaust the memory limits. depending on the data, check to see if it uses some common csv delimiters. (even tho comma may sound good, likelyhood of a comma being in a descriptrion is very high, consider using | but the sql fetch loop should be your csv output loop some sample code $filename = 'database.csv'; $file = fopen ($filename, "a"); while($row = mssql_fetch_row($result)) { fputcsv($file,$row,','); } fclose($file); -
Not shure why ya used tinyints, but based on your db, i would prolly opt for something like $ethnics=array('Asian,'Black','East Indian','Mid Eastern','Hispanic','Native','Pac Haw','White EU'); $string=null; if($row=mysql_fetch_assoc(mysql_query("SELECT * FROM ethn WHERE userId = 'test'"))) { $user_eth=array_values(array_pop(array_shift($row))); foreach($user_eth as $key=>$val) { if($val) $string[]=isset($ethnics[$key])?$ethnics[$key]:$row['othr_Ethn_txt']; } $string=implode(', ',$string); } if(empty($string)) $string="Unknown';
-
str_replace looking for a better method. or ideas on how to improve
laffin replied to monkeytooth's topic in PHP Coding Help
a nice tutorial, for the basics a online regex tester one of my favorite tools for windows is expresso -
file_put_contents($filename,'');
-
well u got the pefect task to do so, right now. I will try to make this easy, and not complex to understand its not a tutorial, but some explanations and already made code. Intergers - Most computers these days, you'll hear are 32 bits. This just means that whole numbers are represented by 32 bis within the computer. with that in mind, u can say, you can have a bit flag system with 32 flags per integer in php Okay, so with that in mind. we want to go ahead and assign each bit position with a specific flag. so we want to make a list, and number them accordingly email, birthday, gender, real name to assign them a positiong, we want to use a bitwise operator known as left bit shift. like this: $private_email = 0; $private_birthday = 1; $private_gender = 2; $private_realname = 3; since we are working with 32 bit integers the position will be in the range of 0-31 (yes, zero counts). now let me introduce some functions for setting and reading specific bit. function setbit($flags,$position) { return $flags|(1<<$position); } function resetbit($flags,$position) { return $flags&(~(1<<$position)); } function togglebit($flags,$position) { return $flags^(1<<$position); } function readbit($flags,$position) { return ($flags|(1<<$position))?1:0; } even tho they are small functions, we now have enough tools to write our code you turn on a bit flag in our integer with setbit. turn it off with resetbit toggle it (if on, turn it off, if off turn it on) with togglebit and of course check a flag with readbit. so lets write a little script using the above function, with our bit flags all together header('Content-type: text/plain'); // we just want a quick display, so we can use plain text to display our sample // Function to aid in our quick display, just adds a newline to a string function nle($str) { echo "{$str}\n"; } $flags=0; // this is our initial setting of our flags $flags=setbit($flags,$private_emal); // turn on private email flag $flags=setbit($flags,$private_gender); // turn on private gender flag nle($flags); $flags=resetbit($flags,$private_gender); // turn off the private gender flag nle($flags); $flags=togglebit($flags,$private_birthday); // since its off, this will turn it on nle($flags); nle("Private Email: ".readbit($flags,$private_email)); nle("Private Birthday: ".readbit($flags,$private_birthday)); nle("Private Gender: ".readbit($flags,$private_gender)); a simple but pretty self explanatory script
-
str_replace looking for a better method. or ideas on how to improve
laffin replied to monkeytooth's topic in PHP Coding Help
the pattern string is pretty simple ( ) - grab this as a variable [ ] - charaters in range ^ - negate (characters not in this range) a-zA-Z0-9- - alpha-numerics as well as - so it should grab everything else, and change it to '-' -
You can also use bit flags. bools are simple to use, but if yer dealing with a lot of flags, a bit flag system is more compact. but bit flags do take a lil more coding in sql & php.
-
str_replace looking for a better method. or ideas on how to improve
laffin replied to monkeytooth's topic in PHP Coding Help
not tested $seo=preg_replace('/([^a-zA-Z0-9])/','-',$string -
Please help me revise this log in code.
laffin replied to shinichi_nguyen's topic in PHP Coding Help
its a typo replace foreach($var as $item) with foreach($vars as $item) its an example of how to avoid redundant code. for example if we expand the function to: function processpost($vars=array()) { foreach($vars as $item) $_GLOBAL[$item]=isset($_POST[$item])?mysql_real_escape_string(stripslashes(trim($_POST[$item]))):''; } processpost(array('myusername','mypassword')); if (empty($myusername) || empty($mypassword)) { header("location:http://www.mysite.com/login.html"); die(); } You can do away with these lines if (!isset($_POST['myusername']) || !isset($_POST['mypassword'])) { header("location:http://www.mysite.com/login.html"); } //check that the form fields are not empty, and redirect back to the login page if they are elseif (empty($_POST['myusername']) || empty($_POST['mypassword'])) { header( "location:http://www.mysite.com/login.html" ); // Define $myusername and $mypassword $myusername=$_POST['myusername']; $mypassword=$_POST['mypassword']; // To protect MySQL injection (more detail about MySQL injection) $myusername = stripslashes($myusername); $mypassword = stripslashes($mypassword); $myusername = mysql_real_escape_string($myusername); $mypassword = mysql_real_escape_string($mypassword); Its basicly the same code, but more generic to handle a range of $_POST variables and assign them into a global variable. The odd thing you will notice is the isset($_POST[$item])? the question mark, designates this as a trenary operator. which is basicly an if statement if(isset($_POST[$item])) $_GLOBAL[$item]=mysql_real_escape_string(stripslashes(trim($_POST[$item]))); else $_GLOBAL[$item]=''; so if $_POST is set, it does all the extra functions, otherwise, it just makes an empty string. -
Please help me revise this log in code.
laffin replied to shinichi_nguyen's topic in PHP Coding Help
Nope, he got redirected, because he didnt use start_session in the second script. the first script, if correctly logged in, would send him to the second script, which in turn sent him back to the first script, because the session variables werent available, so failed the first comparison. And it should be OR, which is either or both are not set or empty. would be silly to use AND, if you gave it a password with no username, or vice versa. -
strtotime takes a date format string, and converts it to time format. if you already have the time, than there is no need to convert. those strings should be just numbers (int) if(time() > 1262930400 && time() < 1263427199){
-
Please help me revise this log in code.
laffin replied to shinichi_nguyen's topic in PHP Coding Help
No page outputs allowed on header redirects, created a processpost, to do away with repetitive code. <?php function processpost($vars=array()) { foreach($var as $item) $_GLOBAL[$item]=isset($_POST[$item])?trim($_POST[$item]):''; } // process our form fields, and make them as variables // Define $myusername and $mypassword processposts(array('myusername','mypassword')); //check that the form fields are not empty, and redirect back to the login page if they are if(empty($myusername) || empty($mypassword)) { header( "location:http://www.mysite.com/login.html" ); die(); } $host="localhost"; // Host name $username="mod"; // Mysql username $password="modpass"; // Mysql password $db_name="mydbname"; // Database name $tbl_name="users"; // Table name // Connect to server and select databse. mysql_connect("$host", "$username", "$password") or die("cannot connect"); mysql_select_db("$db_name") or die("cannot select DB"); // To protect MySQL injection (more detail about MySQL injection) $myusername = stripslashes($myusername); $mypassword = stripslashes($mypassword); $myusername = mysql_real_escape_string($myusername); $mypassword = mysql_real_escape_string($mypassword); $sql="SELECT * FROM $tbl_name WHERE username='$myusername' and password='$mypassword'"; $result=mysql_query($sql); // Mysql_num_row is counting table row $count=mysql_num_rows($result); // If result matched $myusername and $mypassword, table row must be 1 row if($count == 1){ $row = mysql_fetch_array($result); //start the session and register a variable session_start(); $_SESSION['mysession']="mysession"; //successful login code will go here... //we will redirect the user to another page where we will make sure they're logged in header( "location:http://www.mysite.com/administrative.php" ); // Cant user header location with page output // echo 'Success!'; } else { //if nothing is returned by the query, unsuccessful login code goes here... header( "location:http://www.mysite.com/login.html" ); // Cant user header location with page output // echo 'Incorrect login name or password. Please try again.'; } ?> Avoid using php short tags, u must start a session, in order to use session variables <?php session_start(); if($_SESSION["mysession"]<>"mysession"){ header("location:http://www.mysite.com/login.html"); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Administrative page</title> </head> <body> <h2>Log in successful!!</h2> </body> </html> -
With a bit more sql u can do it very simply <?php function get_prev_and_last_items($id){ $arr['prev'] = 0; $arr['next'] = 0; $id = (int) $id; if($id == 0) return $arr; $cond = ""; if(isset($_GET['category_id'])){ $cat = (int) $_GET['category_id']; if($cat != 0){ $cond = " AND category_id = '$cat' "; } } $select = $this->db->query("SELECT item_id AS prev,(SELECT id from item WHERE id>{$id}{$cond} ORDER BY item_id ASC LIMIT 1) AS next FROM items WHERE item_id<{$id}{$cond} ORDER BY id DESC LIMIT 1"); return mysql_fetch_assoc($select); )
-
Well long as it works.
-
These are your variables $passName = "pass"; $passPhrase = "password"; $expName = "exp"; $authName = "auth"; $domain = "http://test.com"; $image = "/image.jpg"; $expTime = time() + (600); So question is which ones stay the same, and which ones change. Once you know which ones are the same, and which ones change, its easier to create a function. I reedited the function, so it generates the md5 as well function imageSource($image) { global $domain, $expName, $expTime, $authName, $sig; $sigUrl; $sigUrl = $image . "?" . $expName . "=" . $expTime . "&" . $passName . "=" . $passPhrase; $sig = MD5($sigUrl); $imageSrc = $domain . $image . "?" . $expName . "=" . $expTime . "&" . $authName . "=" . $sig; return $imageSrc; }
-
so does this $output_url = "{$domain}{$image}?{$expName}={$expTime}&{$authName }={$sig}"; if you have a lot of strings, using the string replacement with double quotes looks a lot cleaner
-
you normally cant Echo an array, this requires special handling. if u try to echo an array, all you get is 'Array'. So I think you need a finer description of what you are trying to do. if this is for an sql query, which I think it is given the name of the variable. sql_query("INSERT INTO table (tp1,tp2...) VALUES (". implode(',',$SQLQuery) .")"; the other code seems find for finding max consecutive sequences
-
this should not interfere with links with http/https/ftp Its kinda hard to break down the regex pattern strings, its something you learn with time and testing. but here is a good tutorial
-
My bad, i just wrote it without thinking bout it, or testing it $body = preg_replace_all("/(<a.*?)href\s*=\s*(['\"])(\/.*?)['\"](.*?>)/is", '$1href=$2..$3$2$4', $body); if all your links dont use domain names, just the filename as "index.html" [code]$body = preg_replace_all("/(<a.*?)href\s*=\s*(['\"])(.*?)['\"](.*?>)/is", '$1href=$2../$3$2$4', $body); shud do the trick
-
preg_replace_all('/(<a.*?)href\s*=\s*(['"])(\/.*?)['"](.*?>)/is','$1href=$2..$3$2$4',$body); only does links without domain names first character in url must be /
-
There shouldnt be an issue with cron. It runs at specified intervals. but like I said, since this is going to be an ongoing process. You may as well run it as a background task instead (continually running). if your updates takes awhile (depends on how many tasks it has to update) and you find that its taking more than a minute, you may encounter problems with overlapped background processes. The same issue as stated before. with an LRP solution, you wouldnt have that issue. but you would find that optimizing the code to minimize queries is going to be the hard part.
-
Using Ajax to me in this situation is silly. Because You need a user to update the db (No Ajax, nothing gets built) but your user scheduler will also need to update all users, not the ones just logged in or the current user logged in. so shure your getting that 1 sec update, however this script is gonna be hammered. in order to update all tasks completed, and return the result to the ajax script. In short, I recommend using a LRP (Long Running Process).
-
Reason I said: If the Scheuleder handles all tasks, than users dont matter. as you only want 1 scheduler running. All You have to do is check if your Scheuleder is running, if not, start it up. And it wont use up all resources.
-
to me the problem code is here while(list(,$vi) = each($MyImages)) why use while & list? replace with foreach($MyImages as $vi)
-
What's the best way to make a multi variable search
laffin replied to aeroswat's topic in PHP Coding Help
go with option 1). but use an array as form variables <form action="form_action.asp" method="get"> First name: <input type="text" name="form[fname]" /><br /> Last name: <input type="text" name="form[lname]" /><br /> <input type="submit" value="Submit" /> </form> on your processing script create a loop to process form array, then create the sql. foreach($_GET['form'] as $key=>$val) { if(strlen(trim($val)) { $form[]="{$key}='". mysql_real_escape_string(trim($val)) .'\''; } } if(!count($form)) $where=''; else $where=' WHERE '. implode(' AND ',$form); I think that should do it as you said, store the sql in a session variable (it would be bad if users were able to edit the sql statement) as well as the offset. than ya can query the records as you go along Edit: After rereading your post, the 2nd method is better. using serialize,unserialize to store the query result set from the database, so all records ids are in an array, into a session variable or cookie (if using cookies, remember to check the ids, or use intval on them). it should provide a performance boost, as well. since sql just has to locate the record id, instead of a series of WHERE clauses.