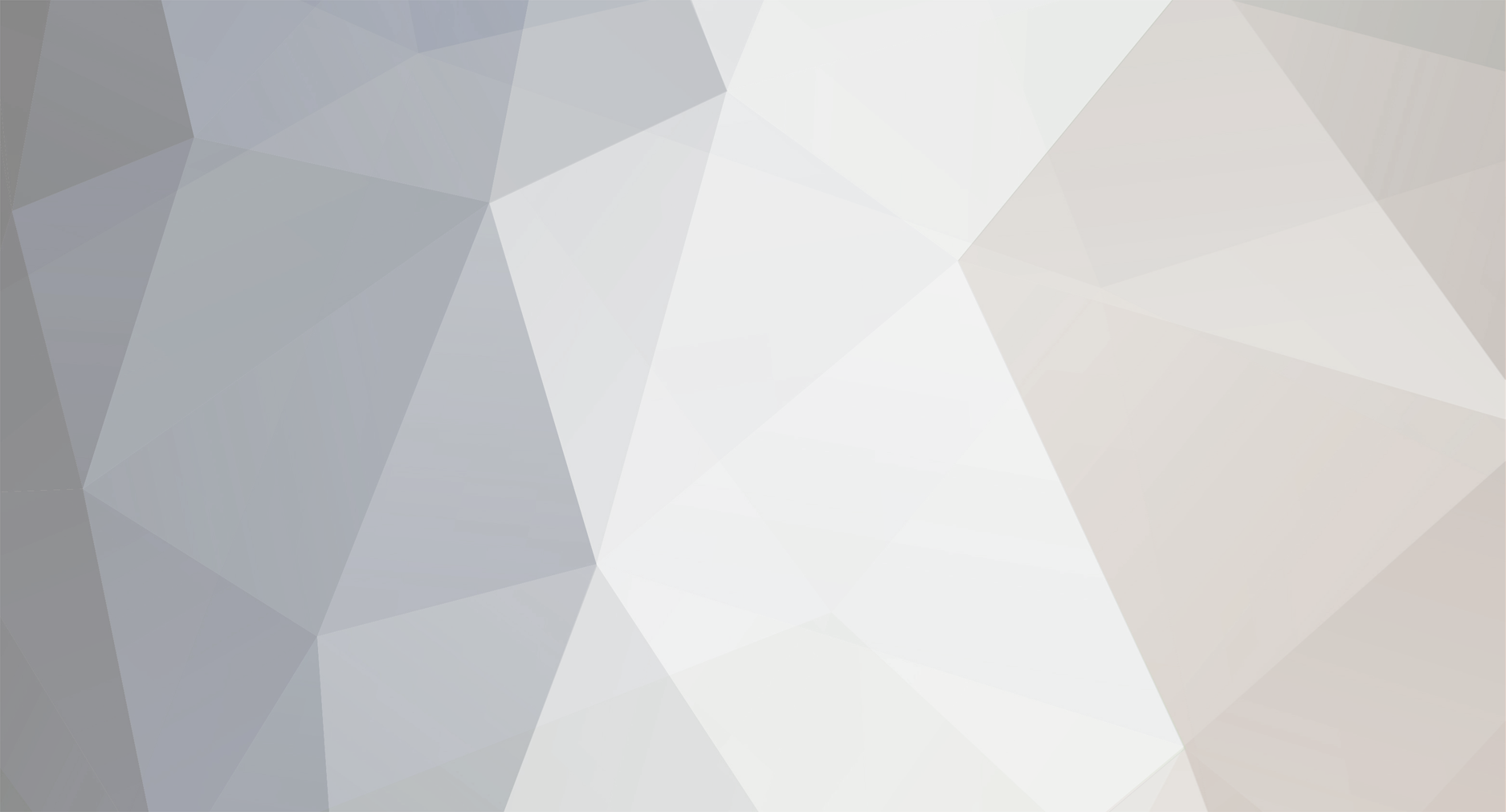
J.Daniels
-
Posts
143 -
Joined
-
Last visited
Never
Posts posted by J.Daniels
-
-
$curramm is an array.
$ammount = $_POST['ammount'] + $curramm;
should be:
$ammount = $_POST['ammount'] + $curramm[0];
-
I think the problem is in this line:
26 if (@mysqli_num_rows($r) == 1) { // A match was made.
To check the number of rows returned when using mysqli:
26 if ($r->num_rows == 1) { // A match was made.
Also, that probably didn't throw an error because error suppression was on (The @ before the function call)
EDIT:
After looking through your code more, it appears all the SQL function calls may be through a custom interface. I would take off the error suppression to see if any errors come up:
26 if (mysqli_num_rows($r) == 1) { // A match was made.
-
That is because you are trying to echo out the results of the query:
$result = $mysql->query($sql); if ($row = $result->fetch_assoc()){ echo $row['rating']; } else { echo "no results, but here is the error: ",$mysqli->error; }
-
Also, you can add mysql_error() after trying to INSERT to see if there is an error:
mysql_query("INSERT INTO place (ID, Name) VALUES ($newID, $_POST[name]") or die("Error, Insert Failed: ".mysql_error());
-
If you are using single quotes inside of double quotes, you don't have to escape them.
$sql = "SELECT rating from users where username = 'Chris'";
As a side note, MySQL works fine with double quotes.
-
Does your host allow a custom php.ini?
One way to view what settings are loaded is to create a file with:
<?php phpinfo(); ?>
View that in your web browser and it will list all the PHP settings. Look for upload_max_filesize.
-
Can you do a view source and post the output of the generated code?
-
You are missing a closing quote for the href:
<a href="view.php?ID=<?php echo ($_SESSION['results'][$i]['ID'])?>"><?php echo htmlspecialchars(stripslashes($_SESSION['results'][$i]['name'])); ?></a>
-
To format output text from PHP, you need new line characters for line breaks:
<?php echo "\n"; ?>
-
There is upload_max_filesize set in php.ini which defaults to 2 MB.
-
Also, you need to specify $this when referencing a class variable:
Class ABC { static $taken = array(); function setArray() { // connect and query etc.. // extract all the mysql and store it in an array of taken urls while($row = mysql_fetch_array($results)) { $this->taken[] = $row['the_url']; } } function getArray() { // return the array so that a btree can search through them. return $this->taken; } }
-
Sorry, misread the question.
Since the url will be passed through the form, you should be able to access it through the $_POST['url'] variable.
-
<form action="populate.php?url=<?php echo $_SERVER['REQUEST_URI'] ?>" method="post" name="url">
-
Looking through the class code, $conn->getResultSet('SELECT * FROM blog'); returns an instance of Pos_MysqlImprovedResult.
-
Do either a print_r() or var_dump() on $result. Make sure it is an array that you can loop through.
-
This may work:
$query_product = "SELECT *, MATCH (prodName,prodBrand,prodCategory) AGAINST (UPPER(".quote_smart($pluspieces).") IN BOOLEAN MODE) AS relevance FROM affiliSt_products1 WHERE MATCH (prodName,prodBrand,prodCategory) AGAINST (UPPER(".quote_smart($pluspieces).") IN BOOLEAN MODE) $extracondition HAVING relevance > 0 $sortby";
-
PHP is a server side scripting language, so once content is rendered, PHP cannot modify it. You'll have to use JavaScript to change the action value when selecting an option.
-
I think the search is case sensitive.
-
$links = "http://site.com<br> http://site2.com<br> http://site3.com<br>"; $linksArray = explode('<br>', $links); $counter = 1; $links = ''; foreach ($linksArray as $l) { if ($l != '') { $links .= $l . '<br> ' . $counter . ' '; $counter++; } } echo $links;
You have to reset $links before adding the result. Also, if you don't want the last blank line, check to see if it is an empty string.
-
By default, MySQL will perform a natural language search. To change it to a boolean search, try making these changes:
$pluspieces .= $pieces[$pi].' ';
to:
$pluspieces .= '+'.$pieces[$pi].' ';
and
$query_product = "SELECT *, MATCH (prodName,prodBrand,prodCategory) AGAINST (".quote_smart($pluspieces).") AS relevance FROM affiliSt_products1 WHERE MATCH (prodName,prodBrand,prodCategory) AGAINST (".quote_smart($pluspieces).") $extracondition HAVING relevance > 0 $sortby";
to:
$query_product = "SELECT *, MATCH (prodName,prodBrand,prodCategory) AGAINST (".quote_smart($pluspieces)." IN BOOLEAN MODE) AS relevance FROM affiliSt_products1 WHERE MATCH (prodName,prodBrand,prodCategory) AGAINST (".quote_smart($pluspieces)." IN BOOLEAN MODE) $extracondition HAVING relevance > 0 $sortby";
-
What is the value of $pluspieces when you do a search?
-
You can also filter them through the SELECT statement. Depending on how site is stored
$r=mysql_query("SELECT * , itemsa - itemsb AS itemstotal FROM `$table[0]` WHERE site != '' ORDER BY itemstotal DESC");
or
$r=mysql_query("SELECT * , itemsa - itemsb AS itemstotal FROM `$table[0]` WHERE site IS NOT NULL ORDER BY itemstotal DESC");
-
I don't think register_globals would make a difference. He is assigning $_GET['name'] to $name then trying to echo $name.
-
What isn't working?
I can see one problem. There is a syntax error:
$query="CREATE TABLE websitestable (websites varchar(100) NOT NULL,destination varchar(50) NOT NULL)
should be:
$query="CREATE TABLE websitestable (websites varchar(100) NOT NULL,destination varchar(50) NOT NULL)";
However, that query will fail after the first time the script is run since the table will already be created.
SQL Question
in MySQL Help
Posted
Just calculate the range before creating your SQL query then use BETWEEN