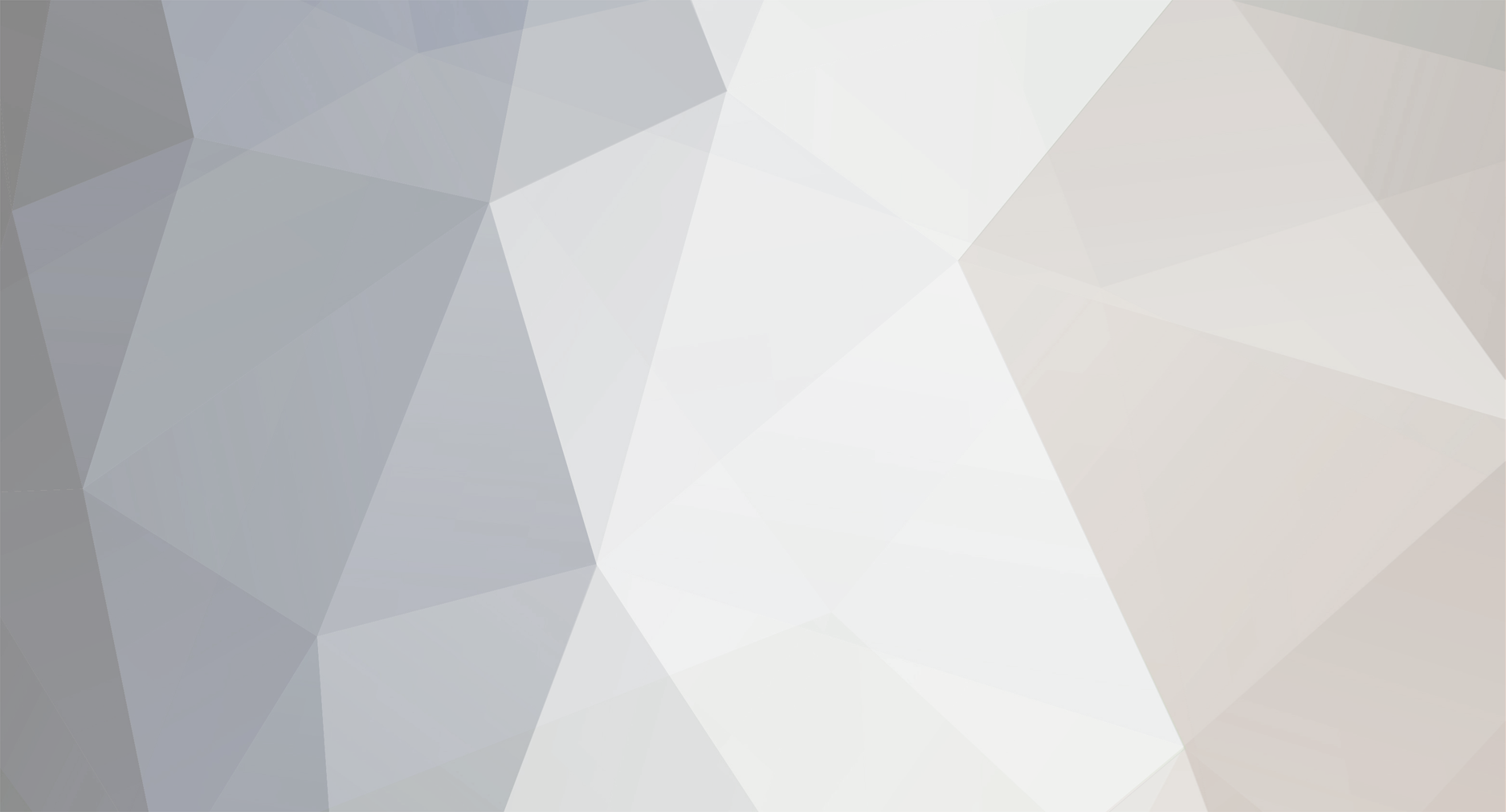
deansaddigh
Members-
Posts
440 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
deansaddigh's Achievements

Advanced Member (4/5)
0
Reputation
-
Can anyone point me to a really good script for building an online shop. ive tried zencart and dont really like it tbh. Cheers guys
-
Whats the best way to protect phpmyadmin. Im not that techy and im trying to learn about securuity. how would i use htacess to protect it. and also should i rename the phpmyadmin folder to something more obscure
-
Fixed it.
-
Ok so im assuming after doing alot of research that the htacess file is only allowing the maintanance page to be displayed, so do i need to modify the htacess code to show the css aswell?
-
any ideas at all. ive still been tying to figure it out for awhile now
-
i have this code RewriteEngine On RewriteBase / RewriteCond %{REMOTE_ADDR} !^11\.111\.111\.111 RewriteCond %{REQUEST_URI} !^/maintenance\.html$ RewriteRule ^(.*)$ http://www.brewskies.co.uk/maintenance.html [R=307,L] Which redirects to a maintance page ignore the allowed ip for now, im jst testing and what to see the maintanance page. the site can be seen here http://www.brewskies.co.uk/maintenance.html why isnt the css being displayed, if i look at firefox and check the css its all there. I am veery confused any any help would be lovely. kind regards Dean
-
Hey and thanks for the link. Would it be to much trouble to show me how i implement that on my code. The reason i ask you to do that if possible , is i have been trying for a week with this to no avail what so ever. So if you could possibly show me how to implement it, ill know for future reference. Kind Regards Dean
-
Hi guys, i have a form that uploads a school photo. All i want to do is resize the image to its widest dimension of a width of 480 px and then obviously constrain the height. can anyone help me, ive tried to do this myself but when it comes to image properties i really struggle etc. heres my upload code. <?php include("includes/connection.php"); // Where the file is going to be placed $schoolimage = "SchoolImages/"; //This path will be stored in the database as it does not contain the filename $currentdir = getcwd(); $path = $currentdir . '/' . $schoolimage; // Get the schoolid for the image and school linker table $schoolid = $_POST['schoolid']; //Get the school name $query = "SELECT * FROM school WHERE school_id = ".$schoolid; $result = mysql_query($query) or die("Error getting school details"); $row = mysql_fetch_assoc($result); $schoolname = $row['name']; //Use this path to store the path of the file in the database. $filepath = $schoolimage . $schoolname; //Create the folder if it does not already exist if(!file_exists('SchoolImages')) { if(mkdir('SchoolImages')) { echo 'Folder ' . 'SchoolImages' . ' created.'; } else { echo 'Error creating folder ' . 'SchoolImages'; } } //Store the folder for the course title. if(!file_exists( $filepath )) { if(mkdir( $filepath )) { echo 'Folder ' . $schoolname . ' created.'; } else { echo 'Error creating folder ' . $schoolname; } } // Where the file is going to be placed $target_path = $filepath; // Add the original filename to our target path. Result is "uploads/filename.extension" echo $target_path = $target_path . '/' . basename( $_FILES['uploadedfile']['name']); if(move_uploaded_file($_FILES['uploadedfile']['tmp_name'], $target_path)) { echo "The file ". basename( $_FILES['uploadedfile']['name'])." has been uploaded"; $filename = $_FILES['uploadedfile']['name']; //Store the filename, path other criteria in the database echo $query = "INSERT INTO image(image_id, name, path) VALUES(0, '$filename', '$filepath')"; //Perform the query $add = mysql_query($query, $conn) or die("Unable to add the image details to the database"); $imageid = mysql_insert_id(); //Store the filename, path other criteria in the database echo $query = "INSERT INTO image_school( image_id, school_id ) VALUES('$imageid', '$schoolid')"; //Perform the query $add = mysql_query($query, $conn) or die("Unable to add the image details to the database"); $message = 'Upload Successful'; } else { $message = 'There was an error uploading the file, please try again!'; } //Close the connection to the database mysql_close($conn); header("Location: add_school_photo_form.php? message={$message}&schoolid={$schoolid}"); //header("Location: add_school_photo_form.php? message=$message, schoolid=$schoolid"); exit(); ?> Id be eternially gratefull. Kind Regards Dean
-
Many thanks, this looks easy enough to follow.
-
I have an upload script which uploads photos. However i want them to all be resized to the same size, as at the moment im having to manually hardcode the dimensions when i want to show them on the front end. Heres my code <?php include("includes/connection.php"); // Where the file is going to be placed $schoolimage = "SchoolImages/"; //This path will be stored in the database as it does not contain the filename $currentdir = getcwd(); $path = $currentdir . '/' . $schoolimage; // Get the schoolid for the image and school linker table $schoolid = $_POST['schoolid']; //Get the school name $query = "SELECT * FROM school WHERE school_id = ".$schoolid; $result = mysql_query($query) or die("Error getting school details"); $row = mysql_fetch_assoc($result); $schoolname = $row['name']; //Use this path to store the path of the file in the database. $filepath = $schoolimage . $schoolname; //Create the folder if it does not already exist if(!file_exists('SchoolImages')) { if(mkdir('SchoolImages')) { echo 'Folder ' . 'SchoolImages' . ' created.'; } else { echo 'Error creating folder ' . 'SchoolImages'; } } //Store the folder for the course title. if(!file_exists( $filepath )) { if(mkdir( $filepath )) { echo 'Folder ' . $schoolname . ' created.'; } else { echo 'Error creating folder ' . $schoolname; } } // Where the file is going to be placed $target_path = $filepath; // Add the original filename to our target path. Result is "uploads/filename.extension" echo $target_path = $target_path . '/' . basename( $_FILES['uploadedfile']['name']); if(move_uploaded_file($_FILES['uploadedfile']['tmp_name'], $target_path)) { echo "The file ". basename( $_FILES['uploadedfile']['name'])." has been uploaded"; $filename = $_FILES['uploadedfile']['name']; //Store the filename, path other criteria in the database echo $query = "INSERT INTO image(image_id, name, path) VALUES(0, '$filename', '$filepath')"; //Perform the query $add = mysql_query($query, $conn) or die("Unable to add the image details to the database"); $imageid = mysql_insert_id(); //Store the filename, path other criteria in the database echo $query = "INSERT INTO image_school( image_id, school_id ) VALUES('$imageid', '$schoolid')"; //Perform the query $add = mysql_query($query, $conn) or die("Unable to add the image details to the database"); $message = 'Upload Successful'; } else { $message = 'There was an error uploading the file, please try again!'; } //Close the connection to the database mysql_close($conn); header("Location: add_school_photo_form.php? message={$message}&schoolid={$schoolid}"); //header("Location: add_school_photo_form.php? message=$message, schoolid=$schoolid"); exit(); ?> If anyone can help me that would be brilliant because i have no idea, how to implement it even after searching google. thanks guys
-
I did indeed sir.
-
Cheers guys, i didnt even realise people posted a reply. thanks for your help
-
i want to remove any links which get pulled out in my general information query. $general_info = $row['general_info']; $general= preg_replace('/<a href="([^<]*)">([^<]*)<\/a>/', '', $general_info); echo '<h2>General Information : <img src="images/mag.png"></h2>'; echo '<div id="featurecontent">'; echo '<p>'. ucfirst($general).'</p>'; echo '</div>'; But the above code doesnt seem to be stripping out links. Can anyone see what i am doing wrong. Kind Regards Dean
-
Many thanks, i have done it. thanks again mate kind regards Dean
-
i would like to resize it before its saved onto the server ideally