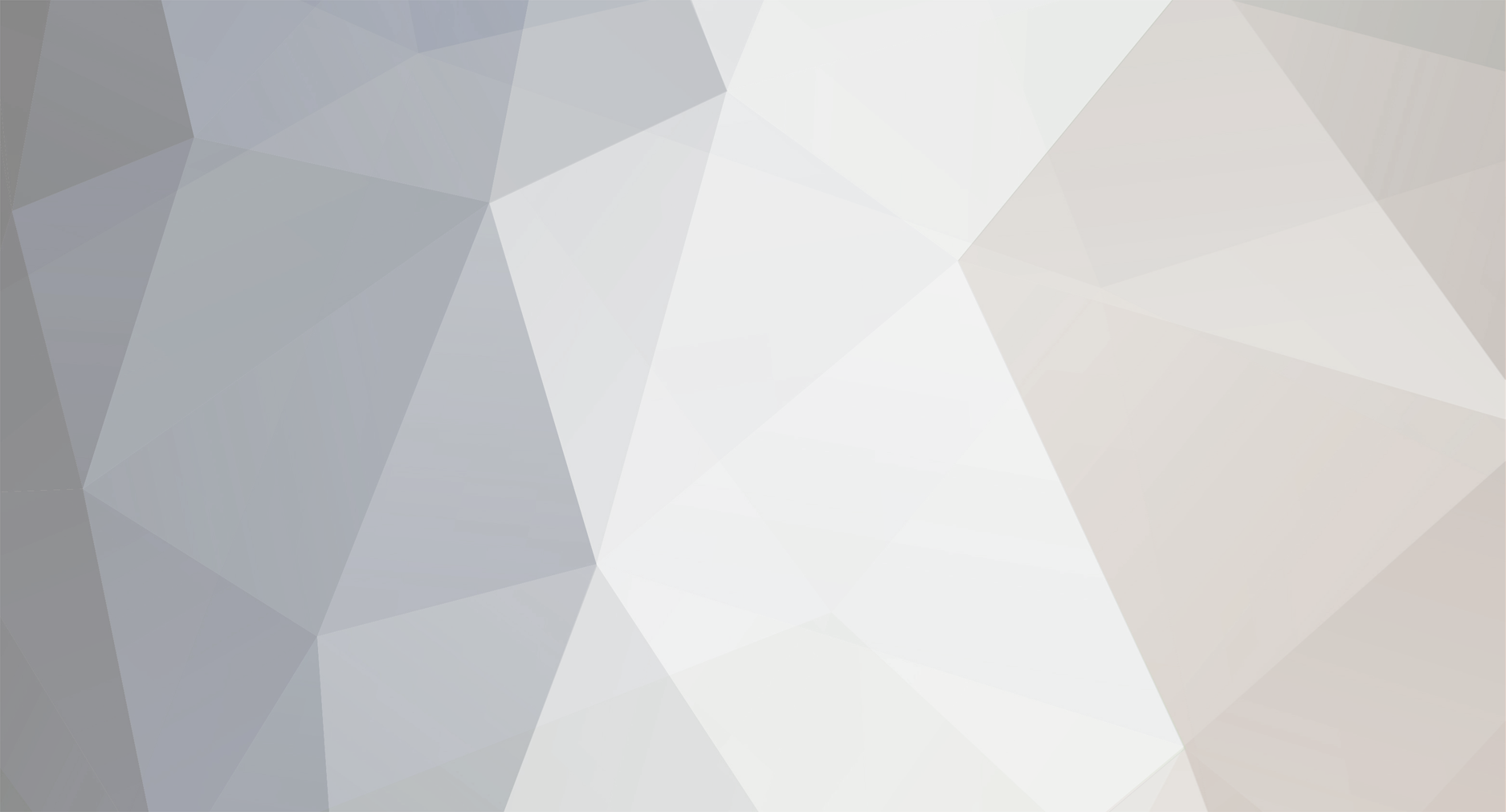
Zyx
Members-
Posts
129 -
Joined
-
Last visited
Everything posted by Zyx
-
Both nginx and Symfony produce logs: nginx: /var/log/nginx/ Symfony: [your project directory]/var/logs/dev.log or prod.log If you're able to run the default page, I think that Nginx works, and the problem is on the framework side. Routing problems are generally reported in Symfony log files and they should give you some clue. For development purpose, use app_dev.php file instead of app.php. In the development mode, Symfony shows detailed information about exceptions directly in the browser, and you have an access to the profiler, too.
-
Moreover, extracting variables from arrays fetched from external sources poses a threat to the security of the application. What if someone puts a key into an array that overwrites an existing variable belonging to your application? You can repack it to use it more conveniently, but always keep these data in some array or object. $json_ary = json_decode($file, true); $weatherInfo = array( 'temperature' => $json_ary['properties']['temperature']['value'], ); echo $weatherInfo['temperature'];
-
Many general-purpose frameworks are in fact optimized for relatively simple CRUD applications. Claiming that a single, hard-coded approach is good for every kind of application is a nonsense. Of course, it is possible to write many different kinds of applications with almost every framework, but possible does not mean that it is well-designed. The problem is that many programmers have no idea about software architectures and they simply don't know that they could solve their problems much easier just by picking a different architecture. The outgrowing problem has the following reasons: - the programmer discovers that he or she has difficulties in writing non-trivial applications, - performance reasons - the framework is not able to handle big traffic or has problems with scalability (i.e. because of the invalid caching) - it is very hard to integrate the framework with third party tools, - the programmer does not agree with some of the design concepts. I know many programmers who decided not to use frameworks, just because they forced them to use Object-Relational Mapping. Many frameworks have a big fanbase, because most of the programmers actually write relatively simple CRUD applications, and they have the tool that perfectly suits their needs. The last thing: being tied to a single framework is not a good thing for programmer, too, especially that the general idea of web frameworks in PHP follows the same architecture and the differences are not too big. You know one, you know all of them.
-
You haven't precised, what area of telecom you are interested in, but for the telecommunications middleware, Erlang is often chosen. It has a certain benefit over C/C++/Java: a usable, predictable, and scalable concurrency model.
-
It's an architectural pattern, or - in other words - a well-tested recipe, how to organize the structure of your application in order to get certain benefits. You can implement it yourself, or use an application framework that does it for you. If you do not know what design and architectural patterns are, you should try to learn something about object-oriented programming and object-oriented design. MVC is an ancestor of the whole family of patterns. In the webdevelopment circles, most articles claiming they describe MVC, actually describe one of its distant descendants.
-
Singletons are evil. Registries are evil. Global variables are evil. Static class variables are evil. Do not use them. EVER. Why? They introduce a global state which is hard to track, hard to test and hard to debug. Once your application gets bigger, you will get lost in the number of dependencies and the way the application works. Every change in component A will destroy component B without no visible reason. If you want to improve it, take a look at the dependency injection container pattern, and the dependency injection idea itself. You do not even have to implement it on your own, because there are existing, standalone implementations, such as the Symfony 2 Dependency Injection Component ( http://components.symfony-project.org )
-
The topic was discussed several times already: http://www.phpfreaks.com/forums/application-frameworks/the-yii-framework/ http://www.phpfreaks.com/forums/application-frameworks/anyone-tried-yii/ And I'll never understand people who still use CodeIgniter... it's like writing websites under DOS in 2010. Have you seen Kohana?
-
Yes, these are the boundary cases. In the if that splits the columns, you must put each tag in one more if: if(! its_the_first_element) { echo '</div>'; } if(! its_the_last_element) { echo '<div ... >'; } You can use the counter to test, whether it is the first or the last element. In addition, to recognize the last element, you must take the total number of results with mysql_num_rows().
-
I haven't heard of such a language, but if there exists any, I'd rather say that you should not look at indoeuropean languages. However, in some languages both forms are commonly used. Polish: "Krok pierwszy", "Krok 1" In other, the word order is different. Slovak: "Prvý krok", "1. krok"
-
Programming is not everything. How about application design, requirement analysis? These are more challenging jobs, and often - better paid. I find building ordinary websites a bit boring too, but on the other hand, I really enjoy designing tools for building them. You could also check it out.
-
Which database system? For MySQL, you have the following construct: http://dev.mysql.com/doc/refman/5.0/en/insert-on-duplicate.html
-
[C] Creating a 2D array of character arrays
Zyx replied to Porl123's topic in Other Programming Languages
Is this array statically or dynamically allocated? Multidimensional, contignuous arrays are in fact one-dimensional arrays with a more complex index calculation and this is how I always implement them: int *array = (int*) malloc(sizeof(int) * size_x * size_y); array[x + y * size_y] = 13; You can write a macro to wrap it and use more easily. String types are also pretty simple: // allocate array memory char **array = (char**) malloc(sizeof(char*) * size_x * size_y); // allocate string memory array[x + y * size_y] = (char*) malloc(sizeof(char) * 30); // write something to the string array[x + y * size_y][2] = 'z'; It will allocate an array of pointers to string arrays. -
Hmmm... wait, I read wrong. You want to put it into columns vertically, not horizontally. However, it's also a simple mathematics. You must count the total number of elements you want to display, divide it by three to estimate, how many you will get within a single column. Then you apply the modulo division every time you display an element: $counter = 0; foreach($elements as $element) { if($counter % $elementNumInAColumn == 0) { echo '</div><div>'; } // display the element here. $counter++; } Boundary cases are boundary cases: the first and the last element. Run the script above, and see what's happening with the tags before the first and after the last element. This is because the script does not handle the boundary cases correctly. I leave it to you as an exercise. You have all the necessary information you need: you know when you reach the first element, and when you reach the last. Make use of this knowledge. PS. Do you really have no clue what modulo division is and how it works?
-
Use the modulo division. It's a simple mathematics: if($counter % 3 == 0) { // break } You just have to remember about the boundary cases.
-
The code in the tutorial does not show any MVC. It's just a piece of code, where some parts were given the names of "model", "view" and "controller" without any noticeable sense and understanding what they actually mean. Remember that naming something "observer" and "observable" does not give us the Observer pattern, if we do not implement the correct interactions between them. The same is with MVC. Most of the current PHP frameworks (and Ruby on Rails that started the game) do not actually implement MVC, but one of its many derivatives, i.e. Model-View-Presenter with Passive View. I was working with MVC for a while, and believe me (or look for the original pattern definitions and check it yourself) - this is a completely different world from what we see. In addition, if you want to learn OOP, I would throw away the outdated CodeIgniter code. I even wonder why people are still using this framework, if there are plenty of much better alternatives. Take a look at Symfony 2, which aims to set a completely new quality among the frameworks: https://github.com/symfony/symfony . You can also look at my experimental framework, where I make MVC experiments: https://github.com/zyxist/Trinity . First of all, try to understand the concepts behind them, and their structure. Writing a framework is not easy, at least if you want it to be usable .
-
PEAR 1.x installer is a bit messy. The C:\PHP directory is probably a rubbish from some previous installations. I'd recommend switching to the installation directory with "cd" and then executing "go-pear.phar", because otherwise there could be a problem with working directory paths.
-
The general OOP rules are universal. If you have an experience with Java, you should already know, how to design an object-oriented application in PHP. In addition, many features look exactly the same, so there should be even less confusion. The most important difference are namespaces, and class autoloading, but they are not directly related to the OOP design. Another useful PHP-specific thing that might be helpful can be found here: - http://groups.google.com/group/php-standards/web/psr-0-final-proposal
-
PHP manual is your friend: http://docs.php.net/strtr - strtr() replaces single characters, not substrings. I guess you want to use str_replace().
-
I've been using Yii for a while. Good sides: - Contrary to most developers, Yii authors actually looked at MVC description and implemented something that tries to follow the goals of this architectural patterns. Most of all, they do understand that views should have a direct reference to model, not to obtain all the data through controllers. - As a consequence, in this framework the view- and model-specific code does not have to be placed in controllers. - Good l10n subsystem - The framework is self-contained and provides everything you should need. Bad sides: - The hierarchical module system. Although it is well-implemented, the general idea has some limitations. For example you cannot divide the website into "frontend" and "backend" and have modules that contribute to both of these areas at the same time. - Completely non-standard class naming style. - The framework is good for typical web applications, but for those ones which have very specific needs, it can fail. I tried to develop a modular CMS in it and unfortunately I stuck at the default controller implementation that did not suit the general idea of the project at all, and furthermore - it was so hard-coded that it could not be replaced.
-
Best way to organize namespaced classes in the filesystem? (autoload question)
Zyx replied to mkz's topic in PHP Coding Help
This is what you need: http://groups.google.com/group/php-standards/web/psr-0-final-proposal?pli=1 . If you implement this class naming standard and file organization, you will be able to use any third party autoloader that supports it. There is absolutely no need to scan the application directory first, and then the system's one for several reasons. Autoloaders should never use file_exists() function, because it is not aware of the include_path setting and can report that a file does not exist, even if it exists in fact and can be loaded with require(). The better (and faster) way is to extract the first part of the namespace and check what path is associated to it. Your application and system files simply lie in different namespaces and if you want to overwrite one of the system components, you should do this by overwriting the service that creates it or through the configuration. -
If you have some knowledge about OOP and understand such terms, as "design patterns" and what they are used for, you can give them a try. In fact, it is possible they could learn you some good OOP design techniques.
-
Framework is a website building toolset. You can look at it as a collection of ready-to-use code, design practices and project structure, which you build your website on. Here is a classic example: website is usually divided into separate subpages. The framework gives you a structure to organize your subpages and the code that manages it. If you want to add a navigation to it, you get a navigation component and configure it, and so on. You do not have to write or invent it on your own. It's not a CMS, which is actually a complete web application, where you simply point and click to make a website. Frameworks can be used to write CMS. The question whether to learn it or not depends on your current skills. Frameworks use object-oriented programming techniques, so if you do not know it, you have to learn at least the basics, and some general programming knowledge is also recommended. In PHP it is a small problem, because it's often the first programming language for many people, and to be honest - a complete programming newbie usually has problems with understanding the whole concept. What to use: many people recommend here CodeIgniter, which is something I don't understand; in my country this framework is almost completely forgotten because it uses outdated technologies (PHP4). Instead they often choose its clone, Kohana, rewritten to PHP5. There is also Symfony, ZF, and more rarely - CakePHP.
-
PHP large file uploads: memory_limit ? max_execution_time?
Zyx replied to akshay's topic in PHP Coding Help
The execution of the script starts when the file is already uploaded. The web server handles the entire process and stores the uploaded file in a temporary directory, not in memory. You do not have to touch any of these PHP settings, but just the maximum size of the POST upload. -
MrAdams -> I didn't say that PHPTAL syntax is somehow worse than PHP one. I agree that it is much cleaner than pure PHP. ProjectFear -> for example because well-designed template engines don't do "just a foreach", but can hide many implementation details, optimize it much better (they do not need to worry about the readability of the output code). Consider a nested foreach - in PHP it's you who must built a relationship between them from scratch and you must know, how such a relationship is represented in the template input. If there is a change in a script that requires rewriting it, you end up with lots of templates to fix. On the other hand, the template language can hide everything and you just select a requested way before the template compilation. Something changes - you change one line. You are starting another project? Copy your templates and change the configuration. This is a real situation I encountered. I was using Doctrine and pure PHP templates. After a while it turned out that I don't need to hydrate the data to objects in three or four places, and I can use faster arrays. There was just one problem: I have already implemented three or four quite complex templates (and a bit problematic to read) that worked on these objects and I had to rewrite all the calls to arrays. This is why I think that having two template languages to choose: PHP and some XML-based that can deal with such issues automatically, I would always choose it, not PHP.
-
I think it is a good alternative. It is one of those rare template engines which at least try do to something interesting with the template language, not "reinvent" a small and limiting subset of PHP. I don't agree. Smarty syntax is a limited subset of PHP and esoteric mess with lots of parts broken because they didn't know how to do it better. Contrary to it, TAL language follows well-known XML-rules, and all the functional parts are just XML attributes. Furthermore, it can validate your HTML template syntax which is not possible with Smarty and PHP. Don't listen this stuff about "learning curve". Pure PHP is worthless for templating, and this is why framework developers put tones of complex template helpers to it. Don't they need to be learned, too? Especially, if part of them uses massive object-oriented programming techniques and the same things in different helpers are incompatible one with another? Don't also forget that the learning curve can benefit you in the future - you need some time to learn new techniques, but it can turn out that using them is faster, simpler and cheaper than writing the same thing in pure PHP. Of course it depends on a template language. I think that if you need to do some simple pages, PHP with helpers will be all right. If you have complex needs, or simply want to deal with a different template language, try the modern approaches that offer you more than "just a limited subset of PHP", like Smarty, Dwoo, and 90% of template engines do.