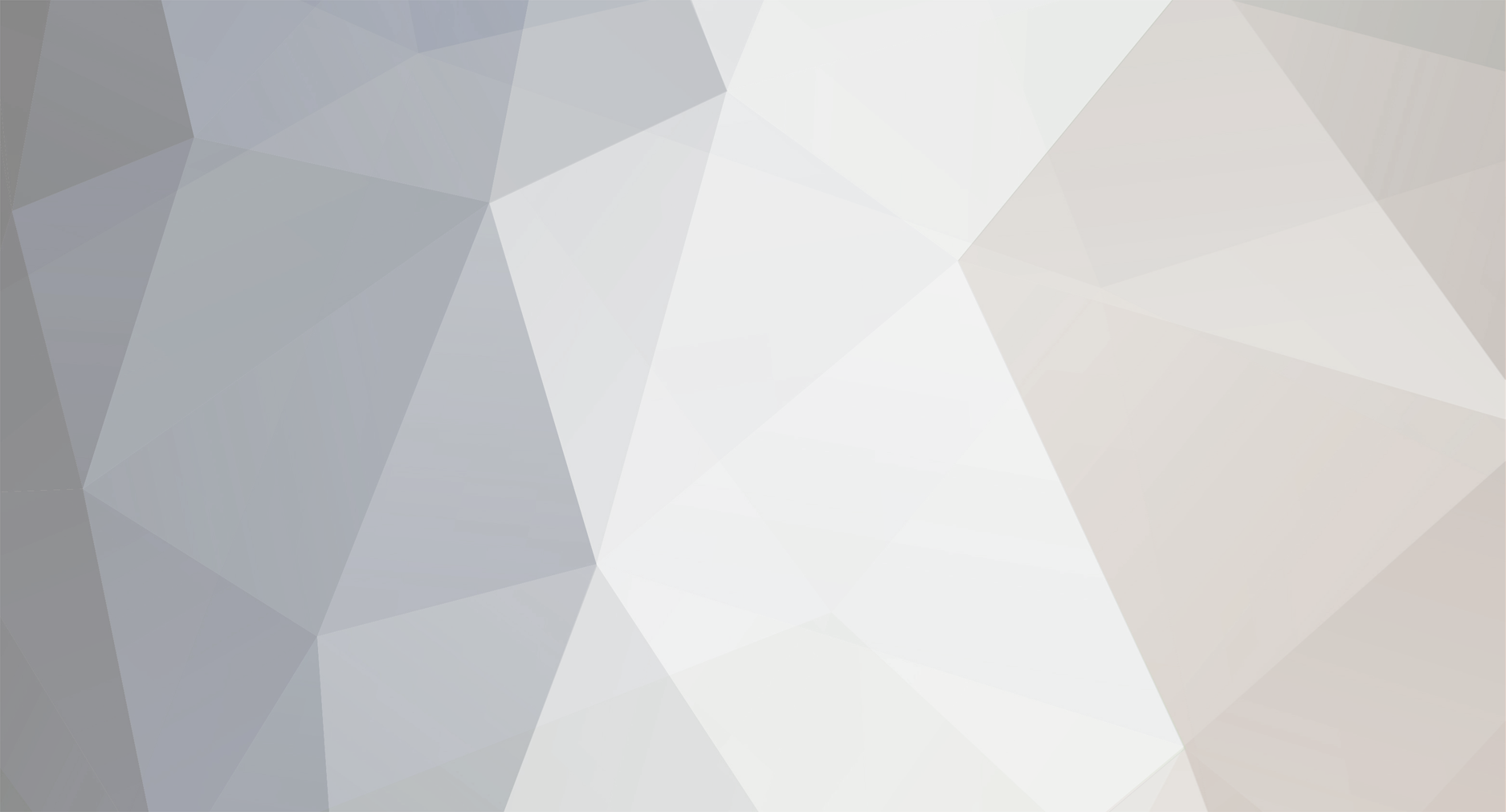
foxsoup
Members-
Posts
19 -
Joined
-
Last visited
Never
About foxsoup
- Birthday 03/31/1983
Contact Methods
-
Website URL
http://www.foxsoup.co.uk
Profile Information
-
Gender
Male
-
Location
London, UK
foxsoup's Achievements

Newbie (1/5)
0
Reputation
-
Not tested this code, but using count() to get the size of the array and then having an incremental counter to determine the last array entry could work: $array_size = count($something); $count = 0; foreach ($something as $thing) { $count++; $something_array = array('title' => $thing->getId()); echo json_encode($something_array); echo ($i == $array_size) ? '' : ','; echo "\n"; }
-
If I understand this correctly, maybe just rearranging your variables and adding a <br /> tag will do the trick? $result_array[] = "<a href='viewgallery.php?cid=".$row[0]."'><img src='/photos/categoryimages/category".$row[0].".jpg' border=0 height='133px' width='200px' /><br />".$row[1]."</a>";
-
Ah, kenrbnsn is indeed right with the assertation that it's your generated SQL statement that's wrong. Your code to generate the numbers for the LIMIT part of the statement is in error, since it's creating a negative number for $pn. Given that $prows is the number of results to show per page, and $page is the page number taken from the $_GET variable, try using these lines to get the page number and LIMIT offset: $page = isset($_GET['p']) ? $_GET['p'] : 1; $pn = ($page * $prows) - $prows;
-
It sounds very much like you're getting that error simply because you have no reply posts in the table to fetch. Try putting some sanity-check code around the while statement, i.e. if (mysql_num_rows($q) > 0) { while ($f = mysql_fetch_array($q)) { // put your code in here } } This way your code will only try to run the while loop if there are any results to fetch. Without a num_rows check like this your code is trying to fetch results from an empty result resource, resulting in an error.
-
Hey there. If you want to check a string for a character which isn't a letter, number, period or underscore then you could use the following: if (preg_match('/[^a-z0-9\._]/i', $yourstring) > 0) { // contains bad characters } Basically the '^' character means find matches that aren't in the square brackets. Then we're defining a range of all letters (a to z), all numbers (0 to 9), a period (which needs a backslash in front of it to escape it, since a period is a regex metacharacter) and an underscore. The 'i' at the end of the regex, outside of the delimiters, means to make the search case-insensitive (so a-z also covers A-Z). The reason I've used preg rather than ereg for this is that the ereg functions have been deprecated as of PHP 5.3, and will be removed from PHP 6, so using preg should extend the lifespan of your code somewhat.
-
Just echo the '...' after the cut-down text, e.g. <?php $data = 'Pulp Fiction'; echo substr($data, 0, 7) . '...'; ?>
-
Eeep, slight brainfart moment there. Corollary to what I stated before, you can pass the session ID via the URL by turning session.use_trans_sid on in your php.ini file, but this is generally discouraged for security reasons plus it makes your URLs look pretty ugly. I'd recommend some checking up on the implications of using it before putting it into production.
-
The server still needs a method of identifying a client with a session, so it uses a cookie to match the session ID. From http://www.tuxradar.com/practicalphp/10/0/0 : "Sessions grew up from cookies as a way of storing data on the server side, because the inherent problem of storing anything sensitive on clients' machines is that they are able to tamper with it if they wish. In order to set up a unique identifier on the client, sessions still use a small cookie - this cookie simply holds a value that uniquely identifies the client to the server, and corresponds to a data file on the server." In short, a client with all cookies disabled won't be able to use sessions on your site.
-
Sessions still require cookies to be enabled on the client end so that a 'session tracking cookie' can be written which identifies that particular client with a particular session on the server. A client will need to have cookies enabled to make use of a website that uses either cookies or sessions to remember states across pages.
-
Need some help with build array of a folder
foxsoup replied to jim.davidson's topic in PHP Coding Help
It's possibly failing because the directory doesn't exist ('../Misc' as set on line 72), but hard to tell for sure without knowing the exact error message. If you just want a simple bit of code to scan a directory on the server for image files then this should do the trick though: <?php $dirToScan = '.'; // Directory to scan (current dir) $imgExts = array('jpg', 'jpeg', 'png', 'gif', 'bmp'); // Array of possibly image file extensions $imgFiles = array(); // Create blank results array $allFiles = scandir($dirToScan); // Get all files in dir into an array foreach ($allFiles as $filename) { // Loop through each $fileInfo = pathinfo($filename); // Get the info of each file if (in_array($fileInfo['extension'], $imgExts)) { // Check to see if file ext is in array $imgFiles[] = $filename; // If so, add the filename to results array } } var_dump($imgFiles); // Print the results ?> -
Hi there. If you want to block entire ranges of IP addresses (i.e. 111.222.333.* or 192.168.*.*) then you'd probably need to use regex. I just gave this bit of code a go and it seems to work with the examples I've set in the $deny array: <?php // Set banned IPs and IP ranges - wildcard combinations should all work $deny = array( '111.222.333.444', '111.222.333.*', '222.*', '333.444.*', '444.*.555.*' ); // Loop through each denied entry foreach ($deny as $blockedIp) { $blockedIp = preg_quote($blockedIp); // Escape metachars $blockedIp = str_replace('\*', '.*', $blockedIp); // Relplace escaped * with .* to match multiple unknown chars $regex = '/' . $blockedIp . '/U'; // Construct regex variable with 'ungreedy' mode on if (preg_match($regex, $_SERVER['REMOTE_ADDR']) == 1) { header("location: http://www.yourchoice.co.uk/"); exit(); } } ?> The downside to this method is that every check is now being done with regex, putting more resource use on the server than the in_array() method you were previously using. If you anticipate using this a lot then I'd recommend seperating the checks for static banned IPs and wildcard range IPs with something like this: <?php // Set denied static IPs in here $denyStatic = array( '111.222.333.255', '333.333.333.1', ); // Set denied IP ranges in here $denyRange = array( '111.222.333.*', '222.*', '333.444.*', '444.*.555.*' ); // First to basic check against static list if (in_array($_SERVER['REMOTE_ADDR'], $denyStatic)) { header("location: http://www.yourchoice.co.uk/"); exit(); } // Didn't find them in the static list? Try the range list instead! foreach ($denyRange as $blockedIp) { $blockedIp = preg_quote($blockedIp); $blockedIp = str_replace('\*', '.*', $blockedIp); $regex = '/' . $blockedIp . '/U'; if (preg_match($regex, $_SERVER['REMOTE_ADDR']) == 1) { header("location: http://www.yourchoice.co.uk/"); exit(); } } ?> Best of luck!
-
Hmm... if the error message you're getting is 'Parse error: syntax error, unexpected T_STRING in /home/jjennings3/jjennings3.bimserver2.com/siteadmin.php on line 7' when you have been redirected it's because of a typo in the siteadmin.php page. Almost certainly a missing semi-colon at the end of line 6 or 7 ('unexpected T_STRING' nearly always means a missing semi-colon at the end of a line). NB: I'm off for the night now. Will check in again tomorrow if things are still broked.
-
Need help getting a value inbetween pattern
foxsoup replied to andyhajime's topic in PHP Coding Help
EDIT: As I was writing this monolithic post there were three replies with much more direct answers to the problem. Ah well... --- preg_replace() is definately the way foward with this problem (the ereg functions are being phased out in PHP6, so best to start on the right foot and use preg). Unfortunately regex is a pretty complicated and powerful beast, so I'll stick to addressing the problem in question and try to explain it as best I can. So I'm assuming that you'd like to change a paragraph of text from this: Taken on 24th Feb 2010, where [[Azrael]], [[HarryEx]] and [[Krisgage]] ventures in search for a certain 1936 British Outpost which was reported by [[Redstone]]. to something like this: Taken on 24th Feb 2010, where <a href="Azrael">Azrael</a>, <a href="HarryEx">HarryEx</a> and <a href="Krisgage">Krisgage</a> ventures in search for a certain 1936 British Outpost which was reported by <a href="Redstone">Redstone</a>. Simply put, you can do this with the following code: <?php $data = 'Taken on 24th Feb 2010, where [[Azrael]], [[HarryEx]] and [[Krisgage]] ventures in search for a certain 1936 British Outpost which was reported by [[Redstone]].'; $output = preg_replace('/\[\[(.+)\]\]/iU', '<a href="\1">\1</a>', $data); echo $output; ?> Briefly, you can see that the preg_replace function is split into three parts seperated by commas. The third part is pretty obvious - it's the variable containing the data we want to work on. But what do the first two do? And how the hell do you make sense of them? Well, the first part: '/\[\[(.+)\]\]/iU' is the search pattern. Basically you're looking for some text wedged in between sets of [[ and ]]. Regular expressions use symbols called metacharacters which we use when we don't know exactly what we're looking for but we do know the pattern. For example, we know that a date (in the UK anyway) has the format DD/MM/YYYY. We might not know exactly what the date is, but we do know to expect two numbers, followed by a slash, followed by two more numbers, followed by another slash, followed by four numbers. Since I don't know exactly what to expect between your [[ and ]], I can use the combination of metacharacters '.' and '+'. The period basically means 'this can be any single character', while the plus sign following it means 'one or more of what came in front of me'. So '.+' means 'look for one or more of any character'. So you'd think that the complete regex should be '[[.+]]'. Well unfortunately life isn't quite that simple. You see, the square bracket characters also have special meaning in regex (called a character class), so in order to use them we need to escape them by preceeding each one with a backslash. So now the regex looks like '\[\[.+\]\]'. Next we want to copy the actual text between the square brackets so we can write it into the <a> tag. We do this using subpatterns and backreferences. We can set a subpattern around the stuff we want to keep by putting regular brackets around it, thus: '\[\[(.+)\]\]'. This means that the blurb in between the square brackets will be available to be inserted into the hyperlink. Finally the entire regex (in preg fucntions anyway) has to be encapsulated by delimiter characters. These can actually be anything (as long as both are the same) but typically the / character is used. After the ending delimiter we can add modifiers to extend the functionality of the search. In this case I've put in a lowercase 'i' (to make the search case-insensitive) and an uppercase 'U' (to make the search 'ungreedy' - i.e. it will only find the shortest possible match). For a much better and in-depth description of all that stuff, check this manual section of php.net - http://uk.php.net/manual/en/reference.pcre.pattern.syntax.php The second part of the preg_replace function is the text that we're replacing the original with, and is comparatively simple: '<a href="\1">\1</a>' As you can see it's basically just a <a> tag with the phrase '\1' in it twice. This is the backreference for the subpattern we defined earlier - the bit that we put in regular brackets will be copied into here. If we had defined more than one subpattern then we'd use \1 to reference the first one, \2 for the second, and so on. I hope at least some of all that made sense, it was certainly a lot more than I originally intended to write! For more details about regex and the many, many, many functions of it, check this site out - http://www.regular-expressions.info I go drink beer now. -
No probs. Try replacing your userlogin.php file with this for now: <?php if (empty($_POST['username']) || empty($_POST['username'])) { header("location: http://jjennings3.bimserver2.com/userlogin.html"); exit(); } $conn = mysql_connect('localhost', 'jjennings3db', 'bullet557'); mysql_select_db('jjennings3db', $conn); $username = $_POST['username']; $password = $_POST['password']; $sql = "SELECT * FROM login WHERE username = '$username' AND password = '$password' LIMIT 1"; $result = mysql_query($sql, $conn); if (mysql_num_rows($result) == 1) { echo 'Login was successful!'; } else { echo 'Username / password do not match any records found in database!'; } ?> All that should do for now is take the username and password you entered in the text boxes on the userlogin.html page and tell you either 'Login was successful!' or 'Username / password do not match any records found in database!'. If you keep getting user/pass not found messages even when you're definately entering the right details then the password field in the table is probably encrypted. When you first created the login table in the database how did you add the first user? BTW: If you're just starting out in PHP/MySQL then I could recommend this book for an easy introduction to concepts like writing login systems: http://www.amazon.co.uk/PHP-MySQL-Dynamic-Web-Sites/dp/032152599X/ref=sr_1_fkmr0_1?ie=UTF8&qid=1274368009&sr=8-1-fkmr0 Can't personally vouch for the latest version of it but back in the day when I was learning I found an older version of it very helpful and easy to get into.