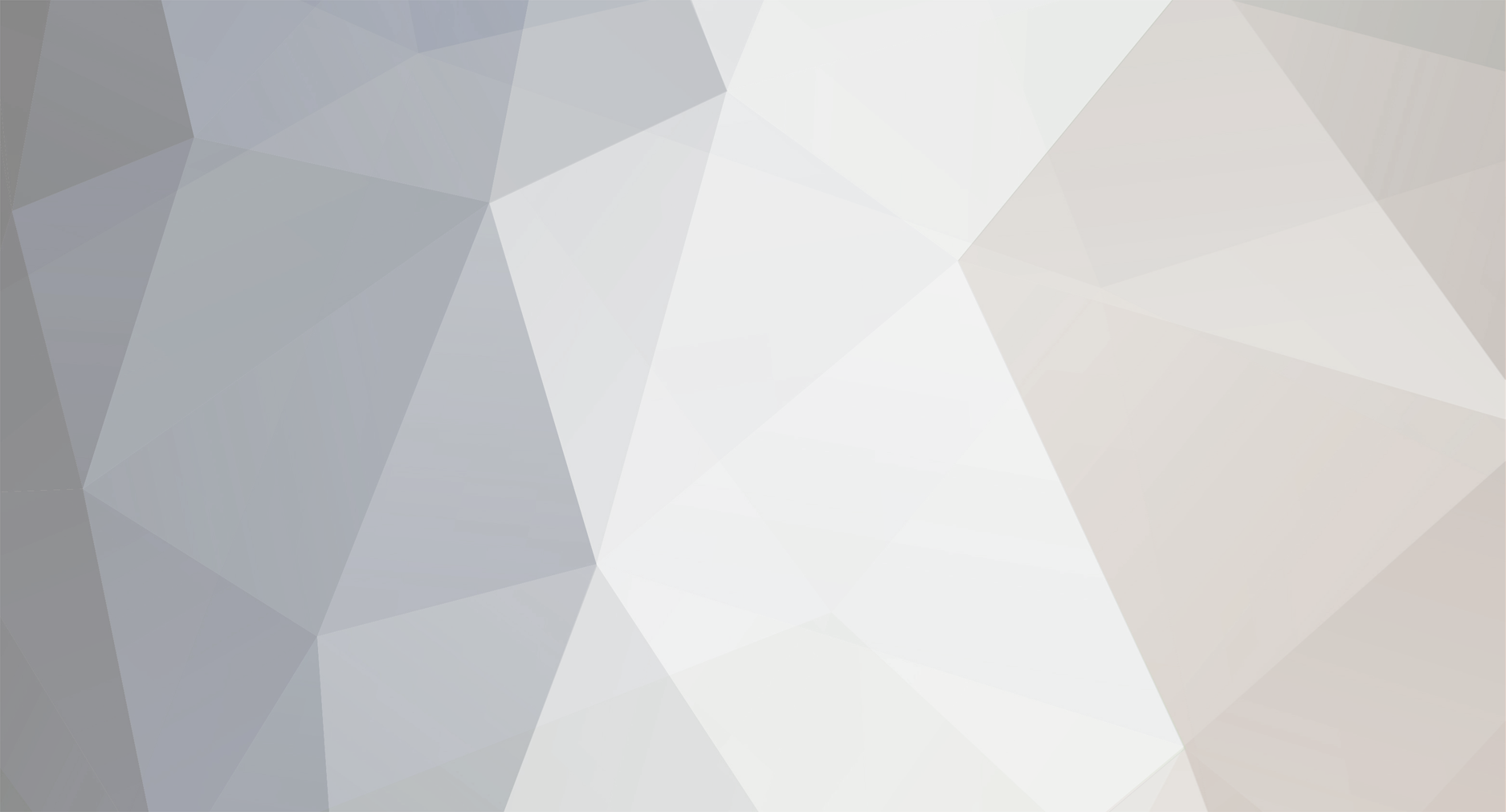
rossh
Members-
Posts
31 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
rossh's Achievements

Member (2/5)
0
Reputation
-
Hi I have the following code to filter xml data. I'm now able to query the xml file, but the results are not well formed, so i can't output specific elements. Does anyone know how to return element names and values from DomXpath? <?php $reader = new XMLReader(); $reader->open('include/staff.xml'); while ($reader->read()){ switch($reader->nodeType){ case(XMLREADER::ELEMENT): if($reader->localName === 'staff'){ $node = $reader->expand(); $dom = new DomDocument(); $dom->formatOutput = true; $n = $dom->importNode($node, true); $dom->appendChild($n); $xp = new DomXpath($dom); $res = $xp->query("//staff/member[groups='HR']"); } } } foreach($res as $staff){ echo $staff->nodeValue; } ?>
-
Hi I want to search an xml document, which could get quite large. The search should allow for a keyword search on specified tags and allow the end-user to filter by certain categories. An example xml file would be: <?xml version="1.0" encoding="UTF-8"?> <staff> <member type="management"> <title>Mr</title> <forename>Robert</forename> <surname>Bobby</surname> </member> I've created a class with a method which will return xml data based on specified categories. I'm using XMLReader with expand, dom and simpleXML, but i'm having difficulty adding in an xpath query string for the keywords. I have two questions: [*]Is this the most efficient way of searching a large document? The results from this search with be used in a search page. [*]How would i include an xpath query before i create the simpleXML array? Any help would be really appreciated. <?php class xmlParser{ public $file; public $node; public function __construct($aFile, $aNode){ $this->file = $aFile; $this->node = $aNode; } public function setFile($newFile){ $this->file = $newFile; } public function getFile(){ return $this->file; } public function setPath($newNode){ $this->node = $newNode; } public function getNode(){ return $this->node; } public function queryXML($types){ var_dump($types); $reader = new XMLReader(); $reader->open($this->file); $results = array(); while($reader->read()){ switch($reader->nodeType){ case(XMLREADER::ELEMENT): if($reader->localName == $this->node){ foreach($types as $type){ if($reader->getAttribute('type') == $type){ $node = $reader->expand(); $dom = new DomDocument(); $n = $dom->importNode($node,true); $dom->appendChild($n); $sxe = simplexml_import_dom($n); array_push($results, $sxe); $reader->moveToAttribute('type'); } } } } } return $results; } } $staff_details = new xmlParser('include/staff.xml', 'member'); $staff_details = $staff_details->queryXML(array('management', 'it', 'hr')); var_dump($staff_details) ?> This was my initial attempt, which could be okay for small xml files. This has the query string i need to include in my XMLReader function. <?php class xmlParser{ public $file; public $path; public function __construct($aFile, $aPath){ $this->file = $aFile; $this->path = $aPath; } public function setFile($newFile){ $this->file = $newFile; } public function getFile(){ return $this->file; } public function setPath($newPath){ $this->path = $newPath; } public function getPath(){ return $this->path; } public function countXMLObjects(){ if(file_exists($this->file)) { $data = file_get_contents($this->file); $xml = simplexml_load_string($data); }else{ exit('Sorry there seems to be a problem, please contact the administrator'); } return $count = count($xml->xpath("$this->path")); } public function queryXML($start=null, $limit=null){ if(file_exists($this->file)) { $data = file_get_contents($this->file); $xml = simplexml_load_string($data); }else{ exit('Sorry there seems to be a problem, please contact the administrator'); } $data = $xml->xpath($this->path); if(isset($start) && isset($limit)){ $data = array_slice($data, $start, $limit); //Used for paging } return $data; } } ?> xpath query i want to use: $query = "//staff/member[contains(translate(firstname,'ABCDEFGHIJKLMNOPQRSTUVWXYZ','abcdefghijklmnopqrstuvwxyz'),'$keywords')]
-
[SOLVED] Delete lines from htm file until body tag is found
rossh replied to rossh's topic in PHP Coding Help
I figured it out. <?php $base_dir = 'c:\\xampp\\htdocs\\work\\sanitize\\'; //CLEAN DATA HERE $sanitize = array( ' & ' => ' & ', '£' => '£', '£' => '£', '<b>' => '<strong>', '</b>' => '</strong>', '<i>' => '<em>', '</i>' => '</em>' ); //Output the current directories content function outputDir(){ global $base_dir; $my_dir = $base_dir.$my_dir; $dir = opendir($my_dir) or die('Couldn\'t open directory, please contact the web administrator'); while(($file = readdir($dir)) !== false){ if($file != '.' && $file != '..'){ $fname[] = $file; } } closedir($dir); foreach($fname as $file){ if(!is_dir($file) && $file != 'sanitize.php'){ $filenames .= $file.','; } } return $filenames = substr_replace($filenames ,'',-1); } //Read file, add title to anchor tags and output to new file function sanitizeFile($file){ global $base_dir, $sanitize; $lines = file($base_dir.$file); if(empty($lines)){ echo 'File: '.$file.' empty!'; } $new_lines = array(); foreach($lines as $line_num => $line){ if($found = strpos($line, 'body') == TRUE){ echo $body_tag_line = $line_num; } /*CLEAN DATA HERE*/ $line = preg_replace('/<a[^>]*?href=[\'"](.*?)[\'"][^>]*?>(.*?)<\/a>/si','<a href="$1" title="$2">$2</a>',$line); //Add titles to anchor tags foreach($sanitize as $search => $replace){ $line = str_replace($search, $replace, $line); } array_push ($new_lines, $line); } for($i=1;$i<$body_tag_line;$i++){ // unset($new_lines[$i]); } $content = implode('', $new_lines); $fp = fopen ($base_dir.'sanitized\\'.$file, w); fwrite ($fp, $content); fclose ($fp); } //Get filenames and sanitize if(isset($_POST['submit'])){ $filenames = outputDir(); $filenames = explode(',', $filenames); $response .= '<ul>'."\n"; foreach($filenames as $file){ sanitizeFile($file); $response .= '<li>Sanitized: '.$file.'</li>'."\n"; } $response .= '</ul>'."\n"; } ?> <p>Base Directory: <?php echo $base_dir; ?></p> <form name="sanitize_data" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <input type="submit" name="submit" value="Sanitize!" id="submit" /> </form> <?php echo $response; ?> -
Hi, I've been working on a sanitize script to clean html files. I'm trying to figure out how to delete lines from the top of the file until the <body> tag is found. Any help would be appreciated. R <?php $base_dir = 'c:\\xampp\\htdocs\\sanitize\\'; //CLEAN DATA HERE $sanitize = array( ' & ' => ' & ', '£' => '£', '£' => '£', '<b>' => '<strong>', '</b>' => '</strong>', '<i>' => '<em>', '</i>' => '</em>' ); //Output the current directories content function outputDir(){ global $base_dir; $my_dir = $base_dir.$my_dir; $dir = opendir($my_dir) or die('Couldn\'t open directory, please contact the web administrator'); while(($file = readdir($dir)) !== false){ if($file != '.' && $file != '..'){ $fname[] = $file; } } closedir($dir); foreach($fname as $file){ if(!is_dir($file) && $file != 'sanitize.php'){ $filenames .= $file.','; } } return $filenames = substr_replace($filenames ,'',-1); } //Read file, add title to anchor tags and output to new file function sanitizeFile($file){ global $base_dir, $sanitize; $lines = file($base_dir.$file); if(empty($lines)){ echo 'File: '.$file.' empty!'; } $new_lines = array(); foreach ($lines as $line){ foreach($sanitize as $search => $replace){ $line = str_replace($search, $replace, $line); } array_push ($new_lines, $line); } $content = implode('', $new_lines); $fp = fopen ($base_dir.'sanitized\\'.$file, w); fwrite ($fp, $content); fclose ($fp); } //Get filenames and sanitize if(isset($_POST['submit'])){ $filenames = outputDir(); $filenames = explode(',', $filenames); $response .= '<ul>'."\n"; foreach($filenames as $file){ sanitizeFile($file); $response .= '<li>Sanitized: '.$file.'</li>'."\n"; } $response .= '</ul>'."\n"; } ?> <p>Base Directory: <?php echo $base_dir; ?></p> <form name="sanitize_data" action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <input type="submit" name="submit" value="Sanitize!" id="submit" /> </form> <?php echo $response; ?>
-
Hi i've been looking into the Google Calendar API had how i can add events via PHP. I downloaded the Zend Framework and installed it. Seems to working fine, but i can't get the sample Google Calendar scripts to work. When i run the code below, i get an error (also below). Reading online it looks like it's something to do with OpenSSL and Windows. I've followed various setting adding .dll files to windows\system32 and the environment variable PATH, but it's still not working. If anyone has any suggestions i'd really appreciate it. Ross <?php include("calendar.php"); $user = '***@googlemail.com'; $pass = '***'; $tzOffset = '0'; if(isset($_POST['submit'])){ $service = Zend_Gdata_Calendar::AUTH_SERVICE_NAME; $client = Zend_Gdata_ClientLogin::getHttpClient($user,$pass,$service); createEvent($client, "ACC--".$_POST['title'], $_POST['desc'], $_POST['project'], $_POST['date'], "09:00", $_POST['date'], "09:15", $tzOffset); }else{ ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> Title: <br> <input type="text" name="title"><br> Description: <br> <textarea rows="5" cols="80" name="desc"></textarea><br> Project: <br> <input type="text" name="project"><br> Date of Accomplishment:<br> <input type="text" name="date"><br> <input type="submit" name="submit" value="submit"> </form> <?php } ?> ERROR: Fatal error: Uncaught exception 'Zend_Gdata_App_HttpException' with message 'Expected response code 200, got 400' in C:\xampp\php\library\Zend\Gdata\App.php:468 Stack trace: #0 C:\xampp\php\library\Zend\Gdata\App.php(459): Zend_Gdata_App->post(Object(Zend_Gdata_Calendar_EventEntry), 'http://www.goog...', 4, 'application/ato...', Array) #1 C:\xampp\php\library\Zend\Gdata\App.php(663): Zend_Gdata_App->post(Object(Zend_Gdata_Calendar_EventEntry), 'http://www.goog...') #2 C:\xampp\php\library\Zend\Gdata\Calendar.php(156): Zend_Gdata_App->insertEntry(Object(Zend_Gdata_Calendar_EventEntry), 'http://www.goog...', 'Zend_Gdata_Cale...') #3 C:\xampp\htdocs\dev\cal\calendar.php(384): Zend_Gdata_Calendar->insertEvent(Object(Zend_Gdata_Calendar_EventEntry)) #4 C:\xampp\htdocs\dev\cal\index.php(11): createEvent(Object(Zend_Http_Client), 'ACC--Test', 'Test', 'Test', '07/05/2008', '09:00', '07/05/2008', '09:15', '0') #5 {main} thrown in C:\xampp\php\library\Zend\Gdata\App.php on line 468
-
Hi i tried out the image upload tutorial on phpFreaks and it worked great on my test server. I'veuploaded it to my host server and it works but it's fails randomly. I get a permission error on copy(). It only happens if you upload more than 2,3 files in a row, and it will be back to normal again a short time after. The directory i'm writing to is set to 777. Speaking to the hosting company they believe it's not possible to upload files as this ability has been removed, but i can upload. Is there any ideas why it would be acting like this or is there an alternative solutioin. I basically need to upload a jpeg resize and create a thumbnail also. Any help would be appreciated.
-
Hi i have the following mySql tables:- property --------- property_id (PK) ... image --------- image_id (PK) ID_property (FK) ... $sql = "SELECT * FROM property LEFT JOIN image ON image.ID_property = property.property_id WHERE property_id = ".$_GET['property_id']." GROUP BY property_id;"; I have the sql statement which displays 1 record which is great, but i also want to display the > 1 images that are in the database, at the moment it just displays 1 image? Thanks Ross
-
Cheers, just went live today and got mixed reactions. 1 person called the font unprofessional? Bit mad, but just thought i'd get some second opinions. Thanks again R
-
Hi wondered if someone can take a look at my site. Comments welcome http://www.dundee.ac.uk/eswce/ Thanks Ross
-
Hi thanks for getting back to me. I did that originally but it was getting difficult to update if i needed to make changes to the stylesheet. Any ideas why random pages whould revert to the previous style? I've printed the session and cookie variables when this happens and they are the have the correct value but the wrong style? Thanks R
-
Hi i'm trying to get a site working with different styles and i'm changing variables in one style.php file which was working fine, however every now and again the old style reappears on random pages? Don't know if it's my conditional statements or a cache problem? I have various areas on the site that are secure https and i had to add the domain for the cookie to work in the secure areas. I could probably figure it out if the error was not random. Also i'm guessing this is not the best solution if anyone can let me know the best way to go about this i would really appreciate it. Thanks R Style.php <?php session_start(); header('Content-type: text/css'); if ($_SESSION['style'] == ""){ $logo = "../image/logo-purple.gif"; }else{ $logo = "../image/logo-".$_SESSION['style'].".gif"; } if ($_SESSION['color'] == ""){ $color = "#9B83B7"; }else{ $color = $_SESSION['color']; } ?> header.php session_start(); require_once ("functions.php"); if ($_SESSION['style'] == ""){ $_SESSION['style'] = "purple"; } if ($_COOKIE["style"] != ""){ $_SESSION['style'] = $_COOKIE["style"]; } if(isset($_GET['style']) && $_GET['style']!=""){ $_SESSION['style'] = $_GET['style']; } setcookie ("style"); //Delete existing entry setcookie('style', $_SESSION['style'], time() + (86400 * 30), '/', '.domain.com'); //.domain.com to deal with https $style = $_SESSION['style']; switch ($style){ case "purple": $_SESSION['color'] = "#9B83B7;"; break; case "green": $_SESSION['color'] = "#8DC63F;"; break; case "pink": $_SESSION['color'] = "#EC008C;"; break; case "orange": $_SESSION['color'] = "#F26521;"; break; case "brown": $_SESSION['color'] = "#726257;"; break; case "blue": $_SESSION['color'] = "#448CCB;"; break; case "print": $_SESSION['color'] = "#000;"; break; default: $_SESSION['color'] = "#9B83B7;"; }
-
Hi i want to be able to remove everything after a question mark in a string. Can someone help me out. Thanks Ross
-
Hi thanks for getting back to me. I've tried both:- Options +FollowSymlinks RewriteEngine on AddType application/x-httpd-php .htm AddType application/x-httpd-php .html and Options +FollowSymlinks RewriteEngine on RewriteRule ^(.+)\.html$ $1.php and both give me a 500 error? Thanks again R
-
Hi, i was trying this example Options +FollowSymlinks RewriteEngine on RewriteRule ^(.*)\.htm$ $1.php [nc] which i don't think is really what i want but i get the 500 error right away no pages from the subdirectory will load. Thanks Ross
-
Hi thanks i've tried using mod_rewrite already and i'm getting an 500 internal server error? my site is in a subdirectory does this make a difference? I've looked on php.ini and it look like it there? Thanks R