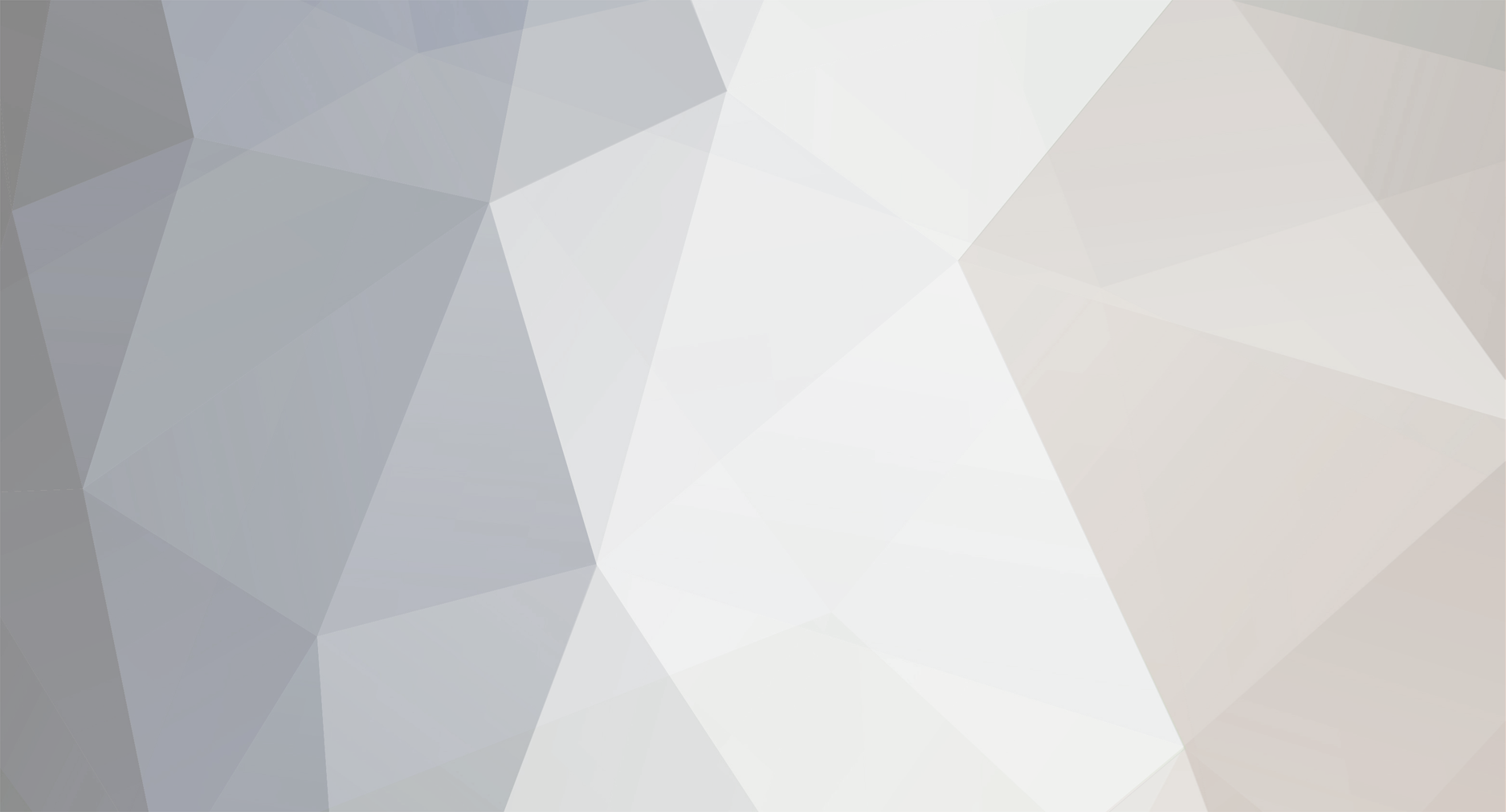
RussellReal
Members-
Posts
1,773 -
Joined
-
Last visited
Everything posted by RussellReal
-
yes, on every page that you are wanting to ACCESS/MODIFY the session variable you will call session_start, it will then load whatever session that the user's session id represents! You only need to define something once in a session variable, after that it will exist in any further interactions with that particular session, unless ofcourse you unset that particular variable so for example.. session_start(); $file = $_GET['file']; if (!isset($_SESSION['answers'])) $_SESSION['answers'] = array(); $_SESSION['answers'][$file] = $_POST['answer'];
-
I gave you code that makes it work, it SHOULD work, but its untested as far as the sessions, yes its a good idea to store the answers to the questions in a session, makes it alot easier obviously, HOWEVER, you don't need a new session PER answer, store it all in an array, in the user's default session
-
as Ken stated, but you could do it like this! <?php session_start(); $file = $_GET['file']; session_register($file); //INITIALIZE ERROR VARIABLES $errorFound = false; $errorIcon[$file] = ''; if (isset($_POST['submitbutton'])) { //SET SESSION VARIABLES $_SESSION[$file] = $_POST[$file]; //TEST FORM INFORMATION if(count($_POST[$file]) != 3) { $errorFound = true; $errorIcon[$file] = 'Error Question 2'; } //IF NO ERRORS WERE FOUND, CONTINUE PROCESSING if (!$errorFound) { header( "Location: hazardresult.php" ); } } ?> and then instead of http://xyz.com/hazard2.php do: http://xyz.com/hazard.php?file=hazard2
-
because of the reason I told you... if it conflicts it will just fail.. there is no failsafe..
-
the best way to handle it is with my example.. the reason being, the first reply's code will generate a random number, attach it, then try and insert it.. if it fails to insert, it has no fallback functionality.. the code I gave you will KEEP TRYING to insert until it is successful.. a unique index is simple to achieve, you go into your PHPMYADMIN, and add the unique index to that field.. its better to index your fields anyway, makes your queries more efficient.
-
your queries are joining tables together without any direction, its not abnormal to get more than one result of the same, when two tables correlate to eachother, obviously you're gonna PULL TWO RESULTS one from each table how to prevent this, use the ON clause for example SELECT * FROM table1 JOIN table2 ON (table1.id = table2.tableId)
-
You could probably use triggers to catch the insert, check if a "Mike" already exists, and then update the inserting row before it actually is inserted into the database.. But you see, without a trigger, you could PROBABLY use INSERT ON DUPLICATE KEY, but what that does is UPDATES the OLD row.. which means you'd be messing with the old row and TOTALLY IGNORING the new row which SHOULD be inserted.. you could ALSO do it the easiest possible way, like this, but its gonna look like crap for any people that look over your code later <?php $tryingName = 'Mike'; $originalName = 'Mike'; while (1) { $qry = mysql_query("INSERT IGNORE INTO users (name) VALUES('$tryingName')"); if (!mysql_num_rows($qry)) { $tryingName = $originalName . rand(1000,9999); } else { break; } } // there should be a unique row in the database now ?> NOTE: My code above will ONLY work if `name` is a unique index
-
inside the for loop.. throw this in there for($counter = 1;$counter<=$numberofrow;$counter++){ $row_Recordset1 = mysql_fetch_assoc($Recordset1); ?>
-
alert if a Java Script function, so it doesn't have to be defined on the PHP page. And what he said works fine besides the fact that he forgot the ending quote after </script> <?php $Text = "Hello World!"; echo "<script type='text/javascript'>alert('{$Text}');</script>" ?> haha we both messed up, you forgot a ; at the end.. sorry btw @OP I can't really see too well you have to pardon my crappy help <?php $Text = "Hello World!"; echo "<script type='text/javascript'>alert('{$Text}');</script>"; ?>
-
echo "<script type='text/javascript'>alert('{$variabl}');</script>
-
Need help with resizing table column on TinyTable V3
RussellReal replied to geekisthenewsexy's topic in Javascript Help
ok well, I can maybe help you out a lil bit, albeit reading things hurt my eyes atm D: When you build the table headers, you can possibly the easiest way, create a 1px * 1px black dot image, and stretch it 100% of the height of the header, and place one on the right side, and 1 on the left side.. give the image an onclick handler which looks something like this: onclick="stretchy($(this),$(nextOrPrevHeader)" so for example: <thead> <tr> <td id="[color=yellow]id[/color]"><img src="stretchy.gif" onmousedown="stretchy(null),null)">id<img src="stretchy.gif" onmousedown="stretchy($(this),$([color=purple]'#place'[/color]))"></td> <td id="[color=purple]place[/color]"><img src="stretchy.gif" onmousedown="stretchy($(this),$([color=yellow]'#id'[/color]))">place<img src="stretchy.gif" onmousedown="stretchy(null,null)"> </thead> now that you have your headers linked up together left and right you could create the onclick handler to set the two divs (the ones that are next to eachother where your cursor is between) var click1,click2; function stretchy(obj1,obj2) { click1 = objq; click2 = obj2; } function moveHandle() { // do your resizing code here } function unstretchy() { click2 = click1 = null; } $(document).mousemove(moveHandle); // start resizing $('thead img').mouseup(unstretchy); // unset stretchy handler -
RewriteRule ^xxx.php$ abc.html [L]
-
put them ABOVE your rewriteConds AND give them the last flag [L]
-
This will probably be moved to Other Libraries and Frameworks. the reason being, nobody knows what is in the included file.. also even if we did see it, nobody would want to review a whole class of working code, to understand why your 10 lines of broken code doesn't work, they'll be able to help you better there, sorry we can't help you in this category .. I can however, offer you some advice, try forcing a text content-type.. Try error handling. If your page has an error it will usually show you the error, but when you're not in a plain text content-type, you usually can't see the error messages
-
its a warning not a fatal error, the problem isn't with your code, its the fact that when there ARE no results to tally together, for example, you have a user, but that user hasn't earned any rewards.. his rewards won't be present, which means $total_rewards[hisUserId] will not be an array, and when you pass it into the function array_sum, it expects an array, and doesn't get it, to avoid this error message.. Try this: $current_points = @array_sum($total_rewards[$row]) - @array_sum($total_sanctions[$row]);
-
unlink?
-
you're meant to declare the table creation inside of a query, on an active mysql connection. You're #1 trying to create the table before any database connection is established.. #2 you're not creating the table within a query, which is why you're getting a string error, you're telling php something it doesn't expect.. try using exactly what you have. inside of a mysql_query call
-
awstats is pro as far as the problem not sure
-
create a db table, called bank id | user_id | total | last_update make last_update a datetime field, total an integer, user_id integer, and id integer.. set up a cron job to run every minute inside the cron job set it up like: mysql_query("UPDATE bank SET last_update = NOW(), total = total + (total * 0.02) WHERE last_update <= DATE_SUB(NOW(), INTERVAL 20 MINUTE)");
-
How would I check for html or web addresses in a text string?
RussellReal replied to johnsmith153's topic in Regex Help
regular expressions -
select what pikachu's query is selecting
-
uhm, date_add would probably work for you
-
if (preg_match('/^(?:\s*\d*\.?\d{1,2})?\s*$/',$v)) continue; that should work >.>
-
if (preg_match('/^\s*\d*\.?\d{1,2}\s*$/,$v')) continue; change that to if (preg_match('/^\s*\d*\.?\d{1,2}\s*$/',$v)) continue;