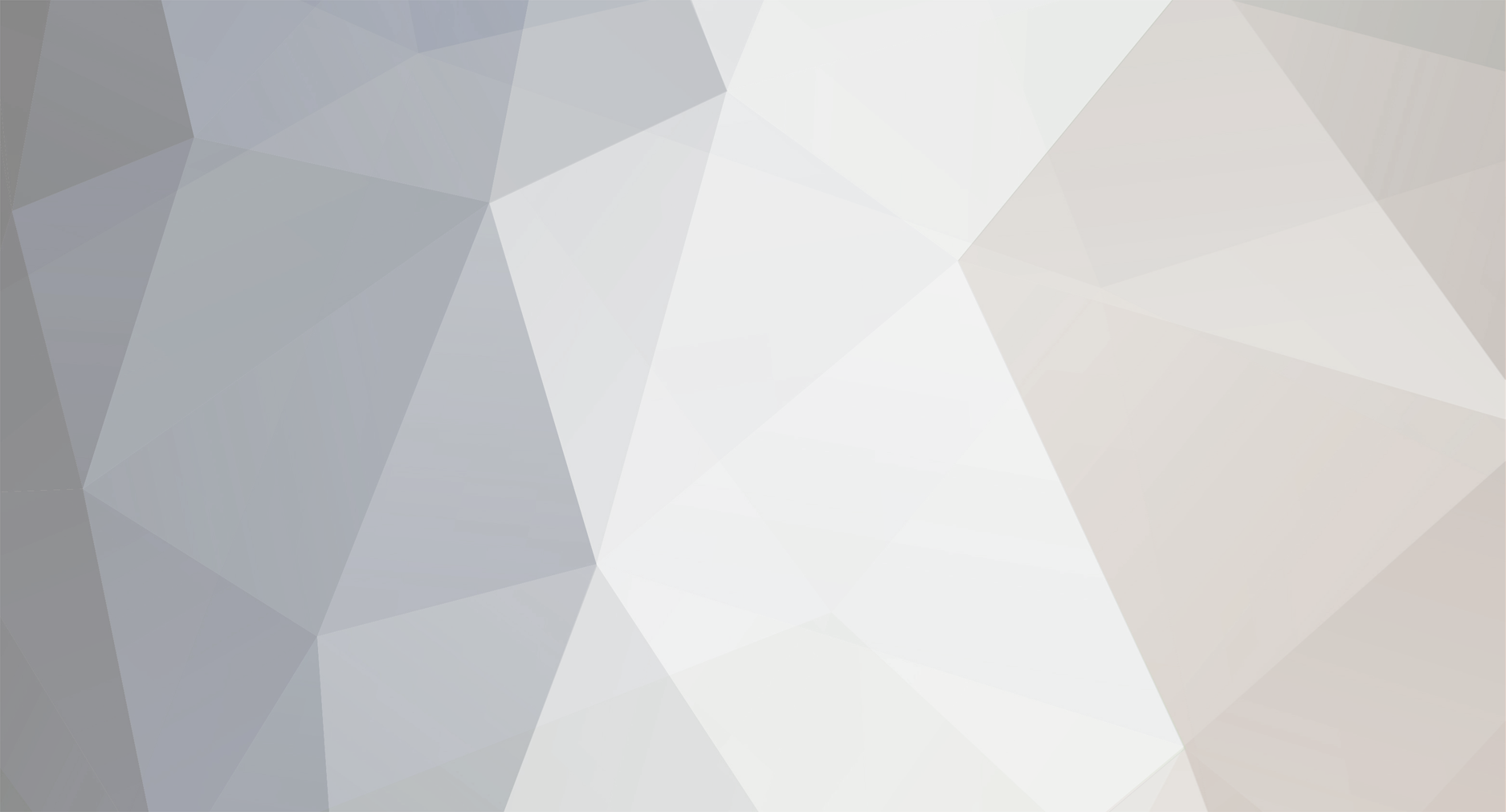
petroz
-
Posts
180 -
Joined
-
Last visited
Never
petroz's Achievements

Member (2/5)
0
Reputation
petroz has no recent activity to show
0
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.