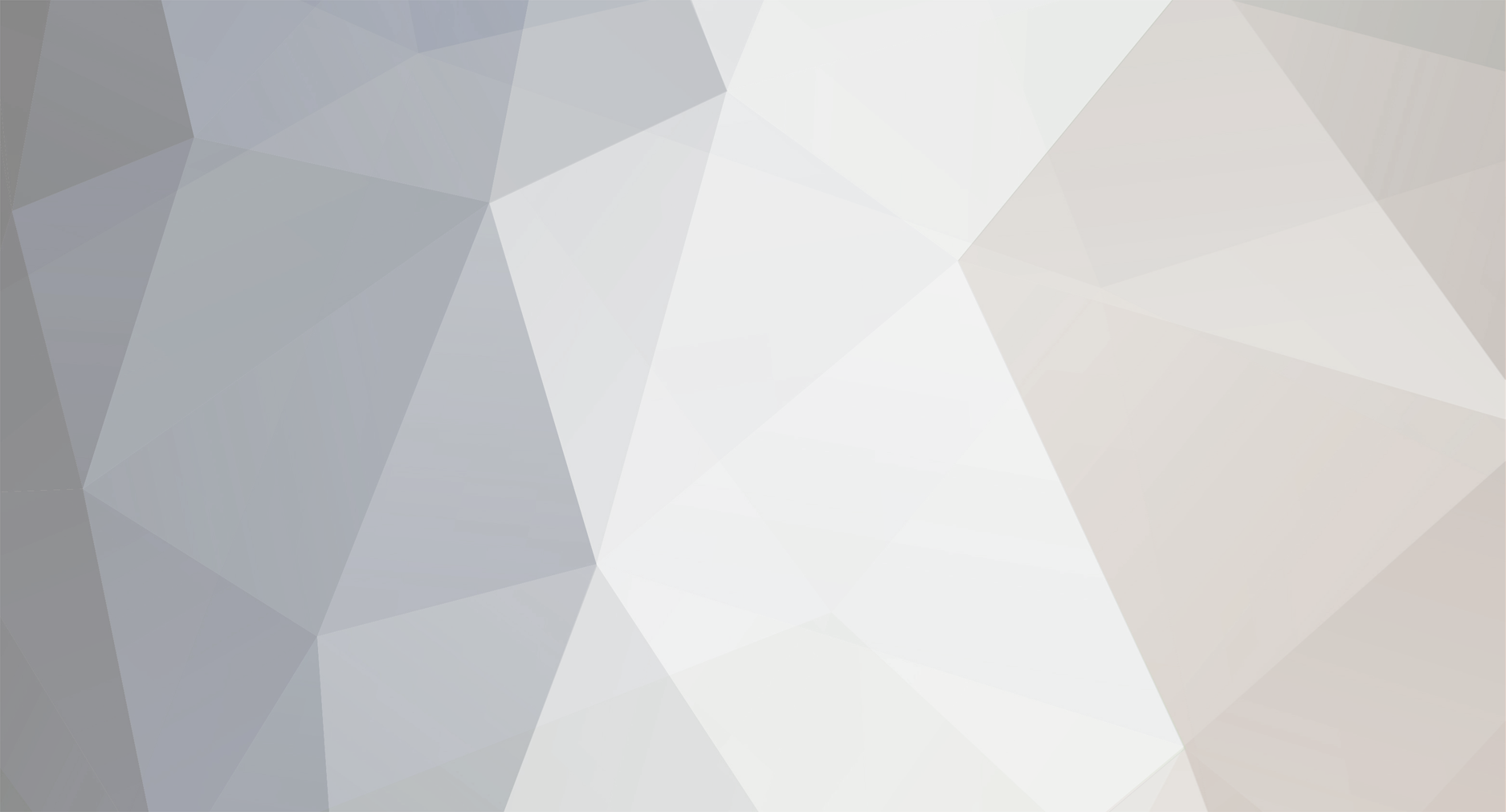
soma56
Members-
Posts
185 -
Joined
-
Last visited
Never
Everything posted by soma56
-
S.C. Chen created a great script known as PHP Simple HTML DOM Parser (http://simplehtmldom.sourceforge.net). It allows for easy manipulation of html. While running the script through a loop I've been experiencing major memory issues. I soon discovered that the script has a function that cleans up the memory. Great! Here's a tidbit from the Author: Due to php5 circular references memory leak, after creating DOM object, you must call $dom->clear() to free memory if call file_get_dom() more then once. Example: $html = file_get_html(...); // do something... $html->clear(); unset($html); This seems simple enough. I'm converting the html to plaintext through his script so I figured this would work: include('simple_html_dom.php'); $html = file_get_html(...); // do something... $text = str_get_dom($html)->plaintext; // do something... $text->clear(); unset($html); I receive the following error "Call to a member function clear() on a non-object in...". This appears to be the results of failing to include the script that contains the function yet other aspects of the script work correctly. This is based on my 2 months of learning php anyways. I suspect I'm not calling the function correctly $text->clear(); or is there something I'm missing?
-
Ok, I'll start mastering cookies now. Thanks.
-
Well if that's the case let me give you guys a more specific example. Let's assume that the user is receiving mass amounts of data over a couple of hours to their browser but for my purposes we'll use this code: <?php $i = 0; while ($i < 20){ $i++; echo $i; ob_flush(); flush(); usleep (1000000); } ?> Should the connection become lost from the user to the server would it be more wise to use cookies or sessions?
-
Thanks, being new to php > 2 months I've learned a lot and I had a feeling that sessions would be the right direction.
-
Let's say we have a script the does some echoing to the user through a loop. What happens if the users Internet connection goes down? Once it does come back up is there any way to resume or otherwise continue the script from where the user left off? If anyone has a specific page that I can read into this more (if it's even possible) then that would be great.
-
Thanks Ken, I appreciate it but it didn't work. I discovered that this did for anyone looking: <?PHP //Bring in Array Data $mytextarea = explode("\n", $_POST['mytextarea']); //Remove Return $mytextarea = str_replace("\r",'',$mytextarea) ; ?> The only difference from my original post, and likely what hindered the function correctly, is that I placed the 'str_replace' right after the $_POST.
-
I'm trying to remove blank spaces after a textarea submit. For example: Blank space Blank space text1(enter key pressed) text2(enter key pressed) text3(enter key pressed) Blank space Blank space Everything I've researched says something like this should work (Confused as to why it's not): foreach ($mytextarea as $e) { if ($e == "") { $e = preg_replace( '/\r\n/', '', $e); } } I also thought a string replace would do the trick after checking the length: function validElement($element) { return strlen($element) > 1; } $mytextarea = array_values(array_filter($mytextarea, "validElement")); $mytextarea = str_replace("\r",'',$mytextarea; Neither seem to be working as when I: count($mytextarea); echo $mytextarea; I always see the extra 'return' as values in the array.
-
It seems that after I bring the data in: $filter = explode("\n", $_POST['filter']); If I remove 'return' from the array then it works: $filter = str_replace("\r",'',$filter) ;
-
Javascript Guru Need - How to Select all instances of same CSS class?
soma56 replied to soma56's topic in Javascript Help
Sure: <h1>How Do I Highlight All The Numbers?</h1> <form> <input type="button" value="Select/Highlight" onClick="fnSelect('mydiv')"> <div class="my class" id="mydiv">1</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">2</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">3</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">4</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">5</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">6</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">7</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">8</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">9</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">10</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">11</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">12</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">13</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">14</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">15</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">16</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">17</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">18</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">19</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">20</div><br /><div id="anotherdiv">I Like Numbers</div><br /><div class="my class" id="mydiv">21</div><br /><div id="anotherdiv">I Like Numbers</div> </form> The php script runs through a simple loop echoing out numbers. While the button can highlight the first number I'm trying to figure out how to highlight all instances of the same div. I think it can only be done through a class or name associated with the div. While I did figure out how to actually highlight the div: <script type="text/javascript"> function highlight (item) { var items = document.getElementsByName (item); var j=items.length; for (var i=0; i<j; i++) { items[i].className = "highlight"; } } </script> <style type="text/css"> .highlight { background: #FF0000; color: #0000FF; } </style> <h1>This is one way to highlight the target DIVs</h1> <form> <input type="button" value="Select/Highlight" onClick="highlight('thisone')"><br><br> <?php $i = 0; while ($i < 20){ $i++; echo '<div name="thisone">Highlight this DIV - '.$i.'</div>'; echo '<br />'; echo '<div>I Like Numbers</div>'; echo '<br />'; } ?> </form> I couldn't figure out how to highlight them as a means of 'selecting' the text for copying. I.E. hit CTRL-C right after highlighting to copy. -
Here's an example of what I've been banging my head against the wall with for the last couple of days. If you throw it in a browser it will make sense. [code=php:0] <script type="text/javascript"> function fnSelect(objId) { fnDeSelect(); if (document.selection) { var range = document.body.createTextRange(); range.moveToElementText(document.getElementById(objId)); range.select(); } else if (window.getSelection) { var range = document.createRange(); range.selectNode(document.getElementById(objId)); window.getSelection().addRange(range); } } function fnDeSelect() { if (document.selection) document.selection.empty(); else if (window.getSelection) window.getSelection().removeAllRanges(); } </script> <h1>How Do I Only Highlight the Numbers?</h1> <form> <input type="button" value="Select/Highlight" onClick="fnSelect('mydiv')"> <?PHP $i = 0; while ($i <= 100){ ob_flush(); flush(); $i++; echo "<div class=\"my class\" id=\"mydiv\">$i</div>"; echo "<br />"; echo "<div id=\"anotherdiv\">I Like Numbers</div>"; echo "<br />"; // wait for 1 second usleep(1000000); } ?> </form> My php script echoes out the div's I need highlighted along with other information in chunks. I need to be able to click the button and highlight the same css class that is on the page.
-
I've discovered that the script is comparing against the last instance of where the 'enter' key is in the textarea prior to pushing the submit button. Here's a complete copy of the script: <form action="" method="post"> Filter Array Input<textarea name="filter"> </textarea> <br><br> Compare Against Array Input<textarea name="compare" ></textarea> <br><br> <input type="submit" name="Submit" value="Start"> </form> <?PHP if (isset($_POST['Submit'])) { $filter = explode("\n", $_POST['filter']); $compare = explode("\n", $_POST['compare']); function validElement($element) { return strlen($element) > 1; } $filtered_array = array_values(array_filter($filter, "validElement")); function filterArrayByWords($inputAry, $excludeWordsAry, $returnInclude=true) { $excludeAry = array(); $includeAry = $inputAry; foreach($includeAry as $keyInt => $valueStr) { foreach($excludeWordsAry as $excludeWordStr) { //Check for exclude word using word boundrys if(preg_match("/\b{$excludeWordStr}\b/i", $valueStr)!=0) { $excludeAry[$keyInt] = $valueStr; unset($includeAry[$keyInt]); break; } } } return ($returnInclude) ? $includeAry : $excludeAry; } print_r(filterArrayByWords($compare, $filtered_array)); echo "<br /><br />"; print_r(filterArrayByWords($compare, $filtered_array, false)); //end isset } ?> Try placing this in the bottom box: good bad ugly and one of the same to compare against in the top box. Clicking submit should result in: Array ( [1] => bad [2] => ugly ) Array ( [0] => good ) Now try it again and hit enter a few times after placing a word in the top box. The results come back: Array ( [0] => good [1] => bad [2] => ugly ) Array ( ) And as a result the filter doesn't work. There must be some explanation or easy fix.
-
After searching for a solution for the past few hours I'm concluding that it's centered around something to do with the extra blank line being received in the form. This function will not work if I bring it in an array that has a new line - if someone hits 'enter' in the textarea before hitting submit: function strpos_array($haystack, $search){ foreach($search as $term){ if(stristr($haystack, $term) !== false){ return true; } } return false; } foreach($mylist as $key => $val){ if (strpos_array($val, $myarray)){ unset ($mylist[$key]); } I tried remove the line by checking the length: function validElement($element) { return strlen($element) > 1; } $filtered_array = array_values(array_filter($myarray, "validElement")); This looked promising: function replace_newline($string) { return (string)str_replace(array("\r", "\r\n", "\n"), '', $string); } I've also tried adding a new line after each variable thinking maybe the function needed a "\n" $newlist = array_values($filtered_array); foreach ($newlist as $key => $val){ $val = "$val"."\n"; echo $val; } Oddly, the script works fine if the textarea is submitted as: Apples Oranges Peaches But returns nothing when brought in like this: Apples Oranges Peaches wtf?
-
Complicated for me anyways. The script I'm working on works fine. When 'My list' check-box is ticked and there are values in the textarea then it scrubs fine against my 'check list'. <?PHP //Asign variables $notselected = "Not Selected"; $selected = "Selected"; if (isset($_POST['Submit'])) { //A general purpose list if (isset($_POST['mylistcheckbox'])) { $mylist = explode("\n", $_POST['mylist']); $mylistcheckbox = $selected; } else { $mylist = $notselected; } //A list to srub against for duplicates if (isset($_POST['listcheckbox'])){ $checklist = explode("\n", $_POST['checklist']); $listcheckbox = $selected; } else { checklist = $notselected; } //Strpos function function strpos_array($haystack, $search){ global $$mylist; foreach($search as $term){ if(stristr($haystack, $term) !== false){ return true; } } return false; } //if statement for $mylist if (($mylistcheckbox == $selected) && ($checklist != 0)){ foreach($mylist as $key => $val){ if (strpos_array($val, $checklist)){ unset ($mylist[$key]); } } } //end isset } ?> However, I'm having issues when one of two issues occurs: When the checkbox is ticked on the previous form (and the corresponding textarea is blank) the script still wants to process the above function. I receive a "Warning: stristr() [function.stristr]: Empty delimiter in..." I've tried 'less then', 'empty', 'Not equal to' but it still wants to process resulting in this error. Further more should I hit the enter button a few times after typing a line in my 'checklist' I receive the same error. I did some research and discovered this: $checklist = str_replace("\0", "", $checklist); but alas it is not working. Suggestions are always appreciated.
-
For anyone that needs to have this done as far as case insensitive goes I figured it out: function strpos_array($haystack, $search){ foreach($search as $term){ if(stristr($haystack, $term) !== false){ return true; } } return false; } $arr = array("Bad Dog", "Bad Cat", "Bad Bear", "Nice Bear", "Nice Dog"); $angry_animals = array('bad', 'ugly', 'mad'); foreach($arr as $key => $val){ if (strpos_array($val, $angry_animals)){ echo $val; } } I simply replaced 'strpos' with 'stristr'. The code above echoes out the 'angry_animals'. Thanks again for everyone's help on this one.
-
Ok, so it's likely the browser being sluggish by containing upwards of 40k to 60k lines of code? Nothing I can do except delete then lines every 10k perhaps through js?
-
I have a script that I recently created and it works great...for the first while. I'm trying to figure out different ways to 'optimize' it or otherwise run smoother. My script echo's thousands of lines of code while running through a loop. Firefox, after about an hour, runs so slow to the point of crashing. It is quite obvious - the program doesn't echo as quickly and performance is dramatically reduced. I've edited the memory limit by doing the following: ini_set('memory_limit','16M'); I've 'flushed' the buffer?: ob_flush(); flush(); usleep(500000); And I've also checked how much memory is being used during every 'parse': if ($mem_usage < 1024) echo $mem_usage." bytes"; elseif ($mem_usage < 1048576) echo round($mem_usage/1024,2)." kilobytes"; else echo round($mem_usage/1048576,2)." megabytes"; echo "<br/>"; It averages at about 10megs through every loop. Is there anything else I should be doing to increase the performance? Unloading or unsetting something?
-
Grabbing from <textarea> no problem, but what about a CSS box?
soma56 replied to soma56's topic in PHP Coding Help
Thanks for your reply. I think I thought about this one too much. Assigning the css styles directly to the <textarea> solved it. -
Grabbing from <textarea> no problem, but what about a CSS box?
soma56 replied to soma56's topic in PHP Coding Help
I still can't figure this out. Here's a correction on the original code: <form action="" method="post"> <table width="500"> <tr><td>What's your name?<input name="name" type="text" /></td></tr> <tr><td><input type=Submit value="Enter Name" name="Submit"></td></tr> </table></form> <?PHP echo "<textarea name=\"mytextbox\">"; unset($name); if (isset($_POST['Submit'])) { $name = trim($_POST["name"]); echo $name; } echo "</textarea>"; //Use 'name' for other php functions ?> It seems PHP is able to grab everything from a text box based on the <input name="name" attribute. Does any such element work for div tags in CSS? I have everything styled in CSS with divs for boxes however the only way to use PHP as a means of playing with the output is by grabbing the name within a <textarea>. I tried wrapping a visibility:hidden; within the code but that made everything hidden. I saw somewhere that someone assigned a name to a div tag <div id="mydiv" name="myname" however I wasn't able to use the contents in a similar fashion as a <textarea> I also tried wrapping the textarea within the CSS however that made everything look terrible. -
I'm familiar with getting/using my output from a <textarea> like so: <form action="" method="post"> <table width="500"> <tr><td>What's your name?<input name="name" type="text" /></td></tr> <tr><td><input type=Submit value="Enter Name" name="Submit"></td></tr> </table></form> <?PHP echo "<textarea name=\"mytextbox\">"; unset($name); if (isset($_POST['Submit'])) { echo $name; } echo "</textarea>"; //Use 'name' for other php functions ?> However, can this be done with a CSS box? Here's an example: <style> div#status {position:absolute; overflow:auto; height:250px; background-color:#999; top:230px; font-family:Verdana, Geneva, sans-serif; font-size:10px; color:#333; margin-left:540px; width:240px; border:2px lightblue solid;} </style> <html> <body> <form action="" method="post"> <table width="500"> <tr><td>What's your name?<input name="name" type="text" /></td></tr> <tr><td><input type=Submit value="Enter Name" name="Submit"></td></tr> </table></form> <?PHP echo "<div id=\"status\">"; unset($name); if (isset($_POST['Submit'])) { echo $name; } echo "</div>"; //Use 'status' for other php functions ?>
-
Thanks, removing the table solved it. CSS will work better.
-
I have a page that contains html and php. When the php is called everything beneath it (all the html) disappears. Here's an example: <html> <body> <form action="" method="post"> <table width="500"> <tr><td>What's your name?<input name="name" type="text" /></td></tr> <tr><td><input type=Submit value="Enter Name" name="Submit"></td></tr> <?PHP if (isset($_POST['Submit'])) { echo $name; unset($targetkeyword); } ?> </table></form> <table width="500"> <tr><td>This text is not displayed when and after PHP function is set</td></tr> </table> </body> </html> When the page initially loads I can see everything however once the submit button is clicked everything after the php code is gone - even after the PHP has completed doing it's thing.
-
I figured it out. Here's what did it if anyone is having the same problem: if(!function_exists('parse')){ function parse(){ global $text; //text returned function return_between($string, $start, $stop){ $st = $string; $list = array(); for($i=0;$i<strlen($string);$i++){ $temp = strpos($st, $start); $str = substr($st, $temp+1); $split_here = strpos($str, $stop); $parsed_string = substr($str, 0, $split_here); if($parsed_string == '') break; $st = substr($str, $split_here+1); $list[] = $parsed_string; } return $list; } $temp = return_between($page, '<td class=\'s\'>', '<a'); echo "<br />"; $i = 0; foreach($temp as $match) { $i = $i +1; echo $match . "<br />". PHP_EOL; flush(); ob_flush(); usleep(50000); if ($i == 100) { exit; } } } } parse();
-
I found this code which appears to be a more advanced type of 'preg_match'. <?php function return_between($string, $start, $stop){ $st = $string; $list = array(); for($i=0;$i<strlen($string);$i++){ $temp = strpos($st, $start); $str = substr($st, $temp+1); $split_here = strpos($str, $stop); $parsed_string = substr($str, 0, $split_here); if($parsed_string == '') break; $st = substr($str, $split_here+1); $list[] = $parsed_string; } return $list; } $text = 'This is a %string%, get text %some% text...'; print_r(return_between($text, '%', '%')); ?> My question is if I had a page like this: sample.html <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Sample Page</title> <link href="_css/styles.css" rel="stylesheet" type="text/css" /> </head> <body> <h1>Hello PHP Freaks!</h1> <p>I've recived a lot of help here in the past few months and I greatly appreciate it. I <td class='s'>Highly Recommend <a href="http://www.phpfreaks.com"</a></td> reading the posts in this forum as I have found them to be very informative</p> </body> </html> And I wanted to grab everything between <td class='s'> and <a href=" (This would result with "Highly Recommend") Would I be better off trying to learn 'preg_match' or trying to make it work with the code above? This is the root I'm taking so far to figure this out: <?PHP //Specifies if parse includes the delineator define("EXCL", true); //Get the page contents $page = file_get_contents('sample.html'); //call to return between $infoIneed = return_between($page, "<td class=\'s\'>", "(<a href=\"", EXCL); //separate into a list $data = explode(" ", "$infoIneed"); foreach ($data as $value) if (empty($value)){ var_dump($value); } else { echo $value . "<br />"; } ?>
-
Ok, Arrays are starting to make my head spin now...
soma56 replied to soma56's topic in PHP Coding Help
Thank you Nightslyr. I'm going to have to take a step back and review arrays and classes. I appreciate everyone's help! -
Ok, Arrays are starting to make my head spin now...
soma56 replied to soma56's topic in PHP Coding Help
Thank you. I've looked over the continue function. I can't see how it will help with my problem. <?PHP $buyshoes = 1; $size = 'null'; $manager = 'null'; $pos = true; $ladies = explode("\n", $_POST['ladies']); foreach($ladies as $lady){ foreach($_POST['mall'] as $store){ $size = 'sale'; $manager = 'possibly talking about sale'; $pos = strpos($manager,$sale); if($pos === false) { $buyshoes = $buyshoes +1; echo $buyshoes; $lady--; //This is where I need help (How to remain within first value? $firstlady = function($ContunueShoppingFirstLady);{ } else { //Continue Cycling Through foreach with next lady //Next lady in $ladies array to first $store } } } ?> I don't need to 'continue' but rather remain within the first value or 'array1' while exhausting all the values of 'array2'. I think 'continue' may be the opposite of what I need. If a certain condition exists - my code doesn't remain at the first value but rather goes to the next one. 'value2'. An idiotic metaphorical example: Currently: Group of ladies => Dorothy => Goes to a every store and buys only one pair at sale price Group of ladies => Elaine => Goes to a every store and buys only one pair at sale price Group of ladies => Judy => Goes to a every store and buys only one pair at sale price Ideally: Group of ladies => Dorothy => Goes to a every store and buys every pair at sale price Group of ladies => Elaine => Goes to a every store and buys every pair at sale price Group of ladies => Judy => Goes to a every store and buys every pair at sale price