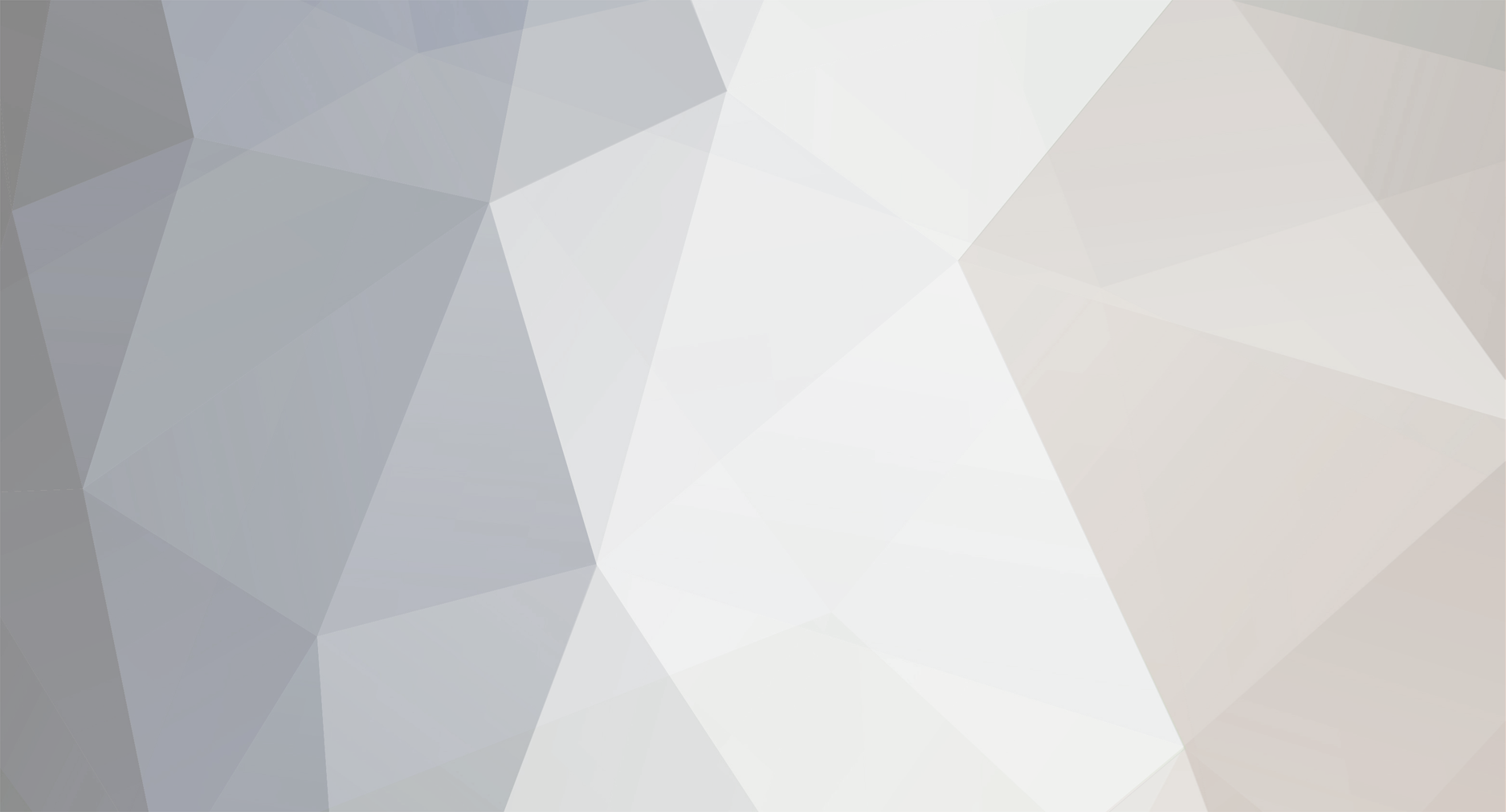
RussellReal
Members-
Posts
1,773 -
Joined
-
Last visited
Everything posted by RussellReal
-
idk if u could use 'or' laik dat lol
-
one class I made was to handle data recieved by a server script (which is just a socket and a loop) but it was pretty neat and then I extended that into a whole class for just starting/running a server.. and then I made a class for mysql connections to make it easier, and then I made a user class which handled data pertaining to a specific user in a members' only area.. there is millions of ways/reasons to use OOP, but there is some things which really don't need OOP like my user class or my server class.. you see,, OOP is exactly what its name states "Object Oriented" objects are meant to be reinitialized, over, and over.. for example <?php class Pet { public $name, $age, $type; public function __construct($type,$name,$age) { $this->name = $name; $this->age = $age; $this->$type = $type; } public function getAge() { return $this->age; } public function __toString() { return $this->name.'('.$this->age.') is a '.$this->type; } } ?> $rosco = new Pet('dog','rosco',3); echo $rosco->getAge(); // 3 echo $rosco; // rosco(3) is a dog but also some people make classes so that they could package a script into a file which is suitable for opensource packaging, or reusability.. because with a class its about being a blank shell.. like plant pot it has ALL the means to grow a plant.. you just need to plant the seed you want inside the pot, and apply some PlantPot::love() and PlantPot::waterThePlantPot() until it is completely as you want it to be.
-
file_exists returns TRUE or FALSE if the specified filename exists ornot.. NOW I think as of 4.6 or 5.0 file_exists now will return true or false for some urls like http://whatever.com/whatever.php now.. if that exists on whatever.php then it will return true, and then whatever code you have inside "whatever.php" that will be "included" into your page. now if they have un-evaluated php inside of whatever.php then now they hacve control over your files
-
[SOLVED] PHP Form | check box issues | need help!
RussellReal replied to passam's topic in PHP Coding Help
landavia name="check[]" is supported by php it will form an array inside of $_POST['check'] so his code is correct, it is just not grabbing anything.. OOOH also if the checkbox isn't checked, it won't show up in your POST.. which could explain the error, you could hide warnings.. or output buffer ob_start -
ok most times with objects (I'm assuming OCI is a package or something) when you do like $oci = new OCI; echo $oci; when you echo the variable it activates the ->toString() method inside the function which then gives the output in STRING form.. most pre-built objects have this, however, if you're working on a class you'd use __toString with the prepended underscores. now, you can't store a resource/stream/object inside of a session or file either basically because its impossible for the script to keep the connection/stream alive when php terminates. what you can do, is pass the data that you will USE with OCI via sessions
-
as in global $varName; ? well thats really outdated and hardly practiced anymore, you should instead use references since global you know you want that variable.. so you'd do like function whatever(&$var) { $var = "hello!"; } $abc = ''; whatever($abc); echo $abc; // "hello!" instead of function whatever() { global $abc; $abc = "hello!"; } $abc = ''; whatever(); echo $abc; // "hello!"
-
your while loop should be something like while ($row = mysql_fetch_assoc($result)) { } HOWEVER you set $result inside a function's scope, not the script's scope, therefore once the function exits the variable is garbage collected. what you could do is ABOVE function getChallenges in your class somewhere after class *** { // like right here } you'd put public $result; (assumingh you're on 5.0+); then when you do: $result = mysql_query($q, $database->connection); you would do $this->result = mysql_query($q, $database->connection); instead so then later in the script for the while loop you'd do while ($row = mysql_fetch_array($challenges->result)) { }
-
anytime man, and I think its nice you're doing sumfin for the kids (judging by your username)
-
sasa beat me to it SELECT COUNT(unit_id) FROM `squads` GROUP BY `unit_type`
-
[SOLVED] PHP Form | check box issues | need help!
RussellReal replied to passam's topic in PHP Coding Help
Warning: Invalid argument supplied for foreach() in /home/content/a/c/r/mysite/html/sms.php on line 31 this warning is what sends your headers.. then you get the error seen below which is headers already sent.. and as for line 31 $_POST['check'] is not an array.. try <?php print_r($_POST); ?> and see what it has for 'check' -
when a php script ends, your script destroys all resources, objects, variables, streams, etc.. not sure what OCI is but I'm sure the above explains your problem
-
use die instead of echo or ofcourse use header("Location: [ADDRESS]");
-
I'm assuming 70 days would be 10 weeks.. and if so create a table with 5 fields id - int - auto increment home_team - text ^^ something like "BunnySlippers" away_team - text ^^ something like "TeddyBears" week - int ^^ something like 1-10 for like.. week 1.. week 2.. day - int ^^ something like 1 - 7 for day1 day2 day3 then when you want to pull week 2 of the database you'd just do "SELECT * FROM `yourTable` WHERE `week` = '2'"
-
first of all this: $contentData = file_get_contents($downloadFile); does nothing in ur script second of all.. there is no extra data/whitespace beng shown.. it must be put into the DB when you UPLOAD it in there. - Russell
-
oooh I'm sorry!!! lol I tested it then edited it and I messed it up <?php function flipele(&$ele) { $z = ''; for ($i = 0; ($a = $ele{$i}) !== false; $i++) { $z = $a.$z; } $ele = $z; } $string = "0110 1011"; $arr = explode(" ",$string); array_walk($arr,"flipele"); print_r($arr); ?> there use that bro I'm sorry
-
not that hard.. a simple session or cookie.. and a login page setcookie session_start
-
ok.. create a landing page for all of the images.. then echo the content of the image inside the php file.. example image.php?file=whatever.jpg then inside of image.php do something like <?php $file = "images/".$_GET['file']; // here record it as a click for $file in mysql $e = getimagesize($file); header("content-type: {$e['mime']}"); readfile($file); ?>
-
ahh u c thats a problem.. you could put the <option> tag's values and html inside an array like $options = array( 'male' => 'Male', 'female' => 'Female' 'other' => 'I\'m A Freak!' ); and then <select name="gender"> <?php foreach ($options as $value => $text) { if ($_REQUEST['gender'] == $value) $selected = " selected='selected'"; echo '<option value="'.$value.'"'.$selected.'>'.$text.'</option>'; $selected = ''; } ?> </select>
-
ok <input type="text" name="location" value="<?php echo $_REQUEST['location']; ?>" />