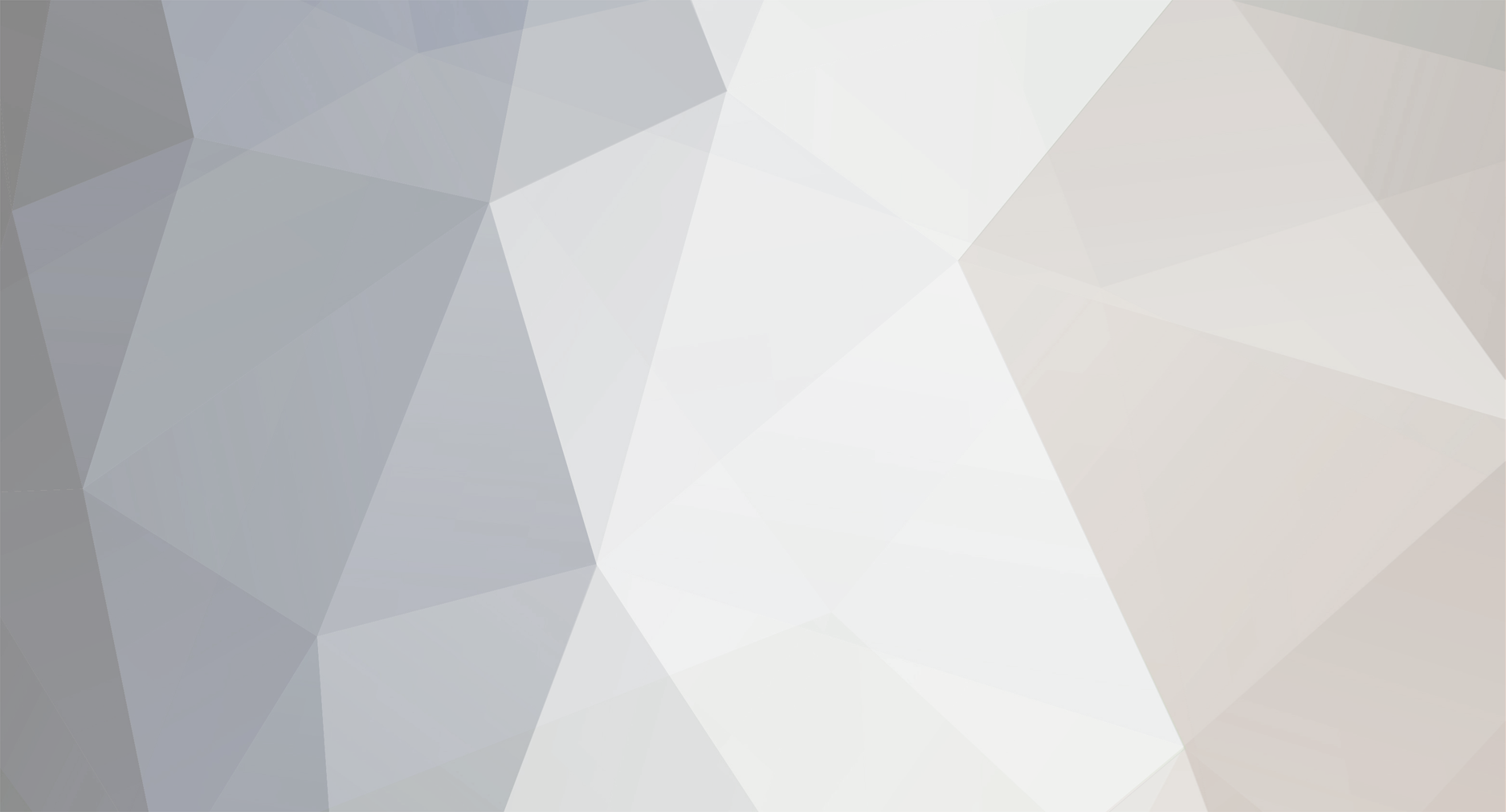
MattDunbar
-
Posts
32 -
Joined
-
Last visited
Never
MattDunbar's Achievements

Newbie (1/5)
0
Reputation
MattDunbar has no recent activity to show
0
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.