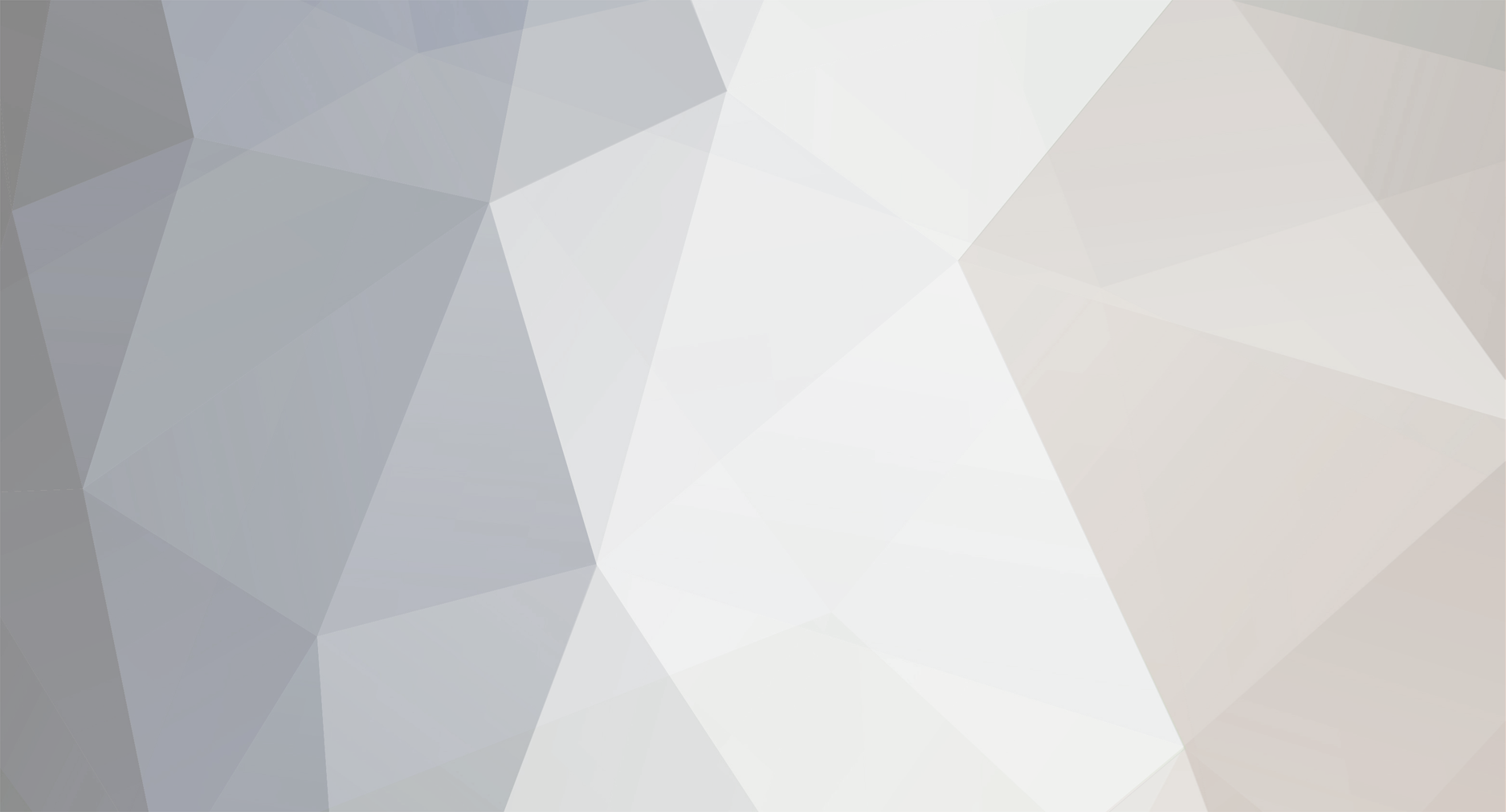
ManiacDan
-
Posts
2,604 -
Joined
-
Last visited
-
Days Won
10
Posts posted by ManiacDan
-
-
I'll chip in and we can triple Barand's pay.
-
Perhaps it's not writing to the file because it doesn't exist. Create the file, fix the permissions, and try again. Also put the file in php.ini just to be safe.
-
An easier way to answer this question is:
1) Write an erroneous PHP page.
2) Visit it.
3) Check the default error location in lighttpd.conf
-
Java supports POST.
The rest of my advice still applies.
-
You should probably anchor your exp<b></b>ression (and end statements with a semicolon), but otherwise Zane is right:
if(preg_match("#^[0-9]{3}-[0-9]{3}-[0-9]{4}$#", $phoneNumber)) echo "valid phone number";
-
Quotes are required around strings in mysql:
mysql_query ("INSERT INTO `reports`(`user`, `reportedplayer`, `by`, `reason`) VALUES ('$myusername', '$reportedplayer', '$by', '$reason')");
You can't just stick an echo after mysql_query() and expect that to tell you if the query succeeded. You need to actually handle the error:
$result = mysql_query ("INSERT INTO `reports`(`user`, `reportedplayer`, `by`, `reason`) VALUES ('$myusername', '$reportedplayer', '$by', '$reason')"); if ( $result === false ) { echo "MySQL Error Encountered: " . mysql_error(); }
Edit: Also, if you're using GET for this form, stop. Use POST. You should never send usernames and passwords over GET, they show up in the URL. You also must run these values through mysql_real_escape_string(). Move your mysql_connect up to the top of the script, and then:
$myusername = mysql_real_escape_string($_POST['user']);
-
Commas at the ends of the array declaration are valid, oddly enough.
There are two places in PHP where errant commas are valid:
1) Trailing commas in array declarations:
$a = array( 1,2,3, );
2) Initial commas within list():
list(,$a) = explode(",", $Foo);
-
Of course, go right ahead. Make a new thread for every general question you have.
-
These scripts were poorly written, even "back then." This line, for example, is wrong and has always been wrong. It was only through a fluke of multiple overlapping layers of wrong that it managed to work in the first place.
-
How does levenshtein work for you at all? Maybe "jo" to "john" would have a distance of 4, but it would have a distance of 1 to "Bo".
-
Very true as a general note, his queries didn't use joins so I didn't mention it. Foreign keys are sometimes indexed, but new users especially rarely know they even exist.Just FYI. Fields used in the ON clause of a JOIN matter as well. Since those are usually FOREIGN KEYS, they are usually indexed anyway; but it bears mentioning here.
-
You're kidding. You must be kidding. You've been asking us to fix a FAKE query all day?
-
Run an EXPLAIN on the query and see if it's using the right indexes.
-
Your queries filter on artist and title, that's all. The items in the WHERE are what matter.
-
24/7...every hour?
How many inserts per second are you doing? If the answer is less than 10, you can enable proper indexes. Your database is relatively small.
-
$sth2=mysql_query($query2); $Location=$sth2['fullname'];
If "not working' means "PHP throws a serious warning about this line being wrong," then you're not calling the appropriate fetch function on your result.
If "not working" means "no errors, I just don't know what's happening," turn errors on, then see sentence #1.
-
Jo and Ja are not initials, they're some portion of the first name.
You have to do heavy processing of this on the PHP end to turn it into something like:
WHERE (firstname LIKE 'Jo%' OR lastname LIKE 'Jo%') AND (firstname LIKE 'Doe%' OR lastname LIKE 'Doe%');
Even then, you may get false positives since there's no way to tell which order the names are in.
-
Session_start() must be on every single page which uses the session, and if you don't die() after your header call, the session may not save properly (though in your instance it proably will regardless).
-
if ($_SESSION["authorized"] = true) {
= is assignment.
== is comparison.
-
Please use [ code ] or [ php ] tags so we can actually see your code properly.
There's nothing I could see in login.php that would automatically do a redirect. Are you sure you're hitting that page? How are you being redirected? Do you have firebug or anything installed so you can confirm the header() calls are working?
You should call die(); after every header redirect.
-
I can't believe there's not a custom-unique function...weird.
This is a handshake problem. A quick and dirty solution to it is:
foreach ( $array as $key => $val ) { foreach ( $array as $key2 => $val2 ) { if ( $key == $key2 ) continue; if ( $val['b'] == $val2['b'] && $val['c'] == $val2['c'] ) { //the current pair is a duplicate according to your criteria } } }
Note that this is inefficient, for loops would be better, but this is easier to understand.
-
Just to be clear: This method works perfectly well for every non-reserved character. Characters like @, #, &, ?, /, and = are reserved for use as URL delimiters, which is why they need to be escaped. All you'd have to do is change #hello to $hello (or something else) and it would have started working. Unless you're relying on the specific behavior of jump-to-tag while also trying to get that tag in a PHP script, in which case you should know that jumping to tags on the same page never refreshes the page, so PHP never happens.
-
If the string is UTF-8, the string is UTF-8. All characters in the string are UTF-8 encoded. Strings aren't like MP3s, they're not variable bitrate.
If, like requinix said, you want to find the first character in the string which has a multi-byte encoding, then that's a whole other question and you could conceivably do it in regex with a unicode range. Look up how to do unicode characters in regex (something like \u123), then put a range [a-z] in your code where A is the first character you want to detect and Z is the maximum possible value.
Or just use string functions.
-
Also, $_GET is an array, not a function. It's a regular variable except for its scope.
&body Not Validating In Mailto
in HTML Help
Posted
What do you mean "validate" it? Normally URLs must be run through urlencode(), but first you have to tell us what you mean by validate.