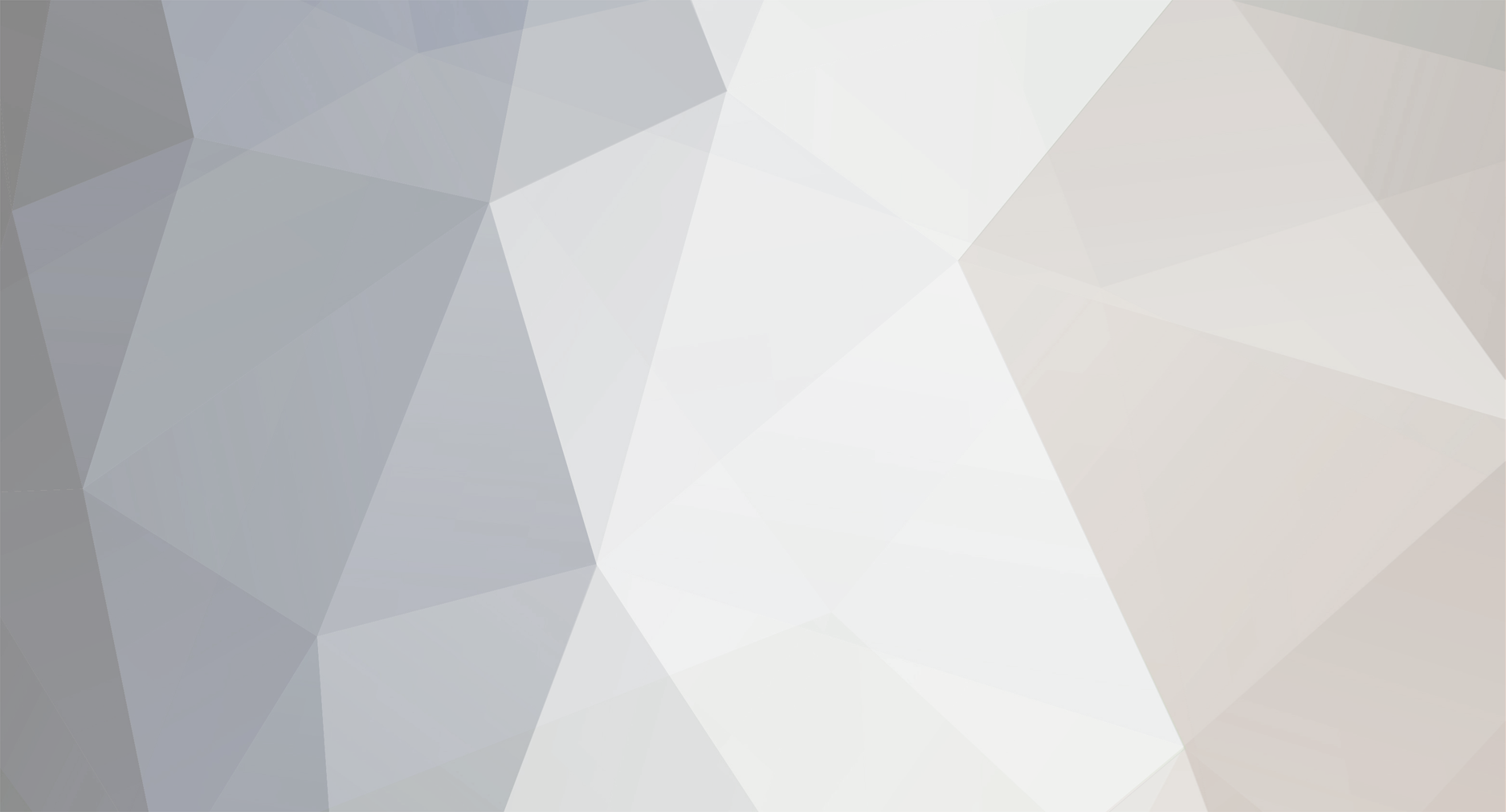
3raser
Members-
Posts
815 -
Joined
-
Last visited
Everything posted by 3raser
-
Thanks for the reply. I put the large bit of JavaScript in an external file. Also, about the "deletepost.php" thing. I was thinking of doing something like that, but isn't one line with the required data already there better than creating a whole new file? //for administrators to delete posts if(isset($_GET['delete'])) $post->deletePost($_GET['delete'], $rank); vs. deletepost.php <?php require('../../includes/config.php'); require('../../structure/database.php'); require('../../structure/forum.post.php'); require('../../structure/base.php'); require('../../structure/user.php'); $database = new database($db_host, $db_name, $db_user, $db_password); $post = new post($database); $base = new base($database); $user = new user($database); $rank = $user->getRank($user->getUsername($_COOKIE['user'], 2)); if($rank > 2) { $post->deletePost($_GET['id'], $rank); } $base->redirect('viewthread.php?forum='. $_GET['forum'] .'&id='. $_GET['id']); ?>
-
I really wish you could be more specific.
-
I know this isn't the neatest code in the world, but I'm really trying my best to make the neatest code possible. If you have spare time, can you give me feedback & suggestions regarding making this code at least average when it comes to being neat? Thanks. viewthread.php (for my forum) <?php require('../includes/config.php'); require('../structure/base.php'); require('../structure/forum.php'); require('../structure/forum.thread.php'); require('../structure/forum.post.php'); require('../structure/database.php'); require('../structure/user.php'); $database = new database($db_host, $db_name, $db_user, $db_password); $base = new base($database); $user = new user($database); $forum = new forum($database); $thread = new thread($database); $post = new post($database); //set some variables that are used a lot throughout the page $username = $user->getUsername($_COOKIE['user'], 2); $rank = $user->getRank($username); $f = $_GET['forum']; $i = $_GET['id']; //preform basic checks if(!$thread->checkExistence($i)) $base->redirect('index.php'); if(!$thread->canView($i, $username, $rank)) $base->redirect('index.php'); //if the GOTO field is set, let's skip to the selected post if(ctype_digit($_GET['goto'])) { $getPageNum = $thread->getPageNum($_GET['goto'], $i); if($getPageNum) $base->redirect('viewthread.php?forum='. $f.'&id='.$i.'&page='.$getPageNum.'&highlight='. $_GET['goto'] .'#'.$_GET['goto']); } //if a moderator chooses to hide, lets make it happen if((isset($_GET['hide']) && !isset($_GET['pid'])) && $rank > 2) $thread->hideThread($i, $rank); if((isset($_GET['hide']) && isset($_GET['pid'])) && $rank > 2) $post->hidePost($_GET['pid'], $rank); //for administrators to delete posts if(isset($_GET['delete'])) $post->deletePost($_GET['delete'], $rank); //extract thread details $detail_query = $database->processQuery("SELECT `id`,`lock`,`sticky`,`title`,`username`,`status`,`content`,`date`,`lastedit`,`qfc` FROM `threads` WHERE `id` = ? LIMIT 1", array($i), true); //assign data to details[] array $details[] = $detail_query[0]['lock']; $details[] = $detail_query[0]['sticky']; $details[] = htmlentities($detail_query[0]['title'], ENT_NOQUOTES); $details[] = $detail_query[0]['username']; $details[] = $detail_query[0]['status']; $details[] = $detail_query[0]['content']; $details[] = $detail_query[0]['date']; $details[] = $detail_query[0]['lastedit']; $details[] = $detail_query[0]['qfc']; //get any extra icons if($details[1] == 1) $extra .= '<img src="../img/forum/sticky.gif"> '; if($details[0] == 1) $extra .= ' <img src="../img/forum/locked.gif">'; //check if the POST has been edited, then adjust the $date variable accordingly if(empty($details[7])) { $date = $details[6]; } else { //get USERNAME:DATE/TIME $edit_details = explode('@', $details[7]); $date = $details[6].'<br/>Last edit on '. $edit_details[1] .' by '. $edit_details[0]; } //get forum details $forum_details = $database->processQuery("SELECT `title` FROM `forums` WHERE `id` = ?", array($f), true); //pagination $per_page = 10; //get # of pages $database->processQuery("SELECT * FROM `posts` WHERE `thread` = ?", array($i), false); $pages = ($database->getRowCount() == 0) ? 1 : ceil($database->getRowCount() / $per_page); //get current page (!ctype_digit($_GET['page']) || $_GET['page'] > $pages) ? $page = 1 : $page = $_GET['page']; //get next link ($page < $pages) ? $next = $page+1 : $next = $page; //get prev link (($page-1) >= 1) ? $prev = ($page-1) : $prev = $page; //start $start = ($page == 1) ? 1 : ($page-1)*$per_page; ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html xmlns:IE> <head> <meta http-equiv="Expires" content="0"> <meta http-equiv="Pragma" content="no-cache"> <meta http-equiv="Cache-Control" content="no-cache"> <meta name="MSSmartTagsPreventParsing" content="TRUE"> <title><?php echo $data['wb_title']; ?></title> <link href="../css/basic-3.css" rel="stylesheet" type="text/css" media="all" /> <link href="../css/forum-3.css" rel="stylesheet" type="text/css" media="all" /> <link href="../css/forummsg-1.css" rel="stylesheet" type="text/css" media="all" /> <style> </style> <script language="JavaScript"> document.getElementById("smilytxt").style.display=""; function addsmiley(code) { var msgtext=document.getElementById("charlimit_text_a"); msgtext.focus(); if (document.selection && document.selection.createRange && !msgtext.setSelection) { document.selection.createRange().text=code; } else { var pretext = msgtext.value.substring(0,msgtext.selectionStart); var pos = msgtext.selectionStart; var posttext = msgtext.value.substring(msgtext.selectionEnd, msgtext.value.length); msgtext.value = pretext + code + posttext; msgtext.selectionEnd=pos+code.length; } } </script> <script type="text/javascript"> var alerted=false; function do_watch(msg, element, count, max, submit) { try { var stri=element.value.replace(/\r/g, ""); if(submit) if(stri.length>max) submit.disabled=true; if(stri.length>max) { if(msg==true && alerted==false) { alert('You have gone over your character limit for this message'); alerted=true; } element.value=stri=stri.substring(0,max); } count.childNodes[0].nodeValue=max-stri.length; } catch(e) {} } function install_watch(msg, element, count, max, form, submit, reset) { try { element.onkeyup=function() { do_watch(msg, element, count, max, submit); }; element.onkeydown=function() { do_watch(msg, element, count, max, submit); }; element.onkeypress=function() { do_watch(msg, element, count, max, submit); }; element.onmousemove=function() { do_watch(msg, element, count, max, submit); }; element.onchange=function() { do_watch(false, element, count, max, submit); }; if(form) { form.onsubmit=function() { do_watch(msg, element, count, max, submit); }; } if(reset && form) { reset.onclick=function() { form.reset(); do_watch(msg, element, count, max, submit); } } do_watch(false, element, count, max, submit); } catch(e) {} } var charlimiter_run=false; function install_charlimiters() { if(charlimiter_run) return; charlimiter_run=true; try { var textboxes=document.getElementsByTagName("textarea"); for(var i=0; i<textboxes.length; i++) install(textboxes[i]); var inputs=document.getElementsByTagName("input"); for(var i=0; i<inputs.length; i++) install(inputs[i]); } catch(e) {} } function install(element) { var textbox_id_len = new String("charlimit_text").length; var text_id=element.id.toString(); if(text_id.match(/^charlimit_text/i) && text_id.length>=textbox_id_len) { var identifier=text_id.substr(textbox_id_len); var info=document.getElementById("charlimit_info" + identifier); var count=document.getElementById("charlimit_count" + identifier); var form=document.getElementById("charlimit_form" + identifier); var submit=document.getElementById("charlimit_submit" + identifier); var reset=document.getElementById("charlimit_reset" + identifier); if(info && count) { var msg=false; if(identifier.match(/^_msg/i)) msg=true; var max_val=parseInt(count.childNodes[0].nodeValue); install_watch(msg, element, count, max_val, form, submit, reset); info.style.display='inline'; } } } if(window.addEventListener) window.addEventListener('load', install_charlimiters, true); else if(window.attachEvent) window.attachEvent('onload', install_charlimiters); else window.onload=install_charlimiters; </script> <!--[if IE 8]> <link rel="stylesheet" type="text/css" href="../css/forummsg-ie-1.css" /> <![endif]--> </head> <body> <div id="body"> <div class="frame e"> <span style="float: right;"> <?php if($user->isLoggedIn()) { if($rank > 3) { echo '<a href="../index.php">Main Page</a> | <a href="acp.php">Forum ACP</a> | <a href="../logout.php">Logout</a>'; } else { echo '<a href="../index.php">Main Page</a> | <a href="../logout.php">Logout</a>'; } } else { echo '<a href="../index.php">Main Page</a> | <a href="../login.php">Login</a>'; } ?> </span> <div> <?php if($user->isLoggedIn()) { echo 'You are logged in as <span style="color: rgb(255, 187, 34);">'. $username .'</span>'; } else { echo 'You are not logged in.'; } ?> </div> </div><br /><br /> <div id="picker"> <form method="post" action="jump.php"> <ul class="flat"> <!--<li><a href=""><img src="../img/forums/search_threads.gif"> Search threads</a></li>--> <li>Jump to Thread: <input size="8" name="qfc" maxlength="14" /></li> <li><input class="b" name="jump" value="Go" type="submit" /></li> </ul> </form> </div> <div id="infopane"> <span class="title"><?php echo $extra.' '.$details[2]; ?></span> <div class="about"> <ul class="flat"> <!--<li><a href="#"><img src="../img/forum/code_of_conduct.gif" /> Code of Conduct</a></li>--> </ul> </div> </div> <!--<div id="nocontrols" class="phold"></div>--> <br> <div id="breadcrumb"> <a href="../index.php">Home</a> > <a href="index.php"><?php echo $data['wb_name']; ?></a> > <a href="viewforum.php?forum=<?php echo $f; ?>"><?php echo $forum_details[0]['title']; ?></a> > <a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>"><?php echo $details[2]; ?></a> </div> <div class="actions" id="top"> <table> <tbody> <tr> <td class="nav"> <form action="viewthread.php" method="get" style="display: inline;"> <input type="hidden" name="id" value="<?php echo $i; ?>" /> <input type="hidden" name="forum" value="<?php echo $f; ?>" /> <ul class="flat"> <li><a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>"><< first</a></li> <li><a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>&page=<?php echo $prev; ?>">< prev</a></li> <li>Page <input type="text" class="textinput" id="page" name="page" value="<?php echo $page; ?>" size="2" maxlength="3" /> of <?php echo $pages; ?></li> <li><a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>&page=<?php echo $next; ?>">next ></a></li> <li><a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>&page=<?php echo $pages; ?>">last >></a></li> </ul> </form> </td> <td class="commands"> <ul class="flat"> <?php if($thread->canReply($i, $rank) && !$user->checkMute($username)) echo '<li><a href="reply.php?forum='. $f .'&id='. $i .'"><img src="../img/forum/new_thread.gif" alt="T" /> Reply</a></li>'; ?> <li><a href=""><img src="../img/forum/refresh.gif" alt=""> Refresh</a></li> </ul> </td> </tr> </tbody> </table> </div> <!--<form action="javascript://" method="post">--> <div id="contentmsg"> <a name="188090"></a> <?php //set the action bar $action_bar = (($rank < 3 && $details[4] == 0 && $details[0] == 0) || $rank > 2) ? $thread->getActionBar($rank, $i, $f) : null; //only display thread on page 1 if($page == 1) { if($details[4] == 1 && $rank < 3) { ?> <table class="<?php echo $thread->getPostType($details[4], $details[3], ($_GET['goto'] == 'start') ? true : false); ?>"> <tbody> <tr> <td class="leftpanel"> <div class="msgcreator uname"> </div> <div class="modtype"> </div> <div class="msgcommands"> </div> </td> <td class="rightpanel"> <div class="msgtime"><br/></div> <div class="msgcontents"> <i>The contents of this message is hidden</i> </div> <span style="float:right; margin-right: 5px; margin-bottom: 5px; margin-top: -20px;"><?php if($rank > 2) echo $action_bar; ?></span> </td> </tr> </tbody> </table> <?php } else { ?> <table class="<?php echo $thread->getPostType($details[4], $details[3], ($_GET['goto'] == 'start') ? true : false); ?>"> <tbody> <tr> <td class="leftpanel"> <div class="msgcreator uname"><?php echo $user->dName($details[3]); ?></div> <div class="modtype"><?php echo $forum->getPostTitle($details[3]); ?> <div class="msgcommands"></div> </td> <td class="rightpanel"> <div class="msgtime"><?php echo $date; ?></div> <div class="msgcontents"> <?php echo $base->br2nl($thread->formatPost($details[5], $details[3])); ?> </div> <span style="float:right; margin-right: 5px; margin-bottom: 5px; margin-top: -20px;"><?php echo ($details[3] == $username && $rank < 3 && $details[4] == 0 && $details[0] == 0) ? '<a href="edit.php?forum='. $f .'&id='. $i .'&type=2">Edit</a> | '.$action_bar : $action_bar; ?></span> </td> </tr> </tbody> </table> <?php } } //display posts $replies = $database->processQuery("SELECT `id`,`username`,`content`,`lastedit`,`date`,`status` FROM `posts` WHERE `thread` = ? ORDER BY `id` ASC LIMIT $start,$per_page", array($i), true); foreach($replies as $reply) { //set the user's action bar $action_bar = (($rank < 3 && $details[4] == 0 && $details[0] == 0) || $rank > 2) ? $post->getActionBar($rank, $reply['id'], $i, $f) : null; //check if the POST has been edited, then adjust the $date variable accordingly if(empty($reply['lastedit'])) { $date = $reply['date']; } else { //get USERNAME:DATE/TIME $edit_details = explode('@', $details[7]); $date = $reply['date'].'<br/>Last edit on '. $edit_details[1] .' by '. $edit_details[0]; } if($reply['status'] == 1 && $rank < 3) { ?> <a name="<?php echo $reply['id']; ?>"></a> <table class="<?php echo $thread->getPostType($reply['status'], $reply['username'], ($_GET['highlight'] == $reply['id']) ? true : false); ?>"> <tbody> <tr> <td class="leftpanel"> <div class="msgcreator uname"> </div> <div class="modtype"> </div> <div class="msgcommands"> </div> </td> <td class="rightpanel"> <div class="msgtime"><br/></div> <div class="msgcontents"> <i>The contents of this message is hidden</i> </div> <span style="float:right; margin-right: 5px; margin-bottom: 5px; margin-top: -20px;"><?php if($rank > 2) echo $action_bar; ?></span> </td> </tr> </tbody> </table> <?php } else { ?> <a name="<?php echo $reply['id']; ?>"></a> <table class="<?php echo $thread->getPostType($reply['status'], $reply['username'], ($_GET['highlight'] == $reply['id']) ? true : false); ?>"> <tbody> <tr> <td class="leftpanel"> <div class="msgcreator uname"><?php echo $user->dName($reply['username']).' ['. $reply['id'] .']'; ?></div> <div class="modtype"><?php echo $forum->getPostTitle($reply['username']); ?></div> <div class="msgcommands"></div> </td> <td class="rightpanel"> <div class="msgtime"><?php echo $date; ?></div> <div class="msgcontents"> <?php echo $base->br2nl($thread->formatPost($reply['content'], $reply['username'])); ?> </div> <span style="float:right; margin-right: 5px; margin-bottom: 5px; margin-top: -20px;"><?php echo ($reply['username'] == $username && $rank < 3 && $details[4] == 0 && $details[0] == 0) ? '<a href="edit.php?forum='. $f .'&id='. $i .'&type=1&pid='. $reply['id'] .'">Edit</a> | '.$action_bar : $action_bar; ?></span> </td> </tr> </tbody> </table> <?php } } ?> </div> <!-- --> <div id="breadcrumb"> <a href="../index.php">Home</a> > <a href="index.php"><?php echo $data['wb_name']; ?></a> > <a href="viewforum.php?forum=<?php echo $f; ?>"><?php echo $forum_details[0]['title']; ?></a> > <a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>"><?php echo $details[2]; ?></a> </div> <div class="actions" id="top"> <table> <tbody> <tr> <td class="nav"> <form action="viewthread.php" method="get" style="display: inline;"> <input type="hidden" name="id" value="<?php echo $i; ?>" /> <input type="hidden" name="forum" value="<?php echo $f; ?>" /> <ul class="flat"> <li><a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>"><< first</a></li> <li><a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>&page=<?php echo $prev; ?>">< prev</a></li> <li>Page <input type="text" class="textinput" id="page" name="page" value="<?php echo $page; ?>" size="2" maxlength="3" /> of <?php echo $pages; ?></li> <li><a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>&page=<?php echo $next; ?>">next ></a></li> <li><a href="viewthread.php?forum=<?php echo $f.'&id='.$i; ?>&page=<?php echo $pages; ?>">last >></a></li> </ul> </form> </td> <td class="commands"> <ul class="flat"> <?php if($thread->canReply($i, $rank) && !$user->checkMute($username)) echo '<li><a href="reply.php?forum='. $f .'&id='. $i .'"><img src="../img/forum/new_thread.gif" alt="T" /> Reply</a></li>'; ?> <li><a href=""><img src="../img/forum/refresh.gif" alt=""> Refresh</a></li> </ul> </td> </tr> </tbody> </table> </div> <div id="uid">Quick find code: <?php echo $details[8]; ?></div> <br> <div class="tandc"><?php echo $data['wb_foot']; ?></div> </div> </body> </html>
-
public function br2nl($string) { return str_replace('<br />', "\n", $string); } Eh, gets rid of the <br /> - But no new lines. I'm assuming I need to use something other than \n?
-
Using your test: Before: %3Cbr+%2F%3E After: %0A Like I said, when using the br2nl function - nothing seems to change. The <br /> stays the same. <?php require('../structure/base.php'); $base = new base(); if(!isset($_POST['derp'])) { ?> <form action="test.php" method="POST"> <textarea cols="55" rows="30" name="derp"></textarea><br/><input type="submit" value="Derp"> </form> <?php } else { $content = nl2br($_POST['derp']); echo $base->br2nl(htmlentities($content)).'<br/><hr><br/>'.$content; } ?>
-
No differences from what I can tell. It remains: fdsfdsfdsfdsf<br /> <br /> <br /> <br /> <br /> <br /> <br /> fdsfdsfdsfds<br /> <br /> <br /> <br /> <br /> <br /> dfdsfdsfdsf<br /> <br /> <b>wut</b>
-
Highly annoying. Data is submitted to the database wrapped in the nl2br() function. When viewing the data, htmlentities is used to keep away any XSS or HTML vulnerabilities. But all new lines show up as <br /> instead of a new line. I even made a function to stop them from happening: public function br2nl($string) { return str_replace('<br />', "\n", $string); } Which doesn't work. Any advice?
-
Ah, thank you. And yeah, thanks for pointing that out. Was a small bug.
-
I want to ensure that the data received is set. Is there any other way I can shorten this condition? I was thinking of a for loop, but I'm not sure that'd be considered more efficient. if((!ctype_digit($_GET['forum']) && !ctype_digit($_POST['forum'])) || (!ctype_digit($_GET['id']) && !ctype_digit($_POST['id'])) || (!ctype_digit($_GET['type']) || !ctype_digit($_POST['type']))) $base->redirect('index.php');
-
I'm making a cookie tester. I'm generating a random hash, and then I want to go to the website and test it out. Is it possible to edit the cookiefile format? I mean the little bit after session_hash - I want to change the old hash to the newly generated one. # Netscape HTTP Cookie File # http://www.netscape.com/newsref/std/cookie_spec.html # This file was generated by libcurl! Edit at your own risk. .urlhiddd.com TRUE / FALSE 1340769586 session_hash 0023353ce95b5f89bd11e68757426a41cbd8a028
-
I still can't seem to figure out why this code doesn't work. I tested it on a basic string: '<br/>test<br/>test<br/>', and it worked properly. public function br2nl($string) { return str_replace('<br />', "\n", $string); //return preg_replace('#<br(| )\/>#', "\n", $string); } Here is where it's used: <?php echo $base->br2nl($thread->formatPost($details[6], $details[4])); ?> Format post: public function formatPost($content, $username) { //get the rank of the user $user = $this->database->processQuery("SELECT `acc_status` FROM `users` WHERE `username` = ? LIMIT 1", array($username), true); //remove HTML from all posts besides administrators if($user[0]['acc_status'] < 4) $content = htmlentities($content, ENT_NOQUOTES); //now let's do BBCode for mods and admins if($user[0]['acc_status'] > 2) { $bbcode = array('#\[b\](.+?)\[\/b\]#', '#\[i\](.+?)\[\/i\]#'); $replace = array('<b>$1</b>', '<i>$1</i>'); $content = preg_replace($bbcode, $replace, $content); if($user[0]['acc_status'] == 4) { //convert QUOTE BBcode to actual HTML format $content = preg_replace('/\[quote\=(.+?)](.+?)\[\/quote\]/s', '<div style="border:1px solid #957C07;margin: 14px 0 0"> <p style="background:#645305;margin:0;padding:2px;font-style:normal"> <strong>Original Content</strong> (Posted by: $1) </p> <div style="position:relative;float:right;overflow:hidden;height:33px;top:-28px;left:10px"> <span style="color:#957C07;font-family:Engravers MT,Felix Titling,Perpetua Titling MT,Times New Roman;font-style:normal;font-size:120px;line-height:81px">"</span> </div> <div style="font-style:italic;margin:8px 6px">$2 </div> </div>', $content); } } return stripslashes($content); }
-
Thanks for the quick tip about the Regex stuff. Very useful. Firstly, whether or not you use the brackets is personal choice, though you certainly need them if you want to execute more than one line of code inside the condition. but as a side note, your ternary is kinda funky. The ternary would be cleaner like so: $icon = ($s_forum['type'] == 4) ? '' : ''; But on that note... you are also assigning an empty string to $icon whether whether true or false, so there's no point in having that at all...unless you typoed and meant to assign different values, you should just do if ($s_forum['type'] == 4) $icon = ''; Yeah, I haven't put anything in for the strings yet. But wouldn't that also be a matter of preference for the ternaries? I mean, in the end it gets the same thing done. I'm just curious about this. I don't want to start a bad habit.
-
I'll continue looking. But may I ask how I could improve the regex? Thanks. Thanks for the Regex. I'll have to look into what the ~ means. Also, since I don't want to create another thread: I decided to ask this here. What do you believe is neater? //get appropriate icon for the section if($s_forum['type'] > 3) ($s_forum['type'] == 4) ? $icon = '' : $icon = ''; else $icon = $forum->getIcon($s_forum['icon']); or //get appropriate icon for the section if($s_forum['type'] > 3) { ($s_forum['type'] == 4) ? $icon = '' : $icon = ''; } else { $icon = $forum->getIcon($s_forum['icon']); }
-
I wasn't sure if I was supposed to put this in the Regex section, or PHP help. Seeing as I >think< the Regex part is fine. public function br2nl($string) { return preg_replace('#<br(| )\/>#', "\n", $string); } I built this to change <br /> tags to new lines. Could anyone tell me what I'm doing wrong, as it doesn't convert them.
-
/* * @METHOD checkExistence * @DESC check if the thread exists */ public function checkExistence($id) { $thread = $database->processQuery("SELECT * FROM `threads` WHERE `id` = ? LIMIT 1", array($id), false); return ($database->getRowCount() == 1) ? $x = true : $x = false; } /* * @METHOD canView * @DESC checks if the user has permissions to see thread */ public function canView($id, $username, $powerLevel) { //extract thread details $thread = $database->processQuery("SELECT `parent`,`hidden` FROM `threads` WHERE `id` = ? LIMIT 1", array($id), true); if($database->getRowCount() == 1) { $canSee = true; //get the parent's type $parent = $database->processQuery("SELECT `type` FROM `forums` WHERE `id` = ? LIMIT 1", array($thread[0]['parent']), true); if($parent[0]['type'] == 3 && ($username != $thread[0]['username'] && $powerLevel < 3)) { $canSee = false; } if($thread[0]['hidden'] == 1 && $powerLevel < 3) { $canSee = false; } return false; } return false; } And then I preform the checks here: //preform basic checks if($thread->checkExistence($_GET['id']) == false) $base->redirect('index.php'); if($thread->canView($_GET['id'], $username, $rank) == false) $base->redirect('index.php'); But as you can see in the canView() function, it returns false if the thread is not found. So, should I remove the checkExistence() function, leave the code as is, or just remove the if thread exists check from canView()?
-
<?php require('../structure/base.php'); require('../structure/forum.php'); require('../structure/forum.forum.php'); require('../structure/database.php'); require('../structure/user.php'); if($user->getRank($user->getUsername($_COOKIE['user'], 2)) > 2) { //get selected threads and our action $threads = explode('-', $_GET['threads']); $action = $_GET['action']; //ACTION LIST //1 = HIDE //2 = LOCK //3 = MOVE //4 = AUTO-HIDE //5 = STICKY //6 = DELETE if($action == 3) { foreach($threads as $thread) { moveThread(); } function moveThread($id, $moveTo) { //make sure it exists $thread = $database->processQuery("SELECT * FROM `threads` WHERE `id` = ? LIMIT 1", array($id), true); if($database->getRowCount() == 1) { //array on a different line so it's not so messy $values = array($thread['parent'], $thread['title'], $thread['username'], $thread['date'], $thread['lastpost'], $thread['lastposter'], $moveTo.':'.$id, $thread['timestamp'], $thread['autohiding']); //thread exists, so let's continue and create a new thread where it was orgionally to indicate it has been moved $database->processQuery("INSERT INTO `threads` VALUES (null, ?, ?, '', ?, ?, '', ?, ?, '', '', '', '', '', ?, '0', ?, ?)", array($values), false); //now let's make the orgional thread be moved to the selected destination $database->processQuery("UPDATE `threads` SET `parent` = ? WHERE `id` = ? LIMIT 1", array($moveTo, $id), false); } } } else { } } else { $base->redirect('index.php'); } ?> I'm completely rewriting my website to more efficient/neater code, and I'm curious: the function in the above code moveThread() - should I leave it where it's at, implement the code within the function as a part of the foreach loop, or should I put the function at the very bottom of the page where it's in every scope?
-
Can I input data like this in an associative array?
3raser replied to 3raser's topic in PHP Coding Help
Thanks! That's a creative way to do it. I appreciate you taking the time to help out. -
$array = array(); while($row = mysql_fetch_assoc($query)) { array_merge(postcount($row['username']) => $row['username'].':'.$row['lastlogin']); } Is this possible? It returns: Parse error: syntax error, unexpected T_DOUBLE_ARROW in /homepages/31/d396088066/htdocs/forums/rankings.php on line 67 Thanks!
-
I was reading the documentary for strip_tags() and it mentioned nothing about removing JavaScript tags (which I'm assuming is HTML since it's in the form of it - e.g: <script></script>). I just want to be assured that it does protect against XSS. Do you have any better recommendations? Thanks.
-
One of my features on my website relies on the following function that I wrote up real quick: function getPage() { $page = preg_replace('#\/(.+)\/#', '', $_SERVER['PHP_SELF']); $page = str_replace('/', null, $page); return $page; } It gets the page name you're currently on. Such as: http://www.test.com/test.php - It'll return test.php Is there a built in PHP method to do this?
-
Ah, thank you. I was curious was 256 stood for.
-
Will hash(sha256, $string) always return the same length no matter what the string is?
-
Hi guys! I've been working on a project now for quite some time. Security is a big deal to me. Please, if you feel like you could spare some of your time: could you test my website for vulnerabilties or anything else that could cause a problem? Thanks! URL: http://www.osremake.org Verified: http://www.osremake.org/phpfreaks.txt