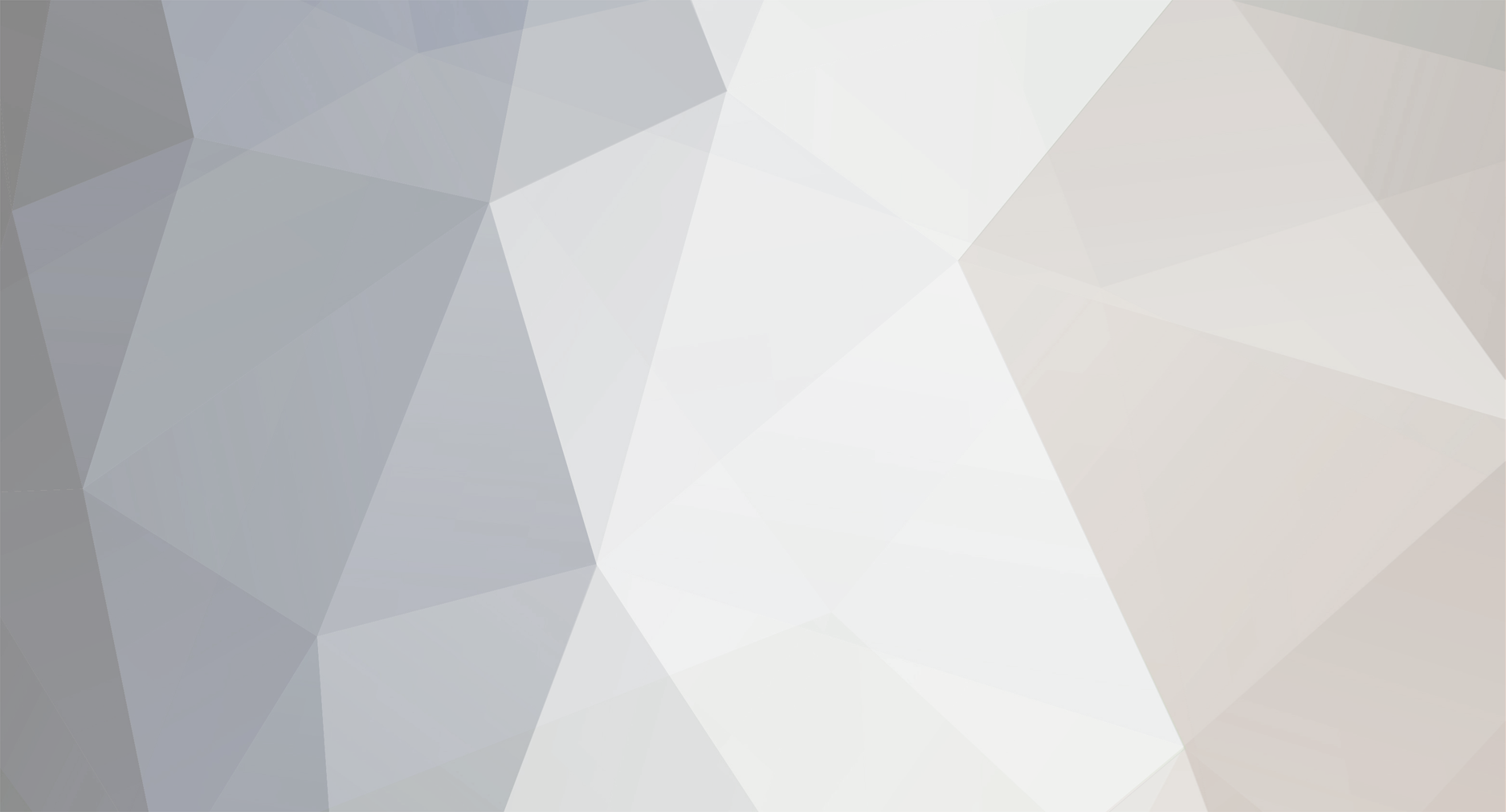
shamuraq
Members-
Posts
142 -
Joined
-
Last visited
Everything posted by shamuraq
-
Hi, I have an array that i want to 'echo' in a foreach loop: foreach($numbers as $num){ echo $num.':'; } i need the output to be 459:534:141 instead of 459:534:141:. How do i remove the ':' at the final loop? Thanx in advance.
-
I have this list as an array: 1. [Question - Geography Chapter2] How would you describe humans' relationship with the physical environment? (Page 42) 2. [Question - Geography Chapter4] What is a natural resource? (Page 67) 3. [Question - Geography Chapter3] What are two or three resources which you cannot do without? What are the reasons for your choices? (Page 52) ... 129. [Question - Geography Chapter6] What is the human-centered view on the use of natural resources? (Page 339) 130. [Question - Geography Chapter7] What are the benefits of using products with a smaller environmental footprint? (Page 342) I would like to remove: - The square brackets and everything in it. I am hoping that the questions would start without the eg; [Question - Geography Chapter7] etc. - The round brackets and everything in it. I am trying to delete the references to the page number. Is there a simple an clean way to do it? Any pointer is truly appreciated. Thanx in advance.
-
Using preg match to find specific cluster of files
shamuraq replied to shamuraq's topic in Regex Help
What does the values in 0,5 in substr($v, 0, 5) refers to? -
Using preg match to find specific cluster of files
shamuraq replied to shamuraq's topic in Regex Help
I tried as suggested: $arrFiles = array(); $dirPath = "./"; $files = scandir($dirPath); $required = array_filter($files, fn($v)=>str_starts_with($v, 'alg00')); echo '<pre>' . print_r($required, 1) . '</pre>'; The error: Fatal error: Uncaught Error: Call to undefined function str_starts_with() ...php:29 Stack trace: #0 [internal function]: {closure}('.') #1 /...php(29): array_filter(Array, Object(Closure)) #2 {main} thrown in ...php on line 29 -
Hi guysm I have a folder containing these files: alg001.php alg002.php alg003.php alg004.php alg005.php algComp.php ranFrac.php ranalg.php randec.php randint.php I would only like to store the names of files alg001.php, alg002.php, alg003.php, alg004.php, alg005.php into an array. How do i achieve this with preg match?
-
how do i convert the following script to adopt ternary operator to shorten it? $radint = mt_rand(1,33); $flip = mt_rand(1,2); if($flip == 2){ $radint *= -1; } Thanx in advance.
-
How to output array instead of a 'loop' echo return from a function?
shamuraq replied to shamuraq's topic in PHP Coding Help
Tes... Thats exactly what i wanted. But can you explain the breakdown of "%-35 = %5d <br>"? -
I have a function randomly take 4 unique numbers from an array and multiply them. Problem with the script below is it only print out the products. I would like for each set of 4 numbers and its product to be in its own array and all the arrays into a main array, forming a 2 dimensional array. My current script: //Pruning outcome from controlled lists of Prime Numbers $primes = array(2, 3, 5, 7, 11, 13, 17, 19, 23); function getAllCombinations($arr, $n, $selected = array(), $startIndex = 0) { if ($n == 0) { $product = 1; foreach ($selected as $prime) { $pr[] = $prime; $product *= $prime; $pr[] = $prime; } echo "Product: $product\n"; return; } for ($i = $startIndex; $i < count($arr); $i++) { $selected[] = $arr[$i]; getAllCombinations($arr, $n - 1, $selected, $i + 1); array_pop($selected); // Backtrack and remove the element for next iteration } } getAllCombinations($primes, 4); The output: Product: 210 Product: 330 Product: 390 Product: 510, etc. The preferred method is a 2 dimensional array as such: [0][0] =>2 [0][1] =>3 [0][2]=>5 [0][3]=>7 and the product would be in [0][4] =>210 [1][0] =>2 [1][1] =>3 [1][2]=>5 [1][3]=>11 and the product would be in [1][4] =>330 etc. Any pointers is greatly appreciated.
-
Hi guys, I am trying to convert randomized float to fractions. For eg; 66.666666666667 = 66 2/3 Any pointers?
-
Its like this:' $value = array( array(3075,15,461.25,16,535.05), array(3075,15,461.25808,16,535.05),//to be removed array(3075,15,461.2,16,535.05234)//to be removed ); $value = array_filter($value, function ($v) { foreach ($v as $x) { if (strlen(substr(strrchr($x, "."), 1)) > 2) return false; } return true; }); var_dump($value); But nothing came out during testing. What could be wrong?
-
Hi guys, I am looking fer a way to delete numbers with decimal values more than 2 decimal place in my arrays. Any pointers? Thanx in advance.
-
Hi guys, am not very good with regex. I need to replace all double backslashes '\\' in my string into single backslashes '\'. I tried: $pattern = '[\\]'; $replacement = '/\/'; ?> <td width="100%"> <?php echo preg_replace($pattern, $replacement,$q[$i]);?></td> i also tried a few other combinations but the output is either blank or more backslashes are added. Any pointers are appreciated. Thanx in advance.
-
Hi all, I'm trying to list the possible combinations in fraction from 1/1 + 1/1 until 9/9 + 9/9. So i came up with this <?php //numbers of combination for w/x + y/z $num1=1; $den1=1; $num2=1; $den2=1; $row=1; for($w=0; $w<9; $w++){ echo $row.') '; $row=$row+1; echo $num1.'/'.$den1.' + '.$num2.'/'.$den2.'<br>'; $num1=$num1+1; for($x=0; $x<9; $x++){ echo $row.') '; $row=$row+1; echo $num1.'/'.$den1.' + '.$num2.'/'.$den2.'<br>'; $den1=$den1+1; for($y=0; $y<9; $y++){ echo $row.') '; $row=$row+1; echo $num1.'/'.$den1.' + '.$num2.'/'.$den2.'<br>'; $num2=$num2+1; for($z=0; $z<9; $z++){ echo $row.') '; $row=$row+1; echo $num1.'/'.$den1.' + '.$num2.'/'.$den2.'<br>'; $den2=$den2+1; } } } } ?> The problem is it parse all the way to 7380) 10/82 + 730/6561. Where did it go wrong? Thanx in advance...
-
Solved it... I guess the problem is because am trying to store it in a variable... But i changed to to echo nl2br("$summary"); But am curious tho... Is there anyway to store it in a variable without causing those errors?
-
Followed ur advice and removed the nl2br from the insert into sql file. But am still getting the same error.
-
Hi... I read on php.net manual that its better to use str_replace than preg_replace. I used $summary = clean($_POST['summary']); $summary = nl2br($summary); to convert the carriage return(is that the correct term?) to insert them into mysql. So naturally, i want them converted into <br> when i pull them from mysql. I used this: $num_rows=mysql_num_rows($result); if($num_rows == 0){ } else{ for($x = 0; $x < $num_rows; $x++){ $row = mysql_fetch_assoc($result); $id = $row['id']; $position = $row['position']; $summary = $row['summary']; $order = array("\r\n", "\n", "\r"); $replace = '<br />'; $newstr = str_replace($order, $replace, $summary); But i got this error: Parse error: syntax error, unexpected T_CONSTANT_ENCAPSED_STRING in /home/...php on line 310 Is it a syntax error? Thanx in advance...
-
thanx .josh. Really appreciate it...
-
I tried this <?php $string = 'what do u get 1/2?'; $pattern = '/(\d+) (/) (\d+)/i'; $replacement = '$1 | $3'; echo preg_replace($pattern, $replacement, $string); ?> but i didn't work... Nothing came out
-
What do u get if 1/2 + 1/3? How do i use preg replace to select the fractions part? I need to add html <strong> to bold only the fractions part. Is there regex involved?
-
Please advise me on what are the complications i could encounter if i do a table for every user? I am still learning the ropes and would appreciate the guidance...
-
yupp...
-
Hi guys, I have been using the "CREATE TABLE IF NOT EXISTS" with no problem until today... It keeps on giving me error. So i logged in to my phpMyAdmin to test it out on the sql console and it responded with #1064 - You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near '"CREATE TABLE IF NOT EXISTS `[email protected]` ( `id` INT( 7 ) NOT NULL AUTO_IN' at line 1 this is the syntax that i used on phpMyAdmin: "CREATE TABLE IF NOT EXISTS `[email protected]` ( `id` INT( 7 ) NOT NULL AUTO_INCREMENT PRIMARY KEY , `ref` VARCHAR ( 200 ) NOT NULL , `date` VARCHAR(10) NOT NULL , `time` VARCHAR(10) NOT NULL , `action` TEXT , `score` VARCHAR(4), `credit_charge` INT( 4 ) NOT NULL , ) ENGINE = innodb;"; i referred to the error that phpMyAdmin returned (error #1064) and found out it highly likely the usage of reserved keyword (http://dev.mysql.com/doc/refman/5.0/en/reserved-words.html). But i cannot find any reserved keyword that i could've used in my query so far... Any ideas?
-
can u describe the scenario in better detail?