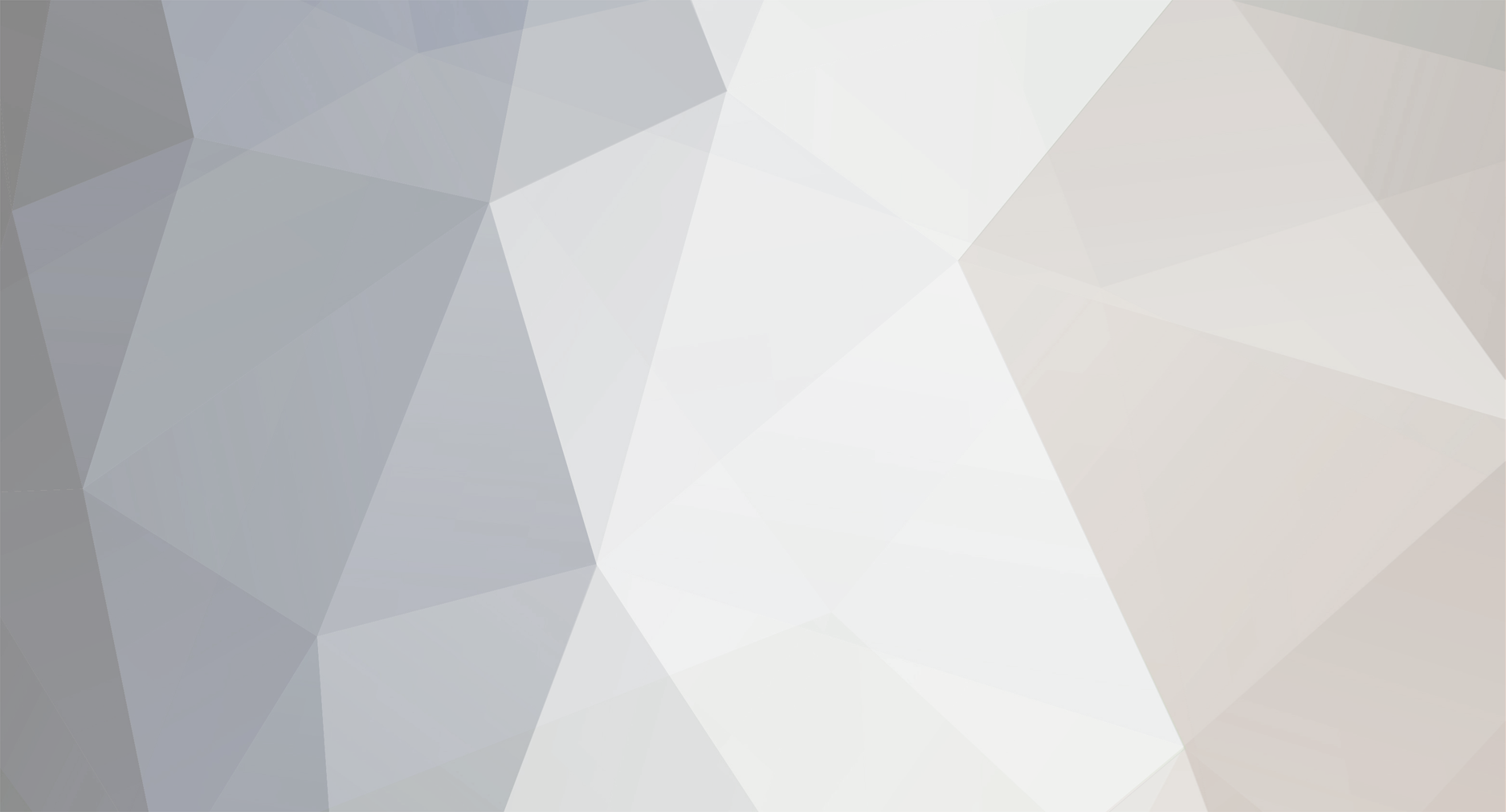
jjfletch
Members-
Posts
7 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
jjfletch's Achievements

Newbie (1/5)
0
Reputation
-
Trying to sort an array by zip, then by distance, and then filter out any duplicates. Right now, my code just yields: Warning: usort() [function.usort]: Invalid comparison function I'm not sure, but I suspect that since this whole thing is in a class, I can't call it the way I'm currently doing? Anyway, I've got an array that looks like this: Array ( [0] => Array ( [zip] => '01505' [distance] => '7.8405015332347' [tpid] => 32112 ) [1] => Array ( [zip] => '01505' [distance] => '6.50172229325415' [tpid] => 32112 ) [2] => Array ( [zip] => '01505' [distance] => '4.50967688350022' [tpid] => 43444 ) [3] => Array ( [zip] => '01564' [distance] => '6.91151548994925' [tpid] => 32112 ) [4] => Array ( [zip] => '02021' [distance] => '6.52192787895371' [tpid] => 43444 ) [5] => Array ( [zip] => '02021' [distance] => '6.91070971397532' [tpid] => 32112 ) ) First, I'm trying to sort them by zip and second, I'm trying to delete the array element if it's a duplicate and whose distance value is higher than the other one. So, based on the example above, I'm trying to create: Array ( [0] => Array ( [zip] => '01505' [distance] => '4.50967688350022' [tpid] => 43444 ) [1] => Array ( [zip] => '01564' [distance] => '6.91151548994925' [tpid] => 32112 ) [2] => Array ( [zip] => '02021' [distance] => '6.52192787895371' [tpid] => 43444 ) ) This is all within a class. So, I have this: function cmp($a, $b) { if ($a['zip'] == $b['zip']) { if ($a['distance'] == $b['distance']) { return 0; } return ($a['distance'] < $b['distance']) ? -1 : 1; } return ($a['zip'] < $b['zip']) ? -1 : 1; } function processRes($post) { $r2=0; for($i=0; $i < count($post); $i++) { $fetch = $this->zipProx($post[$i]['zipcode'], $post[$i]['radius']); for($f = 0; $f < count($fetch); $f++) { $zips[$r2]['zip'] = "'".$fetch[$f]['zip']."'"; $zips[$r2]['distance'] = "'".$fetch[$f]['distance']."'"; $zips[$r2]['tpid'] = $post[$i]['tpid']; $r2++; } } usort($zips, 'cmp'); for ($z=1, $j=0, $n=count($zips); $z<$n; ++$z) { if ($zips[$z]['zip'] == $zips[$j]['zip']) { unset($zips[$z]); } else { $j = $z; } } }
-
Yes, it actually is... That was just me trying to see if that would work... But, it didn't...
-
The code below works insomuch that I can successfully download the directory recursively. But, I want to download the directories within this directory. So, when it connects it's in . Within the . directory is a subdirectory "IN". I want to recursively retrieve the contents within the "IN" directory. The directory names themselves will change, so I can't specify what that's going to be in the script itself... Anyone know how to do this? ftp_sync ("./In/"); ftp_close($conn_id); function ftp_sync ($dir) { global $conn_id; if ($dir != ".") { if (ftp_chdir($conn_id, $dir) == false) { echo ("Change Dir Failed: $dir<BR>\r\n"); return; } if (!(is_dir($dir))) mkdir($dir); chdir ($dir); } $contents = ftp_nlist($conn_id, "./In/"); foreach ($contents as $file) { if ($file == '.' || $file == '..') continue; if (@ftp_chdir($conn_id, $file)) { ftp_chdir ($conn_id, ".."); ftp_sync ($file); } else ftp_get($conn_id, $file, $file, FTP_BINARY); } ftp_chdir ($conn_id, ".."); chdir (".."); }
-
Basically, there is a secured site, which I can log into and then add data via a form once I'm logged in. I can pass the login information in the URL, and I can get it to work if, for example, I type the address into my browser bar, like so: [code=php:0]https://my.example.com/authentication.php?user=Username&pass=PW&destination=https://someurl.example.com/Something [/code] This will bring me to the page I want to post form data to. Which is great, except that if I use this url in my code, it doesn't work. It just brings me back to the initial login page. I should also say that if I use: [code=php:0] header("Location: https://my.example.com/authentication.php?user=Username&pass=PW&destination=https://someurl.example.com/Something"); [/code] I will be brought to the form that I want to post data to. The problem is that I don't want to actually redirect to the page, I just want to get to the form that I need to post information to. If I attempt to call this URL in my code, it doesn't work. There are multiple redirects that occur if I type it in the address bar, and I've used FOLLOWLOCATION, 1, but that doesn't seem to help. The latest iteration of code attempts to request the page that sets the cookies, and then make subsequent requests that include the cookies, but it still winds up kicking me back to the first page. [code=php:0] $cookie_jar = tempnam('/tmp','cookie'); $c = curl_init('https://my.example.com/authentication.php?user=Username&pass=PW'); curl_setopt($c, CURLOPT_RETURNTRANSFER, 1); curl_setopt($c, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($c, CURLOPT_COOKIEJAR, $cookie_jar); $page = curl_exec($c); curl_close($c); $c = curl_init('https://someurl.example.com/Something'); curl_setopt($c, CURLOPT_POST, 1); curl_setopt($c, CURLOPT_POSTFIELDS, 'field=value'); curl_setopt($c, CURLOPT_RETURNTRANSFER, 1); curl_setopt($c, CURLOPT_COOKIEFILE, $cookie_jar); $page = curl_exec($c); curl_close($c); [/code] Can anyone help?
-
Is there a way to have the effect of array_combined, but ensure that duplicate elements of an array are not removed? For example, the way things are now, this happens: [code=php:0]Array ( [name] => Array ( [0] => Joe [1] => Joe [2] => Joe ) [animal] => Array ( [0] => Dog [1] => Cat [2] => Rabbit ) [submit] => Validez ) [/code] Returns: [code=php:0]Array ( [Joe] => Dog [] => )[/code] What I would want is for it to return: [code=php:0]Array ( [Joe] => Dog [Joe] => Cat [Joe] => Rabbit)[/code] Upon array_combine. This is the array_combine function I'm using: [code=php:0]if (!function_exists('array_combine')) { function array_combine($a, $b) { $c = array(); if (is_array($a) && is_array($b)) while (list(, $va) = each($a)) if (list(, $vb) = each($b)) $c[$va] = $vb; else break 1; return $c; } } [/code]
-
When I print_r($_POST) to determine what's being posted from my form, I get this: [code=php:0] Array ( [name] => Array ( [0] => Joe [1] => [2] => [3] => [4] => [5] => ) [animal] => Array ( [0] => Dog [1] => Cat [2] => [3] => [4] => [5] => ) [submit] => Validez ) [/code] Then, I array_combine to (duh) combine the arrays, and I get this: [code=php:0]Array ( [Joe] => Dog [] => )[/code] I'd like to get this: [code=php:0]Array ( [Joe] => Dog [] => Cat) [/code] Anyone know how I can accomplish this?
-
The values of a field in my db looks like: [code]i.php, j.php, d.php?o=113, i.php, g.php, d.php?0=115, ... [/code] So, the following foreach statement will basically pluck the elements that start with d.php and display them. [code] preg_match_all('|d\.php\?.+?(?=,)|i', $row['longInfo'], $matchIt); foreach ($matchIt[0] as $sampX) { echo "$sampX<br />"; } [/code] The above foreach statement will generate the following: [code] // We'll call this part Main Blob d.php?o=113 d.php?o=113 d.php?o=113 d.php?o=113 d.php?o=115 d.php?o=115 [/code] What I actually need to display is: d.php?o=113 = 4 d.php?o=115 = 2 Can someone show me how to do this? I tried nesting another foreach statement, but keep receiving "Wrong parameter count" errors.