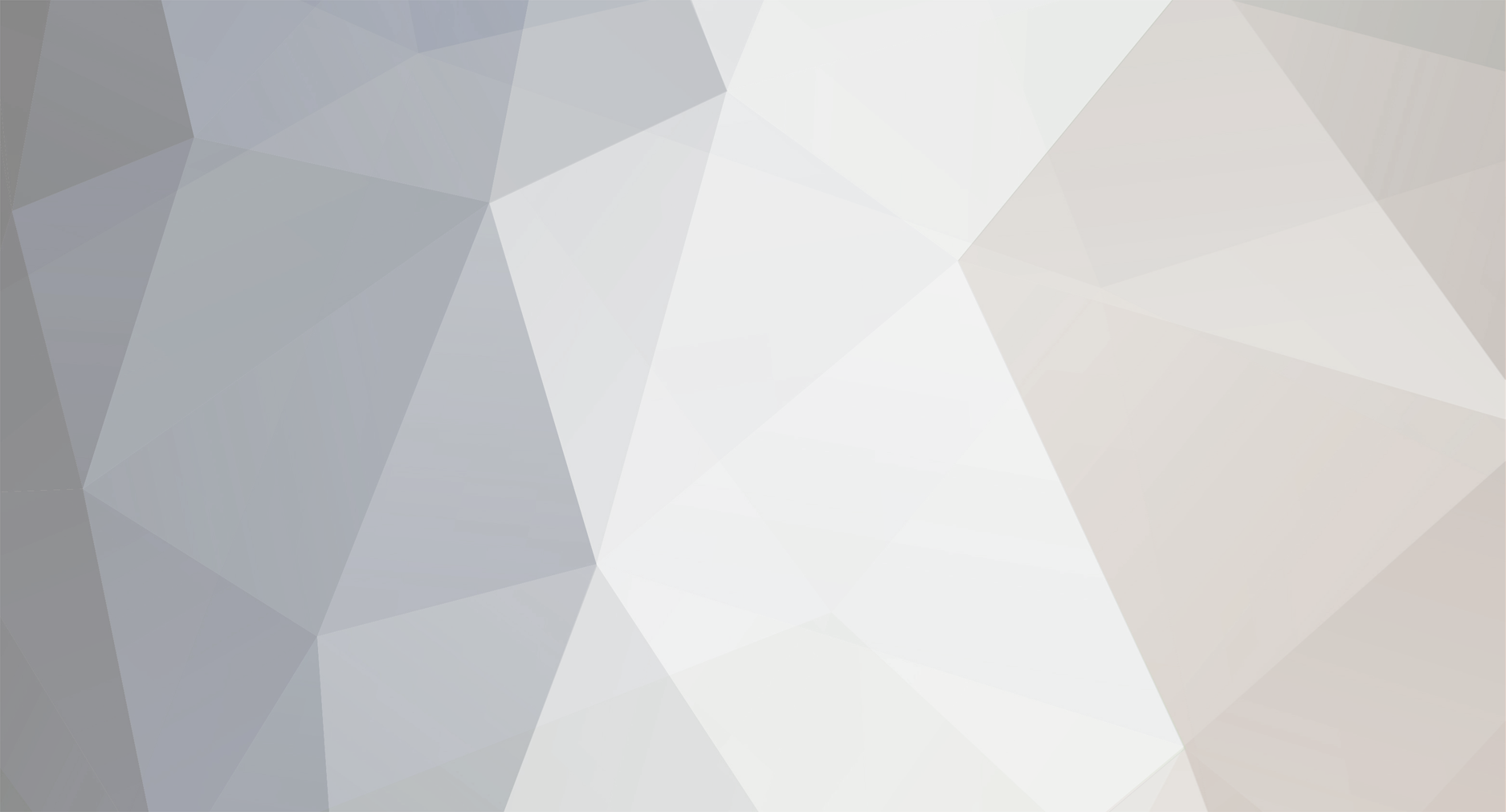
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
Here's the way I do it. Use it in any way you desire. <?php function checkEmails($email_address){ if ($email_address != '') { $domain = end(explode("@", strtolower(trim($email_address)))); $good_emails = array('yahoo.com','aol.com','live.com','hotmail.com','gmail.com'); if (in_array($domain, $good_emails)){ return "$email_address accepted"; return True; } else { return "$email_address not accepted"; return False; } } else { return "Email is empty"; return False; } } //usage echo checkEmails('bobby@yahoo.com')."<br />"; echo checkEmails('mary@mooomail.com')."<br />"; echo checkEmails('mary@live.com')."<br />"; $email1 = ""; echo checkEmails($email1)."<br />"; $email2 = "gsgaarg@spammail.com"; echo checkEmails($email2)."<br />"; if(checkEmails($email1) == True){ echo "do whatever you want here"; } ?> You can even expand onto this and do a rejected full emails array.
-
you have to also set the header to switch mime types as well can't name the file a .png when your header mime type is reading as image/jpeg
-
I realized you said database to database Copy and rename the database folder itself, place new copy into MYSQL\data, then do the repair.
-
There are ways to import the tables into new tables. Here's the easiest method without taking a long time or running into time and memory limits. Method 1: Browse to your MYSQL folder, then data folder, then databasename folder. copy the files associated to that table, rename them and place them back into the database folder example: MySQL\data\databasename It will contain 3 files for each table name, copy and rename them table_name.frm table_name.MYD table_name.MYI to something like below or what you want table_name_copy.frm table_name_copy.MYD table_name_copy.MYI After you rename and place back the files, do a REPAIR on the table and will have an exact clone. Method 2: export your table into a .sql file, create a new table and import the .sql back in, but you have to edit the .sql file to have the proper table name first. Note: if you include the "create table" function in the sql export file, is no need to manually create the new table Method 3: Otherwise you can do it the long way if you have a very large table SELECT * INTO table_name_copy FROM table_name; If I remember correctly this method will not retain your index information.
-
<html><head><title>br4n.com</title></head><frameset rows='100%, *' frameborder=no framespacing=0 border=0><frame src="http://www.br4n.com/" name=mainwindow frameborder=no framespacing=0 marginheight=0 marginwidth=0></frame></frameset><noframes><h2>Your browser does not support frames. We recommend upgrading your browser.</h2><br><br><center>Click <a href="http://www.br4n.com/" >here</a> to enter the site.</center></noframes></html> That's the source of your page. Is no meta data for description and keywords. Search engines can't get any other links your site. Why on earth are you using iframes to link the pages? Just link directly to them. If doing many images, instead of many large images on the same page you should do one or more of the following.... Make a thumbnail image gallery and either popup images enlarged or link to the single image. Cycle the images automatic with javascript one at a time or some sort of navigation previous/next. Use a lazy loader for images, only images in direct view get loaded. Paginate the pages, limiting just a few images to each page.
-
or ltrim <?php $var ="/assets/img/defaultuser.jpg"; $var = ltrim($var, '/'); echo $var; ?> beat me to it
-
For this one... http://get.blogdns.com/logged.php .25 hours equals 15 minutes .5 is 30 minutes .75 is 45 minutes and then everything in between like .99 hours being 59 minutes So basically it equates something like 1.7 hours as 1 hour and 42 minutes The first one.. and at your site sees 1.7 hours as 1.7 hours and doesn't convert the .7 to minutes
-
How to check a string for words in an array
QuickOldCar replied to etrader's topic in PHP Coding Help
There's a few ways to check for items, it's all in how you set it up..what you are looking for..and what kind or results you expect. These are things you have to do unique to your project with making some checks and balances somehow. Sometimes you need to use a mixture to get the exact desired results. You may even have to explode the array and a foreach just to clean it up a bit before checking it. some useful stuff I usually stick to preg_match,preg_match_all,preg_quote,in_array for the most part. http://php.net/manual/en/function.preg-match.php http://www.php.net/manual/en/function.preg-match-all.php http://www.php.net/manual/en/function.preg-quote.php http://php.net/manual/en/function.array-search.php The code that sasa gave is most likely what you need, you need to adjust the pattern for how you exactly want to look for items. -
Well when doing many big numbers using a combination of decimals and whole numbers it gets a little off due to the converting process of each. I did manage to make one more accurate though. Code is below on page. http://get.blogdns.com/logged.php I have another with a different method, then also another in the works using mostly explode. (we'll see how that goes, or if I get frustrated enough... say "that's good enough already") I'm going to convert them all down to seconds, then convert to minutes, then convert to hours. Add the remainder minutes from hours back onto minutes.
-
How to check a string for words in an array
QuickOldCar replied to etrader's topic in PHP Coding Help
Might be better off using the case insensitive version if looking for words. stristr() http://www.php.net/manual/en/function.stristr.php -
Most professionals use 3dmax. http://usa.autodesk.com/3ds-max/. You can get Blender, a free 3d/animation/game engine all in one. Should do what you need. http://www.blender.org/ To make 3d's or animations takes some time to learn.
-
I made a fix for certain conditions of a decimal in the function. <?php $hours = $_POST['hour']; $minutes = $_POST['minute']; if($_POST['hour'] != '' || $_POST['minute'] != '') { function decHrMinSec($number){ if(strpos($number,".") !== false){ $number_explode = explode(".", $number); $pos_zero = $number_explode['0']; $round_pos_one = round($number_explode['1'],2); $pos_one = substr($round_pos_one,0,2); if(strlen($pos_one) == range(1,2) && strlen($pos_one) != '') { $pos_one = $pos_one * 10; } $multiply_sixty = $pos_zero * 60; //multiply_sixty = floor($pos_zero * 60); $total_result = $pos_one + $multiply_sixty; } else { $total_result = $number * 60; } return $total_result; } function convertMinSec($number_value) { $divided = floor($number_value / 60); return $divided; } $sum_minutes = array_sum(array_filter($_POST['hour'])); $finalminutes = decHrMinSec($sum_minutes); $addminutes = array_sum(array_filter($_POST['minute'])) + $finalminutes; $totalhours = convertMinSec($addminutes); $deduct_amount = 60 * $totalhours; $allminutes=$addminutes - $deduct_amount; $totalminutes = round($allminutes); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Flight Log Calculator</title> </head> <body> <form action="" method="post" enctype="multipart/form-data"> Pilots Logbook Calculator<br /> <p>Put your times in the hours and minutes boxes then click the "Add Time" button to get your answer. </p> <table width="401" border="1" cellspacing="5" cellpadding="5"> <tr> <td width="119"><input type="int" name="hour[]" value="<?php echo $hours['0'];?>"size="10" maxlength="10" /> Hrs</td> <td width="241"><input type="int" name="minute[]" value="<?php echo $minutes['0'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]" value="<?php echo $hours['1'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]" value="<?php echo $minutes['1'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]2" value="<?php echo $hours['2'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]2" value="<?php echo $minutes['2'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]3" value="<?php echo $hours['3'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]3" value="<?php echo $minutes['3'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]4" value="<?php echo $hours['4'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]4" value="<?php echo $minutes['4'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]5" value="<?php echo $hours['5'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]5" value="<?php echo $minutes['5'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]6" value="<?php echo $hours['6'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]6" value="<?php echo $minutes['6'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]7" value="<?php echo $hours['7'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]7" value="<?php echo $minutes['7'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]8" value="<?php echo $hours['8'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]8" value="<?php echo $minutes['8'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]9" value="<?php echo $hours['9'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]9" value="<?php echo $minutes['9'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]10" value="<?php echo $hours['10'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]10" value="<?php echo $minutes['10'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]11" value="<?php echo $hours['11'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]11" value="<?php echo $minutes['11'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="submit" name="submit" value="Add Time" /></td> <td><input value="<?php echo $totalhours;?>" type="int" size="10" maxlength="10" /> Hrs <input type="int" value="<?php echo $totalminutes;?>" size="10" maxlength="10" /> Mins</td> </tr> </table> <tr></tr> </form> </body> </html>
-
I revised this even more and made it to do whole or decimal numbers. As for the seconds or decimals for the minutes, I rounded them. I was going to make a selection on how many input fields to do and do it dynamic versus assigning them, but I think already spent too much time on this. Was certainly challenging though, it was fun. You're just seeing the working one, and not all the test versions. I tried it a while and seems pretty good and accurate. Here's a demo http://dynainternet.com/flight-log.php Code: <?php $hours = $_POST['hour']; $minutes = $_POST['minute']; if($_POST['hour'] != '' || $_POST['minute'] != '') { function decHrMinSec($number){ if(strpos($number,".") !== false){ $number_explode = explode(".", $number); $pos_zero = $number_explode['0']; $round_pos_one = round($number_explode['1'],2); $pos_one = substr($round_pos_one,0,2); if(strlen($pos_one) != range(1,2)) { $pos_one = $pos_one * 10; } $multiply_sixty = $pos_zero * 60; //multiply_sixty = floor($pos_zero * 60); $total_result = $pos_one + $multiply_sixty; } else { $total_result = $number * 60; } return $total_result; } function convertMinSec($number_value) { $divided = floor($number_value / 60); return $divided; } $sum_minutes = array_sum($_POST['hour']); $finalminutes = decHrMinSec($sum_minutes); $addminutes = array_sum($_POST['minute']) + $finalminutes; $totalhours = convertMinSec($addminutes); $deduct_amount = 60 * $totalhours; $allminutes=$addminutes - $deduct_amount; $totalminutes = round($allminutes); } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Flight Log Calculator</title> </head> <body> <form action="" method="post" enctype="multipart/form-data"> Pilots Logbook Calculator<br /> <p>Put your times in the hours and minutes boxes then click the "Add Time" button to get your answer. </p> <table width="401" border="1" cellspacing="5" cellpadding="5"> <tr> <td width="119"><input type="int" name="hour[]" value="<?php echo $hours['0'];?>"size="10" maxlength="10" /> Hrs</td> <td width="241"><input type="int" name="minute[]" value="<?php echo $minutes['0'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]" value="<?php echo $hours['1'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]" value="<?php echo $minutes['1'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]2" value="<?php echo $hours['2'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]2" value="<?php echo $minutes['2'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]3" value="<?php echo $hours['3'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]3" value="<?php echo $minutes['3'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]4" value="<?php echo $hours['4'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]4" value="<?php echo $minutes['4'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]5" value="<?php echo $hours['5'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]5" value="<?php echo $minutes['5'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]6" value="<?php echo $hours['6'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]6" value="<?php echo $minutes['6'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]7" value="<?php echo $hours['7'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]7" value="<?php echo $minutes['7'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]8" value="<?php echo $hours['8'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]8" value="<?php echo $minutes['8'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]9" value="<?php echo $hours['9'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]9" value="<?php echo $minutes['9'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]10" value="<?php echo $hours['10'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]10" value="<?php echo $minutes['10'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]11" value="<?php echo $hours['11'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]11" value="<?php echo $minutes['11'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="submit" name="submit" value="Add Time" /></td> <td><input value="<?php echo $totalhours;?>" type="int" size="10" maxlength="10" /> Hrs <input type="int" value="<?php echo $totalminutes;?>" size="10" maxlength="10" /> Mins</td> </tr> </table> <tr></tr> </form> </body> </html>
-
Nothing, and I mean nothing can be output before a header redirect. <?php if($_POST['agree']){ header("Location: http://www.google.com"); } else if ($_POST['disagree']){ header("Location: http://www.yahoo.com"); } ?> <form id="nda" name="nda" method="post" action=""> <input type="submit" name="agree" value="I agree" /> <input type="submit" name="disagree" value="I disagree" /> </form>
-
I redid this to place into your form, also changes the form a little to fix it. <?php $hours = $_POST['hour']; $minutes = $_POST['minute']; function convertMinSec($number_value) { $divided = floor($number_value / 60); return $divided; } $addminutes = $minutes['0'] + $minutes['1']; $hoursfromminutes = convertMinSec($addminutes); $deduct_amount = 60 * $hoursfromminutes; $totalminutes = $addminutes - $deduct_amount; $totalhours = $hours['0'] + $hours['1'] + $hoursfromminutes; ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=UTF-8" /> <title>Untitled Document</title> </head> <body> <form action="" method="post" enctype="multipart/form-data"> <table width="527" border="0" cellspacing="5" cellpadding="5" bgcolor="#c0c5c9"> <p>Time Calculator</p> <p>Put your hours and minutes in the top boxes. Click the add time button<br /> to get the answer </p> <table width="401" border="1" cellspacing="5" cellpadding="5"> <tr> <td width="119"><input type="int" name="hour[]" value="<?php echo $hours['0'];?>"size="10" maxlength="10" /> Hrs</td> <td width="241"><input type="int" name="minute[]" value="<?php echo $minutes['0'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="int" name="hour[]" value="<?php echo $hours['1'];?>" size="10" maxlength="10" /> Hrs</td> <td><input type="int" name="minute[]" value="<?php echo $minutes['1'];?>" size="10" maxlength="10" /> Mins</td> </tr> <tr> <td><input type="submit" name="submit" value="Add Time" /></td> <td><input value="<?php echo $totalhours;?>" type="int" size="10" maxlength="10" /> Hrs <input type="int" value="<?php echo $totalminutes;?>" size="10" maxlength="10" /> Mins</td> </tr> </table> <tr></tr> </form> </body> </html>
-
Here's a simple example There is no checking for errors or numeric numbers this code, which you should do. You can even add seconds to this, expand it to days,months or years even. Add hours and minutes <form action="" method="post" enctype="multipart/form-data"> Hours: <input type="text" name="hour[]" /> Minutes: <input type="text" name="minute[]" /><br /> Hours: <input type="text" name="hour[]" /> Minutes: <input type="text" name="minute[]" /><br /> <input type="submit" value="Add Them" /> </form> <?php $hours = $_POST['hour']; $minutes = $_POST['minute']; function convertMinSec($number_value) { $divided = floor($number_value / 60); return $divided; } $addminutes = $minutes['0'] + $minutes['1']; $hoursfromminutes = convertMinSec($addminutes); $deduct_amount = 60 * $hoursfromminutes; $totalminutes = $addminutes - $deduct_amount; $totalhours = $hours['0'] + $hours['1'] + $hoursfromminutes; echo $hours['0']." hours ".$minutes['0']." minutes<br />"; echo $hours['1']." hours ".$minutes['1']." minutes<br /><hr />"; echo "Total $totalhours hours $totalminutes minutes<br />"; ?>
-
BAN words, number and emails in a text area
QuickOldCar replied to perfexa's topic in PHP Coding Help
I don't know why it's doing that. I can run the code on my server if i eliminate the includes and the first if statement regarding the api. Could it be your copy/paste is converting the quotes? Try using notepad2 http://sourceforge.net/projects/notepad2/ -
It's not unique for every system. Now if a combination of that cpu id, the computer name, and some other hardware comparisons then could be a unique value. As to the answer of how to make this, I'm not sure.
-
Here's that example in a more complex code that does other things too. http://get.blogdns.com/dynaindex/generator.php?character=alphabetnumber&length=2&amount=10000&sort=asc&protocol=&tldext=&hyperlink=no There's a post here at the forums that a few members were posting various ways on how to do this. http://www.phpfreaks.com/forums/index.php?topic=320258.0
-
I noticed I could curl it and redirects, I use a code to handle the javascript redirects. Inserted url: https://auctions.godaddy.com/ Redirected url: http://auctions.godaddy.com/trpHome.aspx Date: 2011-04-12 20:35:01 Title: GoDaddy Domain Auctions, Buy and Sell Domain Names Description: GoDaddy Auctions is the place to go for great domain names that are expiring or have been put up for auction. GoDaddy Auctions makes it easy to get the domain name you have been looking for. Keywords: GoDaddy, GoDaddy Auctions, Domain Auctions, Domain Auction I'll be a nice sport and relinquish my code. Here's my complete curl code for connecting and displays the html as an example. I disabled cookies because I was collecting too many of them. <?php $url = "https://auctions.godaddy.com/"; /*connect to the url using curl to see if exists and get the information*/ //$cookie = tempnam('tmp','cookie'); //$cookie_file_path = "tmp/"; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, $url); //curl_setopt($ch, CURLOPT_COOKIEJAR, $cookie); //curl_setopt($ch, CURLOPT_COOKIEFILE, $cookie_file_path); curl_setopt($ch, CURLOPT_USERAGENT, 'Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.9.2.3) Gecko/20100401 Firefox/3.6.3'); curl_setopt($ch, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_1_1); curl_setopt($ch, CURLOPT_TIMEOUT, 15); curl_setopt($ch, CURLOPT_MAXREDIRS, 15); curl_setopt($ch, CURLOPT_HEADER, 1); curl_setopt ($ch, CURLOPT_RETURNTRANSFER, 1); curl_setopt($ch, CURLOPT_AUTOREFERER, true); curl_setopt ($ch, CURLOPT_FILETIME, 1); curl_setopt ($ch, CURLOPT_FOLLOWLOCATION, 1); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, FALSE); curl_setopt($ch, CURLOPT_ENCODING , ""); $curl_session = curl_init(); //curl_setopt($curl_session, CURLOPT_COOKIEJAR, $cookie); //curl_setopt($curl_session, CURLOPT_COOKIEFILE, $cookie_file_path); curl_setopt($curl_session, CURLOPT_USERAGENT, 'Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.9.2.3) Gecko/20100401 Firefox/3.6.3'); curl_setopt($curl_session, CURLOPT_HTTP_VERSION, CURL_HTTP_VERSION_1_1); curl_setopt($curl_session, CURLOPT_ENCODING , ""); curl_setopt($curl_session, CURLOPT_TIMEOUT, 15); curl_setopt($curl_session, CURLOPT_HEADER, 1); curl_setopt($curl_session, CURLOPT_SSL_VERIFYPEER, FALSE); curl_setopt($curl_session, CURLOPT_HEADER, true); curl_setopt($curl_session, CURLOPT_MAXREDIRS, 15); curl_setopt($curl_session, CURLOPT_RETURNTRANSFER, true); curl_setopt( $curl_session, CURLOPT_AUTOREFERER, true ); curl_setopt ($curl_session, CURLOPT_HTTPGET, true); curl_setopt($curl_session, CURLOPT_URL, $url); $string = mysql_real_escape_string(curl_exec($curl_session)); $html = mysql_real_escape_string(curl_exec ($ch)); $info = curl_getinfo($ch); /*curl response check and to resolve url to the actual location*/ $response = curl_getinfo( $ch ); if ($response['http_code'] == 301 || $response['http_code'] == 302) { ini_set("user_agent", "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.9.2.3) Gecko/20100401 Firefox/3.6.3"); $headers = get_headers($response['url']); $location = ""; foreach( $headers as $value ) { if ( substr( strtolower($value), 0, 9 ) == "location:" ) return get_final_url( trim( substr( $value, 9, strlen($value) ) ) ); } } if ( preg_match("/window\.location\.replace\('(.*)'\)/i", $con_addtent, $value) || preg_match("/window\.location\=[\"'](.*)[\"']/i", $con_addtent, $value) || preg_match("/location\.href\=[\"'](.*)[\"']/i", $con_addtent, $value) ) { $finalurl = get_final_url($value[1]); } else { $finalurl = $response['url']; } $html = curl_exec($ch); $header = "Location: "; echo "$finalurl<br />"; echo $html; ?>
-
<?php for($i=10;$i<=100;$i +=10){ echo $i."<br>"; } ?> result: 10 20 30 40 50 60 70 80 90 100
-
BAN words, number and emails in a text area
QuickOldCar replied to perfexa's topic in PHP Coding Help
Whenever your server works again try this one. I moved the check for bad words up to the top, is no need to execute the script any further than that if they aren't allowed. Also added a header redirect with a message. This was from your original index.php file <?php require ('settings.php'); require('functions.php'); session_start(); $badwords = array('monkey', 'banana', 'justin bieber', 'barbara streisant' ); $pattern = implode("|",$badwords); if(preg_match("/$pattern/i", trim($_POST['facetimeid']))){ header( "refresh:5;url=index.php" ); echo 'Not allowed, You\'ll be redirected in about 5 secs. If not, click <a href="index.php">here</a>.'; EXIT; } else { if ($_GET['do']=='start') { if ($_POST['facetimeid']=='') { header("Location:".SITE_BASE); } else { include('init.php'); } } elseif ($_GET['do']=='random') { // Make sure we have a session, otherwise redirect to homepage if ($_SESSION['CurrentUser']=='') { header("Location:".SITE_BASE); } } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.1//EN" "http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xml:lang="tr-TR"> <head> <title>Facekandi for iPhone</title> <base href="<?php print SITE_BASE; ?>/" /> <meta name="keywords" content="facekandi, livecam, webcam, facelette, facetime chat, iphone 4g, facetime, itouch 4g, face chatroom, video chat, world video chat, "> <meta name="description" content="Facekandi is an iPhone and iPad application designed for randomly pairing you with people all over the world. Try it today to chat, engage and have fun in all kinds of ways. "> <link rel="stylesheet" type="text/css" href="style.css" /> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <meta name="viewport" content="width=device-width" /> <link href="http://facekandi.com/iphone/favicon.ico" rel="icon" type="image/x-icon" /> </head> <body> <img src="facekandi.png" alt="" width="265" height="39" /> <?php if ($_GET['do']=='start') { $ruser = getRandomUser($_POST['facetimeid']); $ruser=$ruser['UserFacetimeID']; ?> <div class="content"> Great! You can now start a Facetime session with <a href="external://facetime://<?php echo $ruser; ?>"><?php echo $ruser; ?></a>! <br/> <form action="index.php?do=random" method="post" /><input type="image" src="next.png" class="usersubmit" /><p> </p> <p><font color="#C0C0C0">Go social facekandi crazy..</font></p><!-- AddThis Button BEGIN --> <div class="addthis_toolbox addthis_default_style addthis_32x32_style"> <a class="addthis_button_preferred_1"></a> <a class="addthis_button_preferred_2"></a> <a class="addthis_button_preferred_3"></a> <a class="addthis_button_preferred_4"></a> <a class="addthis_button_compact"></a> </div> <script type="text/javascript">var addthis_config = {"data_track_clickback":true};</script> <script type="text/javascript" src="http://s7.addthis.com/js/250/addthis_widget.js#username=facelette"></script> <!-- AddThis Button END --> <p> </p> </form> </div> <?php } elseif ($_GET['do']=='random') { $ruser = getRandomUser($_SESSION['CurrentUser']); $ruser=$ruser['UserFacetimeID']; ?> <div class="content"> Here's a random Facekandi user for you: <a href="external://facetime://<?php echo $ruser; ?>"><?php echo $ruser; ?></a> <br/> <form action="index.php?do=random" method="post" /><input type="image" src="next.png" class="usersubmit" /><p> </p> <p><font color="#C0C0C0" size="3">Share Facekandi with everyone..</font></p> <!-- AddThis Button BEGIN --> <div class="addthis_toolbox addthis_default_style addthis_32x32_style"> <a class="addthis_button_preferred_1"></a> <a class="addthis_button_preferred_2"></a> <a class="addthis_button_preferred_3"></a> <a class="addthis_button_preferred_4"></a> <a class="addthis_button_compact"></a> </div> <script type="text/javascript">var addthis_config = {"data_track_clickback":true};</script> <script type="text/javascript" src="http://s7.addthis.com/js/250/addthis_widget.js#pubid=ra-4d8a80540dedf0f0"></script> <!-- AddThis Button END --> <p> </p> <p> </p> <p align="center"></p> <p align="center"> <!--Insert your Adsense code --> <!--Adsense code end--></font></a></p> </form> </div> <?php } else { ?> <form action="index.php?do=start" method="post" > <fieldset style="width: 120; height: 60; font-size: 18px;"> <legend><span class="Facekandi"><font color="#F0F0F0">Enter your Apple Facetime ID</font><font color="#0066FF">*</font></span></legend> <input type="text" name="facetimeid" class="userinput" maxlength="35" size="37" /> </fieldset> <input type="image" src="button.png" class="usersubmit" /> <br /> <br /> <p align="left" class="smallprint"><font color="#0066FF">*</font>Your Facetime ID is either your <b> iPhone mobile number</b> or <b>email address</b> you use for Facetime.<br /> <br/> </p> <p align="left" class="smallprint"><font color="#FF3E3E" size="1">Once you exit the Facekandi application your session will terminate from our server</font><br /> <br /> Remember: be safe and most importantly have fun!</p> <p align="left"></p> </form> <?php } } ?> </div> <!-- insert your Google Analytics here --> <!-- End of Google Analytics code --> </body> </html> -
My first PHP send form ... how about a review please?
QuickOldCar replied to autoworld's topic in PHP Coding Help
We didn't comment on your code, merely expressing to place them onto the site in code tags versus uploading them. Will get more responses if people can see it easily. Looking at it....it's quite a large piece of code with many POST fields. -
My first PHP send form ... how about a review please?
QuickOldCar replied to autoworld's topic in PHP Coding Help
To save some from downloading. wrap code in tags. [code=php:0] [/code] <?php // get posted data into local variables $EmailFrom = Trim(stripslashes($_POST['EmailFrom'])); $EmailTo = "my email address here"; $Subject = "I need a quote"; $Name = Trim(stripslashes($_POST['Name'])); $Tel = Trim(stripslashes($_POST['Tel'])); $Company = Trim(stripslashes($_POST['Company'])); $State = Trim(stripslashes($_POST['State'])); $Website = Trim(stripslashes($_POST['Website'])); $Manufacturer = Trim(stripslashes($_POST['Manufacturer'])); $Retailer = Trim(stripslashes($_POST['Retailer'])); $JobberRestyler = Trim(stripslashes($_POST['JobberRestyler'])); $Distributor = Trim(stripslashes($_POST['Distributor'])); $Motorsports = Trim(stripslashes($_POST['Motorsports'])); $ProfessionalServices = Trim(stripslashes($_POST['ProfessionalServices'])); $Employees = Trim(stripslashes($_POST['Employees'])); $GeneralLiability = Trim(stripslashes($_POST['GeneralLiability'])); $GarageKeepers = Trim(stripslashes($_POST['GarageKeepers'])); $PropertyBuilding = Trim(stripslashes($_POST['PropertyBuilding'])); $PropertyEquipment = Trim(stripslashes($_POST['PropertyEquipment'])); $LossofIncome = Trim(stripslashes($_POST['LossofIncome'])); $WorkersComp = Trim(stripslashes($_POST['WorkersComp'])); $InternetLiability = Trim(stripslashes($_POST['InternetLiability'])); $LifeDisability = Trim(stripslashes($_POST['LifeDisability'])); $ProductLiability = Trim(stripslashes($_POST['ProductLiability'])); $LegalLiability = Trim(stripslashes($_POST['LegalLiability'])); $PropertyContents = Trim(stripslashes($_POST['PropertyContents'])); $CargoMobileProperty = Trim(stripslashes($_POST['CargoMobileProperty'])); $EmployeeDishonesty = Trim(stripslashes($_POST['EmployeeDishonesty'])); $Retirement = Trim(stripslashes($_POST['Retirement'])); $Health = Trim(stripslashes($_POST['Health'])); $Other = Trim(stripslashes($_POST['Other'])); $Comments = Trim(stripslashes($_POST['Comments'])); // validation $validationOK=true; if (Trim($EmailFrom)=="") $validationOK=false; if (Trim($Name)=="") $validationOK=false; if (Trim($Tel)=="") $validationOK=false; if (Trim($State)=="") $validationOK=false; if (!$validationOK) { print "<meta http-equiv=\"refresh\" content=\"0;URL=error.htm\">"; exit; } // prepare email body text $Body = ""; $Body .= "Name: "; $Body .= $Name; $Body .= "\n"; $Body .= "Tel: "; $Body .= $Tel; $Body .= "\n"; $Body .= "Company: "; $Body .= $Company; $Body .= "\n"; $Body .= "State: "; $Body .= $State; $Body .= "\n"; $Body .= "Website: "; $Body .= $Website; $Body .= "\n"; $Body .= "Employees: "; $Body .= $Employees; $Body .= "\n"; $Body .= "\n"; $Body .= "===== Type of Business ====="; $Body .= "\n"; $Body .= "Manufacturer: "; $Body .= $Manufacturer; $Body .= "\n"; $Body .= "Retailer: "; $Body .= $Retailer; $Body .= "\n"; $Body .= "Jobber/Restyler: "; $Body .= $JobberRestyler; $Body .= "\n"; $Body .= "Distributor: "; $Body .= $Distributor; $Body .= "\n"; $Body .= "Motorsports: "; $Body .= $Motorsports; $Body .= "\n"; $Body .= "Professional Services: "; $Body .= $ProfessionalServices; $Body .= "\n"; $Body .= "\n"; $Body .= "===== Insurance Needs ====="; $Body .= "\n"; $Body .= "General Liability: "; $Body .= $GeneralLiability; $Body .= "\n"; $Body .= "Garage/Keepers: "; $Body .= $GarageKeepers; $Body .= "\n"; $Body .= "Property Building: "; $Body .= $PropertyBuilding; $Body .= "\n"; $Body .= "Property Equipment: "; $Body .= $PropertyEquipment; $Body .= "\n"; $Body .= "Loss of Income: "; $Body .= $LossofIncome; $Body .= "\n"; $Body .= "Worker's Comp.: "; $Body .= $WorkersComp; $Body .= "\n"; $Body .= "Internet Liability: "; $Body .= $InternetLiability; $Body .= "\n"; $Body .= "Life & Disability: "; $Body .= $LifeDisability; $Body .= "\n"; $Body .= "Product Liability: "; $Body .= $ProductLiability; $Body .= "\n"; $Body .= "Legal Liability: "; $Body .= $LegalLiability; $Body .= "\n"; $Body .= "Property Contents: "; $Body .= $PropertyContents; $Body .= "\n"; $Body .= "Cargo/Mobile Property: "; $Body .= $CargoMobileProperty; $Body .= "\n"; $Body .= "Employee Dishonesty: "; $Body .= $EmployeeDishonesty; $Body .= "\n"; $Body .= "401K & Retirement: "; $Body .= $Retirement; $Body .= "\n"; $Body .= "Health: "; $Body .= $Health; $Body .= "\n"; $Body .= "Other: "; $Body .= $Other; $Body .= "\n"; $Body .= "\n"; $Body .= "Personal Comments: "; $Body .= $Comments; $Body .= "\n"; $Body .= "\n"; $Body .= "========================="; $Body .= "\n"; // send email $success = mail($EmailTo, $Subject, $Body, "From: <$EmailFrom>"); // redirect to success page if ($success){ print "<meta http-equiv=\"refresh\" content=\"0;URL=ok.htm\">"; } else{ print "<meta http-equiv=\"refresh\" content=\"0;URL=error.htm\">"; } ?> -
BAN words, number and emails in a text area
QuickOldCar replied to perfexa's topic in PHP Coding Help
Does your original index.php file execute and work properly before the check for preg_match? Maybe your server is down? it is a 500 error