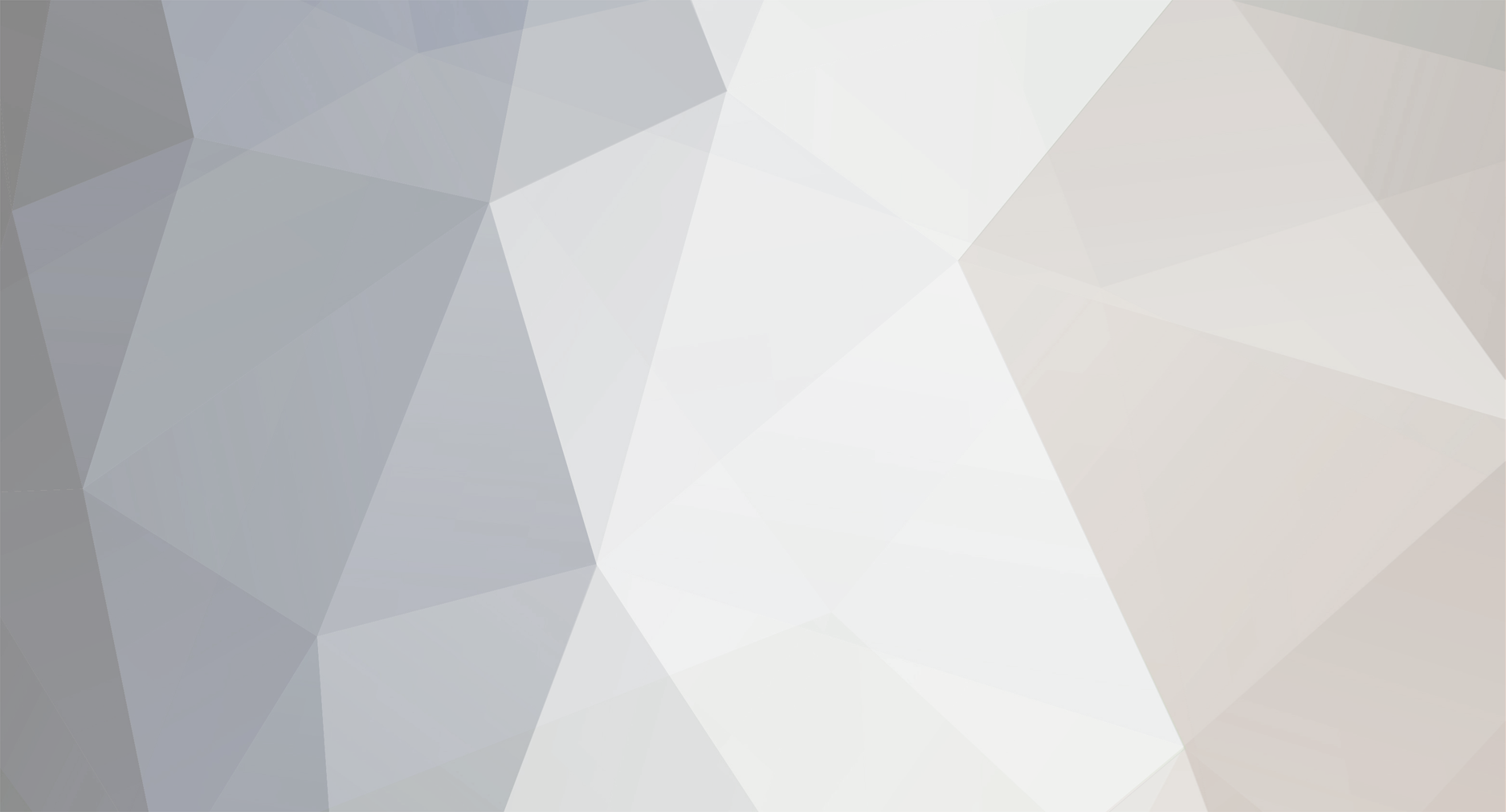
drisate
Members-
Posts
805 -
Joined
-
Last visited
Profile Information
-
Gender
Not Telling
drisate's Achievements

Advanced Member (4/5)
1
Reputation
-
Select a col without knowing the table it's contained in
drisate replied to drisate's topic in MySQL Help
It's actually complicated ... we own the website but not the DB and that makes it hard for us to develop any new features. They want us to hire them ... Just trying to show my boss we can manage with out them lol -
Hey guys I am testing a query that returns: function is called Allowed memory size of 134217728 bytes exhausted (tried to allocate 2097160 bytes) now i know i can change the memory limit ... but I would prefer optimizing the query instead. select * from devices where hardware_id=(select hardware_id from (SELECT TABLE_NAME FROM INFORMATION_SCHEMA.COLUMNS WHERE COLUMN_NAME ='hardware_id' order by TABLE_NAME asc limit 1) as T where hardware_id!='' order by id asc limit 1) Is there a better way of searching for hardware_id in the first found table that contains them? I tryed adding the limit 1 param in both sub query's ... but that did not change the allowed memory error. I wish i had the database table structure but I am flying blind ... Thx in adavnce
-
Hey guys, i have an XML file that looks like this: <?xml version="1.0" encoding="UTF-8"?> <data> <debug>1</debug> <db> <host>localhost</host> <user>***</user> <pass>***</pass> <name>***</name> </db> <config> <path_to_site>***:81</path_to_site> <path_to_movies>media</path_to_movies> <full_path_to_movies>/var/www/media/movies/</full_path_to_movies> <full_path_to_shows>/var/www/media/shows/</full_path_to_shows> <tmdb_api_key>***</tmdb_api_key> <lang_api_key>***</lang_api_key> <tvdb_api_key>***</tvdb_api_key> <allowed_movie_ext> <ext>mp4</ext> <ext>m4v</ext> </allowed_movie_ext> <delete_skiped>1</delete_skiped> <system>linux</system> <lastmovies>25</lastmovies> <language>en</language> <country>US</country> <debug>0</debug> <junk> <word>1080p</word> <word>BluRay</word> <word>x264</word> <word>YIFY</word> <word>YIFY</word> <word>BrRip</word> <word>BOKUTOX</word> <word>YIF</word> <word>bitloks</word> <word>deceit</word> <word>BrRip</word> <word> GAZ</word> <word>GAZ</word> <word>UNRATED</word> <word>ECE</word> <word>mkv</word> <word>DVDSCR</word> <word>XViD</word> <word>FRENCH</word> <word>TS</word> <word>MD</word> <word>SKuLL322</word> <word>DTS</word> <word>Multi</word> <word>bluray</word> <word>net</word> <word>torrentfrancais</word> <word>truefrench</word> <word>5.1</word> <word>\xb7</word> </junk> <user_lang> <lang>en</lang> <lang>fr</lang> <lang>es</lang> </user_lang> </config> <hdd> <path> <torrent_path>/var/Simflix/scripts/addons/torrent/</torrent_path> <temp_path>/var/Simflix/scripts/addons/temp/</temp_path> <save_path>/mnt/My Book 4/media/series temp/</save_path> </path> <path> <torrent_path>/var/Simflix/scripts/addons/rss_download/torrents/</torrent_path> <temp_path>/var/Simflix/scripts/addons/temp/</temp_path> <save_path>/mnt/My Book 4/media/movies temp/</save_path> </path> </hdd> </data> I use that XML data in my website like this: $config = simplexml_load_file('/var/Simflix/www/config.xml'); It working great but now i need to create a code that would edit that XML file ... So fare i manager to do this: function print_input($name, $value){ print (' <div class="formRow"> <label>'.ucfirst($name).': </label> <div class="formRight"> <input id="name" name="'.$name.'" value="'.$value.'" class="span input" type="text"/> </div> </div> '); } function print_input_array($name, $value, $title){ $rand = rand("999999999", "999999999999"); print (' <div class="formRow"> <label><span class="addmore" name="'.$name.'" rel="g'.$rand.'" style="cursor:pointer">(ADD)</span> '.ucfirst($title).': </label> <div id="g'.$rand.'" class="formRight"> <input id="name" name="'.$name.'[]" value="'.$value.'" class="span input" type="text"/> </div> </div> '); } $config_array = get_object_vars($config); foreach($config_array[db] as $name=>$value){ print_input($name, $value); } foreach($config_array[config] as $name=>$value){ if (is_array($value)){ foreach($value as $name1=>$value1){ print_input_array($name1, $value1, $name); } }else{ print_input($name, $value); } } But for some reason, the print_input_array() is not triggured when i get to the allowed_movie_ext, junk, user_lang [...] Is there a better way of making an HTML form from a XML object?
-
Normaly "imagepng($dst,$dest_file);" replaces that and avoids uploading user content to the server in case the file is infected
-
I need to upload 3 images and resize them as part of a forme but for some reason, the images does not end up in the server directory. What am i missing? The fille array looks like this: Array ( [file1] => Array ( [name] => s_casafolio.png [type] => image/png [tmp_name] => /tmp/phpENy7j4 [error] => 0 [size] => 2047496 ) [file2] => Array ( [name] => lawjournal.png [type] => image/png [tmp_name] => /tmp/phpT0fu1Y [error] => 0 [size] => 1139448 ) [file3] => Array ( [name] => s_mdj_2.png [type] => image/png [tmp_name] => /tmp/phpUsRk6W [error] => 0 [size] => 792873 ) ) And my processing looks like this: <?php $tmp = md5(md5(date("YmdHis"))); $img_quality = 70; $maxDim = 700; $dest_folder = "/home/user/public_html/userfiles/inscription_designer/"; $valid_mime_types_images = array( "image/gif", "image/png", "image/jpeg", "image/pjpeg", ); if (in_array($_FILES["file1"]["type"], $valid_mime_types_images)) { $ext = pathinfo($_FILES['file1']['name'], PATHINFO_EXTENSION); $dest_file = $dest_folder.$tmp.'1.'.$ext; $fn = $_FILES['file1']['tmp_name']; $size = getimagesize($fn); $ratio = $size[0]/$size[1]; // width/height if( $ratio > 1) { $width = $maxDim; $height = $maxDim/$ratio; } else { $width = $maxDim*$ratio; $height = $maxDim; } $src = imagecreatefromstring(file_get_contents($fn)); $dst = imagecreatetruecolor($width,$height); imagecopyresampled($dst,$src,0,0,0,0,$width,$height,$size[0],$size[1]); imagedestroy($src); imagepng($dst,$dest_file); // adjust format as needed imagedestroy($dst); }else{ $err .= "- Image 1 est invalide.<br />"; } if (in_array($_FILES["file2"]["type"], $valid_mime_types_images)) { $ext = pathinfo($_FILES['file2']['name'], PATHINFO_EXTENSION); $dest_file = $dest_folder.$tmp.'2.'.$ext; $fn = $_FILES['file2']['tmp_name']; $size = getimagesize($fn); $ratio = $size[0]/$size[1]; // width/height if( $ratio > 1) { $width = $maxDim; $height = $maxDim/$ratio; } else { $width = $maxDim*$ratio; $height = $maxDim; } $src = imagecreatefromstring(file_get_contents($fn)); $dst = imagecreatetruecolor($width,$height); imagecopyresampled($dst,$src,0,0,0,0,$width,$height,$size[0],$size[1]); imagedestroy($src); imagepng($dst,$dest_file); // adjust format as needed imagedestroy($dst); }else{ $err .= "- Image 2 est invalide.<br />"; } if (in_array($_FILES["file3"]["type"], $valid_mime_types_images)) { $ext = pathinfo($_FILES['file3']['name'], PATHINFO_EXTENSION); $dest_file = $dest_folder.$tmp.'3.'.$ext; $fn = $_FILES['file3']['tmp_name']; $size = getimagesize($fn); $ratio = $size[0]/$size[1]; // width/height if( $ratio > 1) { $width = $maxDim; $height = $maxDim/$ratio; } else { $width = $maxDim*$ratio; $height = $maxDim; } $src = imagecreatefromstring(file_get_contents($fn)); $dst = imagecreatetruecolor($width,$height); imagecopyresampled($dst,$src,0,0,0,0,$width,$height,$size[0],$size[1]); imagedestroy($src); imagepng($dst,$dest_file); // adjust format as needed imagedestroy($dst); }else{ $err .= "- Image 3 est invalide.<br />"; } ?>
-
Ok well i am gething closser ... I decided to use the xpath querry to point at the correct table in the page. It will be alot easyer to pin-point the data this way. The dump looks like: http://eric.webiummedia.com/scrap.php Now what i need is from the $elements loop all the TD's and retrive the HTML inside
-
Well as you can imagine, the dump of the $tr var is very big ... would be a lot easyer if you would just execute the provided code.
-
added data in todo list is not visible without refreshing
drisate replied to surveen's topic in PHP Coding Help
You can do that from your script.js page. Inside the sucess part of the script, you can add something like: $("#todoList").load( "index.php #todoList"); In order for this to work, add an id attribute to the <ul class="todoList"> like this <ul class="todoList" id="todoList"> Then in the code i provided insert the URL of your totdo page at the place of index.php This will fetch the page, retreive the #todoList content and replace it and all that with out refreshing the users page ... only the content will change. -
Hey guys i need help with a CURL code. I need to retreive all the data in a table located at http://www.listenlive.nl/tvgenre.php?g=1&page=0 and parse the data to finish with an array that looks like this: $row[0][station_name] = '3BTV'; $row[0][stream_link] = '420'; $row[0][stream_type] = 'wma'; $row[0][country] = 'United Kingdom'; $row[0][genre][0] = 'Variety'; $row[0][genre][1] = 'Alternative'; $row[0][genre][2] = 'Culture'; $row[0][rating] = '1/5'; I need that for each rows of the table where the first array number would increment $row[1], $row[2] ... My PHP code is incomplete. I currently have a loop for all the TR's of the page ... and when i loop all the TD's inside the TR's it returns a load of crap ... I am unsure where to go from here. Any help would be apreciated thx This is my code: $ch = curl_init(); $categ[url] = 'http://www.listenlive.nl/tvgenre.php?g=1&page=0'; curl_setopt($ch, CURLOPT_URL, $categ[url]); curl_setopt($ch, CURLOPT_POST, false); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_COOKIEFILE, $cookies); curl_setopt($ch, CURLOPT_COOKIEJAR, $cookies); curl_setopt($ch, CURLOPT_COOKIE, $cookies); curl_setopt($ch, CURLOPT_REFERER, $categ[url]); curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true); curl_setopt($ch, CURLOPT_USERAGENT, $nav); $data2 = curl_exec($ch); $dom2 = new DOMDocument(); libxml_use_internal_errors(true); $dom2->loadHTML($data2); $trs = $dom2->getElementsByTagName('tr'); foreach ($trs as $tr) { echo "<pre>"; print_r($tr); echo "</pre>"; }
-
Hey guys! I am using a script i found here (http://vikku.info/programming/geodata/geonames-get-country-state-city-hierarchy.htm) It works really nice for what I need to do but I have only one problem. When the client enters data but forget something, the form returns an error and the POST values are inserted in each inputs so they don't need to start the whole form. My problem is I can't seem to find a way to auto select the pre-entered data ... I made a pastie of my code here http://pastie.org/8743532 So fare, I tried adding the selected option id inside the select tag like this: <option value="<?=$_POST[continent]?>"></option> But that did not work ... I also tried playing with the window.onload part by adding more getplaces() like this: window.onload = function() { <?php if ($_POST[continent]){ echo 'getplaces('.$_POST[continent].',\'country\');'."\n"; }else{ echo 'getplaces(6295630,\'continent\');'."\n"; } if ($_POST[country]){echo 'getplaces('.$_POST[country].',\'country\');'."\n";} if ($_POST[province]){echo 'getplaces('.$_POST[province].',\'province\');'."\n";} if ($_POST[region]){echo 'getplaces('.$_POST[region].',\'region\');'."\n";} if ($_POST[city]){echo 'getplaces('.$_POST[city].',\'city\');'."\n";} ?> } But that did not work ... I also tried playing with the listPlaces() function like this: for(var i=0;i<counts;i++){ who.options[who.options.length] = new Option(jData.geonames[i].name,jData.geonames[i].geonameId); if (jData.geonames[i].geonameId==whos){ who.options[who.options.length].selected = true; } } But you guessed it right ... it did not work ... I am out of options. I would hate having to change the script ... Any help would be apreciated!
-
Ok i finaly got this to work and it's VERY VERY VERY fast compare to what i was doing previously. // Create the page array // FROM: http://www.tommylacroix.com/2008/09/10/php-design-pattern-building-a-tree/ // Thx ! function mapTree($dataset) { $tree = array(); foreach ($dataset as $id=>&$node) { if ($node['parent'] === null) { $tree[$id] = &$node; } else { if (!isset($dataset[$node['parent']]['children'])) $dataset[$node['parent']]['children'] = array(); $dataset[$node['parent']]['children'][$id] = &$node; } } return $tree; } // Start the page tree view function page_treeview(){ // Retreive all pages of the DB and formating it for mapTree() $pages = mysql_query("SELECT id, parent, titre FROM pages"); $t=0; while($page = mysql_fetch_array($pages)){ $tree[$page[id]][name] = $page[titre]; $tree[$page[id]][parent] = $page[parent]; $tree[$page[id]][id] = $page[id]; $t++; } // Creating the recursive page array $pages = mapTree($tree,"0"); // Looping the first level if($pages[0][children]){ echo '<ul class="menu-tree accordion dark strips">'; foreach($pages[0][children] as $page){ if ($page[parent]=='0'){ echo '<li>'.$page[name]; if ($page[children]){ page_enfant($page[children]); } echo'</li>'; } } echo '</ul>'; } } // Recursive function function page_enfant($sub_page){ echo "\n".'<ul>'; foreach ($sub_page as $child){ echo "\n".'<li>'.$child[name]; if ($child[children]){ page_enfant($child[children]); } echo'</li>'."\n"; } echo '</ul>'."\n"; } // How to use?? echo page_treeview(); This code will generate a <ul><li> infinite tree structure in only one SQL line I hope it helps
-
I can't get your exemple to work ... function mapTree($dataset) { $tree = array(); foreach ($dataset as $id=>&$node) { if ($node['parent'] === null) { $tree[$id] = &$node; } else { if (!isset($dataset[$node['parent']]['children'])) $dataset[$node['parent']]['children'] = array(); $dataset[$node['parent']]['children'][$id] = &$node; } } return $tree; } // Liste des pages function page_treeview(){GlOBAL $_SESSION; $pages = mysql_query("SELECT id, parent, titre FROM pages"); $t=0; while($page = mysql_fetch_array($pages)){ $tree[$page[id]][name] = $page[titre]; $tree[$page[id]][parent] = $page[parent]; $tree[$page[id]][id] = $page[id]; $t++; } $pages = mapTree($tree,"0"); if($pages[0][children]){ echo '<form method="POST" id="ajaxform" class="load_form"> <ul class="menu-tree accordion dark strips">'; foreach($pages[0][children] as $page){ if ($page[parent]=='0'){ echo '<li> <input type="checkbox" id="category-'.$page[id].'" /> <label for="category-'.$page[id].'"> <i onclick="location.href=\'index.php?mod=page&id='.$page[id].'&pid='.$page[parent].'\'" class="icon-edit-sign"></i> <i onclick="location.href=\'index.php?mod=page&del='.$page[id].'\'" class="icon-remove-sign"></i> <i onclick="location.href=\'index.php?mod=page&id='.$page[id].'&pid='.$page[parent].'&action=add\'" class="icon-plus-sign-alt"></i> '.$page[name].' <span class="notification"></span> </label>'; page_enfant($page[id], $pages); echo'</li>'; } } echo '</ul><br><input type="submit" value="Sauvegarder l\'ordre" name="saveordre"></form>'; } } function page_enfant($id, $page){ echo "\n".'<ul>'; foreach ($page['children'] as $child){ echo "\n".'<li> <input type="checkbox" id="category-'.$child[id].'" /> <label for="category-'.$child[id].'"> <i class="icon-edit-sign"></i> <i class="icon-remove-sign"></i> <i class="icon-plus-sign-alt"></i> '.$child[name].' <span class="notification"></span> </label>'; page_enfant($child); echo'</li>'."\n"; } echo '</ul>'."\n"; }
-
I saw that link already. If you take a look at my seconde code i posted, thats teh function i am trying to use. But i am failing in the recuresive function. Can you show me an exemple of your "b" point?
-
I posted my pages table here http://tinyurl.com/drisate. ( it's 110 Mo ... )