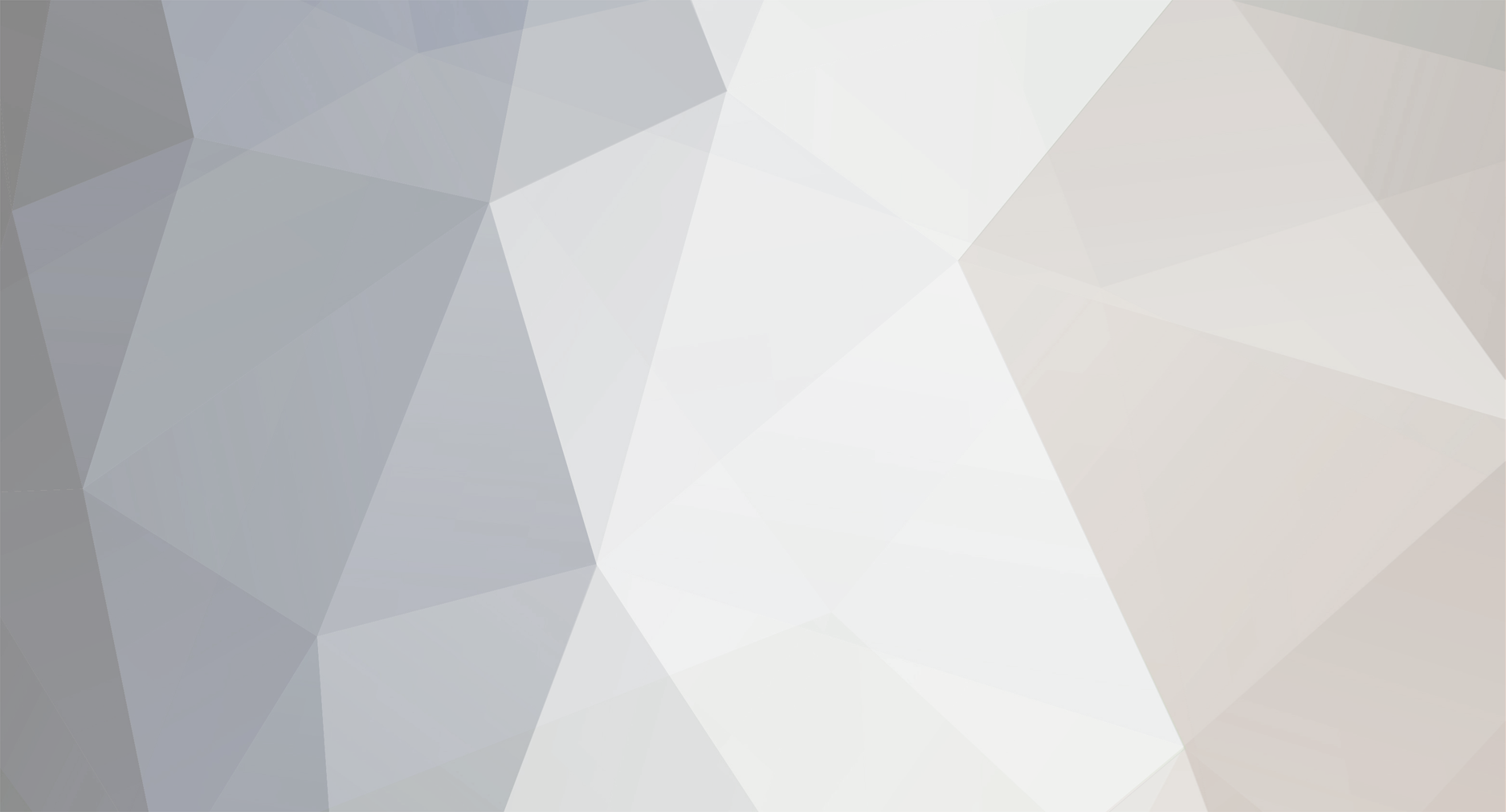
Solar
Members-
Posts
147 -
Joined
-
Last visited
Profile Information
-
Gender
Not Telling
Solar's Achievements

Regular Member (3/5)
0
Reputation
-
PHP Template Class Rendering with Arrays/Loops/PregMatch/PregReplace
Solar replied to Solar's topic in PHP Coding Help
Yes thanks, I guess that could work and my loop function could work as follows: protected function loop($output, $key, $values){ $pattern = "/\($key\)(.*?)\(\/$key\)/s"; preg_match($pattern, $output, $matches); $section = $matches[1] ?? ''; $result = ''; $out = $section; foreach($values as $key => $value){ $out = str_replace("(/".$key.")", $value, $out); } $result .= $out; return preg_replace($pattern, $result, $output); } HTML: <div> (0)<b>(/name)</b> (/age)(/0) (1)<b>(/name)</b> (/age)(/1) </div> This would be great for fixed results. I could write another loop function to check the output and if there is any (/name)s or (/age)s that are not filled in, strip/replace/fill them with blanks. This would be great for pagination rows/lists/tables or even a fixed navigation! @jodunno I appreciate your help but I just used push as an example. There is nothing wrong with using push if you need to add multiple arrays inside a one-liner -- while array[] does save time if adding one or two. My question which was geared more towards the Template class in how I can loop. -
I have a Template class that accepts single values and 3d multidimensional arrays. So, if I provide a simple array: array("name"=>"Steven", "age" => 34); it works nicely by looping and replacing the HTML (/name) and (/age). foreach($this->values as $key => $value){ $output = str_replace("(/".$key.")", $value, $output); } If I provide it with a multidimensional array, it works too. Example: array("logos" => $portfolio->getPortfolioByCat("2")); //puts an array inside of an array so that the HTML file can loop between (logos)(/name)(/image)..etc(/logos) I'm struggling with a 2d (I think?) array. Here's an example: Let's say I have two names and ages and push into one array. $multi = array(); $steven = array("name" => "Steven", "age" => 34); $jason = array("name" => "Jason", "age" => 32); array_push($multi,$steven,$jason); I now have two (/name) and two (/age). I do not wish to load the view template twice (or multiple times). I would like to reference once and echo the output multiple times but I'm stumped. The template class below is an example of how the 3d array loops which is called by a render function. Since my 2d array above does not contain an actual unique key and a values that is an array, how can safely loop through? Or do you recommend that I wrap it into another array (seems repetitive) that contains a key. $newMulti = array("names" => $newMulti) and the html would be (names)(/name)(/age)(/names). class Template{ //variables //render function (grabs template file, sets an output, calls the loop function, echo the results in output) protected function loop($output, $key, $values){ $pattern = "/\(\$key\)(.*?)\(\/$key\)/s"; preg_match($pattern, $output, $matches); $section = $matches[1] ?? ''; $result = ''; foreach($values as $item){ $out = $section; foreach($item as $key => $value){ $out = str_replace("(/".$key.")", $value, $out); } $result .= $out; } return preg_replace($pattern, $result, $output); } }
-
Simply perfect! To know that this is fine to create a new object and then call the get function to retrieve the pdo relieves a lot of stress! Thanks a billion for this quick reply!
-
Hey, I'm stumped. Through out this post, I will bold my questions. Is global $pdo; frowned upon? is it safe? is there a better way to access it? As of right now, I'm using the easy way of creating a database php file and including it in specific php pages to access the $pdo variable but I would like a different approach. I would like to use a database class. Let's say I have my class/class_database.php -- converted from a non-class. <?php class Database{ private $pdo; function __construct(){ try{ $pdo = new PDO('mysql:host=HOST;dbname=DBNAME', "USER", "PASSWORD"); $this->pdo->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); }catch(PDOException $e){ echo 'Connection failed: '.$e->getMessage(); } } } ?> I would like to create a separate class called Statements: class Statements{ function select($sql,$arr){ $stmt = $this->pdo->prepare($sql); if(empty($arr) == false){ foreach($arr as $k => $v){ $stmt->bindValue($k, $v); } } $stmt->execute(); return $stmt->fetchAll(PDO::FETCH_ASSOC); } } The problem I'm having is that I would have to use extends in order to access the private pdo: class Statements extends Database Is extending a Database class safe or recommended? If not, how can I use a construct inside of Statements to obtain the Database Class PDO variable? Because every time Statements is called, it will need to use the pdo from the Database Class. class Statements{ private $pdo; function __construct(Database $newPDO){ $this->pdo = $newPDO; } //functions below... } How exactly do I pass the pdo to the __construct function and make the call? I would easily assume that creating a get function inside of the Database Class to get the private $pdo variable is risky business. After doing a bunch of research online, it seems like programmers fight over global pdo variables and extends of the Database because it logically doesn't make sense. Unfortunately, I have yet to find a reasonable answer. I appreciate your time!
-
As if it's that simple! Thanks a ton! Edit: I guess either way, I still have to specify the variables. I'm good with this! $account = new Account(); $account->getUserInfo($email,$rank); class Account{ function getUserInfo(&$email,&$rank){ global $con; $stmt = $con->prepare('SELECT email, rank FROM accounts WHERE id = ?'); $stmt->bind_param('i', $_SESSION['id']); $stmt->execute(); $stmt->bind_result($email, $rank); $stmt->fetch(); $stmt->close(); } }
-
Let's say I have a simple statement. $stmt = $con->prepare('SELECT email,rank FROM accounts WHERE id = ?'); $stmt->bind_param('i', $_SESSION['id']); $stmt->execute(); $stmt->bind_result($email,$rank); $stmt->fetch(); $stmt->close(); I can then echo the e-mail and rank variables very easily in PHP <?=$email?> <?=$rank?> What is the best way to echo the results with a bind_result while it's inside of a function? function getUserInfo(){ global $con; $stmt = $con->prepare('SELECT email,rank FROM accounts WHERE id = ?'); $stmt->bind_param('i', $_SESSION['id']); $stmt->execute(); $stmt->bind_result($email,$rank); $stmt->fetch(); $stmt->close(); } I could easily stick email and rank into an array and return it but that's duplicating code. Is there a way to fetch the bind_result and return all variables inside of it? I greatly appreciate it 🙂
-
Why are you re-initializing the following $params array? public function query($sql, $params = array()) { Should be just this, no? public function query($sql, $params) {
-
Apparently since I cannot enforce referential integrity, I must deal with it using an INNER JOIN statement if I want to run SELECT statements. INNER JOIN TimeZonesTableON Location=SUBSTRING(ClientID,6,3) Thanks Barand for your help.
-
I don't see why not. The instructions given to me did not inform me that ClientID was unique or not. Would there be a huge difference if it was unique? If the concept is stripping the numbers off of ClientID and referencing it that way... is that possible?
-
Hey PHPFreaks! I've ran into a problem and hoping you could help me out. I did not design these tables and I am having problems enforcing referential integrity. Is a constraint required for this task? Here are the two tables ClientsTable (ClientID, ClientName) VALUES("11111TOR","JOE"); VALUES("22222OTT","BOB"); VALUES("33333NEW","PAM"); TimezoneTable (Location, Timezone) VALUES("OTT", "EST") VALUES("TOR", "EST") VALUES("CAL", "MDI") VALUES("NEW","EST") There is a one to many relationship between TimezoneTable and ClientsTable. Due to the data, I cannot enforce referential integrity. What is a solution in joining these together? Could I do so by creating a new table? How could I go from there? Thanks in advanced, Solar
-
I have decided to take a different approach to this method. After researching; everyone's reply is the same response I recieved when reviewing the PHP.net website. Thank-you for your time, if the method was possible, it would have saved me a ton of time. But in the long run, the method I expected is not proper. Thanks again, Solar
-
I understand the $_GET[''] function.. but I have ran into an error. Lets say my URL is localhost.php/test.php?say=#hello The $_GET[''] function will only take the print after the equal and before the pound sign. - Which is blank My question is... What function should I use to parse after the # sign? Thanks, Steven
-
I've decided to use STRIP_TAGS method and it worked. $message = htmlentities(strip_tags($_POST['message'])); Please correct me if I am using this wrong.
-
Well, I never realized this but when submitting a form, either Quotation Marks or Apostrophes works. I maybe "bad coding" it. To have Quotation Marks work <?php mysql_query("INSERT INTO wallposts (username, userfriend, date, ip, message)VALUES(\"$username\", \"$userfriend\", \"$date\", \"$ip\", \"$message\")"); ?> To have Apostrophes work <?php mysql_query("INSERT INTO wallposts (username, userfriend, date, ip, message)VALUES('$username', '$userfriend', '$date', '$ip', '$message')"); ?> I mainly need both to work for $message. Is there a simple solution? Do I need to extend a variable? Thanks! Edit: Extending my question for a better understanding. When a user uses characters like quotation marks and or apostrophes one or the other works, depending on the code provided above. How can I fix this?
-
I was able to find some old files. /****** build the pagination links ******/ // range of num links to show $range = 3; // if not on page 1, don't show back links if ($currentpage > 1) { // show << link to go back to page 1 echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=1'><<</a> "; // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$prevpage'><</a> "; } // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($x == $currentpage) { // 'highlight' it but don't make a link echo " [<b>$x</b>] "; // if not current page... } else { // make it a link echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$x'>$x</a> "; } // end else } // end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $currentpage + 1; // echo forward link for next page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage'>></a> "; // echo forward link for lastpage echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$totalpages'>>></a> "; } // end if /****** end build pagination links ******/ I hope the issue gets fixed soon! Thanks!