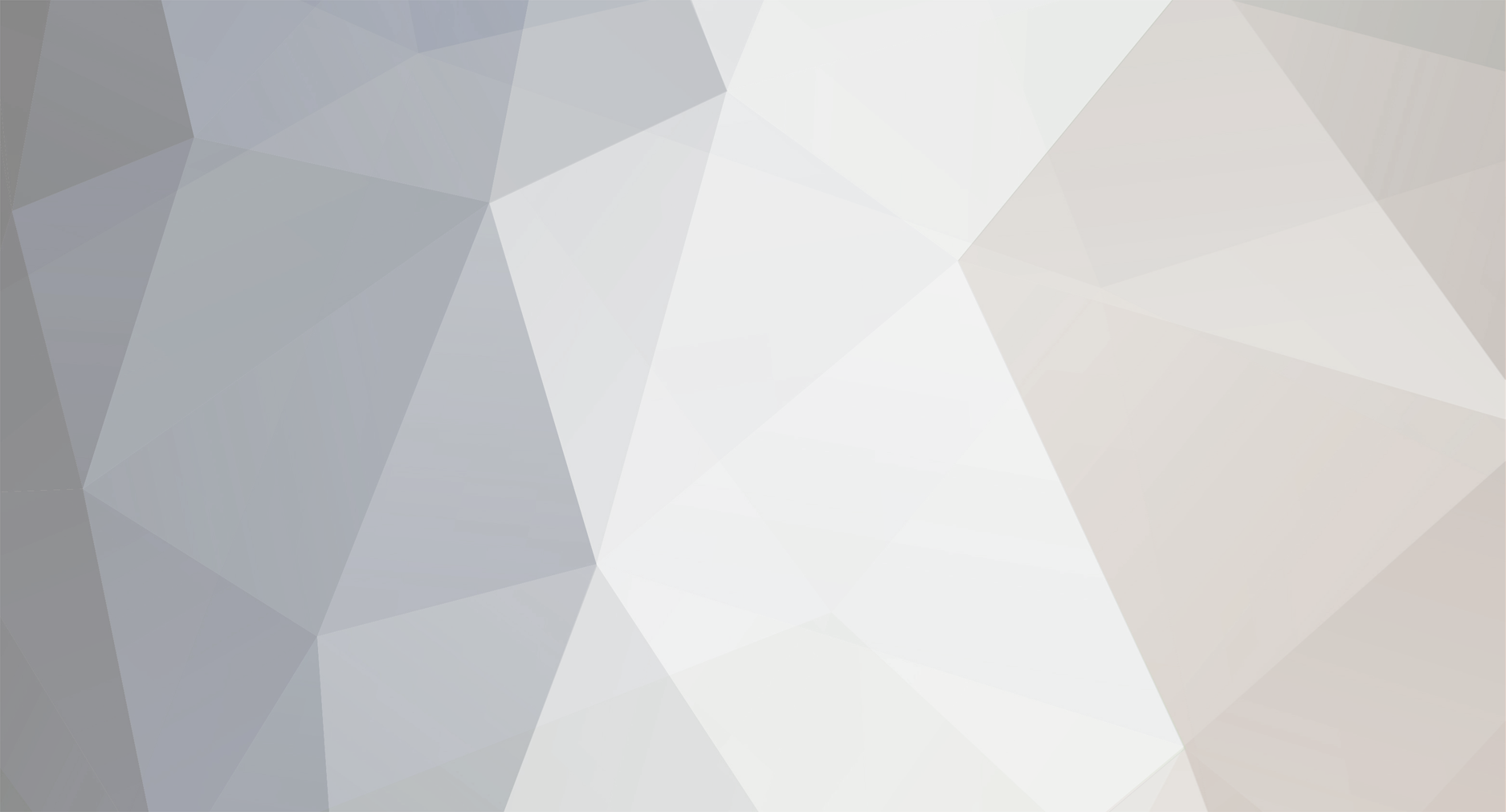
TheEddy
-
Posts
32 -
Joined
-
Last visited
Never
Posts posted by TheEddy
-
-
Recaptcha has been cracked and bots can now get past it.
-
I am leaning towards Zend but is it worth my time? Don't I also have to pay yearly to be able to use their framework on websites?
-
I am a noob, what exactly are frameworks?
-
It was better for me to use this:
function curPageURL() { $pageURL = 'http'; if ($_SERVER["HTTPS"] == "on") {$pageURL .= "s";} $pageURL .= "://"; if ($_SERVER["SERVER_PORT"] != "80") { $pageURL .= $_SERVER["SERVER_NAME"].":".$_SERVER["SERVER_PORT"].$_SERVER["REQUEST_URI"]; } else { $pageURL .= $_SERVER["SERVER_NAME"].$_SERVER["REQUEST_URI"]; } return $pageURL; } $r=curPageURL(); $_SESSION['REFERER']=$r;
Because I also needed to record the direct input from the browser. Thanks for your help though!
-
How does one use the HTTP_REFERER method?You could use $_SERVER['HTTP_REFERER'], or use sessions to store the previous page URI, then re-direct to that after login.
-
I want people to login and then be redirected back to the page from where they came from. I was thinking that maybe I would have to use $_GET for 3 pages but I don't know if there is any easier way.
-
What are you getting for your output?
Nothing will appear unless I search for email and only one result when there should be two. If I search for email, I don't get the message that there is nothing there but no results are shown.
-
That doesn't work btw.mysql_fetch_assoc stores the values in an array. So you could either use the list function like:
while(list($uid,$userName) = mysql_fetch_assoc($result)){
Or when you print the HTML you would do something like
echo "<li><a href=\"membersprofiles.php?uid=".$row['uid']."\" title=\"".$row['userName']."\">".$row['userName']."</a></li>\n";
-
What does "\n" do?
-
if (mysql_num_rows($result) != 0) { print (" <ul> "); while ($row = mysql_fetch_assoc($result)){ print (" <li><a href=\"memberprofiles.php?uid=$uid\" title\"$userName\">$userName</a></li> "); exit; } print (" </ul> "); } else { print (" There were no results based on your criteria. "); }
I don't know how I made that mistake where I had that equal to 1. But I don't know how to loop the results
-
That looks like it would work as written. Have you echoed the query string to make sure it contains the values it should contain?
This is what I have for results:
$sql = "SELECT * FROM `users` WHERE `$searchField` LIKE '%$search%'"; $result = mysql_query($sql) or die(mysql_error()); $row = mysql_fetch_array($result); $userName = $row['userName']; $uid = $row['userID']; if (mysql_num_rows($result) == 1) { print (" <ul> "); while ($row = mysql_num_rows($result)){ print (" <li><a href=\"memberprofiles.php?uid=$uid\" title\"$userName\">$userName</a></li> "); exit; } print (" </ul> "); } else { print (" There were no results based on your criteria. "); }
-
Post an example of the search term you used and the data in the database that you feel it should have returned.
$searchField would have been "emailAddress" and $search should have been "webmaster" and should have pulled:
webmaster@test.com and webmaster@testing.com
-
$sql = "SELECT * FROM `users` WHERE `$searchField` like '%$search%'";
I still have to put in the EXACT value, it can't be similar.
-
yeah that should work..
It didn't work lol.
-
I want to submit a form using ajax so that the page doesn't have to reload. How do I do this?
-
ThanksUse brackets, like
Where ('emailadres' = '"blabla"' OR 'username' = '"blabla'") AND 'password' = '"blabla"';
-
$sql = "SELECT * FROM `users` WHERE `emailAddress` = '".mysql_real_escape_string($_POST['email'])."' AND `password` = '".mysql_real_escape_string(md5($_POST['password']))."'";
I currently have it accepting just the email address but I want it to be the email address OR the username. How do I do this?
-
<?php $pageTitle='Edit Profile'; require_once ("header.php"); ?>
How can I pass a variable like that through header.php? I know it doesn't work the way I have it.
What doesn't work? If you echo $pageTitle in header.php it will work, unless header.php has already been included/required.
I am an idiot, I see the problem now haha.
I had:
<title>$pageTitle</title>
in header.php and it displayed $pageTitle as the title.
-
<script type="text/javascript"> var $J = jQuery.noConflict(); jQuery(document).ready(function($){ $J("#featured > ul").tabs({fx:{opacity: "toggle"}}).tabs("rotate", 5000, true); }); // Use Prototype with $(...), etc. $('div').hide(); </script>
Like that?
-
<?php $pageTitle='Edit Profile'; require_once ("header.php"); ?>
How can I pass a variable like that through header.php? I know it doesn't work the way I have it.
-
Including a file over HTTP won't get you the source code, only the output (which in your case is nothing). Try this:
include("cookie.php");
would it work if he had
<?php include ("www.website.com/cookie.php"); ?>
???
-
you need to use an update query instead of insert.
<?php require_once ("dbconn.php"); ?> <?php $userID = $_SESSION['userID']; $insert_query = 'UPDATE users SET aim="' . $_POST['aim'] . '", msn="' . $_POST['msn'] . '", yim="' . $_POST['yim'] . '", psnID="' . $_POST['psnID'] . '", xblGamertag="' . $_POST['xblGamertag'] . '", otherContact="' . $_POST['otherContact'] . '", WHERE userID = $userID;' mysql_query($insert_query); ?>
It says I did something wrong but I can't find it
-
<?php if(!isset($_SESSION)) { session_start(); } // UNCOMMENT NEXT LINE TO PRINT THE $_SESSION ARRAY TO THE SCREEN . . . // echo '<pre>'; print_r($_SESSION); echo '</PRE>'; if(empty($_SESSION['userID']) || $_SESSION['authorized'] != true ) { header("Location: login.php"); exit; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html lang="EN" dir="ltr" xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="content-type" content="text/xml; charset=utf-8" /> <meta http-equiv="refresh" content="5;url=editprofile.php"> <title>Saving Profile</title> </head> <body> <?php require_once ("dbconn.php"); ?> <?php $userID = $_SESSION['userID']; $insert_query = 'insert into users WHERE userID='$userID'( aim, msn, yim, psnID, xblGamertag, otherContact ) values ( "' . $_POST['aim'] . '", "' . $_POST['msn'] . '", "' . $_POST['yim'] . '", "' . $_POST['psnID'] . '", "' . $_POST['xblGamertag'] . '", "' . $_POST['otherContact'] . '" )'; mysql_query($insert_query); ?> Your profile has been saved! You will now be redirected from where you came from. <br /><a href="editprofile.php" title="Click here if you don't want to wait">Click here if you don't want to wait.</a> </body> </html>
It creates a new record but I want it to update an existing one. It's an editprofile script.
-
You posted some more while I was writing, I guess. In the editprofile.php script, the session_start() needs to be before any attempt to use a $_SESSION var. You should also check specifically for the $_SESSION array element you need to be set. This is untested, but it should work as written. Let me know if it doesn't . . .
<?php session_start(); // UNCOMMENT NEXT LINE TO PRINT THE $_SESSION ARRAY TO THE SCREEN . . . // echo '<pre>'; print_r($_SESSION); echo '</PRE>'; if(empty($_SESSION['userID']) || $_SESSION['authorized'] != true ) { header("Location: login.php"); exit; } else { ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-strict.dtd"> <html lang="EN" dir="ltr" xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="content-type" content="text/xml; charset=utf-8" /> <title>Edit Profile</title> <style type = "text/css"> table { margin: 0 auto; </style> </head> <body> <?php require_once ("dbconn.php"); $result = mysql_query("SELECT * FROM `users` WHERE `userID` = '{$_SESSION['userID']}' LIMIT 1") or die(mysql_error()); $row = mysql_fetch_array($result); $userName = $_SESSION['userID']; print ("Testing. Your ID is $userID<br /><a href=\"http://localhost/mgnml/logout.php\">Log Out</a></body></html>"); } ?>
Had to also make some changes to the other code. Now it works. Thanks for the help!
Is it worth my time to learn a framework?
in Frameworks
Posted
And how does one exactly use a framework? If I am going to make an application for others to use as well, is it a good idea to develop it in a framework?