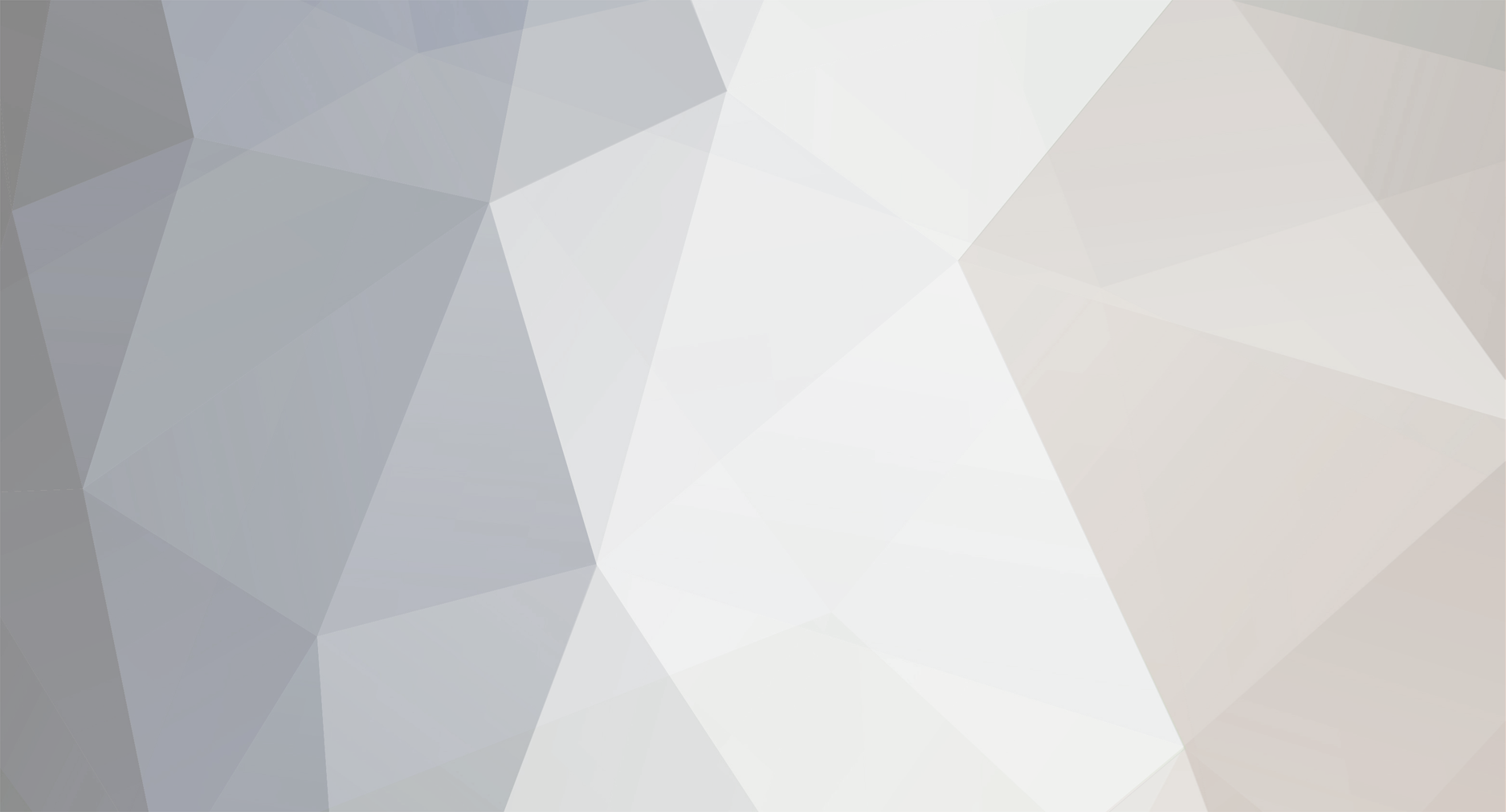
rockindano30
-
Posts
141 -
Joined
-
Last visited
Never
Posts posted by rockindano30
-
-
yes they were. thank you very much swissbeets
-
hey anybody knows of any good tool to test your websites before going live?
-
thanks hopelessX for the advice. its just ie6 that gives me trouble other than that i think its good. any more feedback from anyone?
-
well in ie 7, mozzila, safari, opera they all work well. i have not tested in ie6 or lower.
-
Hello everyone,
this is a site i did for some clients of mine and would like some feedback
http://secondtwononegraphics.com/
thank you
-
oh i get it. thank you thorpe
-
thats what i was looking for. so for every vhost i have a separate file and include that line in every file?
-
no, i purchased my server to run my site and other clients' sites.
-
i c thanks for the hand.
-
more of giving the view a option to view one or two videos.
-
oh so i dont have to add another dir. to the httpd.config file for apache. it all goes with whoever i registered my domain name?
-
no not like youtube. more of just embedded windows media player but able to play different videos or have a list below links to different videos.
-
hello all,
i am working on a project and my client wants to put videos on his site and a list of videos that viewers can choose to view. any idea on how to go about it or start it?
-
hello all,
well i have a webserver that i'm working on. now for all my websites i used apache/php/mysql. my question is how do i host three or multiple website in my server using apache as a server with php/mysql? no i have to set it up in the config file for apache if so how would i go about that?
-
well don't know what it was but changed my login.php file to a login.html file and seems to be working just fine. thank you craygo for all your help and advise.
-
oh i c. thank you
-
anybody know of a good tutorial for that? or guide me to a tut?
-
huh? what do you mean. sorry for being a nubbie
-
well craygo i did your steps and still same thing???
-
MiCR0
how could i fix my code for that?
-
thank you let me try that and see if it works.
-
Hi everyone,
i am working on a app. for a client. now i have my login.php page with a simple form. this page calls my validation2.php page and then validation2 page calls my update page.
now when i type in the user name and password, my validation page displays my error that i have:
"invalid username and password. login first"
but if i hit go back on my browser and refresh the page and retype the info, it logs me in. i typed my info in correctly. but seems that the first time that i run it it doesn't finds anything in the mysql table users, so when i go back and refresh and retype it finds it in the table and continues with the update form.??? ??? ???
i will be including my files here:
login.php
<form method="post" action="validate2.php"> User Name:<Br /> <input name="user_name" type="text" value=" " size="15" maxlength="25" /><br /><br /> Password:<br /> <input name="psswrd" type="password" value="" size="15" maxlength="25" /><br /><br /> <input name="submit" type="submit" value="Login" /> </form>
validate2.php
<?php if(!isset($_POST["user_name"]) || !isset($_POST["psswrd"])) die("invalid operation"); $goback = "<br /><br />Please <a href=\"login.php\">go back</a> and try again."; //print "$_POST[\"user_name\"]<br />"; if(empty($_POST["user_name"])) die("<br />The email field cannot be left blank."); if(empty($_POST["psswrd"])) die("<br />The Password field cannot be left blank"); $user_name = $_POST["user_name"]; $psswrd = md5(trim($_POST["psswrd"])); if (!($db = mysql_connect('localhost','user_db','db_password'))) { print"Error: could not connect to the database."; exit; } mysql_select_db(test); //$query = "SELECT * FROM users WHERE user_name='{$_POST['user_name']}' AND psswrd='{$_POST['psswrd']}'"; $query = "SELECT * FROM users WHERE user_name = '{$user_name}' AND psswrd = '{$psswrd}'"; $result = mysql_query($query); if(mysql_num_rows($result)==0) die("<br />Invalid email or passwordmmmmmmmmmmmmmmmmmmmmmmmmmmmmmmm!<br />{$goback}"); $row = @ mysql_fetch_array($result); session_start(); $_SESSION["user_id"]=$row["user_id"]; $_SESSION["ip_addr"]=$_SERVER["REMOTE_ADDR"]; $_SESSION["user_name"]=$row["user_name"]; header("LOCATION: update.php"); ?>
-
or there is no way but to add my template or design to that blog company's edit section of the blog
-
can i just paste my code onto the edit page of blogger for example and link my style sheet and any other links i need to my website style folder etc.....
[SOLVED] media player for videos on website
in Miscellaneous
Posted
this is how i solved it using js.