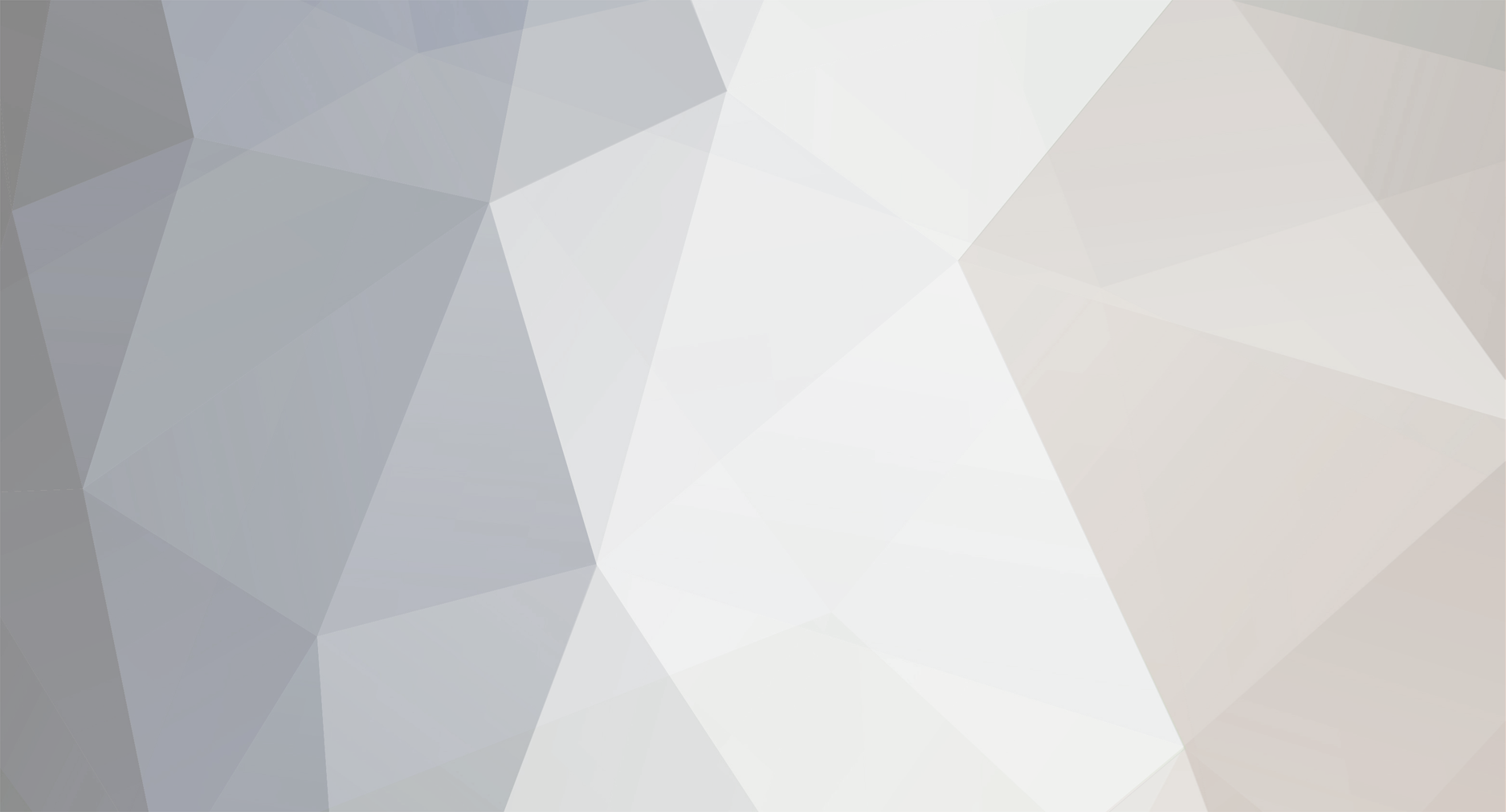
Nelalen
New Members-
Posts
8 -
Joined
-
Last visited
Nelalen's Achievements

Newbie (1/5)
0
Reputation
-
Sorry, yes I just thought of the login script where I require the email as input. It matches it to an existing entry into the database. I'm guessing I need to query the database to assign the email? Apologies if I seem like a bit of an idiot...I've only been learning programming this first semester. Very little prior experience.. Here's the login script: <?php //check for required fields from the form if ((!filter_input(INPUT_POST, 'email')) || (!filter_input(INPUT_POST, 'password'))) { //if ((!isset($_POST["username"])) || (!isset($_POST["password"]))) { header("Location: userlogin.html"); exit; } //connect to server and select database $mysqli = mysqli_connect("localhost", "cs213user", "letmein", "testDB"); //create and issue the query $targetname = filter_input(INPUT_POST, 'email'); $targetpasswd = filter_input(INPUT_POST, 'password'); $sql = "SELECT firstname, lastname FROM members WHERE email = '".$targetname. "' AND password = PASSWORD('".$targetpasswd."')"; $result = mysqli_query($mysqli, $sql) or die(mysqli_error($mysqli)); //get the number of rows in the result set; should be 1 if a match if (mysqli_num_rows($result) == 1) { //if authorized, get the values of firstname lastname while ($info = mysqli_fetch_array($result)) { $firstname = stripslashes($info['firstname']); $lastname = stripslashes($info['lastname']); } //set authorization cookie setcookie("auth", "1", time()+60*30, "/", "", 0); //create display string $display_block = " <p>".$firstname." ".$lastname." is authorized!</p> <p>Authorized Users' Menu:</p> <ul> <li><a href=\"secretpage.php\">Lottery Ticket Selection</a></li> <li><a href=\"fileupload.html\">Upload A File</a></li> </ul>"; } else { //redirect back to login form if not authorized //header("Location: userlogin.html"); //exit; echo "wrongone"; } ?> <html> <head> <title>User Login</title> </head> <body> <?php echo "$display_block"; ?> </body> </html> And the html form: <html> <head> <title>User Login Form</title> </head> <body> <h1>Login Form</h1> <form method="post" action="userlogin.php"> <p><strong>Email:</strong><br/> <input type="text" name="email"/></p> <p><strong>Password:</strong><br/> <input type="password" name="password"/></p> <p><input type="submit" name="submit" value="login"/></p> </form> <h1> Don't have an account?</h1> <a href ='applyaccount.php'>Click here!</a> </body> </html>
- 6 replies
-
- file upload
- file
-
(and 3 more)
Tagged with:
-
Sorry, wasn't sure how to do it and was getting ready for bed. We created a form previously to create a new user where they can input their email as well as some other information. I then used something like mkdir(var/www/html/$email,0733) to create their directory and figured I should be able to use that again on this page. I tried defining it like this: $email = (filter_input(_POST["email"])); previously but it would break the page. Where should I be defining $email? and if I used the post method on the form it should be stored as POST correct? I'm a little confused as to how I can retrieve the variable from the form. When I put in the query to the server I had to make it lowercase $targetemail = strtolower($email); Do I need to use this as the email from the form may not be written in lowercase?
- 6 replies
-
- file upload
- file
-
(and 3 more)
Tagged with:
-
The code works but it puts the files into /uploadir/. The users directories go by their email addresses ($email). Using the error reporting it tells me: ! ) Notice: Undefined variable: email in /var/www/html/Lab5/uploadfile.php on line 10 Call Stack # Time Memory Function Location 1 0.0010 129288 {main}( ) ../uploadfile.php:0 Any help is much appreciated!! <?php error_reporting(E_ALL | E_NOTICE); ini_set('display_errors','1'); session_start(); if ($_COOKIE["auth"] == "1") { $file_dir = "/var/www/html/uploaddir/$email"; foreach($_FILES as $file_name => $file_array) { echo "path: ".$file_array["tmp_name"]."<br/>\n"; echo "name: ".$file_array["name"]."<br/>\n"; echo "type: ".$file_array["type"]."<br/>\n"; echo "size: ".$file_array["size"]."<br/>\n"; if (is_uploaded_file($file_array["tmp_name"])) { move_uploaded_file($file_array["tmp_name"], "$file_dir/".$file_array["name"]) or die ("Couldn't copy"); echo "File was moved!<br/>"; } } } else { //redirect back to login form if not authorized header("Location: userlogin.html"); exit; } ?>
- 6 replies
-
- file upload
- file
-
(and 3 more)
Tagged with:
-
I totally understand. I'm struggling since we've only spent 2 wks on php and he doesn't entirely seem to understand it well himself. The number stuff me and a partner had somewhat figured out now but your code adds on the couple pieces we were missing. Does this seem like the kind of project we should be doing so soon? We're thinking this course is expecting too much and we were going to bring it up with the prof. (it's his first time teaching this specific course). A lot of others seem to be lost as well. Thank you for the code; it really helps! The main issue we're trying to figure out is how the info we put into the checkboxes is processed. I'd think we would output the checkbox data to another php file containing the generator and then taking the output from that and echo-ing the answers with fancy html/css. Thing is we aren't sure how to access the information to process it or store the data to output on another page. Overall, PHP is unbelievably confusing right now.. Thank you for the help though it is much appreciated :]
-
Struggling with creating the following: a. The authorized user can select one or both of the two available lottery services (in form of checkboxes) provided by your website. Generate six sets of LOTTO 6/49 numbers (sorted in ascending order) when option one, labeled as “LOTTO 6/49”, is checked. One set of “LOTTO 6/49” numbers consists of 6 unique numbers between 1 and 49 inclusively. Generate six sets of LOTTO MAX numbers (sorted in ascending order) when option two, labeled as “LOTTO MAX”, is checked. One set of “LOTTO MAX” numbers consists of 7 unique numbers between 1 and 49 inclusively. b. When the authorized user submits the form with one or both of the two available services checked, your web server will generate six sets of lottery numbers for each type accordingly and display these numbers of each type of lotteries to the user. Code so far: <?php error_reporting(E_ALL | E_NOTICE); ini_set('display_errors','1'); if(!isset($_SESSION)){ session_start(); } ?> <html> <head> <title> Choose Tickets</title> </head> <body> <h2> Choose your tickets</h2> <?php if (filter_input(INPUT_POST,'form_tickets')){ if(!empty($_SESSION["tickets"])){ $tickets = array_unique( array_merge(unserialize($_SESSION["tickets"]), filter_input(INPUT_POST, 'form_tickets'))); $_SESSION["tickets"] = serialize($tickets); } else { $_SESSION["tickets"] = serialize(filter_input(INPUT_POST, 'form_tickets')); } echo "<p> Your tickets have been selected!</p>"; } ?> <form method="POST" action=""> <p><strong>Select your ticket(s):</strong><br> Lotto 6/49: <input type="checkbox" value="Lotto 6/49"><br /> Lotto Max: <input type="checkbox" value="Lotto Max"><br /> <p><input type="submit" value="submit"/></p> </form> </body> </html> I honestly don't really know what I'm doing.. This class isn't specifically php so he's going through it too quick for me to grasp/learn. I'm sort of going off examples he's given us but I can't seem to fit the pieces together to make this thing. Again, any help is greatly appreciated I have a vague idea of how it's all supposed to work but not sure of the commands to do it or how to structure it.
-
I got it working!! Thanks so much!! I was worried since I had to submit it by tonight! I will definitely be back here for any more questions in the future!!
-
So this should be opening in browser and appearing correctly?? I suppose that's why the prof was confused as am I.. We've been having issues with the permissions on the server changing. I'll try taking another look at those and try a different browser to open.. Thank you! For anyone else please feel free to point out any other mistakes or syntax errors you find!
-
My prof hasn't gotten back to me and he doesn't seem like even he knows how to do it so I'm posting here for some help. We've barely done any script writing yet and I can't seem to figure out how to go about doing it. He wants us to modify the existing script so that it generates a random number from 1 - 1000 and then counts the number of guesses and outputs how many guesses at the end. It won't open in the browser so I'm not sure what's wrong. Any help would be much appreciated! This is what I have so far: <?php if (!isset($_POST["guess"])) { $message = "Welcome to the guessing machine!"; $_POST["numtobeguessed"] = rand(1,1000); $_POST["counter"] = 0; } else if ($_POST["guess"] > $_POST["numtobeguessed"]) { $message = $_POST["guess"]." is too big! Try a smaller number."; $_POST["counter"]++; } else if ($_POST["guess"] < $_POST["numtobeguessed"]) { $message = $_POST["guess"]." is too small! Try a larger number."; $_POST["counter"]++; } else { // must be equivalent $message = "Well done! It took you '$_POST["counter"]' tries!"; } ?> <html> <head> <title>A PHP number guessing script</title> </head> <body> <h1><?php echo $message; ?></h1> <form action="" method="POST"> <p><strong>Type your guess here:</strong> <input type="text" name="guess"></p> <input type="hidden" name="numtobeguessed" value="<?php echo $_POST["numtobeguessed"]; ?>" ></p> <input type="hidden" name="counter" value="<?php echo $_POST["counter"]; ?>" <p><input type="submit" value="submit your guess"/></p> </form> </body> </html>