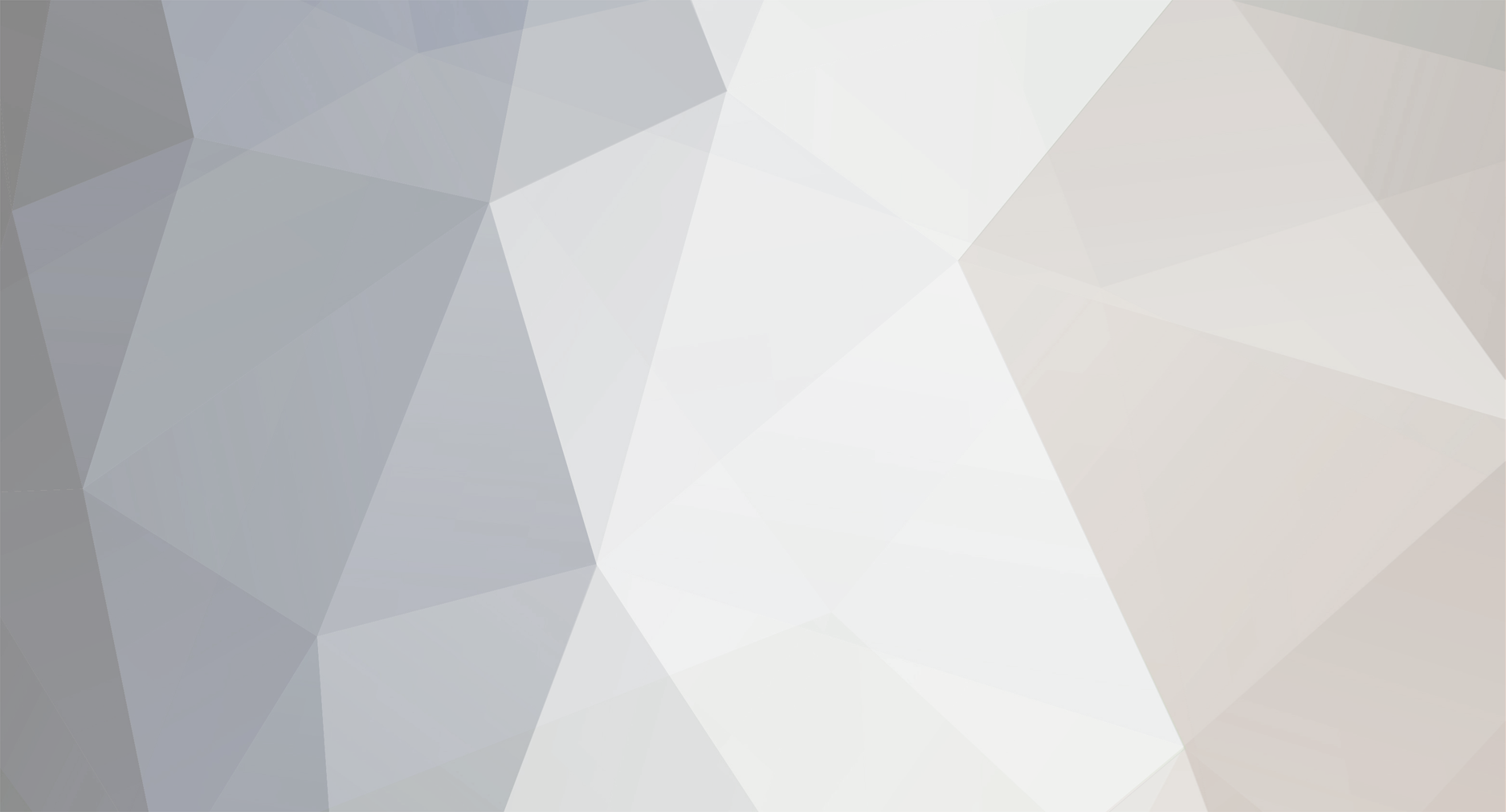
Goat
-
Posts
63 -
Joined
-
Last visited
Goat's Achievements

Newbie (1/5)
0
Reputation
Goat has no recent activity to show
0
We have placed cookies on your device to help make this website better. You can adjust your cookie settings, otherwise we'll assume you're okay to continue.