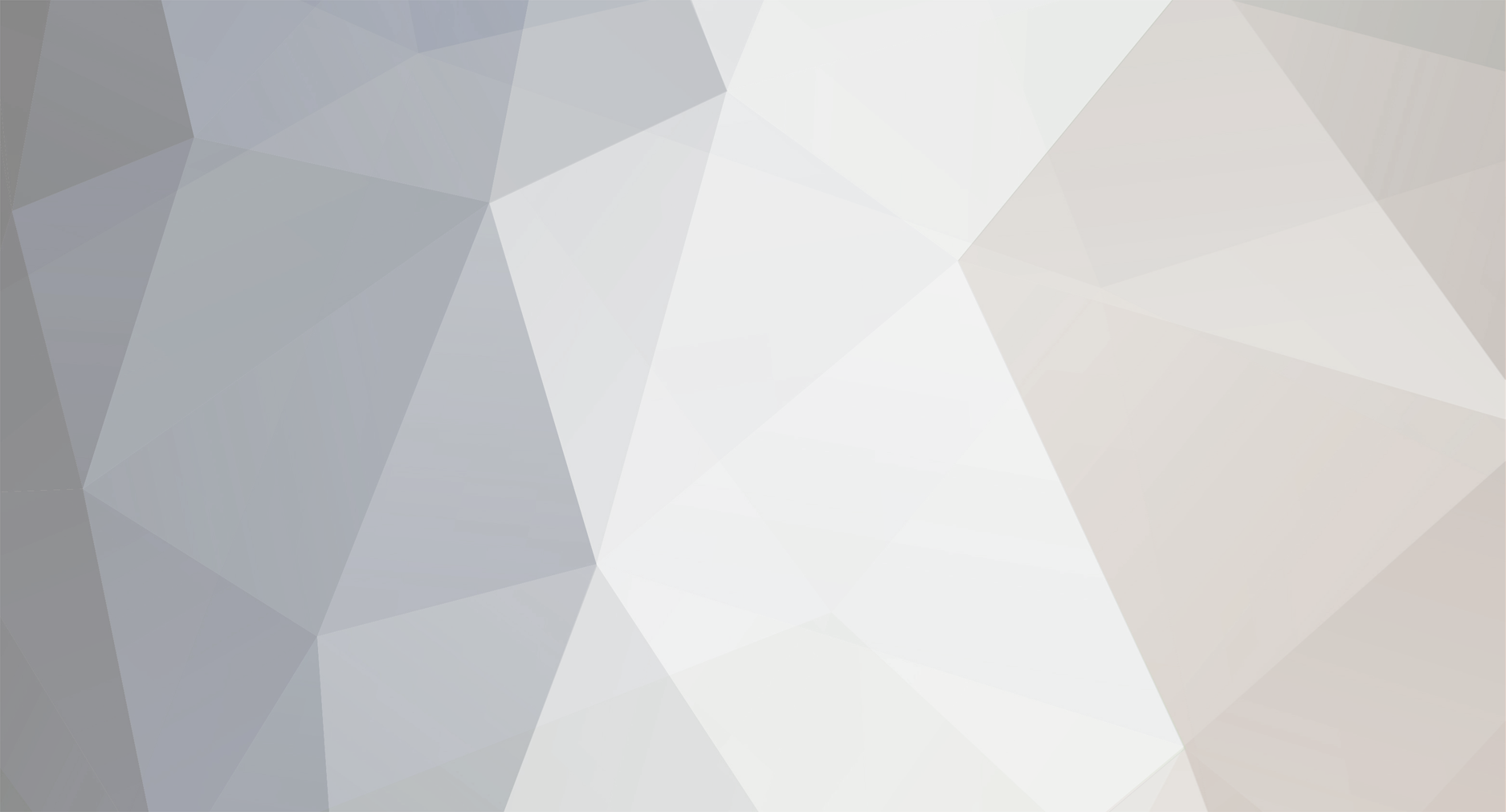
Chidori Soul
-
Posts
20 -
Joined
-
Last visited
Never
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.