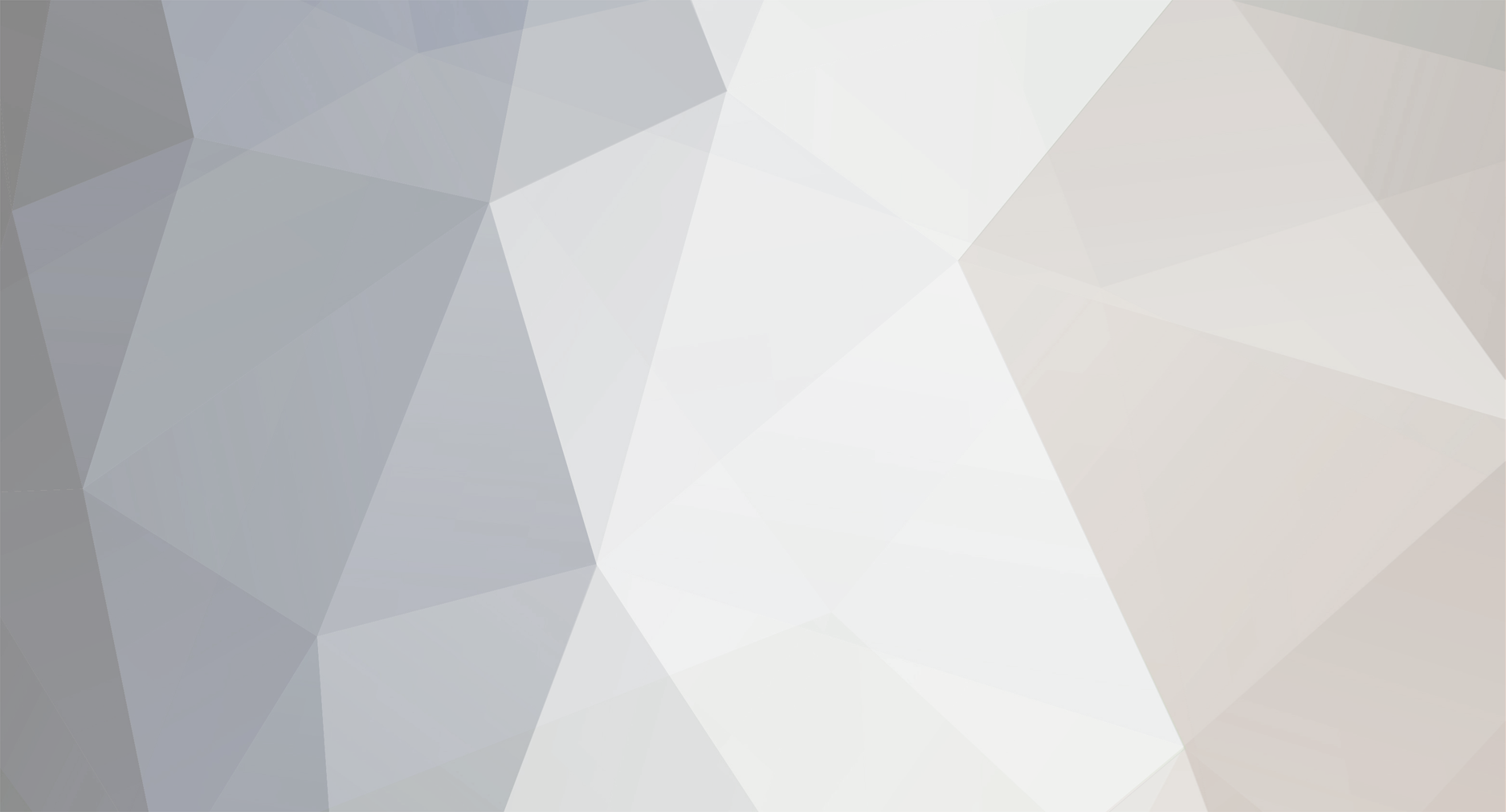
crabfinger
Members-
Posts
110 -
Joined
-
Last visited
Never
Everything posted by crabfinger
-
Try this // Resample // Thumbnail print 'Thumbnal<br />-----'; print 'New Width: ' . $width . "<br />"; print 'New Height: ' . $height . "<br />"; print 'Original Width: ' . $width_orig . "<br />"; print 'Original Width: ' . $height_orig . "<br />"; $image_p = imagecreatetruecolor($width, $height); $image = imagecreatefromjpeg($newname); imagecopyresampled($image_p, $image, 0, 0, 0, 0, $width, $height, $width_orig, $height_orig); // Full size image print 'Full Size Image<br />-----'; print 'New Width: ' . $width2 . "<br />"; print 'New Height: ' . $height2 . "<br />"; print 'Original Width: ' . $width_orig . "<br />"; print 'Original Width: ' . $height_orig . "<br />"; $image_p2 = imagecreatetruecolor($width2, $height2); $image2 = imagecreatefromjpeg($newname); imagecopyresampled($image_p2, $image2, 0, 0, 0, 0, $width2, $height2, $width_orig, $height_orig);
-
Here try this. function get_links($file_array,$baselink) { foreach($file_array as $key => $value) { if(!isset($value['files'])) { $file_name = substr($value['name'],0,1) == '.' ? substr($value['name'],1) : $value['name']; $file_name = substr($file_name,0,1) == '/' ? $file_name : '/' . $file_name; $links[] = $baselink . $file_name . "\r\n"; print_r($links); } else { foreach(get_links($value['files']) as $file) { $links[] = $baselink . $file; } } } return $links; } print_r(get_links(recursive_scan(),'http://www.plagueinfected.com'));
-
is the current copy on your site?
-
try this on for size. Now it will return an array filled with url's to php files outside of your ignored directories and files and inside of your search area. [/code] <?php function recursive_scan($basedir='./',$extension='php',$ignore=array('.','..','include')) { if(file_exists($basedir) && is_dir($basedir)) { $basedir = substr($basedir,-1) != '/' ? $basedir . '/' : $basedir; $list_tmp = glob($basedir . '*'); foreach($list_tmp as $key => $value) { if(!in_array(pathinfo($value,PATHINFO_FILENAME),$ignore)) { if(is_dir($value)) { $files = recursive_scan($value,$extension,$ignore); if(count($files) > 0) { $list[$key]['name'] = $value; $list[$key]['files'] = $files; } } elseif(pathinfo($value,PATHINFO_EXTENSION) == $extension) { $list[$key]['name'] = $value; } } } return $list; } else { return FALSE; } } function get_links($baselink,$file_array=NULL) { if($file_array === NULL) { $file_array = recursive_scan(); } foreach($file_array as $key => $value) { if(!isset($value['files'])) { $file_name = substr($value['name'],0,1) == '.' ? substr($value['name'],1) : $value['name']; $file_name = substr($file_name,0,1) == '/' ? $file_name : '/' . $file_name; $links[] = $baselink . $file_name . "\r\n"; } else { foreach(get_links($value['files']) as $file) { $links[] = $baselink . $file; } } } return $links; } print_r(get_links('http://www.example.com')); ?> [/code]
-
Well what my function is supposed to return is a structured array. Tell me what it gives you.
-
I got to thinking and this is what I came up with. It's fully recursive and you can change where it searches, what it searches for and what it ignores using function arguments. Try this instead of your function. <?php function recursive_scan($basedir,$extension='php',$ignore=array('.','..')) { if(file_exists($basedir) && is_dir($basedir)) { $basedir = substr($basedir,-1) != '/' ? $basedir . '/' : $basedir; $list_tmp = glob($basedir . '*'); foreach($list_tmp as $key => $value) { if(!in_array(pathinfo($value,PATHINFO_FILENAME),$ignore)) { if(is_dir($value)) { $files = recursive_scan($value,$extension,$ignore); if(count($files) > 0) { $list[$key]['name'] = $value; $list[$key]['files'] = $files; } } elseif(pathinfo($value,PATHINFO_EXTENSION) == $extension) { $list[$key]['name'] = $value; } } } return $list; } else { return FALSE; } } print_r(recursive_scan('./','php',array('.','..','include'))); ?>
-
Try this line in your listdir function if ($fn != '.' && $fn != '..' && $fn != 'includes'){ // ignore these
-
Try this out // Get new dimensions list($width_orig, $height_orig) = getimagesize($newname); // Thumbnail if($height_orig > $width_orig) { $ratio = $width_orig/$height_orig; $height1 = 90; $height2 = 380; $width = $height*$ratio; $width2 = $height2*$ratio; } else { $ratio = $height_orig/$width_orig; $width = 90; $width2 = 380; $height = $width*$ratio; $height2 = $width2*$ratio; } // Resample // Thumbnail $image_p = imagecreatetruecolor($width, $height); $image = imagecreatefromjpeg($newname); imagecopyresampled($image_p, $image, 0, 0, 0, 0, $width, $height, $width_orig, $height_orig); // Full size image $image_p2 = imagecreatetruecolor($width2, $height2); $image2 = imagecreatefromjpeg($newname); imagecopyresampled($image_p2, $image2, 0, 0, 0, 0, $width2, $height2, $width_orig, $height_orig);
-
Sorry brain fart if($height_orig > $width_orig) { $ratio = $width_orig/$height_orig; $height = 380; $width = $height*$ratio; } else { $ratio = $height_orig/$width_orig; $width = 380; $height = $width*$ratio; }
-
Sorry all I saw was unnecessary variables but I guess they aren't list($width_orig,$height_orig) = getimagesize($newname); if($height_orig > $width_orig) { $ratio = $width/$height; $height = 380; $width = $height*$ratio; } else { $ratio = $height/$width; $width = 380; $height = $width*$ratio; }
-
or you could get fancy and do this list($width,$height) = getimagesize($newname); list($width,$height) = $width > $height ? array(380,380 * ($height / $width)) : array(380 * ($width / $height),380);
-
I think this is what you're looking for. list($width,$height) = getimagesize($newname); if($height > $width) { $ratio = $width/$height; $height = 380; $width = $height*$ratio; } else { $ratio = $height/$width; $width = 380; $height = $width*$ratio; }
-
Try this and see if it works. if($queryString = $_SERVER['QUERY_STRING']) { $query = mysql_query('SELECT * FROM user WHERE ActivationKey="' . $queryString . '"'); if(mysql_num_rows($query) == 1) { $array = mysql_fetch_array($query); if($array['UserStatus'] == 'verify') { if(@mysql_query('UPDATE user SET UserStatus="activated" WHERE ActivationKey="' . $queryString . '")) { echo "Congratulations! you are now the proud new owner of an Wine7000.com account."; } else { echo mysql_error(); } } else { echo 'You\'re account has already been activated.' } } else { echo 'We cannot seem to find you\'re account'; } }
-
Wouldn't it be a lot easier to just use a file name 'lock.txt' next to the file with a value of 1 or 0?
-
That's what we want, why do we need to actually have to upload the file when we're just debugging. I've spotted quite a few discrepancies in your code. So what I did was I went ahead and slimmed it down a bit getting rid of unnecessary crap. This should meet your needs. <?php $images = array('image/gif','image/jpeg','image/jpg','image/pjpeg'); if(in_array($_FILES['file']['type'],$images) && $_FILES['file']['size'] <= 10000000 ) { if( $_FILES['file']['error'] > 0 ) { echo 'Return Code: ' . $_FILES['file']['error'] . '<br / >'; } else { echo 'Upload: ',$_FILES['file']['name'],'<br />'; echo 'Type: ',$_FILES['file']['type'],'<br />'; echo 'Size: ',($_FILES['file']['size'] / 1024),'KB</br >'; echo 'Temp File: ',$_FILES['file']['tmp_name'],'<br />'; $upload_dir = 'uploads/' . date("F") . " " . date("y") . '/'; $upload_file = pathinfo($_FILES['file']['name'],PATHINFO_FILENAME); $upload_file .= '-' . intval(microtime(TRUE)); $upload_file .= pathinfo($_FILES['file']['name'],PATHINFO_EXTENSION); if(!file_exists($upload_dir)) { mkdir($upload_dir); } $target = $upload_dir . $upload_file; move_uploaded_file($_FILES['file']['tmp_name'],$target); } } else { echo 'Ongeldig bestand.'; echo 'Kijk grootte en type na.'; } ?>
-
This will never work <textarea value="Hello World!"></textarea> try this instead <textarea>Hello World</textarea>
-
Well you could have the user click a link to "Check out" the file and then it is in their name/ip address until they click a link to "Check in" the file, like in Joomla file edit. And as a fail safe, if they don't check out within a certain period of time or they don't refresh the page within a certain period of time (you can set the page to refresh with javascript if they are still viewing the page and therefore if they aren't viewing the page then the page won't refresh and the file will be checked back in)... or something like that
-
Hey guys, I'm working on a new content management system. Well... I've been working on it for a few years in a few different implementations, but i always end up losing the data, so now I'm starting again. And I didn't know where to start, so I started with the error manager. I need help from you guys with suggestions on what data would be helpful to have when reporting an error, how I should format the error message that is presented to the user, and how to make this code more efficient as well as more dynamic. ./classes/manageErrors.class.php <?php class manageErrors { function add_error($code) { if($error['message'] = $this->get_error($code)) { $error['code'] = $code; $error['time'] = time(); $backtrace = debug_backtrace(); foreach($backtrace as $key => $value) { if($key > 0) { $key = 'func' . str_pad($key,3,'0',STR_PAD_LEFT); $error[$key] = $value['function'] . '(' . implode(',',$value['args']) . ')'; $error[$key] .= ' called on line ' . $value['line']; $error[$key] .= ' in file ' . $value['file']; } } $this->errors[] = $error; return TRUE; } else { return FALSE; } } function get_error($code=NULL) { $error_file = './conf/errors.conf'; if(file_exists($error_file)) { $errors = file_get_contents($error_file); $errors = explode("\n",trim($errors)); foreach($errors as $key => $value) { $error = explode(':',$value); $errors[$error['0']] = $error['1']; } if(is_numeric($code)) { if(isset($errors[$code])) { return $errors[$code]; } else { return FALSE; } } else { return $errors; } } } function format($format='[%time%] (%code%) %message%',$date='h:i:s') { if(isset($this->errors)) { foreach($this->errors as $errori => $errora) { $string_temp = $format; foreach($errora as $key => $value) { if($key == 'time') { $value = date($date,$value); } $string_temp = str_replace('%' . $key . '%',$value,$string_temp); } $string .= $string_temp . "\r\n"; } return 'The following errors have been logged and sent to a site administrator for analysis. We will fix this problem as soon as we can, please try again later.' . "\r\n" . $string; } else { return 'No errors occured during the execution of this script.'; } } } $errors = new manageErrors(); ?> ./conf/errors.conf 001:A function was called that requires a different type of variable than the one supplied. Thanks in advance for all of your help.
-
Though I would watch out in doing this script, some websites may think of this as phishing. I tried something similar once and I got a phone call from the server administrators at the site saying I had 15 minutes to take down the script or the authorities would be notified.
-
in your form none of the variables are the same as the ones you set earlier. $nameField is not the same as $name and therefore none of the variables were actually initialized.
-
That may be but i don't see how that relates to your original complaint
-
$friend_file = 'friends.txt'; $friend_content = file_get_contents($friend_file); if(isset($_POST['fcode'])) { $friend_code = $_POST[fcode]; $friend_content = $friend_content . "\r\n" . $friend_code; if(file_put_contents($friend_file,$friend_content)) { print $friend_code . ' has been added to the friends file.<hr />'; } } print '<hr />' . $friend_content; means that i only have to load the file once because it is being used wether or not the form is posted.
-
Do some debugging, change this move_uploaded_file($_FILES['file']['tmp_name'], $target3); to this //move_uploaded_file($_FILES['file']['tmp_name'], $target3); print $target2 . '<br />' . $target3; and tell us what that gives you
-
All you have to do is look at what the expected output of this code is. $ext = findexts ($_FILES['file']['tmp_name']) ; $ran = date(dmY); $target= "uploads/"; if(file_exists($target . $uploaddir)) { $target2 = $target . $uploaddir . "/" . $_FILES['file']['name']; } else { mkdir($target . $uploaddir); $target2 = $target . $uploaddir . "/" . $_FILES['file']['name']; } $target3 = $target2 . $ran . $ext; It's going to be the original file name with the day month and year at the end. So that means that if you wanted to upload a file with the same name, you're going to have to wait till tomorrow. All you have to do to fix this is change the date at the end to microtime like this. $ran = intval(microtime(TRUE)); Now you can upload a file as long as someone else isn't uploading a file with the same filename at the exact microsecond that you are, but what are the odds of that happening any time soon? Also you should think about taking the contents of the file and sticking it in a database. It saves on lines of code, it's more secure, and i'm pretty sure its faster to read.