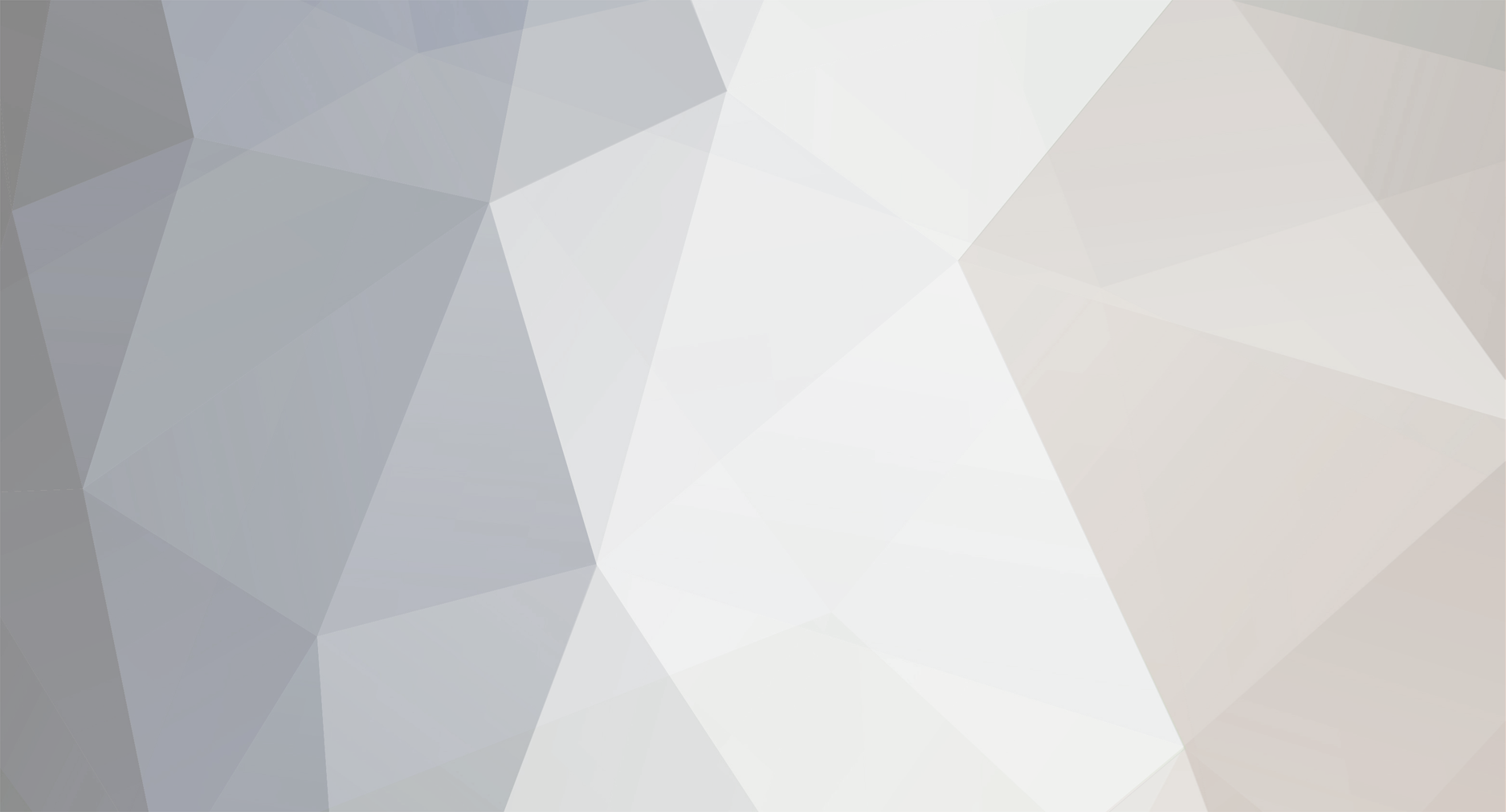
dadamssg87
Members-
Posts
218 -
Joined
-
Last visited
Everything posted by dadamssg87
-
I dropped a table. And now i'm trying to create a new table to replace it with the same name but apparently the old table still exists even though its not showing in the left sidebar of phpmyadmin. Anybody know what's going on?
-
checking to see if a date lands in a season
dadamssg87 replied to dadamssg87's topic in PHP Coding Help
oh and what does it matter if the dates are beyond 2037? -
checking to see if a date lands in a season
dadamssg87 replied to dadamssg87's topic in PHP Coding Help
that took a lot of rereading but i think i understand now. i appreciate it. I was trying to figure out how to do it if the range spanned two years(ex. start: 12/25/2010 End: 2/14/2011) but i think it would make more sense and it be easier if i just make sure if they user checks the ongoing box that the validation throws an error if the two dates aren't in the same year. -
I have a an array of dates, an array of normal weekly rates, and an array of seasonal rates. Items in the seasons array consist of: start, end, ongoing, and weekly rates. If the ongoing value is "Y" the season should apply to every year. If the value is "N" then the season should only last for the specified range. The below code is doing what i want except I just don't know how to incorporate the ongoing-ness of the seasonal rates. The last date in the dates array should affected by the second seasonal rates. Can't think of how to go about figuring this out. <?php $dates[] = "1/26/2010"; $dates[] = "4/13/2011"; $dates[] = "4/19/2034"; $normal_rates = array( 'monday' => 0.99, 'tuesday' => 0.99, 'wednesday' => 0.99, 'thursday' => 0.99, 'friday' => 0.99, 'saturday' => 1.50, 'sunday' => 1.50, ); $seasons[] = array( 'start' => "3/15/2011", 'end' => "4/25/2011", 'ongoing' => "Y", 'monday' => 4.99, 'tuesday' => 4.99, 'wednesday' => 4.99, 'thursday' => 4.99, 'friday' => 4.99, 'saturday' => 10.99, 'sunday' => 10.99, ); $seasons[] = array( 'start' => "3/15/2011", 'end' => "4/25/2011", 'ongoing' => "N", 'monday' => 1.99, 'tuesday' => 1.99, 'wednesday' => 1.99, 'thursday' => 1.99, 'friday' => 1.99, 'saturday' => 19.99, 'sunday' => 19.99, ); foreach($dates as $date) { $x_timestamp = strtotime($date); $day_of_the_week = strtolower(date("l", strtotime($date))); $date_rates[$date] = $normal_rates[$day_of_the_week]; foreach($seasons as $key => $value) { $s_timestamp = strtotime($seasons[$key]['start']); $e_timestamp = strtotime($seasons[$key]['end']); if($x_timestamp >= $s_timestamp && $x_timestamp <= $e_timestamp) //should have an or(||) in it for ongoing { $date_rates[$date] = $seasons[$key][$day_of_the_week]; } } }
-
this great, thanks!
-
i have two multi-dimensional arrays that i'd like to manipulate to check a few things. Their structures are different and i'm giving myself headaches trying to think through this. I have a $sums array which has information on a product in a purchase order. The second array consists of items(products) that are going to update the purchase order. So the first array key of $sums correlates with the "order" of the $items array. I'd like to write a function that: 1. Makes sure the first array key of $sums exists as an "order" value in the $items array. 2. Makes sure the $items price is greater than the $sums price <?php $sums['23']['collected']['price'] = 30; $sums['73']['collected']['price'] = 60; $sums['89']['collected']['price'] = 90; $items['abc123']['order'] = '23'; $items['abc123']['price'] = 14; $items['321cba']['order'] = '73'; $items['321cba']['price'] = 79; function check_items($sums,$items) { $error = ""; //Makes sure the first array key of $sums exists as "order" value in the $items array. //If not -> $error = "Item #".$key." is not found in the items array."; //where $key is either the first array key of $sums or the "order" value in $items because they are the same thing //if found in $sums if($items[$hash]['price'] < $sums[$items[$hash]['collected']['price'] ) { $error = "Item #".$items[$hash]['order']."'s price must not be lower than ".$sums[$items[$hash]['order']]['collected']['price']; } return $error; } // $sums['23'] would cause an error because its price of 14 is less than 30 // $sums['73'] would pass because it exists in $items and its price of 79 is greater than the $sums price of 60. // $sums['89'] would cause an error because there is no $item corresponding to it ?> I just don't know where to put the foreach loops or where to even start really :/
-
works great. Thanks a lot. I would've wrote something way more complicated than need be. I appreciate it!
-
I have an array of products. The array has an ID and price. I'd like to know the most efficient way of adding up all the same ID fields in the array. <?php $items[] = array( 'id' => 1, 'price' => 5.99, ); $items[] = array( 'id' => 2, 'price' => 1.99, ); $items[] = array( 'id' => 3, 'price' => 2.40, ); $items[] = array( 'id' => 2, 'price' => 6.99, ); $items[] = array( 'id' => 4, 'price' => 8, ); $items[] = array( 'id' => 2, 'price' => 3, ); $items[] = array( 'id' => 3, 'price' => 1, ); function add_like_items($items) { //manipulation return $sums; } //$sums would be an array like //$sum[1] = 5.99 //$sum[2] = 11.98 //$sum[3] = 3.40 //$sum[4] = 8 ?> Anybody have suggestions on the best way to go about doing this?
-
wow, never knew looping could be so complicated haha. Thanks for the write up!
-
haha yeah. That was the only instance that i was storing data stupidly and i've fixed that. But that doesn't have anything to do with this question
-
eh, not really. Just trying to optimize where ever possible. I thought if i did the unsetting it would make it run faster, even if it is an insignificant amount. Thanks for the answer!
-
I was wondering if you loop through an array and in the foreach() you specify an if statement that unsets certain items from the array, if those get unset from the original loop. Probably doesn't make much sense so heres an example <?php //say there are 3 items in the $parent array //say there are 10 items in the $items array //say only the second item in the $items array value_one equals "abcd" foreach($parent as $id => $value) { foreach($items as $key => $value) { if($items[$key]['value_one'] == "abcd") { unset($items[$key]); } } } ?> So on the first item in the parent array, all 10 items would be cycled through. On the second second item of the parent array, all 10 items would be cycled through and the one item that has value_one == "abcd" would be unset. So on the third item of the parent array, am i correct in assuming only 9 items would be cycled through because there would be only 9 items in the $items array?
-
updating records based on what they contain in a certain field
dadamssg87 replied to dadamssg87's topic in MySQL Help
dangit...you're definitely right -
I am storing products in my database. The table has id, price, title, description, and add_ons. The add_ons column stores id's of other products that can be purchased with it. These id numbers are separated by commas and there's no limit to how many id numbers can be in the column. So for instance a typical product row will look something like this 34|9.99|Box of Candy|Assorted bag of candy|31,32,56,30 Now i'm trying to figure out the best way to delete a product. If i'm deleting product item 30, i don't want that "30" to still be in the add_ons column of item 34. I didn't know if there was a more efficient way of doing something like the following <?php $item_to_delete = 30; $query = $this->db->query("SELECT * Products WHERE username = '$username' ORDER BY created DESC"); foreach($query->result() as $row) { $add_ons = explode(",",$row->add_ons); foreach($add_ons as $key => $id) { if($id = $item_to_delete) { unset($add_ons[$key]); $add_ons = implode(",",$add_ons); $this->db->query("Update Products SET add_ons = '$add_ons' WHERE id = '$row->id'"); } } } ?>
-
I'm writing a shopping cart type of application for booking something. The cart will holds items as arrays in the session. The problem i'm i'm having is i can store 5 items in my cart and then once i try to store any more, it just won't. I'm not getting any php errors. I'm using the codeigniter framework, so it may be a codeigniter session storage problem. Hopefully you guys can look at it and can see if you spot any bad logic that i'm overlooking. <?php //generates random unique 32 character string $booking_hash = random_string('unique'); //set items foreach night foreach($room['date_rate'] as $date => $rate) { //produces random sha1 16 char length string $sha1 = random_string('sha1', 16); $session['items'][$sha1] = array( 'code' => $room['code'], 'id' => $room_id, 'title' => $room['title'], 'date' => date("n/j/Y",strtotime($date)), 'price' => $rate, 'tax_one' => money_format('%i',$room['tax_one'] * $rate), 'tax_two' => money_format('%i',$room['tax_two'] * $rate), 'sales_tax' => 0, 'guests' => $num_guests, 'booking' => $booking_hash, 'deposit' => $deposit, ); } $existing_items = $this->session->userdata('items'); if(!empty($existing_items)) { $session['items'] = $existing_items + $session['items']; } $this->session->set_userdata($session); ?> I just read some codeigniter session documentation. It says the cookie it's using can hold 4KB of data. I have no idea how much text 4KB can hold...
-
i'm not sure i understand enough about php's default method to store session data to use that function. I see that in one guy's example in the comments section he uses the blob datatype. I can't say i understand the blob datatypes. I also don't understand how arrays get stored by the session. Could you possibly show me a really simple way to store session data in a database table effectively?
-
i'm kind of noob, what exactly do you mean by "compile"?
-
My application i'm developing has the potential to store, what i consider, quite a bit of data into sessions per user and it keeps breaking. I can't say for certain that it's a session storage issue. I'm storing shopping cart items and after i store a certain amount of items and try to add more it will keep the old items but won't store the new items. So i'm 90% sure it's the session storage limit. What i'm considering doing is only storing a session id in the session. Then as the user adds items to their cart, those items get stored temporarily in a table in my database with that session id. So instead of pulling it from my session, i'd be pulling it from a certain database table. I'm guessing thats the best way to handle this issue? I'm developing locally with MAMP and all its default settings.
-
array_merge_recursive was exactly what i was looking for. Thanks!
-
I have two arrays, both with the same key values. I'd like to combine them. So for instance... <?php $array1['abcd'] = array( 'value1' => "blah", 'value2' => "blahblah"); $array1['efgh'] = array( 'value1' => "ha", 'value2' => "haha", 'valuex' => "xyz"); $array2['abcd'] = array('value3' => "three", 'value4' => "four"); $array2['efgh'] = array( 'value3' => "hohoho", 'value6' => "six6"); function combine_arrays($array1,$array2) { //*combining* return $single_array; } echo "<pre>"; print_r(combine_arrays($array1,$array2)); echo "</pre>"; /* would produce ['abcd'] = ( 'value1' => "blah", 'value2' => "blahblah", 'value3' => "three", 'value4' => "four" ) ['efgh'] = ( 'value1' => "ha", 'value2' => "haha", 'valuex' => "xyz", 'value3' => "hohoho", 'value6' => "six6" ) */ ?> What's the easiest way to do this?
-
Right now, my users can delete pieces of content that they've created and the rows really do get deleted from the database. I'd like to change this by adding a "deleted" column in my database table. The column datatype needs to hold the date/time of deletion. So i'm thinking datetime, timestamp, or int(11). I'm just not sure which datatype is ideal. I was just going to set the datatype to int(11) to store the integer value of the timestamp of when the row was "deleted". Then i could just query non-deleted items by "select * from table where deleted != '0'". But i think i should be using datetime or timestamp. I don't really understand the difference between the two. What do the mysql wizards suggest?
-
I wrote a function that produces two form input fields into a div when a link is clicked. The input's default value's are passed through the javascript function. These values are produced by php. I can't figure out how to pass single quotes through it though. I'm using the addslashes() php function on the values before being placed into the onClick function. This handles double quotes fine. But a single apostrophe breaks the function. Has anybody got a clue on what i'm doing wrong? Both addslashes() and addcslashes($value, '"') work for double quotes but but not single. PHP: <?php $code = $add_ons[$id]['code']; $display_title = $add_ons[$id]['title']; $atitle = addslashes($add_ons[$id]['title']); // $atitle = addcslashes($add_ons[$id]['title'], '"'); $price = $add_ons[$id]['price']; echo "<a href='javascript:void;' onclick='add_item(\"$code\",\"$atitle\",\"$price\",\"#add_on_box\")' >$display_title</a>"; ?> Javascript: function add_item(code, title, price, divbox) { var idtag, item_title, item_price, item_code, separator; var room_code, code_text; code_text = "<div id='code_text'>" + code + "</div>"; separator = " "; // Generate an id based on timestamp idtag = "div_" + new Date().getTime(); // Generate a new div. // $(divbox).append("<div id=\"" + idtag + "\"></div>"); $('#order_box').append("<div id=\"" + idtag + "\"></div>"); if (divbox == "#property_box") { item_code = $("<input/>", { type: 'hidden', name: "property_item_code[]", value: code, class: "twelve code_input" }); item_title = $("<input/>", { type: 'text', name: "property_item_title[]", value: title, class: "twelve" }); item_price = $("<input/>", { type: 'text', name: "property_item_price[]", value: price, class: "twelve price_input right" }); // Show in the document. $("#" + idtag).append(item_code, code_text, separator, item_title, separator, item_price, separator); $("#" + idtag).append(" <a href='javascript:void;' onclick=\"return remove_it('" + idtag + "');\" class='twelve'>Remove</a>"); } else if (divbox == "#add_on_box") { item_code = $("<input/>", { type: 'hidden', name: "add_on_item_code[]", value: code, class: "twelve code_input" }); item_title = $("<input/>", { type: 'text', name: "add_on_item_title[]", value: title, class: "twelve" }); item_price = $("<input/>", { type: 'text', name: "add_on_item_price[]", value: price, class: "twelve price_input right" }); // Show in the document. $("#" + idtag).append(item_code, code_text, separator, item_title, separator, item_price, separator); $("#" + idtag).append(" <a href='javascript:void;' onclick=\"return remove_it('" + idtag + "');\" class='twelve'>Remove</a>"); } else { room_code = $("<input/>", { type: 'hidden', name: "room_item_code[]", value: code, class: "twelve code_input" }); item_title = $("<input/>", { type: 'text', name: "room_item_title[]", value: title, class: "twelve" }); item_price = $("<input/>", { type: 'text', name: "room_item_price[]", value: price, class: "twelve price_input right" }); // Show in the document. $("#" + idtag).append(room_code, code_text, separator, item_title, separator, item_price, separator); $("#" + idtag).append(" <a href='javascript:void;' onclick=\"return remove_it('" + idtag + "');\" class='twelve'>Remove</a>"); } } Then i view the source of the webpage to see whats actually being produced and i see this.. The name of this item is "I'm Frustrated". <a href='javascript:void;' onclick='add_item("AAwayY","I\'m Frustrated","25.00","#add_on_box")' >I'm Frustrated</a>
-
got it function add_item( code, title, price, divbox ) { var idtag, item_title, item_price, item_code, separator; separator = " "; // Generate an id based on timestamp idtag = "div_" + new Date().getTime(); // Generate a new div. $( divbox ).append( "<div id=\"" + idtag + "\"></div>" ); if ( divbox == "#item_box1" ) { item_title = $("<input/>", { type: 'text', name: "box1_item_title[]", value: title }); item_price = $("<input/>", { type: 'text', name: "box1_item_price[]", value: price }); // Show in the document. $( "#" + idtag ).append( item_title, separator, item_price ); } else { item_code = $("<input/>", { type: 'text', name: "box2_item_code[]", value: code }); item_title = $("<input/>", { type: 'text', name: "box2_item_title[]", value: title }); // Show in the document. $( "#" + idtag ).append( item_code, separator, item_title ); } }
-
I'm trying to write an onclick function that appends a div with input fields in it to an existing div...if that makes sense. I suck at javascript though. I can't even append the div with one input field in it though. anybody any good with javascript? http://jsfiddle.net/XLuHU/1/
-
wait..just kidding. I switched it to "latin1_bin" and that seemed to do the trick. Is that the correct way to go about doing that?