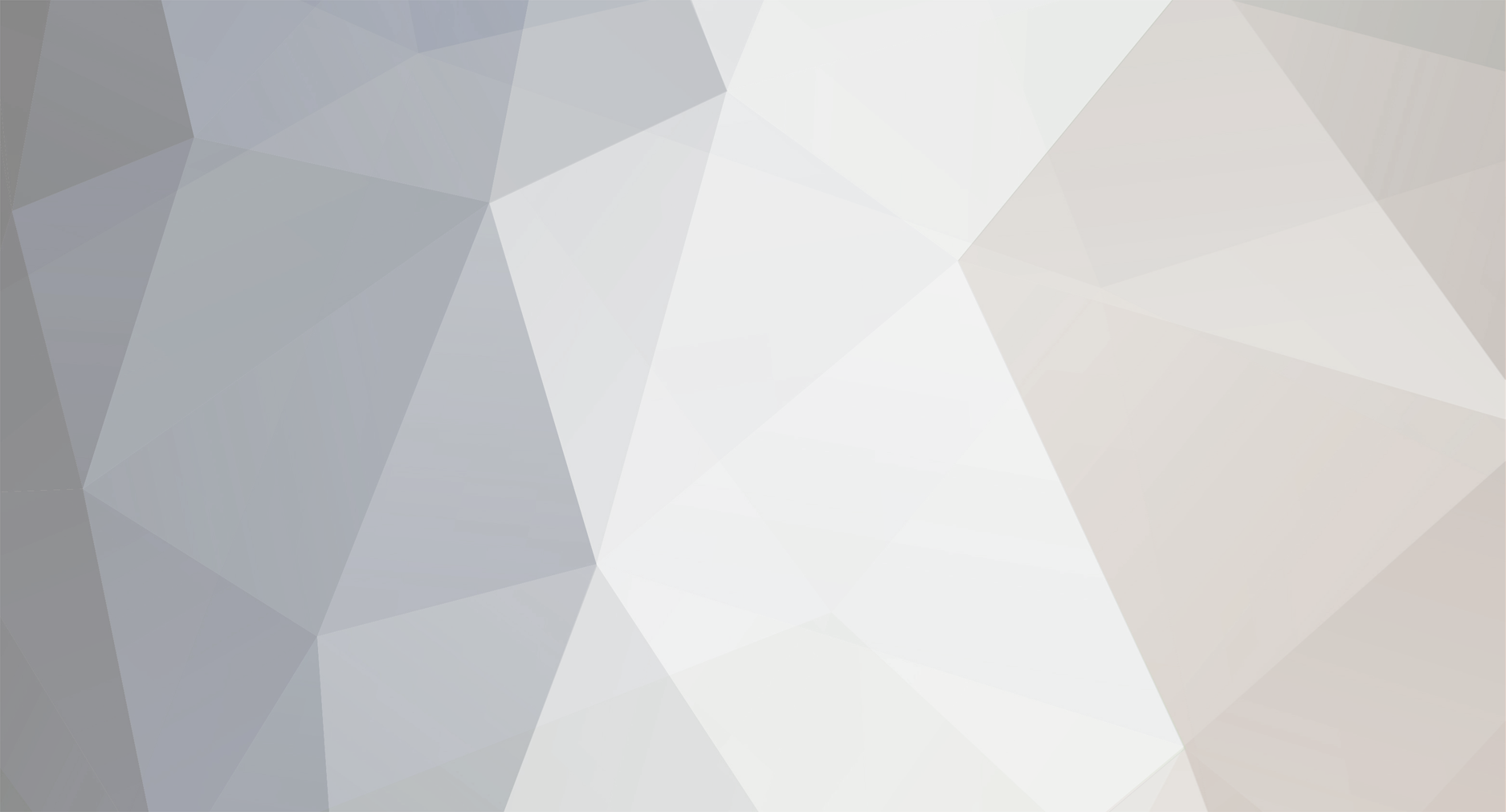
Jragon
Members-
Posts
148 -
Joined
-
Last visited
-
Days Won
1
Everything posted by Jragon
-
Still no errors, I also added some error stuff in user.class.php <?php /** * @author Jragon * @copyright 2011 */ class user { private $username; private $password; private $newPassword; private $salt; private $DBH; public function newUser($user, $pass, $DBH) { //name varibles $this->password = $pass; $this->username = $user; $this->DBH = $DBH; $this->getSalt(); $this->hashPass(); $this->createUser(); } private function getSalt() { //pick random number $a = rand(1, 100); $b = rand(1, 100); $this->salt = rand($a, $b) * 5; } private function hashPass() { //encrypt $this->password with md5 and a salt $plainPass = $this->password . $this->salt; $this->newPassword = md5($plainPass); } private function createUser() { /* //prepare statement $STH = $this->DBH->prepare(" INSERT INTO `users` ( `user` , `pass` , `salt` ) VALUES ( '$this->username', '$this->newPassword', '$this->salt' ); "); //execute statement $STH->execute(); //prepare statement $STH = $this->DBH->prepare(" INSERT INTO `ranks` ( `rank` ) VALUES ( ); "); //execute statement $STH->execute(); */ $STH = $this->DBH->prepare(" INSERT INTO `users` ( `user` , `pass` , `salt` ) VALUES ( '$this->username', '$this->newPassword', '$this->salt' ); "); //execute statement if (!$STH) { print_r($DBH->errorInfo()); die(); } $STH->execute(); //prepare statement $STH = $this->DBH->prepare(" INSERT INTO `ranks` ( `rank` ) VALUES ( 0 ); "); if (!$STH) { print_r($DBH->errorInfo()); die(); } //execute statement $STH->execute(); } } ?>
-
Oops, I forgot to add the includes file... /includes/include.php <?php /** * @author Jragon * @copyright 2011 */ require_once('connect.inc.php'); require_once('connect.php'); require_once('./classes/user.class.php'); ?>
-
Hey guys, I've been doing a little bit of exerementing with PDO's and it seems I can't get it to update the database... I don't know why... Anyway here is the code: index.php <?php /** * @author Jragon * @copyright 2011 */ require_once('includes/include.php'); $user = new user(); $user->newUser('Jragon', 'blenders', $DBH); echo '=D'; ?> /includes/connect.inc.php <?php /** * @author Jragon * @copyright 2011 */ //Define connection details //host define('HOST', 'localhost'); //DataBase define('DB', 'rankometer'); //Username define('USER', 'root'); //Password define('PASS', ''); ?> /includes/connect.php <?php /** * @author Jragon * @copyright 2011 */ //Include connection crap require_once('connect.inc.php'); //connect try { //new PDO $DBH = new PDO("mysql:host=" . HOST . ";dbname=" . DB, USER, PASS); //check for errors } catch(PDOException $e) { echo $e->getMessage(); } ?> /classes/user.class.php <?php /** * @author Jragon * @copyright 2011 */ class user{ private $username; private $password; private $newPassword; private $salt; private $DBH; public function newUser($user, $pass, $DBH){ //name varibles $this->password = $pass; $this->username = $user; $this->DBH = $DBH; $this->getSalt(); $this->hashPass(); $this->createUser(); } private function getSalt(){ //pick random number $a = rand(1, 100); $b = rand(1, 100); $this->salt = rand($a, $b) * 5; } private function hashPass(){ //encrypt $this->password with md5 and a salt $plainPass = $this->password . $this->salt; $this->newPassword = md5($plainPass); } private function createUser(){ //prepare statement $STH = $this->DBH->prepare(" INSERT INTO `users` ( `user` , `pass` , `salt` ) VALUES ( '$this->username', '$this->newPassword', '$this->salt' ); "); //execute statement $STH->execute(); //prepare statement $STH = $this->DBH->prepare(" INSERT INTO `ranks` ( `rank` ) VALUES ( ); "); //execute statement $STH->execute(); } } ?> SQL dump -- phpMyAdmin SQL Dump -- version 3.3.9 -- http://www.phpmyadmin.net -- -- Host: localhost -- Generation Time: Jun 02, 2011 at 06:05 PM -- Server version: 5.5.8 -- PHP Version: 5.3.5 SET SQL_MODE="NO_AUTO_VALUE_ON_ZERO"; -- -- Database: `cakes` -- -- -------------------------------------------------------- -- -- Table structure for table `cakes` -- CREATE TABLE IF NOT EXISTS `cakes` ( `UID` int(11) NOT NULL AUTO_INCREMENT, `cake` int(11) NOT NULL, PRIMARY KEY (`UID`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=1 ; -- -- Dumping data for table `cakes` -- -- -------------------------------------------------------- -- -- Table structure for table `users` -- CREATE TABLE IF NOT EXISTS `users` ( `UID` int(11) NOT NULL AUTO_INCREMENT, `userName` varchar(11) NOT NULL, `passWord` varchar(32) NOT NULL, `salt` int(11) NOT NULL, PRIMARY KEY (`UID`) ) ENGINE=InnoDB DEFAULT CHARSET=latin1 AUTO_INCREMENT=2 ; -- -- Dumping data for table `users` -- I have no idea what is going wrong... I don't get an error. All the output I get is '=D'. Thanks -Jragon
-
<?php //Opens the socket $socket = fsockopen("irc.freenode.com",6667); //Sends Host name fputs($socket, "USER Jragon_Bot Jragon.co.uk PHP :Jragon_Bot\n"); //Send the nickname fputs($socket, "NICK Jragon_Bot\n"); //Joins ##thenewboston fputs($socket, "JOIN ##thenewboston\n"); //Set commands $commands = array( "!version", "!say", "!exit", "Hello" ); while(1){ //Gets data while($data = fgets($socket, 128)){ //Puts data into an array. $get = explode(' ', $data); //Server pinged, time to reply if($get[0] == "PING"){ fputs($socket, "PONG " . $get[1] . "\n"); } if(substr_count($get[2],"#")) { $nick = explode(':',$get[0]); $nick = explode('!',$nick[1]); $nick = $nick[0]; $chan = $get[2]; $num = 3; if($num == 3){ $split = explode(':',$get[3],2); $text = $split[1]; //Processing of commands //This is where we start processing the commands we entered in earlier if(in_array(rtrim($text),$commands)) { switch(rtrim($text)) { case "!version": fputs($socket,"PRIVMSG $chan : Jragon bot, version 0.01 Alpha\n Developed by Jragon(Rory Anderson)\n"); break; case "!say": $arraysize = sizeof($get); //1,2,3 are just nick and chan, 4 is where text starts $count = 4; while($count <= $arraysize) { $saytext = $saytext." ".$get[$count]; $count++; } fputs($socket,"PRIVMSG ".$get[2]." :".$saytext."\n"); unset($saytext); break; case "!exit": fputs($socket,"PRIVMSG $chan :Shutting Down Jragon's very awesome bot!\n"); fputs($socket,"QUIT Client Disconnected!\n"); //IMPORANT TO HAVE THE DIE(), this throws the script out of the infinite while loop! die('Client Disconnected!'); break; case "Hello": fputs($socket,"PRIVMSG $chan :Hi!\n"); break; } } } } } } //Shows the text in the browser as Time - Text echo nl2br(date('G:i:s')."-".$data); //Flush it out to the browser flush(); ?> That is my first bot that worked...
-
Hey guys, I've been working on a IRC bot, but there is something wrong with it, it is ment to be an endless loop, but it runs out of memory before it joins D= Error: Fatal error: Allowed memory size of 134217728 bytes exhausted (tried to allocate 261900 bytes) in /var/www/random/bot2.php on line 90 Code: <?php set_time_limit(0); ini_set('desplay_errors', 'on'); $configure = array( 'server' => 'irc.freenode.net', 'port' => 6667, 'nick' => 'TNB_BOT', 'name' => 'JBot', 'channel' => '##thenewboston', 'pass' => '***' ); class JBot{ //TCP connection holder. public $socket; //Message holder. public $msg = array(); /* * Constucter. * Opens the server connection, and logs in the bot. * * @param array. */ function __construct($configure){ $this->socket = fsockopen($configure['server'], $configure['port']); $this->login($configure); $this->main(); $this->send_data('JOIN', $this->configure['channel']); } /* * Logs bot in to server * * @param array. */ function login($configure){ $this->send_data('USER', $configure['nick'] . ' Jragon.co.uk ' . $configure['nick'] . ' :' . $configure['name']); $this->send_data('NICK', $configure['nick']); } /* * Main function, used to grab all data. * */ function main(){ $data = fgets($this->socket, 128); flush(); $this->ex = explode(' ', $data); if($this->ex[0] == 'PING'){ //Plays ping-pong with the server.. $this->send_data('PONG', $this->ex[1]); } $command = str_replace(array(chr(10), chr(13)), '', $this->ex[3]); //List of commands the bot responds to. switch($command){ case ':!say': $this->say(); break; case ':!join': $this->join_channel($this->ex[4]); break; case ':!quit': $this->send_data('QUIT', $this->ex[4]); break; case ':!op': $this->op_user(); break; case ':!deop': $this->op_user('','', false); break; case ':!time': $this->send_data("PRIVMSG", "Time" . date("F j, Y, g:i a" . time())); break; case 'Hello': fputs($socket,"PRIVMSG " . $this->ex[2] . " : Hi!\n"); break; } $this->main(); } function say(){ $arraysize = sizeof($this->ex); //1,2,3 are just nick and chan, 4 is where text starts $count = 4; while($count <= $arraysize) { $text = $text . " " . $this->ex[$count]; $count++; } $this->privmsg($text, $this->ex[2]); unset($text); } function privmsg($message, $to){ fputs($socket,"PRIVMSG " . $to . " :" . $message . "\n"); } /* * Sends data to the server. */ function send_data($cmd, $msg = null){ if($msg == null){ fputs($this->socket, $cmd . "\n"); echo "<b>$cmd</b>"; }else{ fputs($this->socket, $cmd.' '.$msg."\n"); echo "<b>$cmd $msg</b>"; } } /* * Joins a channel. * * @param text */ function join_channel($channel){ $this->send_data('JOIN', $channel); } /* * Give/Take operator status. */ function op_user($channel = '', $user = '', $op = true){ if($channel == '' || $user == ''){ if($channel == ''){ $channel = $this->ex[2]; } if($user == ''){ $user = strstr($this->ex[0], '!', true); }; if($op){ $this->send_data('MODE', $channel . ' +o ' . $user); }else{ $this->send_data('MODE', $channel . ' -o ' . $user); } } } } $bot = new JBot($configure); ?>
-
Hia, I have 3 tables, and I need to do a join on all 3 of them. I have score_game, score_song and score_score. Score game has only a few entries in it, the coloums are game and ID. In score_song it is song game, and ID I need to join those to gether. So in the score_song I need to put game in the game bit, but the game bit is the ID of the games. so if score_song.game was 3 the score_game would put the row with the ID of 3. score_score is the whole thing. It will need to output songs and games joined so they are not integers. dumps -- phpMyAdmin SQL Dump -- version 3.2.4 -- http://www.phpmyadmin.net -- -- Host: localhost -- Generation Time: Jan 03, 2011 at 01:02 PM -- Server version: 5.1.41 -- PHP Version: 5.3.1 SET SQL_MODE="NO_AUTO_VALUE_ON_ZERO"; -- -- Database: `randoms` -- -- -------------------------------------------------------- -- -- Table structure for table `score_games` -- CREATE TABLE IF NOT EXISTS `score_games` ( `Game` varchar(32) NOT NULL, `ID` int(11) NOT NULL AUTO_INCREMENT, PRIMARY KEY (`ID`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=4 ; -- -- Dumping data for table `score_games` -- INSERT INTO `score_games` (`Game`, `ID`) VALUES ('Guitar Hero', 1), ('Frets on Fire', 2), ('You mother.', 3); -- -------------------------------------------------------- -- -- Table structure for table `score_score` -- CREATE TABLE IF NOT EXISTS `score_score` ( `ID` int(11) NOT NULL AUTO_INCREMENT, `user` varchar(32) NOT NULL, `game` int(11) NOT NULL, `score` int(11) NOT NULL, `song` int(11) NOT NULL, `notestreak` int(11) NOT NULL, `notehit` int(11) NOT NULL, PRIMARY KEY (`ID`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=8 ; -- -- Dumping data for table `score_score` -- INSERT INTO `score_score` (`ID`, `user`, `game`, `score`, `song`, `notestreak`, `notehit`) VALUES (1, '$user', 1, 1, 1, 1, 1), (2, '', 2, 0, 0, 22, 0), (3, '', 2, 0, 0, 22, 0), (4, '', 0, 11, 0, 0, 0), (5, '', 0, 0, 0, 22, 0), (6, 'Jargon', 1, 2442, 2, 99, 245), (7, 'splosion man', 3, 0, 4, 0, 42); -- -------------------------------------------------------- -- -- Table structure for table `score_songs` -- CREATE TABLE IF NOT EXISTS `score_songs` ( `song` varchar(255) NOT NULL, `ID` int(11) NOT NULL AUTO_INCREMENT, `game` int(11) NOT NULL, PRIMARY KEY (`ID`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=6 ; -- -- Dumping data for table `score_songs` -- INSERT INTO `score_songs` (`song`, `ID`, `game`) VALUES ('cddf', 1, 2), ('My guitar is a hero', 2, 1), ('My fret is on fire', 3, 2), ('You mother is on fire.', 4, 3), ('You are somewhat agravating.', 5, 3); Then I need it put in to php. Because I have no idea how. Thanks, Jragon.
-
Could you explain how that works plaese.
-
How can i create a download page that allows you to download *.php files on your server. Because if you just click on it it sends you to the page but I just want it to download it. Also i want to be able to do that on the .jpeg files. Thanks Jragon
-
But that would just save it would'nt it? and i need it to desply the image when you do the
-
I have reached the max ammount of images on my forum siggy, and i want it to be one php file but two images.
-
How can i find out the extension of the picture.
Jragon replied to Jragon's topic in PHP Coding Help
So if i did, $pathinfo = pathinfo($_FILE['file']['name']); that would work? -
Hey, I would like to be able to show more than one image that is made by php on the fly. The two codes i want to join: hitcounter <?php /** * @author Jragon * @copyright 2010 */ getHits(); function getHits() { $file = "hits.txt"; $fh = fopen($file, "r"); $hits = @fread($fh, filesize("$file")); fclose($fh); if ($hits <= 0){ $hits =1; } else { ++$hits; } $fh = fopen($file, "w+"); fwrite($fh, "$hits"); fclose($fh); getImage("$hits"); } function getImage($string) { //Make $string to to a string $string = "$string"; //Define fontsize $font = 5; //use imagefontwidth to find out the widht of each charicter, //then times it by how many charicters theere are, //and add some padding. $width = imagefontwidth($font) * strlen($string) + 10; //height of a charicter, and add some padding. $height = imagefontheight($font) + 10; //random color thingy. $r = rand(25, 250); $g = rand(25, 250); $b = rand(25, 250); //create a blank image using the dimsions set above. $image = imagecreate($width, $height); //set the background color $background_color = imagecolorallocate($image, 0, 0, 0); //set the text color using the random integers made above. $text_color = imagecolorallocate($image, $r, $g, $b); //Put the text into the image. imagestring($image, $font, 6, 6, $string, $text_color); //Change the type of webpage so you see the image header("Content-type: image/png"); //put everything together and make the image imagepng($image); //free up some space on the temp drive imagedestroy($image); } ?> and my random quote thingy: <?php /** * @author Jragon * @copyright 2010 */ $textfile = "quotes.txt"; $quotes = array(); if(file_exists($textfile)){ $quotes = explode("\n",file_get_contents($textfile)); srand ((float) microtime() * 10000000); $string = $quotes[array_rand($quotes)]; text_to_image($string, 800); } else { $string = "Sig file non-existant..."; } function text_to_image($text, $image_width) { $r = rand(25, 250); $g = rand(25, 250); $b = rand(25, 250); $font = 5; $line_height = 15; $padding = 5; $text = wordwrap($text, ($image_width/10)); $lines = explode("\n", $text); $image = imagecreate($image_width,((count($lines) * $line_height)) + ($padding * 2)); $background = imagecolorallocate($image, 0, 0, 0); $colour = imagecolorallocate($image, $r, $g, $b); imagefill($image, 0, 0, $background); $i = $padding; foreach($lines as $line){ imagestring($image, $font, $padding, $i, trim($line), $colour); $i += $line_height; } header("Content-type: image/jpeg"); imagejpeg($image); imagedestroy($image); exit; } ?>
-
Is that my code on the website? Thanks=P
-
Hello, I was wondering how you can find out the extension of the image (so .gif, or .jpg). Because i need to make an image uploader thingy, and i want to change the name of the picture to some random string, but keep the extension the same. My friend said i could do it in regex but i have no idea.. Thanks Jragon.
-
What sort of example?
-
Why doesnt it show it on the error thingy, I use ubuntu now, and in XAMPP it showed all the errors. =S Also now it desplays a 0 then a strange symbolything.
-
Hello, My hit counter doesnt work, at all. It is ment to update hits.txt(add 1 to the number in there.), and desplay how many hits it has using gd2. Here is my code: <?php /** * @author Jragon * @copyright 2010 */ getHits() function getHits() { $file = "hits.txt"; $fh = fopen($file, "r"); $hits = fread($fh, filesize("$file")); fclose($fh); ++$hits; $fh = fopen($file, "w+"); fwrite($fh, "$hits"); fclose($fh); getImage("$hits"); } function getImage($string) { //Make $string to to a string $string = "$string"; //Define fontsize $font = 5; //use imagefontwidth to find out the widht of each charicter, //then times it by how many charicters theere are, //and add some padding. $width = imagefontwidth($font) * strlen($string) + 10; //height of a charicter, and add some padding. $height = imagefontheight($font) + 10; //random color thingy. $r = rand(25, 250); $g = rand(25, 250); $b = rand(25, 250); //create a blank image using the dimsions set above. $image = imagecreate($width, $height); //set the background color $background_color = imagecolorallocate($image, 0, 0, 0); //set the text color using the random integers made above. $text_color = imagecolorallocate($image, $r, $g, $b); //Put the text into the image. imagestring($image, $font, 6, 6, $string, $text_color); //Change the type of webpage so you see the image header("Content-type: image/png"); //put everything together and make the image imagepng($image); //free up some space on the temp drive imagedestroy($image); } ?> I have created hits.txt with the value of 0 inside of it. Thanks Jragon
-
Please, i realy need some help
-
checking to see if there is a allready that result in mysql
Jragon replied to Jragon's topic in PHP Coding Help
Anyone got an idea -
checking to see if there is a allready that result in mysql
Jragon replied to Jragon's topic in PHP Coding Help
here is my new code: <?php /** * @author Jragon * @copyright 2010 */ include ("connect.php"); $referer = $_SERVER['HTTP_REFERER']; $user_agent = $_SERVER['HTTP_USER_AGENT']; $ip_adress = $_SERVER['REMOTE_ADDR']; $dup = mysql_query("SELECT `ip_address` FROM `logged_ips` WHERE `ip_address` = '$ip'", $connection) or die(mysql_error()); $count = mysql_num_rows($dup); if ($count == 0) { $string = "INSERT INTO `logged_ips` (`ip_address`, `ip_visits`, `user_agent`, `referer`) VALUES ('$ip_adress', '1', '$user_agent', '$referer')"; mysql_query($string, $connection) or die(mysql_error()); } else { $string2 = "UPDATE `logged_ips` SET `ip_visits` = `ip_visits` + '1' WHERE `ip_address` = '$ip_adress'"; mysql_query($string2, $connection) or die(mysql_error()); } echo $ip_adress ?> dumps: -- phpMyAdmin SQL Dump -- version 3.2.4 -- http://www.phpmyadmin.net -- -- Host: localhost -- Generation Time: Nov 15, 2010 at 05:15 PM -- Server version: 5.1.41 -- PHP Version: 5.3.1 SET SQL_MODE="NO_AUTO_VALUE_ON_ZERO"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8 */; -- -- Database: `iplog` -- -- -------------------------------------------------------- -- -- Table structure for table `logged_ips` -- CREATE TABLE IF NOT EXISTS `logged_ips` ( `aid` int(11) NOT NULL AUTO_INCREMENT, `ip_address` int(11) NOT NULL, `user_agent` varchar(244) NOT NULL, `referer` varchar(244) NOT NULL, `ip_visits` int(11) NOT NULL, PRIMARY KEY (`aid`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=54 ; -- -- Dumping data for table `logged_ips` -- INSERT INTO `logged_ips` (`aid`, `ip_address`, `user_agent`, `referer`, `ip_visits`) VALUES (53, 1270, 'Mozilla/5.0 (Windows; U; Windows NT 5.1; en-GB; rv:1.9.2.12) Gecko/20101026 Firefox/3.6.12 ( .NET CLR 3.5.30729)', '', 1), (52, 1270, 'Mozilla/5.0 (Windows; U; Windows NT 5.1; en-GB; rv:1.9.2.12) Gecko/20101026 Firefox/3.6.12 ( .NET CLR 3.5.30729)', '', 1), (51, 1270, 'Mozilla/5.0 (Windows; U; Windows NT 5.1; en-GB; rv:1.9.2.12) Gecko/20101026 Firefox/3.6.12 ( .NET CLR 3.5.30729)', '', 1); /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */; It doesnt compleatly work, it outputs the ip fine, but doesnt puts something strange in the ip box, and it doesnt only put the ip up once =S Pelase help Jragon -
checking to see if there is a allready that result in mysql
Jragon replied to Jragon's topic in PHP Coding Help
It does not put anything in to the database, but outputs the ip adress -
checking to see if there is a allready that result in mysql
Jragon replied to Jragon's topic in PHP Coding Help
It still wont work, all it does is out put the ip. -
checking to see if there is a allready that result in mysql
Jragon replied to Jragon's topic in PHP Coding Help
<?php //finds out ip $ip = $_SERVER['REMOTE_ADDR']; //conects to the mysql server $connection = mysql_connect('localhost', 'root', ''); //sellects the database mysql_select_db('iplog', $connection); //looks for duplacute ips $query = "INSERT INTO logged_ips (ip_address, ip_visits) VALUES ('$ip',0) ON DUPLICATE KEY UPDATE SET ip_visits = ip_visits + 1"; mysql_query($query, $connection); echo $ip; ?> doesnt work D= -
checking to see if there is a allready that result in mysql
Jragon replied to Jragon's topic in PHP Coding Help
Smae error with updated code. -
This is my new code: <?php /** * @author Jragon * @copyright 2010 */ $textfile = "quotes.txt"; $quotes = array(); if (file_exists($textfile)) { $quotes = explode("\n", file_get_contents($textfile)); srand((float)microtime() * 10000000); $string = $quotes[array_rand($quotes)]; $string = wordwrap($string, 100, "\n", true); } else { $string = "Sig file non-existant..."; } function text_to_image($text){ //test $width = imagefontwidth($font_size) * strlen($string); $height = imagefontheight($font_size); echo $width . "<br />" . $hight; //end test $colour = array(0, 244, 34); $background = array(0, 0, 0); $font_size = 5; $line_height = 15; $padding = 2; $text = wordwrap($text, ($image_width/10)); $lines = explode("\n", $text); $image = imagecreate($width, $height); $background = imagecolorallocate($image, $background[48], $background[44], $background[44]); $colour = imagecolorallocate($image, $colour[0], $colour[1], $colour[2]); imagefill($image, 0, 0, $background); $i = $padding; foreach ($lines as $line) { imagestring($image, $font, $padding, $i, trim($line), $colour); $i += $line_height; } header("Content-type: image/jpeg"); imagejpeg($image); imagedestroy($image); exit; } ?> It now outputs just the width and doesnt make a picture