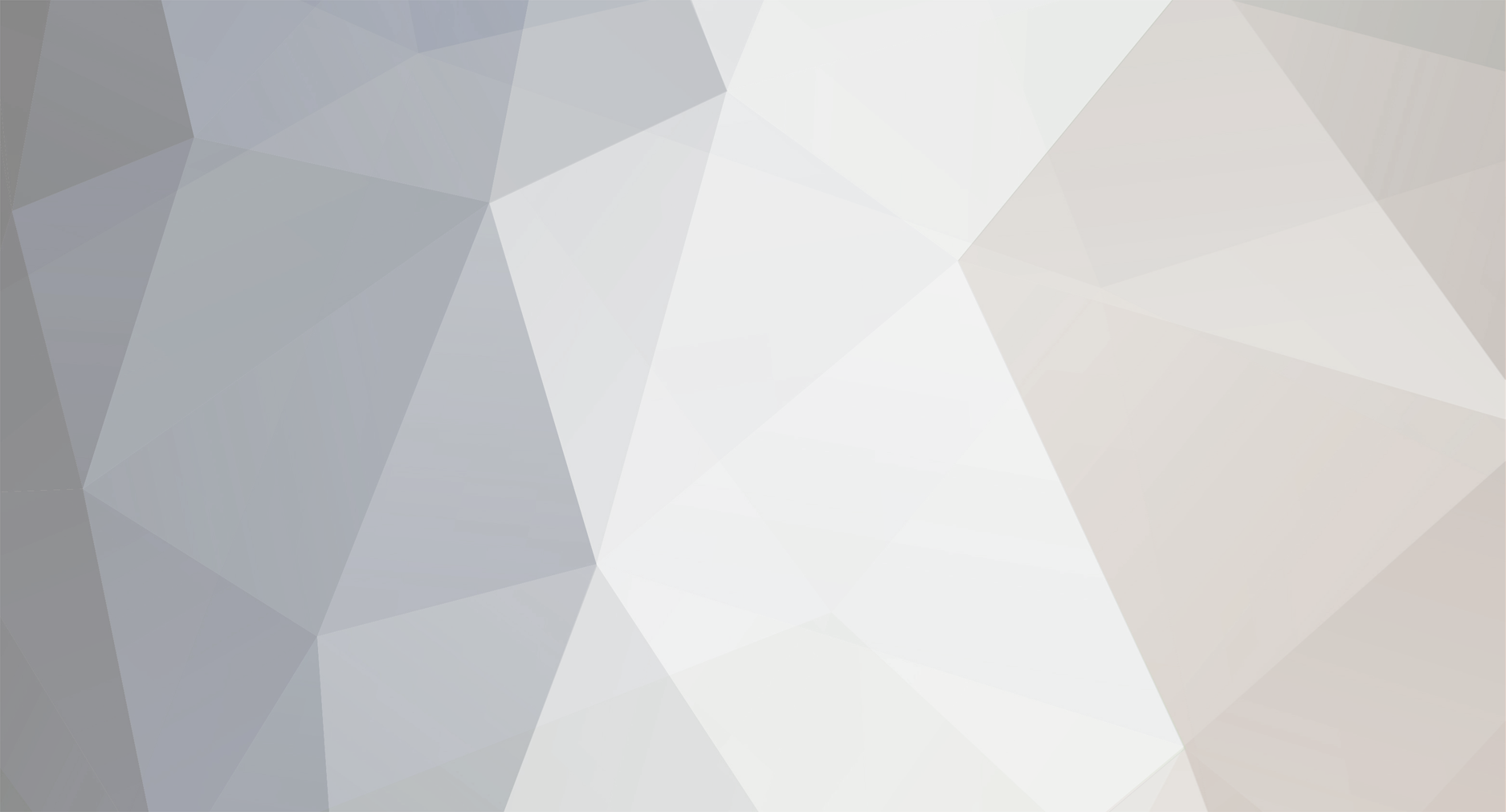
GameYin
Members-
Posts
389 -
Joined
-
Last visited
Everything posted by GameYin
-
Why does any of that matter? I'm looking for an answer to my question. If you cannot provide any help, I ask that you please stop cluttering my thread. Please and thank you. This feature needs to go live ASAP, thus to me, it is urgent. Obviously this is in testing so that's why the HTML code you see is for testing purposes. I cannot post the actual HTML file for security reasons. None of which should mean anything to you or that you should care.
-
What are you talking about? This will IMMEDIATELY go into the live application. Surely you are just pretending to be stupid, right?
-
I have a form where users enter a URL and it will need to pull data from the page (all information is within a div that has class="page-main-content"). I need to select the first occurance of an H1 element, along with a handful of other HTML elements. Can anyone help? I have this code as my test.php page. Then in the URL bar for the form, I enter in test2.php test.php <?php if(true) { if(!isset($_POST['submit'])) { ?> <form action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]); ?>" method="post"> <label for="url">Enter the URL of the article:</label> <input id="url" name="URL" type="text" /> <label for="submit"><input id="submit" class="button" name="submit" type="submit" /></form> <?php } else if(filter_var($_POST['URL'], FILTER_VALIDATE_URL) === false) { ?> <div class="error"><p>Error: The URL you entered was invalid. Please try again</p></div> <form action="<?php echo htmlspecialchars($_SERVER["PHP_SELF"]); ?>" method="post"> <label for="url">Enter the URL of the article:</label> <input id="url" name="URL" type="text" /> <label for="submit"><input id="submit" class="button" name="submit" type="submit" /></form> <?php } else { $url=$_POST['URL']; $doc = new DOMDocument; $doc->preserveWhiteSpace = FALSE; $doc->loadHTMLFile($url); $emailContents=array(); $xpath=new DomXPath($doc); $h1Found=false; //Find element with class="page-main-content" $results=$xpath->query("//*[contains(@class, 'page-main-content')]"); if (!is_null($results)) { foreach ($results as $element) { $nodes = $element->childNodes; foreach ($nodes as $node) { if(trim($node->textContent, " \n\r\t\0\xC2\xA0")!=='' && $node->nodeName==='h1' && !$h1Found) { echo "THIS IS FINDING THE H1-END".$node->textContent."<br>"; $h1Found=true; } elseif(trim($node->textContent, " \n\r\t\0\xC2\xA0")!=='') { echo $node->textContent. "<br>"; } } } } } } ?> Please ignore the stupid stuff like if(true) because I removed the condition for security reasons. test2.php <html> <head> <title>My Page</title> </head> <body> <div class="page-main-content"> <h1>h1 test</h1> <h1>h1 test</h1> <p><a href="mypage1.html">Hello World!</a></p> <p><a href="mypage2.html">Another Hello World!</a></p> </div> <p>THIS SHOULD NOT BE OUTPUTTED</p> </body> </html> Again, ignore the poor HTML, this is purely for testing purposes. Please help.
-
Holy crap a lot of replies... I figured out it was going for the literal string. I ended up doing something like this. default.php part <title>SiteDevs • <? echo $pageTitle; ?></title> index.php part $pageTitle="Home"; include 'default.php';
-
Hah damn been a long time since I've been here.. I have this page http://www.devwebsites.com/sitedevs/ I get an error when trying to do this include "default.php?pageTitle=Home"; default.php includes the dtd, all the same crap that begins every page. I want a custom <title> though for each page and that is where pageTitle comes in. If I went to index.php where I have include "default.php?pageTitle=Home";, the <title> will read: SiteDevs * Home. Get it? Anyway it's giving me an error on include "default.php?pageTitle=Home"; My default.php works just fine because if I go there and manually do it it shows fine.
-
http://gameyin.com/ajaxchat/ GOT IT WORKING.........YESSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSSS. Took me a total of 7 hours of straight work, rewriting the code until I got it. F*** YEA.
-
I've been coding since 13 but I'm more of an HTML/CSS person. Didn't answer my question. Do I combine the 2 functions?
-
In my setName function, I wasn't calling the form...I got part of it owrking. I can get a name to display, but you can't get a message in. Like, if you fill out both fields, ...hard to explain, go there and mess with it. The database is only adding a name OR text depending on which submit button you do. Should I combine functions??
-
How would I set a JavaScript cookie, by looking on submit.php's query string, and if username=so and so exists, create a cookie, and then reference it...HOW DO I DO THAT GAHH.
-
You seem to think I'm pro at this stuff xD. I'm only 16...I know a little bit of PHP but AJAX is my worst part.
-
Ok Now I need help with JavaScript. I want users to set their own username. gameyin.com/ajaxchat/ type in the 2nd text field waht you want your username to be function setName() { var username = document.set.Name.value; username=document.cookie = "name="+ username + ";"; http[username]=createRequestObject(); http[username].open(\'get\', \'submit.php?username=\'+ username); http[username].send(null); } </form> <form name="setMyName" id="set" method="get"> <input type="text" id="Name" /> <input type="submit" onclick="setName()" /> </form>
-
I believed my problem was not with JavaScript/Ajax so I posted this in the PHP help. As it turns out I was right. I'm now trying to make it so you can set your own username. Will post back soon Also I tend to get ancy when I can't figure something out.
-
Ugh yes I do. Sorry for wrong thread but I believe the AJAX part isn't wrong. No errors are given. If you would please go to the site (not trying to be rude) and type something in, you will see nothing happens. The data isn't going to the table and thus nothing shows up. (My theory). (Well, I KNOW it isn't going to the table (SELECT * FROM chat)).
-
Ugh sorry I broke rule # 16, but it's a really long post!! Sorry in advance.
-
http://forums.devshed.com/php-development-5/ajax-chat-555198.html Sorry I don't wanna repost everything as it is a long post. Anyone have reasons? gameyin.com/ajaxchat/
-
Save it as .css. Reference it with the link tag. Google it if you are stupid and don't know lol. Put every thing CSS related in that .css file except the <style></style> tags. You then SAVE your page as .html.
-
Hmm, he could set the background image via javascript, save the file location of the image in a cookie, and reference each time he comes back. Although that's just all theory...
-
line-height:;
-
This goes out to Casper. The best dog a man could ask for. May you rest in peace little buddy. You will always be remembereed in my heart. May God look after you in the next life. 5/26/08
-
It's how my forum works. www.devhunters.com Not MY forum, but I'm SpOrTsDuDe.Reese on there. That's how we operate.
-
But you said only you appoint them. No sucking up allowed. Maybe he is already thinking about being one but you just haven't promoted him?
-
No, LOOK, at my title. See what's there? I'm wondering which mod did it.
-
LOL. Who gave me the interesting title LOL!
-
I'm already a moderator on another forum. Too much work for more forums.
-
[code] tags not working in IE6
GameYin replied to GingerRobot's topic in PHPFreaks.com Website Feedback
I'm 16, 11th grade ;o.