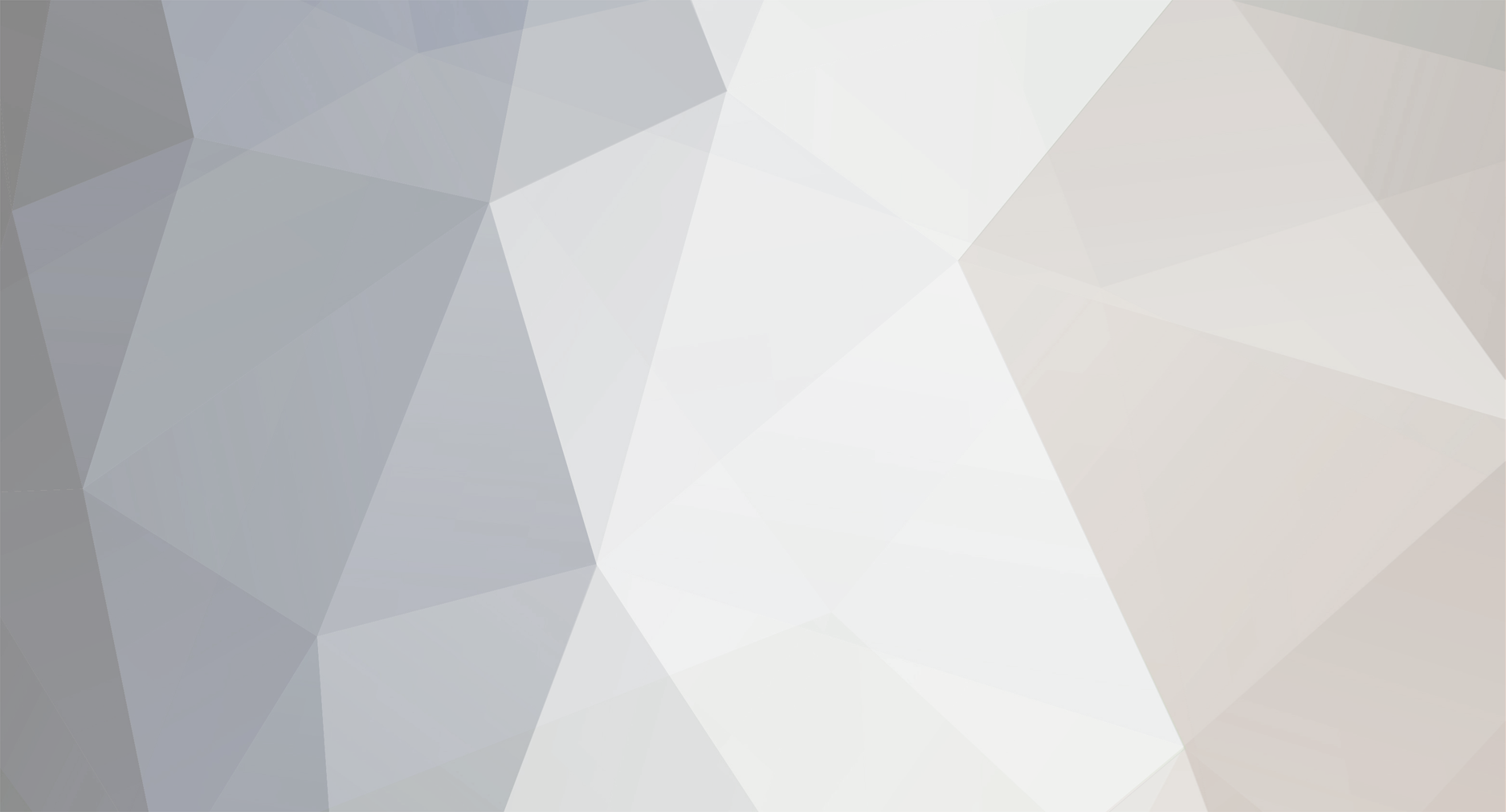
hybmg57
Members-
Posts
52 -
Joined
-
Last visited
Never
Everything posted by hybmg57
-
Hi, Is there an easy way to dynamically add an image in Flash as pre-roll ad for a few seconds? Jae
-
I'm sorry I don't understand what you mean
-
Hi, I have below query which is currently not working properly. It seems like bldgname is not filtering and it comes with all results instead. Is there anything wrong with below? SELECT * FROM idx WHERE 1 AND listprice >=0 AND listprice <=100000000 AND bedrooms >=0 AND baths >=0 AND totintarea >=0 AND landarea >=0 AND highschool LIKE '%Ahuimanu%' AND bldgname LIKE '1040 Kinau' AND catgnum LIKE '1' AND catgnum LIKE '1' OR catgnum LIKE '2' AND catgnum LIKE '1' OR catgnum LIKE '2' OR catgnum LIKE '2' AND catgnum LIKE '1' OR catgnum LIKE '2' OR catgnum LIKE '2' OR catgnum LIKE '4'
-
Hi, I'm trying to get this "NEXT" and "PREVIOUS" button coming out at each end of the loop after page 15 and one before page 1. Is there a way we can do this? I have tried several ways but cannot get this going. Any help would be much appreciated! <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en" xmlns:ice="http://ns.adobe.com/incontextediting"> <head><meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Honolulu Hawaii Real Estate | Honolulu Area Residential Property Search</title> <meta name="description" content="The most complete and comprehensive Honolulu Hawaii real estate search, including homes for sale, properties for sales, condos for sale, new home sales, land for sale in the MLS. Listings of beach real estate by realtors and real estate agents, island real estate, and luxury homes. Complete Hawaii relocation information, community and neighborhood information, real estate resource and library for Hawaii buyers and sellers." /> <meta name="keywords" content="home in honolulu hawaii, honolulu hawaii real estate for sale, homes for sale in honolulu hawaii, hawaii condos, hawaii realtors, hawaii land, hawaii real estate agent, hawaii real estate search, hawaii realty, hawaii condo, hawaii properties, hawaii listings, new homes in hawaii, hawaii mls, hawaii realtor, hawaii houses for sale, hawaii realestate, hawaii homes for sale estate, hawaii beach real estate, hawaii townhomes, hawaii home sales, hawaii townhouse, island real estate, hawaii estate listings, hawaii condos for sale, hawaii brokers real estate, hawaii luxury homes, hawaii listing real estate, hawaii sales real estate, hawaii broker real estate, hawaii home values, hawaii mls estate, hawaii area real estate, hawaii luxury real estate, hawaii homes for sale, buy hawaii real estate, find hawaii real estate, hawaii information real estate, hawaii luxury condos, honolulu real estate, hi real estate, condo real estate, hawaii oceanfront real estate, hawaii condos real estate, oahu real estate, real estate in hawaii, hawaii realty, oahu homes, honolulu hawaii real estate, hawaii mls, condos oahu, hawaii real estate agents, hawaii realtors, kailua real estate, hawaii realtor, oahu real estate, oahu realty, mililani real estate, hawaii real estate market, waikiki real estate, oahu mls, oahu properties, kahala real estate, kaneohe real estate" /> <meta name="abstract" content="Honolulu Hawaii area real estate for sale, Honolulu Hawaii relocation information, Honolulu Hawaii community information, Honolulu Hawaii real estate resources." /> <meta name="page-topic" content="Search Honolulu area residential single family, townhouse, condominium real estate and properties." /> <meta name="classification" content="Honolulu Hawaii real estate, Hawaii real estate, Honolulu real estate, Hawaii homes for sale on Oahu, Honolulu homes for sale on Oahu, Hawaii MLS listings." /> <meta name="robots" content="index, follow" /> <meta name="document-class" content="Living Document" /> <meta name="document-type" content="Public" /> <meta name="distribution" content="Global" /> <meta name="language" content="en-us" /> <meta name="document-rating" content="General" /> <meta name="Author" content="Gabbard Hawaii Properties" /> <meta name="Copyright" content="Copyright © Gabbard Hawaii Properties, All Rights Reserved" /> <LINK REL="SHORTCUT ICON" HREF="/favicon.ico"> <link href="/9style_sub.css" rel="stylesheet" type="text/css" /> <link href="/9layout_sub.css" rel="stylesheet" type="text/css" /> <?php include("db.php"); ?> <script src="/rollover.js" type="text/javascript"></script> <script src="/includes/ice/ice.js" type="text/javascript"></script> <style type="text/css"> <!-- body { background-image: url(/9images/bg3sub.jpg); background-color: #bbbbcc; } a:link { color: #333399; } .style2 {color: #333399} .style5 {color: #666699} --> </style></head> <body id="page1" onload="MM_preloadImages('/9images/m1-act.gif','/9images/m2-act.gif','/9images/m3-act.gif','/9images/m4-act.gif','/9images/m5-act.gif','/9images/m6-act.gif')"><div class="right_bgd"></div> <div class="main"> <div id="header"><form action="/9search_results.html" enctype="application/x-www-form-urlencoded" id="cse-search-box" method="get"> <div class="indent1"><span class="fright">Honolulu Hawaii Real Estate | <a title="Contact Us" onclick="window.open('/info/contactus.html','','width=515,height=590');return false;" href="/info/contactus.html">Contact Us</a>| <a href="../extras/login.html"><span style="font-family: arial,helvetica,sans-serif;">Login</span></a><br /></span><input name="cx" type="hidden" value="005411365982401374147:xhmlagjeh6w" /><input name="cof" type="hidden" value="FORID:9" /><input name="ie" type="hidden" value="UTF-8" /><a title="Home" href="/">Home</a> > <a title="Search" href="/search/overview.html">Search</a></div> </form><a href="/index.html"><img class="logo" src="/9images/logo.gif" alt="Gabbard Hawaii Properties Logo" title="Gabbard Hawaii Properties" width="244" height="92" /></a><br /><a title="Home" onmouseover="MM_swapImage('Image2','','/9images/m1-act.gif',1)" onmouseout="MM_swapImgRestore()" href="/index.html"><img id="Image2" src="/9images/m1.gif" alt="Home" title="Home" height="69" /></a><a title="Start here to find your Hawaii dream home." onmouseover="MM_swapImage('Image3','','/9images/m2-act.gif',1)" onmouseout="MM_swapImgRestore()" href="/search/overview.html"><img id="Image3" src="/9images/m2-act.gif" alt="Search" title="Start here to find your Hawaii dream home." width="150" height="69" /></a><a title="Let us be your guide...we can show you the way." onmouseover="MM_swapImage('Image4','','/9images/m3-act.gif',1)" onmouseout="MM_swapImgRestore()" href="/relocate/overview.html"><img id="Image4" src="/9images/m3.gif" alt="Relocate" title="Let us be your guide...we can show you the way." width="178" height="69" /></a><a title="Explore our communities. Where do you want to live?" onmouseover="MM_swapImage('Image5','','/9images/m4-act.gif',1)" onmouseout="MM_swapImgRestore()" href="/explore/overview.html"><img id="Image5" src="/9images/m4.gif" alt="Explore" title="Explore our communities. Where do you want to live?" width="181" height="69" /></a><a title="Questions? Click for answers." onmouseover="MM_swapImage('Image6','','/9images/m5-act.gif',1)" onmouseout="MM_swapImgRestore()" href="/research/overview.html"><img id="Image6" src="/9images/m5.gif" alt="Research" title="Questions? Click for answers." width="157" height="69" /></a><a title="Our vision, our values, who we are." onmouseover="MM_swapImage('Image7','','/9images/m6-act.gif',1)" onmouseout="MM_swapImgRestore()" href="/meet/overview.html"><img id="Image7" src="/9images/m6.gif" alt="Meet" title="Our vision, our values, who we are." width="158" height="69" /></a><br /> <div class="indent"> <div class="container1"> <div class="col-1"><a title="New Construction" href="/search/newhomes/overview.html"><img src="/search/images/search1.jpg" alt="New Construction" title="New Construction" width="294" height="172" /></a> <div class="block"></div> </div> <div class="col-2"><a title="Financing Information" href="/search/financing/overview.html"><img src="/search/images/search2.jpg" alt="Financing Information" title="Financing Information" width="294" height="172" /></a> <div class="block"></div> </div> <div class="col-3"><a title="Hawaii Market Reports" href="/search/market/overview.html"><img src="/search/images/search3.jpg" alt="Hawaii Market Reports" title="Hawaii Market Reports" width="294" height="172" /></a> <div class="block"></div> </div> <br class="clear" /></div> </div> </div> <div id="content"> <div class="bg-cont png"> <div class="indent-main"> <div class="container"> <div class="col-1"> <div class="box1"> <div class="indent-box1"><a title="Search" href="/search/overview.html"><img src="/search/images/text-search.gif" alt="Search" title="Search" width="225" height="20" /></a> <br /><br /> <ul class="indent-3"> <li><a title="Search O'ahu Island Properties" href="/search/overview.html">Island Property Search</a></li> <li><a title="Search by Building Name"http://www.beautifulhawaiihomes.idxco.com/idx/4745/mapSearch.php">Island Map Search</a></li> <li><a title="Search by Listing Number" href="/search/listingnumber.html">Island Lifestyle Search</a></li> </ul> </div> </div> <div class="indent"> <div class="box"><form action="http://www.beautifulhawaiihomes.idxco.com/idx/4745/userLogin.php" enctype="application/x-www-form-urlencoded" method="post"> <div class="indent-box3"><img class="title1" src="/9images/login_header.gif" alt="Login" title="Login" /> <div class="h1"> <div id="IDX-listingIDsearch" class="IDX-searchBox"> <div id="IDX-listingIDsearchLabel" class="IDX-searchLabel">Email Address</div> <div id="IDX-listingIDsearchInput" class="IDX-searchInput"><input id="IDX-userLoginEmail" style="color: #7f7d7c;" maxlength="255" name="leadEmail" size="30" type="text" value="Email Address:" /> </div> </div> </div> <div class="h1"> <div id="IDX-listingIDsearch" class="IDX-searchBox"> <div id="IDX-listingIDsearchLabel" class="IDX-searchLabel">Password</div> <div id="IDX-listingIDsearchInput" class="IDX-searchInput"><input id="IDX-userLoginPassword" style="color: #7f7d7c;" maxlength="32" name="password" size="30" type="text" value="Password:" /> </div> </div> </div> <div id="IDX-searchReset" class="IDX-searchReset"><input id="IDX-resetButton" type="reset" value="Reset" /> </div> <div id="IDX-searchSubmit" class="IDX-searchInput"><input id="IDX-userLoginSubmit" type="submit" value="Log In" /> </div> </div> </form><br /> Need to Register? <a href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/userSignup.php">Click Here</a><br /><br /></div> </div> <div class="indent"> <div class="box"><input name="stp" type="hidden" value="listingID" /> <div class="indent-box3"><img class="title1" src="/9images/1page_title1a.gif" alt="Quick Search" title="Quick Search" /> <div class="h1"> <ul class="indent-3"> <li><a title="New Single Family Listings" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Residential&ba=0&add=2&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">New Single Family Listings</a></li> <li><a title="New Condominium Listings" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Condo&sfrType[]=Townhouse&ba=0&add=2&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">New Condominium Listings</a></li> <li><a title="Click for $500K Listings" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Residential&sfrType[]=Condo&sfrType[]=Townhouse&lp=500000&hp=500000&ba=0&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">What do you get for $500K?</a></li> <li><a title="Click for $1M Listings" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Residential&sfrType[]=Condo&sfrType[]=Townhouse&lp=1000000&hp=1000000&ba=0&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">What do you get for $1M?</a></li> <li><a title="Click for $5-$6M Listings" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Residential&lp=5000000&hp=6000000&ba=0&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">What do you get for $5M-$6M?</a></li> </ul> </div> </div> <form action="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php" enctype="application/x-www-form-urlencoded" id="form" method="get"> <p><br /><br /><br /><br /></p> <p> </p> </form></div> </div> </div> <div class="indent-colSearch"><br /><img src="/search/images/text-search-results.gif" alt="Property Search" title="Property Search" /></div> <div class="indent-colSearch2"> <div id="rha-mls-pager-container"> <?php $sfrtype = $_GET['sfrType[]']; $showfield = $_GET['showField']; $bycity = $_GET['city'][0]; $byzipcode = intval($_GET['zipCode[]'][0]); $byregion = $_GET['aw_area[]']; if($_GET['lp']!="Min Price" && $_GET['lp']!=NULL){$lp = $_GET['lp'];}else{$lp = intval(0);} if($_GET['hp']!="Max Price" && $_GET['hp']!=NULL){$hp = $_GET['hp'];}else{$hp = intval(100000000);} $landtenure = $_GET['aw_tenure[]']; $beds = intval($_GET['bd']); $bath = intval($_GET['ba']); $sort = $_GET['srt']; $sqft = intval($_GET['sqFt']); $landarea = intval($_GET['kw_landArea']); $numresults = $_GET['per']; $neighbourhood = $_GET['aw_neighborhood[]']; $view = $_GET['aw_view[]']; $schools = $_GET['aw_schools[]']; $building = $_GET['aw_buildingName[]']; $frontage = $_GET['aw_frontage[]']; $pool = $_GET['aw_pool[]']; if(isset($_GET['zipCode'])){ $SEARCH = "WHERE 1 "; foreach ($_GET['zipCode'] as $key1 => $z){if($key1 == 0){$SEARCH .= " AND zip = {$z} ";}else{$SEARCH .= " OR zip = {$z} ";}} } elseif(isset($_GET['city'])){ $SEARCH = "WHERE 1 "; foreach ($_GET['city'] as $key2 => $c){if($key2 == 0){$SEARCH .= " AND city LIKE '%{$c}%' ";}else{$SEARCH .= " OR city LIKE '%{$c}%' ";}} } elseif(isset($_GET['aw_area'])){ $SEARCH = "WHERE 1 "; foreach ($_GET['aw_area'] as $key3 => $r){if($key3 ==0){$SEARCH .= " AND region LIKE '%{$r}%' ";}else{$SEARCH .= " OR region LIKE '%{$r}%' ";}} } else { $SEARCH = "WHERE 1"; } $SEARCH .= " AND listprice >= ".$lp. " AND listprice <= ".$hp; if($_GET['aw_tenure'][0]!=NULL){$SEARCH.=" AND landtenure LIKE '%{$_GET['aw_tenure'][0]}%'";} $SEARCH .= " AND bedrooms >= ".$beds. " AND baths >= ".$bath. " AND totintarea >= ".$sqft. " AND landarea >=".$landarea; if($_GET['aw_neighborhood'] != NULL){ foreach ($_GET['aw_neighborhood'] as $key4 => $n){if($key4 ==0){$SEARCH .= " AND nbrhood LIKE '%{$n}%'";}else{$SEARCH .= " OR nbrhood LIKE '%{$n}%'";}} } if($_GET['aw_view'] != NULL){ foreach ($_GET['aw_view'] as $key5 => $v){if($key5 ==0){$SEARCH .= " AND featstring1 LIKE '%{$v}%'";}else{$SEARCH .= " OR featstring1 LIKE '%{$v}%'";}} } if($_GET['aw_schools'] != NULL){ foreach ($_GET['aw_schools'] as $key6 => $s){if($key6 ==0){$SEARCH .= " AND highschool LIKE '%{$s}%'";}else{$SEARCH .= " OR highschool LIKE '%{$s}%'";}} } if($_GET['aw_buildingName'] != NULL){ foreach ($_GET['aw_buildingName'] as $key7 => $bn){if($key7 ==0){$SEARCH .= " AND bldgname LIKE '%{$bn}%'";}else{$SEARCH .= " OR bldgname LIKE '%{$bn}%'";}} } if($_GET['aw_frontage'] != NULL){ foreach ($_GET['aw_frontage'] as $key8 => $ft){if($key8 ==0){$SEARCH .= " AND featstring1 LIKE '%{$ft}%'";}else{$SEARCH .= " OR featstring1 LIKE '%{$ft}%'";}} } if($_GET['aw_pool'] != NULL){ foreach ($_GET['aw_pool'] as $key9 => $pl){if($key9 ==0){$SEARCH .= " AND featstring7 LIKE '%{$pl}%'";}else{$SEARCH .= " OR featstring7 LIKE '%{$pl}%'";}} } $sort = "listprice"; $sortby = $_GET['srt']; //if($offset != 0){$offset = $_GET['offset']+$_GET['per'];}else{$offset = $_GET['offset'];} $limit = $_GET['per']; $result = mysql_query("SELECT * FROM idx ".$SEARCH." ORDER BY {$sort} {$sortby} LIMIT {$offset}, {$limit}") or die(mysql_error()); mysql_query("SELECT SQL_CALC_FOUND_ROWS * FROM idx ".$SEARCH." ORDER BY {$sort} LIMIT {$offset}, {$limit}"); $total_rows = mysql_query("SELECT FOUND_ROWS()"); $total_found = mysql_fetch_array($total_rows); $pages_count = intval($total_found[0] / $limit); if($total_found[0]%$limit){$pages_count++;} ?> <div> <?php echo $total_found[0]." results found"; echo "<br/>"; echo "<br/>"; if($pages_count <= 1){$onepage = 0;}else{if($pages_count <= 14){$onepage = $pages_count;}else{$onepage = 15;}} for($i=1; $i <= $onepage; $i++){ if($_GET['pg'] == $i){ echo "<b>{$i}</b> "; } else { $url = "<a href='results.php?"; $url .= "pg=".$i."&"; $url .= "offset=".($_GET['per']*($i-1))."&"; if(is_array($_GET['sfrType'])){foreach($_GET['sfrType'] as $sfr){$url .= "sfrType[]=".$sfr."&";}}else{$url .= $_GET['sfrType'];} $url .= "showField=".$_GET['showField']."&"; if(is_array($_GET['city'])){foreach($_GET['city'] as $cities){$url .= "city=".$cities."&";}}else{$url .= $_GET['city'];} $url .= "lp=".$_GET['lp']."&"; $url .= "hp=".$_GET['hp']."&"; if(is_array($_GET['aw_tenure'])){foreach($_GET['aw_tenure'] as $tenure){$url .= "aw_tenure[]=".$tenure."&";}}else{$url .= $_GET['aw_tenure'];} $url .= "bd=".$_GET['bd']."&"; $url .= "ba=".$_GET['ba']."&"; $url .= "srt=".$_GET['srt']."&"; $url .= "sqFt=".$_GET['sqFt']."&"; $url .= "kw_landArea=".$_GET['kw_landarea']."&"; $url .= "per=".$_GET['per']."&"; $url .= "'>{$i}</a> "; echo $url; if($i == 1){ $url1 = "<a href='results.php?"; $url1 .= "pg=".($i+14)."&"; $url1 .= "offset=".($_GET['per']*($i+14))."&"; if(is_array($_GET['sfrType'])){foreach($_GET['sfrType'] as $sfr){$url1 .= "sfrType[]=".$sfr."&";}}else{$url1 .= $_GET['sfrType'];} $url1 .= "showField=".$_GET['showField']."&"; if(is_array($_GET['city'])){foreach($_GET['city'] as $cities){$url1 .= "city=".$cities."&";}}else{$url1 .= $_GET['city'];} $url1 .= "lp=".$_GET['lp']."&"; $url1 .= "hp=".$_GET['hp']."&"; if(is_array($_GET['aw_tenure'])){foreach($_GET['aw_tenure'] as $tenure){$url1 .= "aw_tenure[]=".$tenure."&";}}else{$url1 .= $_GET['aw_tenure'];} $url1 .= "bd=".$_GET['bd']."&"; $url1 .= "ba=".$_GET['ba']."&"; $url1 .= "srt=".$_GET['srt']."&"; $url1 .= "sqFt=".$_GET['sqFt']."&"; $url1 .= "kw_landArea=".$_GET['kw_landarea']."&"; $url1 .= "per=".$_GET['per']."&"; $url1 .= "'>NEXT</a> "; } if($i == 15){echo $url1;} } } echo " "; echo ($pgnum+1)." out of ".($pages_count+1)." pages"; ?> <br /> </div> <tr><td><!-- ################################################### --> <?php while ($row = mysql_fetch_array($result)){ ?> <table width="520" cellspacing="0" cellpadding="0" border="0" align="center"> <tbody><tr><td height="2" colspan="6"><hr size="1"></td></tr> <tr> <td width="32%" rowspan="3"><a href=<?php echo "detailed_results.php?listnum=".$row['listnum']; ?>><img width="169" height="134" border="0" alt=<?php echo $row['listnum']; ?> src=<?php if(file_exists("ftp://RUThGabb:Ruth@gh77@idxftp.hicentralmls.com/IDX/PhotosAll/".substr($row['listnum'],-1)."/".$row['listnum']."_0.jpg")){ $image1 = "ftp://RUThGabb:Ruth@gh77@idxftp.hicentralmls.com/IDX/PhotosAll/".substr($row['listnum'],-1)."/".$row['listnum']."_0.jpg"; echo $image1; }elseif(file_exists("ftp://RUThGabb:Ruth@gh77@idxftp.hicentralmls.com/IDX/PhotosAll/".substr($row['listnum'],-1)."/".$row['listnum']."_1.jpg")){ $image1 = "ftp://RUThGabb:Ruth@gh77@idxftp.hicentralmls.com/IDX/PhotosAll/".substr($row['listnum'],-1)."/".$row['listnum']."_1.jpg"; echo $image1; }elseif(file_exists("ftp://RUThGabb:Ruth@gh77@idxftp.hicentralmls.com/IDX/PhotosAll/".substr($row['listnum'],-1)."/".$row['listnum']."_2.jpg")){ $image1 = "ftp://RUThGabb:Ruth@gh77@idxftp.hicentralmls.com/IDX/PhotosAll/".substr($row['listnum'],-1)."/".$row['listnum']."_2.jpg"; echo $image1; }else{ echo "../images/NoPhoto.jpg"; } ?>></a></td> <td width="4%" rowspan="3"><a href="register.php?url=featured.php%3Flistnum%3D2101425%26amp%3Bproptype%3Dres"></a></td> <td height="27" align="left" class="thumbnailhead" colspan="3"> <a href=<?php echo "detailed_results.php?listnum=".$row['listnum']; ?>><?php echo $row['streetnum']." ".$row['streetname'].", ".$row['city']." ".$row['state'].", ".$row['zip']; ?></a></td> <td align="right" class="thumbnailhead"> <a href=<?php echo "detailed_results.php?listnum=".$row['listnum']; ?>><?php echo "$".number_format($row['listprice']); ?></a></td></tr> <tr> <td width="17%" height="38" class="thumbnailbody"><?php echo $row['bedrooms']." Bedrooms"; ?></td> <td width="15%" height="38" class="thumbnailbody"><?php echo $row['baths']." Baths"; ?></td> <td width="21%" height="38" class="thumbnailbody">MLS#: <?php echo $row['listnum']; ?></td> </tr> <tr> <td width="17%" valign="top" height="25" class="thumbnailbody"><?php echo $row['landarea']; ?> SqFt AC</td> <td width="15%" valign="top" height="25" class="thumbnailbody">Built: <?php echo $row['yearbuilt']; ?> </td> <td width="21%" valign="top" height="26" style="color: rgb(0, 102, 204);" class="thumbnailbody"><?php if($row['landtenure']=="FS"){echo "Fee Simple";}elseif($row['landtenure']=="LH"){echo "Leasehold";} ?></td> </tr> <tr><td colspan="5" class="thumbnailbody"></td></tr> </tbody></table><br/> <?php } ?> <!-- ################################################### --></td></tr> </div> <div class="indent-box4"> </div> </div> </div> <table style="margin: 0px auto; width: 875px; height: 10px; text-align: center;" border="0"> <tbody> <tr> <td style="height: 60px;"> </td> </tr> <tr> <td> <p><img src="/info/images/mininavigation.jpg" alt="" width="875" height="15" /></p> <table style="margin: 0px auto; width: 800px; height: 10px; text-align: center;" border="0"> <tbody> <tr> <td style="width: 195px; height: 0px;" valign="top"> <p style="text-align: left;"><img src="/info/images/text-info-address.gif" alt="Gabbard Hawaii Properties" title="Gabbard Hawaii Properties" width="150" height="71" /><br /><br /><span style="font-family: arial,helvetica,sans-serif;"> 808-534-1850 Telephone<br /> 877-ALOHA HI (256-4244)<br /></span> <a title="beautifulhawaiihomes.com" href="/"><span style="font-family: arial,helvetica,sans-serif;">beautifulhawaiihomes.com</span></a><br /><br /> <a title="Contact Us" onclick="window.open('/info/contactus.html','','width=515,height=590');return false;" href="/info/contactus.html"><span style="font-family: arial,helvetica,sans-serif;">Contact Us</span></a><br /> <a title="Send a Referral" href="/kino/referrals.html" target="_blank"><span style="font-family: arial,helvetica,sans-serif;">Send a Referral</span></a><br /> <a title="Read Our Honolulu Real Estate Blog" href="/aloha"><span style="font-family: arial,helvetica,sans-serif;">Read Our Blog</span></a><br /><br /></p> </td> <td style="width: 225px; height: 0px;" valign="top"> <p style="text-align: left;"><span style="font-family: georgia,palatino;"><strong>SEARCH NAVIGATION<br /></strong></span><a title="Search" href="/search/overview.html">Search</a><br /><br /><a title="Search Residential Listings" href="/search/overview.html">Search Residential Listings</a> <br /><a title="Search Lots and Land Listings" href="/search/land.html">Search Lots and Land Listings</a><br /><a title="Search Multifamily Listings" href="/search/multi.html">Search Multifamily Listings</a> <br /><a title="Search by Building Name" href="/search/building.html">Search by Building Name</a><br /><a title="Search by Listing Number" href="/search/listingnumber.html">Search by Listing Number</a><br /><a title="Search by Map" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/mapSearch.php">Search by Map</a><br /><a title="Search by Property Address" href="/search/address.html">Search by Property Address</a><br /><br /><a title="Need to register?" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/userSignup.php">Need to Register?</a><br /><br /><a title="New Single Family Listings" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Residential&ba=0&add=2&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">New Single Family Listings</a><br /><a title="New Condominium Listings" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Condo&sfrType[]=Townhouse&ba=0&add=2&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">New Condominium Listings</a><br /><a title="What do you get for $500K?" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Residential&sfrType[]=Condo&sfrType[]=Townhouse&lp=500000&hp=500000&ba=0&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">What do you get for $500K?</a><br /><a title="What do you get for $1M?" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Residential&sfrType[]=Condo&sfrType[]=Townhouse&lp=1000000&hp=1000000&ba=0&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">What do you get for $1M?</a><br /><a title="What do you get for $5M-$6M?" href="http://www.beautifulhawaiihomes.idxco.com/idx/4745/results.php?stp=advanced&pt=sfr&idxID=105&sfrType[]=Residential&lp=5000000&hp=6000000&ba=0&srt=ASC&aw_area[]=Central&aw_area[]=DiamondHd&aw_area[]=Ewaplain&aw_area[]=HawaiiKai&aw_area[]=Kailua&aw_area[]=Kaneohe&aw_area[]=Leeward&aw_area[]=Makakilo&aw_area[]=Metro&aw_area[]=Norshore&aw_area[]=PearlCity&aw_area[]=Waipahu">What do you get for $5M-$6M?</a><br /><br /><a title="New Single Family Developments" href="/search/newhomes/overview.html"><span style="font-family: arial,helvetica,sans-serif;">New Single Family Developments</span></a><br /><a title="New Townhome Developments" href="/search/newhomes/townhomes.html"><span style="font-family: arial,helvetica,sans-serif;">New Townhome Developments</span></a><br /><a title="New Condominium Developments" href="/search/newhomes/condos.html"><span style="font-family: arial,helvetica,sans-serif;">New Condominium Developments</span></a></p> </td> <td style="width: 265px; height: 0px;" valign="top"> <p style="text-align: left;"><span style="font-family: georgia,palatino;"><strong>SEARCH NAVIGATION<br />CONTINUED<br /></strong><a title="Financing Information" href="/search/financing/overview.html"><span style="font-family: arial,helvetica,sans-serif;">Financing Information</span></a><br /><a title="15 Must Ask Lender Questions" href="/search/financing/lenderq.html"><span style="font-family: arial,helvetica,sans-serif;">15 Must Ask Lender Questions</span></a><br /><span style="font-family: arial,helvetica,sans-serif;"><a title="Interest Rates & Calculators" href="/search/financing/ratescalculators.html">Interest Rates & Calculators<br /></a></span><br /><span style="font-family: arial,helvetica,sans-serif;"><a title="Hawaii Market Reports" href="/search/market/overview.html">Hawaii Market Reports</a><br /><a title="HBR Market Press Release" href="/search/market/pdf/current/marketpressrelease.pdf" target="_blank">HBR Market Press Release</a><br /><a title="O'ahu Closed and Pending Sales" href="/search/market/pdf/current/closedpendingsales.pdf" target="_blank">O'ahu Closed and Pending Sales</a><br /><a title="O'ahu Median and Average Sale Prices" href="/search/market/pdf/current/medianaverage.pdf" target="_blank">O'ahu Median and Average Sale Prices</a><br /><a title="O'ahu List Price to Sales Price Ratio" href="/search/market/pdf/current/listpriceratio.pdf" target="_blank">O'ahu List Price to Sales Price Ratio</a><br /><a title="O'ahu Days on Market (DOM) to Sale" href="/search/market/pdf/current/dom.pdf" target="_blank">O'ahu Days on Market (DOM) to Sale</a><br /><a title="O'ahu New Listings on the Market " href="/search/market/pdf/current/newlistings.pdf" target="_blank">O'ahu New Listings on the Market</a><br /><a title="O'ahu Market Overview" href="/search/market/pdf/current/marketoverview.pdf" target="_blank">O'ahu Market Overview</a><br /><a title="O'ahu Median Sale Prices v. Affordability" href="/search/market/pdf/affordability.pdf" target="_blank">O'ahu Median Sale Prices v. Affordability</a><br /><a title="Neighborhood Median Price Comparisons" href="/search/market/pdf/annualneighborhodcomparisons.pdf" target="_blank">Neighborhood Median Price Comparisons</a><br /><a title="O'ahu Median Sale Prices" href="/search/market/saleprices.html">O'ahu Median Sale Prices<br /></a></span></span><span style="font-family: georgia,palatino;"><a title="O'ahu Annual Median Sales Price History " href="/search/market/pdf/annualmedian.pdf" target="_blank"><span style="font-family: arial,helvetica,sans-serif;">O'ahu Annual Median Sale Price History</span><br /><span style="font-family: arial,helvetica,sans-serif;">O'ahu Sales Volume History</span></a></span></p> </td> <td style="width: 115px; height: 0px;" valign="top"> <p style="text-align: left;"><span style="font-family: georgia,palatino;"><strong>MAIN MENU<br /></strong><a title="Home" href="/index.html"><span style="font-family: arial,helvetica,sans-serif;">Home</span></a><br /><a title="Search" href="/search/overview.html"><span style="font-family: arial,helvetica,sans-serif;">Search</span></a><br /><a title="Relocate" href="/relocate/overview.html"><span style="font-family: arial,helvetica,sans-serif;">Relocate</span></a><br /><a title="Explore" href="/explore/overview.html"><span style="font-family: arial,helvetica,sans-serif;">Explore</span></a><span style="font-family: arial,helvetica,sans-serif;"> <br /></span><a title="Research" href="/research/overview.html"><span style="font-family: arial,helvetica,sans-serif;">Research</span></a><br /><a title="Meet" href="/meet/overview.html"><span style="font-family: arial,helvetica,sans-serif;">Meet</span></a><br /><br /><br /><span style="font-size: 12px;"><strong>SITE INFO</strong></span><br /><a title="Terms of Use" href="/info/terms.html"><span style="font-family: arial,helvetica,sans-serif;">Terms of Use </span></a><br /><a title="Privacy Policies" href="/info/privacy.html"><span style="font-family: arial,helvetica,sans-serif;">Privacy Policies</span></a><br /><a title="Site Maps" href="/info/sitemaps.html"><span style="font-family: arial,helvetica,sans-serif;">Site Maps</span></a></span></p> </td> </tr> <tr> <td colspan=4> Based on the information from the Multiple Listing Service of the Multiple Listing Service of HiCentral MLS, Ltd. Active listings last updated on <?php echo date("m/d/Y"); ?>. Information is deemed reliable but not guaranteed. Copyright: 2010 by HiCentral MLS, Ltd. IDX information is provided exclusively for consumers' personal, non-commercial use, and may not be used for any purpose other than to identify prospective properties consumers may be interested in purchasing. Information is based on data available to the associate, including county records. The information has not been verified by the associate and should be verified by the buyer. Some of the active listings appearing on this site may be listed by other REALTORS®. If you are interested in those active listings, our company may represent you as the buyer's agent. If the active listing you are interested in is our company’s active listing, you may speak to one of our agents regarding your options for representation. Hawaii and Kauai properties displayed courtesy of Hawaii Information Service. </td> </tr> </tbody> </table> </td> </tr> </tbody> </table> </div> </div> <div id="footer"> <div class="indent-footer"><span class="style5">© Gabbard Hawaii Properties. All rights reserved.</span> <span class="style5">|</span> <a title="Terms of Use" href="/info/terms.html">Terms</a> <span class="style5">| </span><a title="Privacy Policies" href="/info/privacy.html">Privacy</a><span class="style5"> |</span> <a title="Site Maps" href="/info/sitemaps.html">Site Maps</a></div> </div> </div> <script type="text/javascript">// <![CDATA[ var gaJsHost = (("https:" == document.location.protocol) ? "https://ssl." : "http://www."); document.write(unescape("%3Cscript src='" + gaJsHost + "google-analytics.com/ga.js' type='text/javascript'%3E%3C/script%3E")); // ]]></script> <script type="text/javascript">// <![CDATA[ try { var pageTracker = _gat._getTracker("UA-1018066-2"); pageTracker._trackPageview(); } catch(err) {} // ]]></script> <script type="text/javascript">// <![CDATA[ //Disable right mouse click Script var message="Kapu! © Gabbard Hawaii Properties. All rights reserved."; /////////////////////////////////// function clickIE4(){ if (event.button==2){ alert(message); return false; } } function clickNS4(e){ if (document.layers||document.getElementById&&!document.all){ if (e.which==2||e.which==3){ alert(message); return false; } } } if (document.layers){ document.captureEvents(Event.MOUSEDOWN); document.onmousedown=clickNS4; } else if (document.all&&!document.getElementById){ document.onmousedown=clickIE4; } document.oncontextmenu=new Function("alert(message);return false") // ]]></script> <div></div><!-- com.omniupdate.button --><div style="position:absolute; left: 0px; bottom: 0px;"><a href="http://www.omniupdate.com/ox/de.jsp?user=rgabbard&site=AlohaMLS&path=%2Fsearch%2Foverview.html" target="_top"><img src="http://www.beautifulhawaiihomes.com/z-omniupdate/dired.gif" style="border:0px;" width="36px" height="36px" alt="omniupdate" /></a></div><!-- /com.omniupdate.button --></body> </html>
-
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Untitled Document</title> <script src="SpryAssets/SpryValidationPassword.js" type="text/javascript"></script> <script src="SpryAssets/SpryValidationTextField.js" type="text/javascript"></script> <link href="SpryAssets/SpryValidationPassword.css" rel="stylesheet" type="text/css" /> <link href="SpryAssets/SpryValidationTextField.css" rel="stylesheet" type="text/css" /> </head> <?php phpinfo(); include('db.php'); include('functions.php'); if(isset($_POST['Submit'])){ $user = strval(trim(mysql_prep($_POST['user']))); $search = mysql_query("SELECT user FROM hawaii_mls.users WHERE user = '{$user}'"); if(mysql_num_rows($search) == 1){ echo "User already registered"; ?> <body> <form id="registration" name="registration" method="post" action=<?php echo $_SERVER['PHP_SELF']; ?>> <table width="200" border="1"> <tr> <td><label for="user">User: </label></td> <td><span id="sprytextfield1"><input type="text" name="user" id="user" /><span class="textfieldRequiredMsg">A value is required.</span></span></td> </tr> <tr> <td><label for="password">Password: </label></td> <td><span id="sprypassword1"><input type="password" name="password" id="password" /> <span class="passwordRequiredMsg">A value is required.</span></span></td> </tr> <tr> <td><label for="email">Email: </label></td> <td><span id="sprytextfield2"> <input type="text" name="email" id="email" /> <span class="textfieldRequiredMsg">A value is required.</span></span></td> </tr> <tr> <td> </td> <td><input name="Submit" type="submit" value="Submit" /></td> </tr> </table> </form> <script type="text/javascript"> var sprypassword1 = new Spry.Widget.ValidationPassword("sprypassword1"); var sprytextfield1 = new Spry.Widget.ValidationTextField("sprytextfield1"); var sprytextfield2 = new Spry.Widget.ValidationTextField("sprytextfield2"); </script> </body> <?php } else { mysql_query("INSERT INTO hawaii_mls.users ( user , password , email ) VALUES ( '{$_POST['user']}', '{$_POST['password']}', '{$_POST['email']}' ); ") or die(mysql_error()); echo "User registration complete!"; } } ?> </html> This is the code for the entire page. Please have a look. Thank you. Kind regards, Jae
-
Hi, I have done phpinfo() please have a look at the site again. Thank you. Jae
-
I have a website that PHP is not showing. http://www.beautifulhawaiihomes.com/extras/registration.php Please have a look I'm not sure if it's something to do with PHP.ini username: jae password: 1234 Thank you. Kind regards, Jae
-
Is there anything wrong with below code? It was working fine 2 hours ago but after a few changes it's not retrieving data anymore it's just giving the entire data without filtering... Any ideas??? $result = mysql_query("SELECT * FROM residential WHERE PROPSUBTYPEDISPLAY LIKE '%{$Property_Type}%' AND AREA {$Any_City} {$City} AND BEDS >={$Beds} AND BATHSTOTAL >={$Baths} AND LISTPRICE >={$Lowest_Price} AND LISTPRICE {$Any} {$Highest_Price} AND CITY LIKE '%{$CityA}%' AND STREETNAME LIKE '%{$AddressA}%' AND ZIPCODE {$Any_Zip} '{$Zip_codeA}' AND SUBDIVISION LIKE '%{$SubdivisionA}%' AND GARAGECAP >= {$GarageA} AND STORIES >= {$StoriesA} AND FIREPLACES >= {$FireplacesA} AND LOTSIZE {$Yard} AND SCHOOLDISTRICT LIKE '%{$School_districtA}%' AND SCHOOLNAME1 LIKE '%{$School_nameA}%' AND SCHOOLNAME2 LIKE '%{$School_nameA}%' AND SCHOOLNAME3 LIKE '%{$School_nameA}%' AND SCHOOLTYPE1 {$E} AND SCHOOLTYPE2 {$M} AND SCHOOLTYPE3 {$H} AND EXTERIOR {$Guest_quartersA} AND HOUSINGTYPE {$High_riseA} AND INTERIOR {$Loft_A} AND EXTERIOR {$BalconyA} AND ROOMOTHER {$LibraryA} AND ROOMOTHER {$MediaA} AND COMMONFEATURES {$Gated_communityA} AND COMMONFEATURES {$Golf_course_communityA} AND COMMONFEATURES {$Golf_course_lotA} AND COMMONFEATURES {$Lake_front_lotA} AND COMMONFEATURES {$Lake_viewA} {$CitiesA0} {$CitiesA1} {$CitiesA2} {$CitiesA3} {$CitiesA4} {$CitiesA5} {$CitiesA6} {$CitiesA7} {$CitiesA8} {$CitiesA9} {$CitiesA10} {$AreasA0} {$AreasA1} {$AreasA2} {$AreasA3} {$AreasA4} {$AreasA5} {$AreasA6} {$AreasA7} {$AreasA8} {$AreasA9} {$AreasA10} {$CountiesA0} {$CountiesA1} {$CountiesA2} {$CountiesA3} {$CountiesA4} {$CountiesA5} {$CountiesA6} {$CountiesA7} {$CountiesA8} {$CountiesA9} {$CountiesA10} AND PHOTOCOUNT {$Properties_with_photosA} ORDER BY {$sort} LIMIT {$offset}, {$limit}") or die(mysql_error());
-
The error I'm getting is "You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'ORDER BY MODIFIED ASC LIMIT 0, 20' at line 24" Thank you.
-
Hi, I currently have a hard time passing checkbox variables (School Types elementary, middle, high) from a search page to results page. I tried to put some if statements to adjust MySQL commands but it's not working well... Anyone has any ideas? $Property_Type=$_GET['property_type']; $City=$_GET['city']; $Beds=$_GET['beds']; $Baths=$_GET['baths']; $Lowest_Price=$_GET['lowest_price']; $Highest_Price=$_GET['highest_price']; $MLS=$_GET['mls_number']; $CityA=$_GET['citya']; $AddressA=$_GET['address']; $Zip_codeA=$_GET['zip_code']; $SubdivisionA=$_GET['subdivision']; $GarageA=$_GET['garage']; $StoriesA=$_GET['stories']; $FireplacesA=$_GET['fireplaces']; $Yard_sizeA=$_GET['yard_size']; $CitiesA=$_GET['cities']; $AreasA=$_GET['areas']; $CountiesA=$_GET['counties']; $School_districtA=$_GET['school_district']; $School_type_elementaryA=$_GET['school_type']; $School_type_middleA=$_GET['school_type']; $School_type_highA=$_GET['school_type']; $School_nameA=$_GET['school_name']; $E = $School_type_elementaryA['elementary'][0]; $M = $School_type_middleA['middle'][0]; $H = $School_type_highA['high'][0]; if ($E == "E" || $M == "M" || $H == "H"){ if ($E == "E" && $M == "M" && $H == "H"){ $School_type = "SCHOOLTYPE1 LIKE 'E' AND SCHOOLTYPE2 LIKE 'M' AND SCHOOLTYPE3 LIKE 'H'"; } if ($E == "E" && $M == "M" && empty($_GET['school_type']['high'])){ $School_type = "SCHOOLTYPE1 LIKE 'E' AND SCHOOLTYPE2 LIKE 'M'"; } if (empty($_GET['school_type']['elementary']) && $M == "M" && $H == "H"){ $School_type = "SCHOOLTYPE2 LIKE 'M' AND SCHOOLTYPE3 LIKE 'H'"; } if ($E == "E" && empty($_GET['school_type']['middle']) && $H == "H"){ $School_type = "SCHOOLTYPE1 LIKE 'E' AND SCHOOLTYPE3 LIKE 'H'"; } if ($E == "E" && $M == NULL && $H == NULL){ $School_type = "SCHOOLTYPE1 LIKE 'E'"; } if ($M == "M"&& $E == NULL && $H == NULL){ $School_type = "SCHOOLTYPE2 LIKE 'M'"; } if ($H == "H"&& $E == NULL && $M == NULL){ $School_type = "SCHOOLTYPE3 LIKE 'H'"; } $School_type = "SCHOOLTYPE3 LIKE 'H'"; $result = mysql_query("SELECT * FROM residential WHERE PROPSUBTYPEDISPLAY LIKE '%{$Property_Type}%' AND AREA {$Any_City} {$City} AND BEDS >={$Beds} AND BATHSTOTAL >={$Baths} AND LISTPRICE >={$Lowest_Price} AND LISTPRICE {$Any} {$Highest_Price} AND CITY LIKE '%{$CityA}%' AND STREETNAME LIKE '%{$AddressA}%' AND ZIPCODE {$Any_Zip} '{$Zip_codeA}' AND SUBDIVISION LIKE '%{$SubdivisionA}%' AND GARAGECAP >= {$GarageA} AND STORIES >= {$StoriesA} AND FIREPLACES >= {$FireplacesA} AND LOTSIZE {$Yard} AND SCHOOLDISTRICT LIKE '%{$School_districtA}%' AND SCHOOLNAME1 LIKE '%{$School_nameA}%' AND SCHOOLNAME2 LIKE '%{$School_nameA}%' AND SCHOOLNAME3 LIKE '%{$School_nameA}%' AND {$School_type} ORDER BY {$sort} LIMIT {$offset}, {$limit}") or die(mysql_error());
-
I still seem to have problems, is there any other advice out there please???
-
Hi, thanks for your reply. It seems like I'm having an issue with parsing something must be one of below... Please have a look at this link and do a search... http://test1.favstay.com/Advanced_Search.php Thank you.
-
Hi I have a search form that I created and trying to parse info entered from advanced search form to the search results page and I get this error... You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'AND BEDS >=0 AND BATHSTOTAL >=0 AND LISTPRICE >=0 AND LI' at line 5 Here is the code for the search results page... <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en" dir="ltr"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>JR Molina</title> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <meta name="robots" content="index,follow" /> <link rel="shortcut icon" href="/favicon.ico" type="image/x-icon" /> <link rel="apple-touch-icon" href="/apple-touch-icon.png"/> <link charset="utf-8" type="text/css" rel="stylesheet" media="all" href="results.css" /> <?php if (is_numeric($_GET['pg'])) { $pgnum = ((int) $_GET['pg']); } else { $pgnum = 0; $errorMessage = "Invalid value for page number"; } $startnum = 1; $nextpg = ($pgnum + 1); $prevpg = ($pgnum-1); if ($pgnum >= 0) { $offset = ($pgnum * 20); } else { $offset = 0; } $limit = 20; ?> <?php $Property_Type=$_GET['property_type']; $City=$_GET['city']; $Beds=$_GET['beds']; $Baths=$_GET['baths']; $Lowest_Price=$_GET['lowest_price']; $Highest_Price=$_GET['highest_price']; $MLS=$_GET['mls_number']; $AddressA=$_GET['address']; $Zip_codeA=$_GET['zip_code']; $SubdivisionA=$_GET['subdivision']; $GarageA=$_GET['garage']; $StoriesA=$_GET['stories']; $FireplacesA=$_GET['fireplaces']; $Yard_sizeA=$_GET['yard_size']; $CitiesA=$_GET['cities']; $AreasA=$_GET['areas']; $CountiesA=$_GET['counties']; $School_districtA=$_GET['school_district']; $School_elementaryA=$_GET['school_type[elementary]']; $School_middleA=$_GET['school_type[middle]']; $School_highA=$_GET['school_type[high]']; $School_nameA=$_GET['school_name']; $Guest_quartersA=$_GET['property[guest-quarters]']; $High_riseA=$_GET['property[high-rise-condominium]']; $Loft_A=$_GET['property[loft]']; $BalconyA=$_GET['property[balcony]']; $LibraryA=$_GET['property[library-or-study]']; $MediaA=$_GET['property[media-room]']; $Gated_communityA=$_GET['location[gated-community]']; $Golf_course_communityA=$_GET['location[golf-course-community]']; $Golf_course_lotA=$_GET['location[golf-course-lot]']; $Lake_front_lotA=$_GET['location[lake-front-lot]']; $Lake_viewA=$_GET['location[lake-view]']; $Handicap_amenitiesA=$_GET['location[handicap-ammenities]']; $Zero_lot_lineA=$_GET['location[zero-lot-line]']; $Horses_allowedA=$_GET['location[horses-allowed]']; $Fence_woodA=$_GET['fence[wood]']; $Fence_ironA=$_GET['fence[iron]']; $Fence_brickA=$_GET['fence[brick]']; $Fence_rockA=$_GET['fence[rock]']; $Fence_automatic_gateA=$_GET['fence[automatic-gate]']; $Fence_noneA=$_GET['fence[none]']; $Special_requirementsA=$_GET['special_requirements']; $Properties_with_photosA=$_GET['properties_with[photos]']; $Single_detachedA=$_GET['property_types[single-detached]']; $MultiA=$_GET['property_types[multi]']; $TownhomeA=$_GET['property_types[townhome]']; $CondoA=$_GET['property_types[condo]']; $Half_duplexA=$_GET['property_types[half-duplex]']; $LeaseA=$_GET['property_types[lease]']; $Joseph_molinaA=$_GET['only[joseph-molina-properties]']; $Open_housesA=$_GET['only[open-houses]']; include("db.php"); $rets_login_url = "http://ntreisrets.mls.ntreis.net:80/rets/login"; $rets_username = "j_Kp$06$"; $rets_password = "$33D@E862"; $rets_agent = "JKP"; require_once('phRets.php'); $rets = new phRets; $connect = $rets->Connect($rets_login_url, $rets_username, $rets_password); if (!$connect) { print_r($rets->Error()); } ?> <body id="jrmolina-listing-detail" class="our-listings our-listings-landing" text> <div id="page"> <div id="content-container"> <div id="content" class="clearfix"> <div id="container"> <form action="<?php 'Search Results.php?op=Begin+Search&pg=' . 0 . '&property_Type='.urlencode($Property_Type).'&city='.$City.'&beds='.$Beds.'&baths='.$Baths.'&lowest_price='.$Lowest_Price.'&highest_price='.$Highest_Price; ?>" method="post" id="rha-mls-our-listings-sort"> <div id="rha-mls-our-listings-sort-container"> <label for="edit-sort-by" id="for-edit-sort-by"><span>Sort By</span> <select name="sort_by" class="form-select" id="edit-sort-by" ><option value="none">Choose one</option><option value="price-high-to-low">Price Highest to Lowest</option><option value="price-low-to-high">Price Lowest to Highest</option><option value="sqft-high-to-low">Size Largest to Smallest</option><option value="sqft-low-to-high">Size Smallest to Largest</option><option value="street-name-alpha">Street Name</option><option value="zip-code-numeric">Zip Code</option></select></label> <input type="submit" name="op" id="edit-submit" value="Go" class="form-submit" /> <input type="submit" name="op" id="edit-reset" value="Reset" class="form-submit" /> </div><!--/#rha-mls-our-listings-sort-container--> </form><!--/#rha-mls-our-listings-sort--> <input type="hidden" name="redirect" id="edit-redirect" value="/our-listings" /> <input type="hidden" name="page_count" id="edit-page-count" value="140" /> <input type="hidden" name="form_build_id" id="form-524b5209ac82045a8e3c250785a6e75b" value="form-524b5209ac82045a8e3c250785a6e75b" /> <input type="hidden" name="form_id" id="edit-rha-mls-pager" value="rha_mls_pager" /> <div id="listings-container"> <div id="listings"> <?php if(isset($_GET['sort'])){ $sort=$_GET['sort']; } else { $sort = "MODIFIED ASC"; } switch($_POST['sort_by']){ case "none": $sort = "MODIFIED ASC"; break; case "price-high-to-low": $sort = "LISTPRICE DESC"; break; case "price-low-to-high": $sort = "LISTPRICE ASC"; break; case "sqft-low-to-high": $sort = "SQFTTOTAL ASC"; break; case "sqft-high-to-low": $sort = "SQFTTOTAL DESC"; break; case "street-name-alpha": $sort = "STREETNAME ASC"; break; case "zip-code-numeric": $sort = "ZIPCODE ASC"; break; } if($Highest_Price==0 || $Highest_Price=="no_limit"){ if($Highest_Price=="no_limit"){$Highest_Price="5000000";} $Any= ">="; } else { $Any= "<="; } if($City==0){ $Any_City= ">"; } else { $Any_City= "LIKE"; } if($MLS==NULL){ $Any_MLS= "IS NOT"; } else { $Any_MLS= "="; } $result = mysql_query("SELECT * FROM residential WHERE PROPSUBTYPEDISPLAY LIKE '%{$Property_Type}%' AND AREA {$Any_City} {$City} AND BEDS >={$Beds} AND BATHSTOTAL >={$Baths} AND LISTPRICE >={$Lowest_Price} AND LISTPRICE {$Any} {$Highest_Price} ORDER BY {$sort} LIMIT {$offset}, {$limit}") or die(mysql_error()); ?> <div id="rha-mls-pager-container"> <?php mysql_query("SELECT SQL_CALC_FOUND_ROWS * FROM residential WHERE PROPSUBTYPEDISPLAY LIKE '%{$Property_Type}%' AND AREA {$Any_City} {$City} AND BEDS >={$Beds} AND BATHSTOTAL >={$Baths} AND LISTPRICE >={$Lowest_Price} AND LISTPRICE {$Any} {$Highest_Price} ORDER BY {$sort} LIMIT {$offset}, {$limit}"); $total_rows = mysql_query("SELECT FOUND_ROWS()"); $total_found = mysql_fetch_array($total_rows); $pages_count= $total_found[0] / $limit; $num_pages=intval($pages_count); echo $total_found[0]." results found"; echo "<br/>"; if ($pgnum > 0) { echo("<a href='Search Results.php?op=Begin+Search&pg=" . $prevpg ."&property_Type=".urlencode($Property_Type)."&city=".$City."&beds=".$Beds."&baths=".$Baths."&lowest_price=".$Lowest_Price."&highest_price=".$_GET['highest_price']."&sort=".$sort. "'><< Previous page</a> "); } if ($pgnum < $num_pages) { echo("<a href='Search Results.php?op=Begin+Search&pg=" . $nextpg . "&property_Type=".urlencode($Property_Type)."&city=".$City."&beds=".$Beds."&baths=".$Baths."&lowest_price=".$Lowest_Price."&highest_price=".$_GET['highest_price']."&sort=".$sort. "'>Next page >></a> "); } echo " "; echo ($pgnum+1)." out of ".($num_pages+1)." pages"; ?> </div><!--/#rha-mls-pager-container--> <br/> <?php while ($row = mysql_fetch_array($result)){ echo "<div class=\"vcard listing\">"; echo "<div class=\"information\">"; echo "<span class=\"listprice\">\$".number_format($row['LISTPRICE'])."\n </span><br/>"; echo "<p><span>{$row['STREETNUM']},{$row['STREETNAME']} "; echo "<BR/>{$row['CITY']}</span>"; echo "{$row['STATE']}, {$row['ZIPCODE']}</p>"; echo "<p><span>Beds: {$row['BEDS']}, Baths:{$row['BATHSTOTAL']}<br/>Square Feet: ".number_format($row['SQFTTOTAL'])." </span></p>"; echo "</div>"; $photos = $rets->GetObject("Property", "Photo", "{$row['MLSNUM']}", "0", 1); foreach ($photos as $photo) { $listing = $photo['Content-ID']; $number = $photo['Object-ID']; if ($photo['Success'] == true) { echo "<img src='{$photo['Location']}\n' alt=\"\" title=\"\" class=\"listing-photo photo\" width=\"146\" height=\"107\" />"; echo "<a href=\"PropDetails.php?pg=" . $pgnum ."&property_Type=".$Property_Type."&city=".$City."&beds=".$Beds."&baths=".$Baths."&lowest_price=".$Lowest_Price."&highest_price=".$Highest_Price."&MLS=".$row['MLSNUM'].$mainp."#{$photo['Location']}"."\" class=\"button url\">Property Details</a>"; } else { echo "<img src='images/no-image.png' alt=\"\" title=\"\" class=\"listing-photo photo\" width=\"146\" height=\"107\" />"; echo "<a href=\"PropDetails.php?MLS={$row['MLSNUM']}\" class=\"button url\">See Property Details</a>"; } } echo "</div>"; } ?> </div> </div> </div> </div> </div> </div> </body> </head> </html> Any ideas guys???
-
Hi, I have a search form that I built and I'm currently having difficulty sorting search results. I have set up a form with get method for displaying results and another form in a search results page with post method for sorting. I can do sorting on the first page but when I click on next page, it does not display second page... I kinda have an idea of how I might be able to solve this issue but I can't seem to find a comprehensive solution... Please have a look at my search form at below link... Please help me, this is urgent. Thank you very much! http://test1.favstay.com/Search Form.php <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="en" lang="en" dir="ltr"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>JR Molina</title> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <meta name="robots" content="index,follow" /> <link rel="shortcut icon" href="/favicon.ico" type="image/x-icon" /> <link rel="apple-touch-icon" href="/apple-touch-icon.png"/> <link charset="utf-8" type="text/css" rel="stylesheet" media="all" href="results.css" /> <?php if (is_numeric($_GET['pg'])) { $pgnum = ((int) $_GET['pg']); } else { $pgnum = 0; $errorMessage = "Invalid value for page number"; } $startnum = 1; $nextpg = ($pgnum + 1); $prevpg = ($pgnum-1); if ($pgnum >= 0) { $offset = ($pgnum * 20); } else { $offset = 0; } $limit = 20; ?> <?php $Property_Type=$_GET['property_type']; $City=$_GET['city']; $Beds=$_GET['beds']; $Baths=$_GET['baths']; $Lowest_Price=$_GET['lowest_price']; $Highest_Price=$_GET['highest_price']; $MLS=$_GET['mls_number']; $AddressA=$_GET['address']; $Zip_codeA=$_GET['zip_code']; $SubdivisionA=$_GET['subdivision']; $GarageA=$_GET['garage']; $StoriesA=$_GET['stories']; $FireplacesA=$_GET['fireplaces']; $Yard_sizeA=$_GET['yard_size']; $CitiesA=$_GET['cities']; $AreasA=$_GET['areas']; $CountiesA=$_GET['counties']; $School_districtA=$_GET['school_district']; $School_elementaryA=$_GET['school_type[elementary]']; $School_middleA=$_GET['school_type[middle]']; $School_highA=$_GET['school_type[high]']; $School_nameA=$_GET['school_name']; $Guest_quartersA=$_GET['property[guest-quarters]']; $High_riseA=$_GET['property[high-rise-condominium]']; $Loft_A=$_GET['property[loft]']; $BalconyA=$_GET['property[balcony]']; $LibraryA=$_GET['property[library-or-study]']; $MediaA=$_GET['property[media-room]']; $Gated_communityA=$_GET['location[gated-community]']; $Golf_course_communityA=$_GET['location[golf-course-community]']; $Golf_course_lotA=$_GET['location[golf-course-lot]']; $Lake_front_lotA=$_GET['location[lake-front-lot]']; $Lake_viewA=$_GET['location[lake-view]']; $Handicap_amenitiesA=$_GET['location[handicap-ammenities]']; $Zero_lot_lineA=$_GET['location[zero-lot-line]']; $Horses_allowedA=$_GET['location[horses-allowed]']; $Fence_woodA=$_GET['fence[wood]']; $Fence_ironA=$_GET['fence[iron]']; $Fence_brickA=$_GET['fence[brick]']; $Fence_rockA=$_GET['fence[rock]']; $Fence_automatic_gateA=$_GET['fence[automatic-gate]']; $Fence_noneA=$_GET['fence[none]']; $Special_requirementsA=$_GET['special_requirements']; $Properties_with_photosA=$_GET['properties_with[photos]']; $Single_detachedA=$_GET['property_types[single-detached]']; $MultiA=$_GET['property_types[multi]']; $TownhomeA=$_GET['property_types[townhome]']; $CondoA=$_GET['property_types[condo]']; $Half_duplexA=$_GET['property_types[half-duplex]']; $LeaseA=$_GET['property_types[lease]']; $Joseph_molinaA=$_GET['only[joseph-molina-properties]']; $Open_housesA=$_GET['only[open-houses]']; include("db.php"); $rets_login_url = "http://ntreisrets.mls.ntreis.net:80/rets/login"; $rets_username = "j_Kp$06$"; $rets_password = "$33D@E862"; $rets_agent = "JKP"; require_once('phRets.php'); $rets = new phRets; $connect = $rets->Connect($rets_login_url, $rets_username, $rets_password); if (!$connect) { print_r($rets->Error()); } ?> <body id="jrmolina-listing-detail" class="our-listings our-listings-landing" text> <div id="page"> <div id="content-container"> <div id="content" class="clearfix"> <div id="container"> <form action="<?php 'Search Results.php?op=Begin+Search&pg=' . 0 . '&property_Type='.urlencode($Property_Type).'&city='.$City.'&beds='.$Beds.'&baths='.$Baths.'&lowest_price='.$Lowest_Price.'&highest_price='.$Highest_Price; ?>" method="post" id="rha-mls-our-listings-sort"> <div id="rha-mls-our-listings-sort-container"> <label for="edit-sort-by" id="for-edit-sort-by"><span>Sort By</span> <select name="sort_by" class="form-select" id="edit-sort-by" ><option value="none">Choose one</option><option value="price-high-to-low">Price Highest to Lowest</option><option value="price-low-to-high">Price Lowest to Highest</option><option value="sqft-high-to-low">Size Largest to Smallest</option><option value="sqft-low-to-high">Size Smallest to Largest</option><option value="street-name-alpha">Street Name</option><option value="listing-date-desc">Listing Date Newest to Oldest</option><option value="zip-code-numeric">Zip Code</option></select></label> <input type="submit" name="op" id="edit-submit" value="Go" class="form-submit" /> <input type="submit" name="op" id="edit-reset" value="Reset" class="form-submit" /> </div><!--/#rha-mls-our-listings-sort-container--> </form><!--/#rha-mls-our-listings-sort--> <div id="listings-container"> <div id="listings"> <?php switch($_POST['sort_by']){ case "none": $sort = "MODIFIED ASC"; break; case "price-high-to-low": $sort = "LISTPRICE DESC"; break; case "price-low-to-high": $sort = "LISTPRICE ASC"; break; } if($Highest_Price==0 || $Highest_Price=="no_limit"){ if($Highest_Price=="no_limit"){$Highest_Price="5000000";} $Any= ">="; } else { $Any= "<="; } if($City==0){ $Any_City= ">"; } else { $Any_City= "LIKE"; } if($MLS==NULL){ $Any_MLS= "IS NOT"; } else { $Any_MLS= "="; } $result = mysql_query("SELECT * FROM residential WHERE PROPSUBTYPEDISPLAY LIKE '%{$Property_Type}%' AND AREA {$Any_City} {$City} AND BEDS >={$Beds} AND BATHSTOTAL >={$Baths} AND LISTPRICE >={$Lowest_Price} AND LISTPRICE {$Any} {$Highest_Price} ORDER BY {$sort} LIMIT {$offset}, {$limit}") or die(mysql_error()); ?> <div id="rha-mls-pager-container"> <?php mysql_query("SELECT SQL_CALC_FOUND_ROWS * FROM residential WHERE PROPSUBTYPEDISPLAY LIKE '%{$Property_Type}%' AND AREA {$Any_City} {$City} AND BEDS >={$Beds} AND BATHSTOTAL >={$Baths} AND LISTPRICE >={$Lowest_Price} AND LISTPRICE {$Any} {$Highest_Price} ORDER BY {$sort} LIMIT {$offset}, {$limit}"); $total_rows = mysql_query("SELECT FOUND_ROWS()"); $total_found = mysql_fetch_array($total_rows); $pages_count= $total_found[0] / $limit; $num_pages=intval($pages_count); echo $total_found[0]." results found"; echo "<br/>"; if ($pgnum > 0) { echo("<a href='Search Results.php?op=Begin+Search&pg=" . $prevpg ."&property_Type=".urlencode($Property_Type)."&city=".$City."&beds=".$Beds."&baths=".$Baths."&lowest_price=".$Lowest_Price."&highest_price=".$_GET['highest_price']. "'><< Previous page</a> "); } if ($pgnum < $num_pages) { echo("<a href='Search Results.php?op=Begin+Search&pg=" . $nextpg . "&property_Type=".urlencode($Property_Type)."&city=".$City."&beds=".$Beds."&baths=".$Baths."&lowest_price=".$Lowest_Price."&highest_price=".$_GET['highest_price']. "'>Next page >></a> "); } echo " "; echo ($pgnum+1)." out of ".($num_pages+1)." pages"; ?> </div><!--/#rha-mls-pager-container--> <br/> <?php while ($row = mysql_fetch_array($result)){ echo "<div class=\"vcard listing\">"; echo "<div class=\"information\">"; echo "<span class=\"listprice\">\$".number_format($row['LISTPRICE'])."\n </span><br/>"; echo "<p><span>{$row['STREETNUM']},{$row['STREETNAME']} "; echo "<BR/>{$row['CITY']}</span>"; echo "{$row['STATE']}, {$row['ZIPCODE']}</p>"; echo "<p><span>Beds: {$row['BEDS']}, Baths:{$row['BATHSTOTAL']}<br/>Square Feet: ".number_format($row['SQFTTOTAL'])." </span></p>"; echo "</div>"; $photos = $rets->GetObject("Property", "Photo", "{$row['MLSNUM']}", "0", 1); foreach ($photos as $photo) { $listing = $photo['Content-ID']; $number = $photo['Object-ID']; if ($photo['Success'] == true) { echo "<img src='{$photo['Location']}\n' alt=\"\" title=\"\" class=\"listing-photo photo\" width=\"146\" height=\"107\" />"; echo "<a href=\"PropDetails.php?pg=" . $pgnum ."&property_Type=".$Property_Type."&city=".$City."&beds=".$Beds."&baths=".$Baths."&lowest_price=".$Lowest_Price."&highest_price=".$Highest_Price."&MLS=".$row['MLSNUM'].$mainp."#{$photo['Location']}"."\" class=\"button url\">See Property Details</a>"; } else { echo "<img src='images/no-image.png' alt=\"\" title=\"\" class=\"listing-photo photo\" width=\"146\" height=\"107\" />"; echo "<a href=\"PropDetails.php?MLS={$row['MLSNUM']}\" class=\"button url\">See Property Details</a>"; } } echo "</div>"; } ?> </div> </div> </div> </div> </div> </div> </body> </head> </html>
-
Anyone have any ideas??? Please help!!!
-
I have below error when I try to parse a variable via GET request from a php search form to the php result page which is connected to MySQL. You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'LIMIT 0, 20' at line 10 Below is my code...I am getting this error when form name "City is parsed to this php page" funnily enough, when "MLS" is parsed, it works fine and retrieves correct listing... Any ideas?? <?php if (is_numeric($_GET['pg'])) { $pgnum = ((int) $_GET['pg']); } else { $pgnum = 0; $errorMessage = "Invalid value for page number"; } $startnum = 1; $nextpg = ($pgnum + 1); $prevpg = ($pgnum-1); if ($pgnum >= 0) { $offset = ($pgnum * 20); } else { $offset = 0; } $limit = 20; ?> <?php $Property_Type=$_GET['property_type']; $City=$_GET['city']; $Beds=$_GET['beds']; $Baths=$_GET['baths']; $Lowest_Price=$_GET['lowest_price']; $Highest_Price=$_GET['highest_price']; $MLS=$_GET['mls_number']; include("db.php"); $rets_login_url = "http://ntreisrets.mls.ntreis.net:80/rets/login"; $rets_username = "user"; $rets_password = "password"; $rets_agent = "agent"; require_once('phRets.php'); $rets = new phRets; $connect = $rets->Connect($rets_login_url, $rets_username, $rets_password); if (!$connect) { print_r($rets->Error()); } ?> <body id="jrmolina-listing-detail" class="our-listings our-listings-landing" text> <div id="page"> <div id="content-container"> <div id="content" class="clearfix"> <div id="container"> <div id="listings-container"> <div id="listings"> <?php if($Highest_Price==0){ $Any= ">="; } else { $Any= "<="; } if($City==0){ $Any_City= ">"; } else { $Any_City= "LIKE"; } if($MLS==NULL){ $Any_MLS= "IS NOT"; } else { $Any_MLS= "="; } $result = mysql_query("SELECT * FROM residential WHERE PROPSUBTYPEDISPLAY LIKE '%{$Property_Type}%' AND AREA {$Any_City} '%{$City}%' AND BEDS >={$Beds} AND BATHSTOTAL >={$Baths} AND LISTPRICE >={$Lowest_Price} AND LISTPRICE {$Any} {$Highest_Price} AND MLSNUM {$Any_MLS} {$MLS} LIMIT {$offset}, {$limit}") or die(mysql_error()); ?> <div id="rha-mls-pager-container"> <?php mysql_query("SELECT SQL_CALC_FOUND_ROWS * FROM residential WHERE PROPSUBTYPEDISPLAY LIKE '%{$Property_Type}%' AND AREA {$Any_City} '%{$City}%' AND BEDS >={$Beds} AND BATHSTOTAL >={$Baths} AND LISTPRICE >={$Lowest_Price} AND LISTPRICE {$Any} {$Highest_Price} AND MLSNUM {$Any_MLS} {$MLS} LIMIT {$offset}, {$limit}"); $total_rows = mysql_query("SELECT FOUND_ROWS()"); $total_found = mysql_fetch_array($total_rows); $pages_count= $total_found[0] / $limit; $num_pages=round($pages_count,0,PHP_ROUND_HALF_DOWN); echo $total_found[0]." results found"; echo "<br/>"; if ($pgnum > 0) { echo("<a href='Search Results.php?op=Begin+Search&pg=" . $prevpg ."&property_Type=".urlencode($Property_Type)."&city=".$City."&beds=".$Beds."&baths=".$Baths."&lowest_price=".$Lowest_Price."&highest_price=".$Highest_Price. "'><< Previous page</a> "); } if ($pgnum < $num_pages) { echo("<a href='Search Results.php?op=Begin+Search&pg=" . $nextpg . "&property_Type=".urlencode($Property_Type)."&city=".$City."&beds=".$Beds."&baths=".$Baths."&lowest_price=".$Lowest_Price."&highest_price=".$Highest_Price. "'>Next page >></a> "); } echo " "; echo ($pgnum+1)." out of ".($num_pages+1); ?> </div><!--/#rha-mls-pager-container--> <br/> <?php while ($row = mysql_fetch_array($result)){ echo "<div class=\"vcard listing\">"; echo "<div class=\"information\">"; echo "<span class=\"listprice\">\$".number_format($row['LISTPRICE'])."\n </span><br/>"; echo "<p><span>{$row['STREETNUM']},{$row['STREETNAME']} "; echo "<BR/>{$row['CITY']}</span>"; echo "{$row['STATE']}, {$row['ZIPCODE']}</p>"; echo "<p><span>Beds: {$row['BEDS']}, Baths:{$row['BATHSTOTAL']}<br/>Square Feet: ".number_format($row['SQFTTOTAL'])." </span></p>"; echo "</div>"; $photos = $rets->GetObject("Property", "Thumbnail", "{$row['MLSNUM']}", "0", 1); foreach ($photos as $photo) { $listing = $photo['Content-ID']; $number = $photo['Object-ID']; if ($photo['Success'] == true) { echo "<img src='{$photo['Location']}\n' alt=\"\" title=\"\" class=\"listing-photo photo\" width=\"146\" height=\"107\" />"; echo "<a href=\"PropDetails.php?pg=" . $pgnum ."&property_Type=".$Property_Type."&city=".$City."&beds=".$Beds."&baths=".$Baths."&lowest_price=".$Lowest_Price."&highest_price=".$Highest_Price."&MLS=".$row['MLSNUM']."\" class=\"button url\">See Property Details</a>"; } else { echo "<img src='images/no-image.png' alt=\"\" title=\"\" class=\"listing-photo photo\" width=\"146\" height=\"107\" />"; echo "<a href=\"PropDetails.php?MLS={$row['MLSNUM']}\" class=\"button url\">See Property Details</a>"; } } echo "</div>"; } ?>
-
Hi all, I've tried two ways but I cannot get this to work...Basically I'm trying to change the value strings in $row['GARAGEDESC'] to several human readable words... Below string is currently stored in $row['GARAGEDESC'] PARATTACHE,PAROPENER,PARREAR Below is the code... $Garage = $row['GARAGEDESC']; $search = array('[PARSWING]','[PARNONE]','[PARGOLFCAR]','[PARUNCOV]','[PARWKBENCH]','[PARUNASSIG]','[PAROVERSIZ]','[PARREAR]','[PARCOVERED]','[PARPORT-CH]','[PAROTHER]','[PAROPENER]','[PARDETACH]','[PARATTACHE]','[PARSIDE]','[PARCONVERS]','[PARASSIGN]','[PARTANDEM]','[PARSINK]','[PAROUT-ENT]','[PARFRONT]','[PARCIRCLE]'); $replace = array('Swing Drive','None','Golf Cart Garage','Uncovered','Workbench','Unassigned','Oversized','Rear','Covered','Porte-Cochere','Other','Opener','Detached','Attached','Side','Garage Conversion','Assign','Tandem Style','Has Sink in Garage','Outside Entry','Front','Circle Drive'); $msg=str_replace($search,$replace,$Garage); echo $msg; I've also tried this... str_replace("PARSWING","Swing Drive",$Garage); str_replace("PARNONE","None",$Garage); str_replace("PARGOLFCAR","Golf Cart Garage",$Garage); str_replace("PARUNCOV","Uncovered",$Garage); str_replace("PARWKBENCH","Workbench",$Garage); str_replace("PARUNASSIG","Unassigned",$Garage); str_replace("PAROVERSIZ","Oversized",$Garage); str_replace("PARREAR","Rear",$Garage); str_replace("PARCOVERED","Covered",$Garage); str_replace("PARPORT-CH","Porte-Cochere",$Garage); str_replace("PAROTHER","Other",$Garage); str_replace("PAROPENER","Opener",$Garage); str_replace("PARDETACH","Detached",$Garage); str_replace("PARATTACHE","Attached",$Garage); str_replace("PARSIDE","Side",$Garage); str_replace("PARCONVERS","Garage Conversion",$Garage); str_replace("PARASSIGN","Assign",$Garage); str_replace("PARTANDEM","Tandem Style",$Garage); str_replace("PARSINK","Has Sink in Garage",$Garage); str_replace("PAROUT-ENT","Outside Entry",$Garage); str_replace("PARFRONT","Front",$Garage); str_replace("PARCIRCLE","Circle Drive",$Garage); None of them are working... Any ideas????
-
I tried this and it has worked!!! Thanks you very much for all your help!!! mysql_query("SELECT SQL_CALC_FOUND_ROWS * FROM residential WHERE AREA LIKE '{$City}' AND BEDS >={$Beds} AND BATHSTOTAL >={$Baths} AND LISTPRICE >={$Lowest_Price} AND LISTPRICE <={$Highest_Price} LIMIT {$offset}, {$limit}"); $total_rows = mysql_query("SELECT FOUND_ROWS()"); $total_found = mysql_fetch_array($total_rows); echo $total_found[0]." results found";
-
Thank you!!! It's worked!!! You are a legend!!! I have another question... I am trying to get total number of returned results on the page with below query... mysql_query("SELECT SQL_CALC_FOUND_ROWS * FROM residential LIMIT {$limit}"); $total_rows = mysql_query("SELECT FOUND_ROWS()"); $total_found = mysql_fetch_array($total_rows); echo $total_found[0]." results found"; This come out with 2000 results found (which is the total number of rows available in MySQL...) Is there a way we can come up with total number of search returned rows only?
-
I tied that and below error comes up... You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'IS NOT NULL AND 'BATHSTOTAL' >=1 AND 'LISTPRICE' >=1000 ' at line 4
-
Sorry I've actually deleted WHERE 'PROPSUBTYPE' and replaced it with WHERE 'SUBDIVISION' LIKE '{$City}'
-
I seem to be getting this error when I include, WHERE 'PROPSUBTYPE' AND 'SUBDIVISION' LIKE '{$City}' AND 'BEDS' >={$Beds} AND 'BATHSTOTAL' >={$Baths} AND 'LISTPRICE' >={$Lowest_Price} AND 'LiSTPRICE' <={$Highest_Price} inside.... $result = mysql_query("SELECT * FROM residential LIMIT {$offset}, {$limit}");
-
I've tried mysql_error($result); and below error come up. Warning: mysql_error() expects parameter 1 to be resource, boolean given in C:\xampp\htdocs\MLS Search Form\Search Results.php on line 116 Any ideas guys?
-
Sorry the line 116 ($found_results) returns nothing. Below is the code... Any ideas? <?php if (is_numeric($_GET['pg'])) { $pgnum = ((int) $_GET['pg']); } else { $pgnum = 0; $errorMessage = "Invalid value for page number"; } $startnum = 0; $nextpg = ($pgnum + 1); $prevpg = ($pgnum-1); if ($pgnum > 0) { $offset = ($pgnum * 19); } else { $offset = 0; } $limit = 20; ?> <?php $Property_Type=$_GET['property_type']; $City=$_GET['city']; $Beds=$_GET['beds']; $Baths=$_GET['baths']; $Lowest_Price=$_GET['lowest_price']; $Highest_Price=$_GET['highest_price']; echo $Property_Type.", "; echo $City.", "; echo $Beds.", "; echo $Baths.", "; echo $Lowest_Price.", "; echo $Highest_Price.", "; include("db.php"); ?> <body id="www-rogers-healy-com" class="our-listings our-listings-landing" text> <div id="page"> <div id="content-container"> <div id="content" class="clearfix"> <div id="container"> <div id="listings-container"> <div id="listings"> <?php $rets_login_url = "http://ntreisrets.mls.ntreis.net:80/rets/login"; $rets_username = "(Username entered)"; $rets_password = "(Password entered)"; $rets_agent = "(Agent)"; require_once('phRets.php'); $rets = new phRets; $connect = $rets->Connect($rets_login_url, $rets_username, $rets_password); if (!$connect) { print_r($rets->Error()); } $result = mysql_query("SELECT * FROM residential WHERE 'PROPSUBTYPE' AND 'SUBDIVISION' LIKE '{$City}' AND 'BEDS' >={$Beds} AND 'BATHSTOTAL' >={$Baths} AND 'LISTPRICE' >={$Lowest_Price} AND 'LiSTPRICE' <={$Highest_Price} LIMIT {$offset}, {$limit}"); ?> <div id="rha-mls-pager-container"> <?php if ($pgnum > 0) { echo("<a href='Search Results.php?pg=" . $prevpg . "'><< Previous page</a> "); } if ($pgnum > 0) { echo("<a href='Search Results.php?pg=" . $nextpg . "'>Next page >></a> "); } mysql_query("SELECT SQL_CALC_FOUND_ROWS * FROM residential LIMIT {$limit}"); $total_rows = mysql_query("SELECT FOUND_ROWS()"); $total_found = mysql_fetch_array($total_rows); echo $total_found[0]." results found"; ?> </div><!--/#rha-mls-pager-container--> <br/> <?php echo $found_results; while ($row = mysql_fetch_array($result)){ echo "<div class=\"vcard listing\">"; echo "<div class=\"information\">"; echo "<span style=\"size:20px\">\$".number_format($row['LISTPRICE'])."\n </span><br/>"; echo "<p><span>{$row['STREETNUM']},{$row['STREETNAME']} "; echo "<BR/>{$row['CITY']}</span>"; echo "{$row['STATE']}, {$row['ZIPCODE']}</p>"; echo "<p><span>Beds: {$row['BEDS']}, Baths:{$row['BATHSTOTAL']}<br/>Square Feet: ".number_format($row['SQFTTOTAL'])." </span></p>"; echo "</div>"; $photos = $rets->GetObject("Property", "Thumbnail", "{$row['MLSNUM']}", "0", 1); foreach ($photos as $photo) { $listing = $photo['Content-ID']; $number = $photo['Object-ID']; if ($photo['Success'] == true) { echo "<img src='{$photo['Location']}\n' alt=\"\" title=\"\" class=\"listing-photo photo\" width=\"146\" height=\"107\" />"; } else { echo "<img src='images/no-image.png' alt=\"\" title=\"\" class=\"listing-photo photo\" width=\"146\" height=\"107\" />"; } } echo "<a href=\"#\" class=\"button url\">See Property Details</a>"; echo "</div>"; } ?>