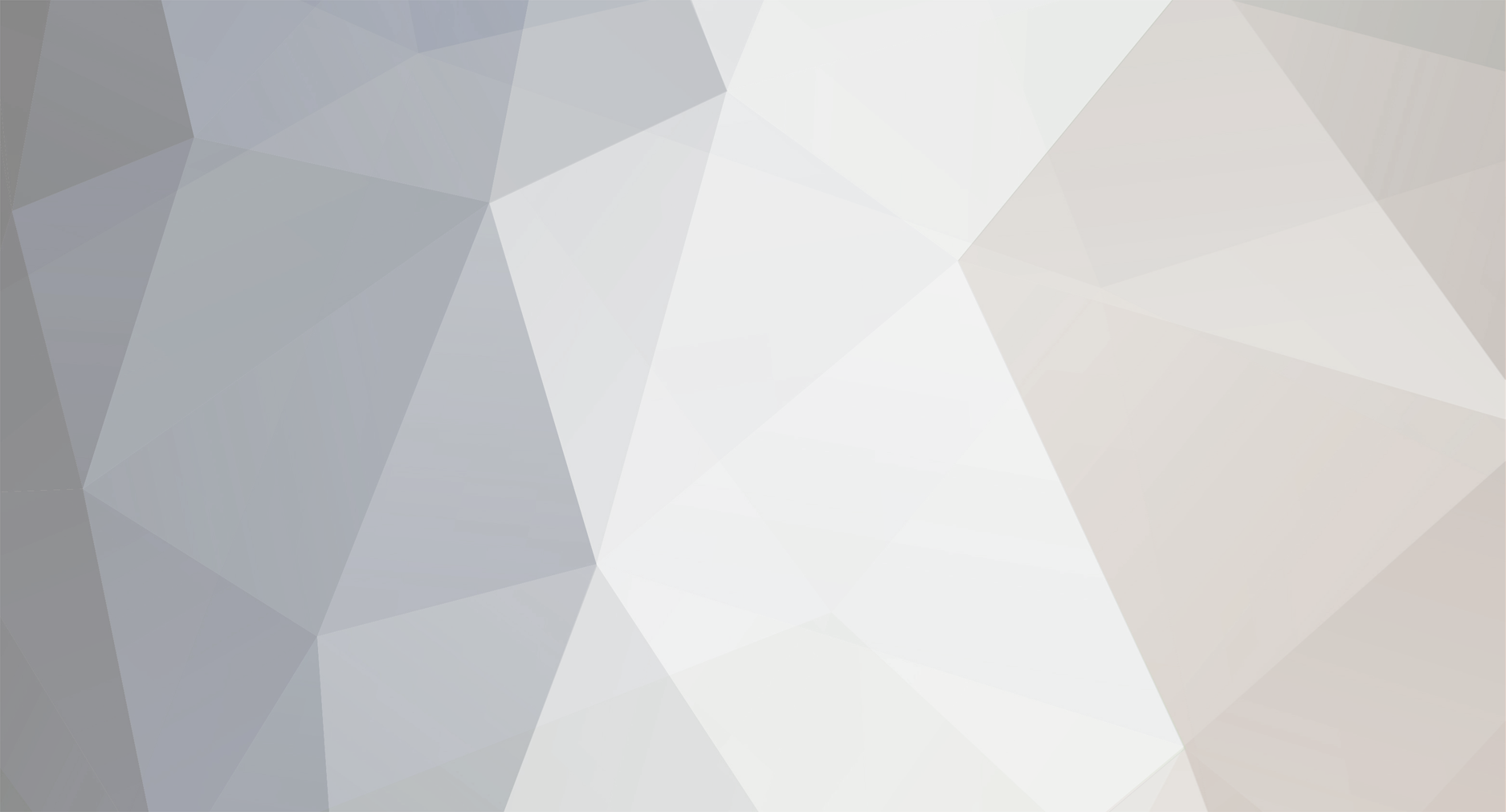
Sudden
-
Posts
22 -
Joined
-
Last visited
Never
Posts posted by Sudden
-
-
Ok...I was thinking storing info in sessions, and then testing it against them later. Which wont work. But your idea seems to be plausable.
-
-
Use the switch function to test what their selection was and display the proper info.
-
Sessions go away after the browser is closed. So it probably wont help.
-
<img src= <?=$image?> />
should probly read
<img src= <?php $image ?> />
-
If the first file contains PHP code it must be saved as .php
-
NP man
Glad to be helpful
-
$ipaddy = $_SERVER['REMOTE_ADDR']; $query = mysql_query("SELECT ip FROM table WHERE ip='$ipaddy'");
Is correct syntax. Tested it.
Just remember your gonna want to test their IP probably before you insert their info into your DB. Just a heads up.
-
NP you just have to have a statment that checks to see if the first form was submitted, and then use a 2nd form to make sure all the data is validated then you can send it off to the next site.
It gets easier the more you use it.
-
Well...I'm not exactly sure what your aiming for, but i'm sure the following link can help you out a little:
http://www.homeandlearn.co.uk/php/php4p11.html
-
You can intergrate the validation page w/ the site in which they input their information.
<?php if(!isset($_POST['validate']){ //checks to see if they have tryed to validate their info ?> //form goes here with action = "<?php $_SERVER['PHP_SELF'] ?>" //fill out info //click validate button returning a value in $_POST['validate'] button HTML below <input type="submit" name="validate" value="Validate"> //website will reload w/ all values that were posted in the form, but the form will not be shown because of the if(!isset($_POST['validate'])) statement. <?php }else{ //all validation code should go here //another form could be displayed to give another submit button after all information checks out ?> <input type="submit" name="submit" value="Submit"> //This form should have the action below so it will post all information in the $_POST[] on the 2nd page <form action="_gdForm/webformmailer.asp" method="post"> <?php } ?>
I hope this helps.
Also there may be an easier way to do this.
-
Set it to insert the data into the correct column then give it the value the user input.
Obviously change vars as needed. And make sure your method for submit is post if you plan on using $_POST[] to assign vars
$input = $_POST['input']; $q = "INSERT INTO db_name (id) VALUES ('$input')"; mysql_query($q);
-
When you hit the submit button it will send all $_POST[] vars to the site specified in:
<form action="_gdForm/webformmailer.asp" method="post">
From there you would just have to call the var
Ex.
$email = $_POST['email'];
Sub email with what the value of your input box was on previous page.
Then just set $email in the email link. All information reguarding $email, and $_POST['email'] should not be able to be viewed with source code.
Hope this helps
-
Also the function:
htmlspecialchars();
might help him handle the ' and ". It reads predefined php characters and turns them into HTML entities.
-
there are sites that wont force adds...but they make you go through a proxy, so people know who supports the site. Nothing is truely free.
-
Yup with php its quite simple...but you will need a website w/ a server that supports it. Make sure you have that before writing the script
-
I'm assuming your questionnaire is a form. So by clicking the submit button you automaticly input all values into an array called
$_POST['value']
If your form method reads:
method="post"
on the page specified in the
action="anwser.php"
The following is a site I made that asks 'do you like sports' and you anwser yes or no. When you hit 'submit' it sends you to another website specified in action="" the page it sends you to automaticly has all the information with-in $_POST[''].
// Input page <form id="form1" name="form1" method="post" action="anwser.php">//page info will be sent to Do you like sports? <table width="200"> <tr> <td><label> <input type="radio" name="sports" value="Yes" /> Yes</label></td> </tr> <tr> <td><label> <input type="radio" name="sports" value="No" /> No</label></td> </tr> </table> <label></label> <label>Submit <input type="submit" name="submit" value="Submit" /> </label> </form>
So say I want to call their input from the last page I simply have to type.
//2nd Page that recieves the info from first page <?php $anwser = $_POST['sports']; //the var $anwser now contains the anwser either Yes/No based on what they gave last page echo $anwser; //will post the anwser on the website ?>
I hope this helps, and there should be no need to make an array with their anwsers in it if you will call
$_POST[];
-
The website states that it extracted it from yahoo finance. So you should probably try going there
-
I'm not sure on an easier way, but I want you to know if you do make the email 'hidden' it will not be seen on the screen, but by viewing the source code you can find the hidden email, if you are only useing:
<input type="hidden" name="email" value= ".$row['field_7'].">
Again hope this helps
-
Try putting "" around .$row['field_7'] and taking out the . after it.
input type="hidden" name="email" value= ".$row['field_7']">
instead of
input type="hidden" name="email" value= .$row['field_7'].>
Not 100% sure this will fix it but it looks like it could be part of your problem.
-
I think you're looking for
stripslashes(string) ;
This removes all "/" added in by php where ' are found.
Ex.
<?php
$str = "Is your name O\'reilly?";
// Outputs: Is your name O'reilly?
echo stripslashes($str);
?>
Hope this helped, but I'm not sure about the r\n\r\n part. Hope someone else out there knows
variables not coming over
in PHP Coding Help
Posted
Try changing:
To:
Not sure if thats the problem tho.