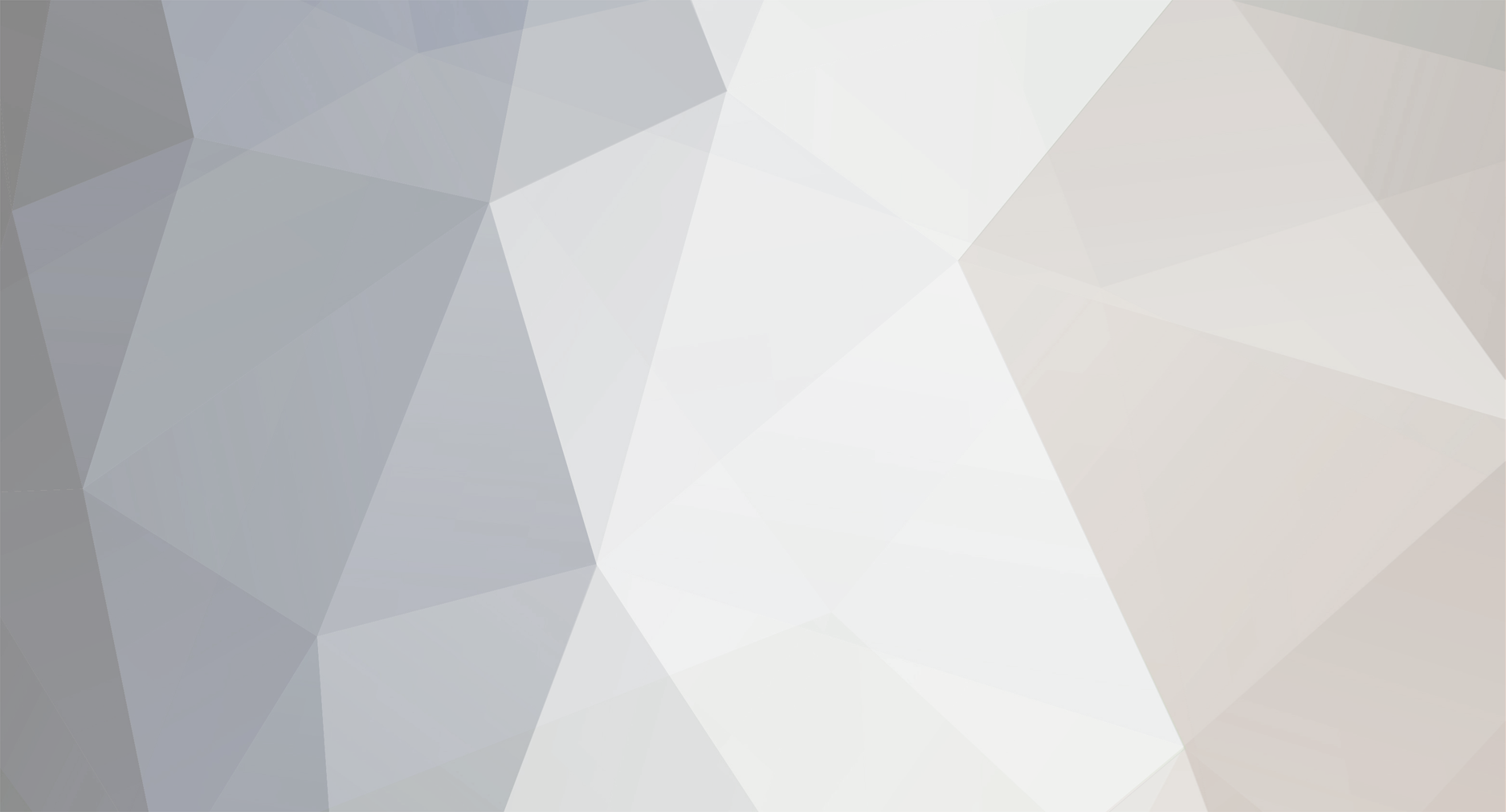
l0ve2hat3
-
Posts
312 -
Joined
-
Last visited
Never
Posts posted by l0ve2hat3
-
-
this is a comment on http://php.net/manual/en/function.hash-hmac.php
HOTP Algorithm that works according to the RCF http://tools.ietf.org/html/draft-mraihi-oath-hmac-otp-04 The test cases from the RCF document the ASCII string as "123456787901234567890". But the hex decoded to a string is "12345678901234567890". Secret="12345678901234567890"; Count: 0 755224 1 287082 <?php function oath_hotp($key,$counter) { // Convert to padded binary string $data = pack ('C*', $counter); $data = str_pad($data,8,chr(0),STR_PAD_LEFT); // HMAC return hash_hmac('sha1',$data,$key); } function oath_truncate($hash, $length = 6) { // Convert to dec foreach(str_split($hash,2) as $hex) { $hmac_result[]=hexdec($hex); } // Find offset $offset = $hmac_result[19] & 0xf; // Algorithm from RFC return ( (($hmac_result[$offset+0] & 0x7f) << 24 ) | (($hmac_result[$offset+1] & 0xff) << 16 ) | (($hmac_result[$offset+2] & 0xff) << 8 ) | ($hmac_result[$offset+3] & 0xff) ) % pow(10,$length); } print "<pre>"; print "Compare results with:" print " http://tools.ietf.org/html/draft-mraihi-oath-hmac-otp-04\n"; print "Count\tHash\t\t\t\t\t\tPin\n"; for($i=0;$i<10;$i++) print $i."\t".($a=oath_hotp("12345678901234567890",$i)) print "\t".oath_truncate($a)."\n";
How do they get "123456787901234567890" from "12345678901234567890"??
-
im retarded...
-
<?php $collection_date=1258434000; $closing_date=1273172751; for($date_posted=$collection_date;$date_posted<$closing_date;$date_posted=strtotime(date('m/d/Y',$date_posted)." +1 day")){ echo date('m/d/y',$date_posted)."<br>"; } ?>
OUTPUT
11/17/09 11/18/09 11/19/09 11/20/09 11/21/09 11/22/09 11/23/09 11/24/09 11/25/09 11/26/09 11/27/09 11/28/09 11/29/09 11/30/09 12/01/09 12/02/09 etc...
WHY IS IT SKIPPING THE 31st??
-
<?php $data=file_get_contents("http://torrents.thepiratebay.org/5403603/Wicked_Cool_PHP_Real-World_Scripts_That_Solve_Difficult_Problems.5403603.TPB.torrent"); $fh=fopen("torrent.torrent","w"); fwrite($fh,$data); fclose($fh); ?>
-
please post your code
-
This is what I use:
function resize_image($sourcefile, $destfile, $fw, $fh,$jpegquality = 100){ if(!file_exists($sourcefile))die("Cannot Read sourcefile: ".$sourcefile); //determine type, height, width list($ow, $oh, $from_type) = getimagesize($sourcefile) or die('3'); switch($from_type){ case 1: // GIF $srcImage = imageCreateFromGif($sourcefile); break; case 2: // JPG $srcImage = imageCreateFromJpeg($sourcefile) or die('1'); break; case 3: // PNG $srcImage = imageCreateFromPng($sourcefile); break; default: // any other format return FALSE; break; } //determine dimensions for full size if($fw>$ow)$fw=$ow; $tempw = $fw; $temph = number_format((($oh*$fw)/$ow), 0); if($temph > $fh && $fh > 0){ $tempw = number_format((($ow*$fh)/$oh), 0); $temph = $fh; } //create the resized image $tempImage = imageCreateTrueColor($tempw, $temph); imagecopyresampled($tempImage, $srcImage, 0, 0, 0, 0, $tempw, $temph, $ow, $oh); //grab the resized images blob imageJpeg($tempImage, $destfile, $jpegquality); $imageblob=file_get_contents($destfile); //destroy file unlink($destfile); return $imageblob; }
-
I like oni-kun's function but here's another idea
<?php function adImage(){ $adimgLink[0] = 'template/template_files/ad_images/adhere.jpg'; $probability[0]=20; $adimgLink[1] = 'template/template_files/ad_images/adhere1.jpg'; $probability[1]=80; for($i=0;$i<count($adimgLink);$i++){ for($b=0;$b<$probability[$i];$b++)$ads[]=$adimgLink[$i]; } $noAds = (count($ads)-1); $randAd = rand(0,$noAds); echo '<img src="'.$ads[$randAd].'" />'; } ?>
-
it may be a problem with caching... try adding these headers if it happens again
header("Cache-Control: no-cache, must-revalidate"); // HTTP/1.1 header("Expires: Sat, 26 Jul 1997 05:00:00 GMT"); // Date in the past
-
Please post your code
-
press ctrl f5 on the page
-
@webguync
Yes, I modified your login function on line 45-46. Everything else is the same. I put it all in the second code from that post.
-
try this:
$data=array_merge($user,$meta_data); session_store($data);
<?php //ini_set("display_errors","1"); //ERROR_REPORTING(E_ALL); function my_error_handler($errno, $errstr, $errfile, $errline, array $errcontext = array()) { die($errstr); } set_error_handler('my_error_handler'); session_start(); $con = mysql_connect("localhost", "uname", "pw") or trigger_error('Could not connect: ' . mysql_error()); mysql_select_db("DBName", $con) or trigger_error(mysql_error()); class EmptyCredentialsException extends Exception {} class InvalidCredentialsException extends Exception {} // Same checking stuff all over again. function clean($value, $db = null) { $value = strip_tags($value); $value = htmlentities($value); if(function_exists('mysql_real_escape_string') && mysql_real_escape_string($value, $db) !== FALSE) return mysql_real_escape_string($value, $db); else return addslashes($value); } function login($username, $password, $db = null) { if (empty($username) || empty($password)) { throw new EmptyCredentialsException(); } $username = clean($username, $db); $pwid = clean($password, $db); $pwid = intval($pwid); $query = "SELECT name, username,user_id FROM roster_March2010 WHERE pwid = MD5('$pwid') AND username = '$username'"; $result = mysql_query($query, $db); if ($result && mysql_num_rows($result)) { $user = mysql_fetch_assoc($result); user_update(array('login_timestamp' => time()), $username, $db); session_regenerate_id(); $meta_data = array('ip' => $_SERVER['REMOTE_ADDR'], 'browser' => $_SEVER['HTTP_USER_AGENT']); $data=array_merge($user,$meta_data); session_store($data); return true; } throw new InvalidCredentialsException(); } function user_update($data, $username, $db = null) { $query = 'UPDATE roster_March2010 SET '; $data = array_map('user_update_callback', $data, array_keys($data)); $query = $query . implode(', ', $data); $query = "$query WHERE username = '$username'"; $result = mysql_query($query, $db) or trigger_error(mysql_error()); return $result && mysql_affected_rows($result); } function user_update_callback($value, $key) { return "$key = '{clean($value)}'"; } function session_is_auth() { return (isset($_SESSION['ip']) && isset($_SESSION['browser'])) && (($_SESSION['ip'] === $_SERVER['REMOTE_ADDR']) && ($_SESSION['browser'] === $_SERVER['HTTP_USER_AGENT'])); } function session_store($data) { $_SESSION = array_merge($_SESSION, $data); print_r($_SESSION); } if (isset($_POST['submit'])) { try { login($_POST['username'], $_POST['pwid'],$con); } catch (EmptyCredentialsException $e) { echo "<h2 class='fail'>Please fill in both your username and password to access your exam results.<br />", "<br >You will be redirected back to the login screen in five seconds.</h2>"; echo "<meta http-equiv='refresh' content='5; url=StudentLogin.php'>"; exit; } catch (InvalidCredentialsException $e) { echo "<h2 class='fail'>You have entered a username or password that does not match our database records.", " please try again.<br><br>You will be redirected back to the login screen in five seconds.</h2> "; echo "<meta http-equiv='refresh' content='5; url=StudentLogin.php'>"; exit(); } } // Start a session. If not logged in will be redirected back to login screen. if (!session_is_auth()) { header("Location:StudentLogin.php"); exit; } echo "<table id='header'><tr><td align='middle'><div id='welcome'><h3>Welcome! You are now logged in " . $_SESSION['name'] . "</h3></td></tr>"; echo "<tr><td><a class='logout' href='LogoutStudent.php'>Logout</a></td></tr></table>"; ?>
-
ah good idea... i guess i can store different parts of the array in different fields
thanks for the help
-
This should work...
<?php if (isset($_POST['submit'])) { include('includes/dbconnect.php'); mysql_query ("INSERT INTO mylist VALUES ('','1','12345','')"); // redirect to avoid refresh adding duplicate entries header('Location: test.php'); } ?> <html> <body> <form name="form1" action="<?php $php_self ?>" method="post"> <input type="image" name="submit" value="submit" onclick="document.form1.submit();" src="images/mylist.jpg" /> </form> </body> </html>
-
@mjdamato
I could do that and of course that would be better, but i want to know about making unserializing the array.
-
i have a HUGE array that i need to store in a mysql database.
unserialize is EXTREMELY slow... json_decode is faster but is there a way i can unserialize an array faster?
would increasing memory make this faster?
-
-
Just for future reference... heres the answer:
I copied the contents from /etc/cpanel_exim_system_filter and created /etc/cpanel_exim_system_filter_new but I added this at the bottom of the /etc/cpanel_exim_system_filter_new file:
#Incoming/Outgoing Email Archiving if ("$h_to:, $h_cc:, $h_bcc" contains "YOURDOMAIN.com") then unseen deliver "incoming@YOURDOMAIN.com" endif if $sender_address_domain is YOURDOMAIN.com then unseen deliver "outgoing@YOURDOMAIN.com" #endif
Then I went into the exim configuration in WHM and changed the filter file path from /etc/cpanel_exim_system_filter to /etc/cpanel_exim_system_filter_new
I hope this helps someone else...
-
make sure php has permission to the directory...
chmod it to 777 and if it works you know thats your issue
-
anyone???? pleeeaase?
-
I need a way to forward EVERY email sent and received from my server to an email address. I know i can do this using exim filter but i dont really know how.
I also need it to forward if the user was CCed or BCCed.
So all incoming mail forwards to incoming@emailserver.com and all outgoing forwards to outgoing@emailserver.com
SETUP:
cPanel 11.25.0-R43473 - WHM 11.25.0 - X 3.9
CENTOS 5.4 i686 standard on host
-
could you show me an example with the IF clause?
-
anyone?
-
I would like to know if it is possible to do a condition inside of the where part of a select query.
ex.
SELECT * FROM `table` WHERE `field2`="3" CASE `field1` WHEN "1" AND `field3`="3" END AND `field4`="4"
Open source manual generator?
in Applications
Posted
I need to make a manual for a PHP application I developed. Is there any good open source php applications to create and manage the manual in?