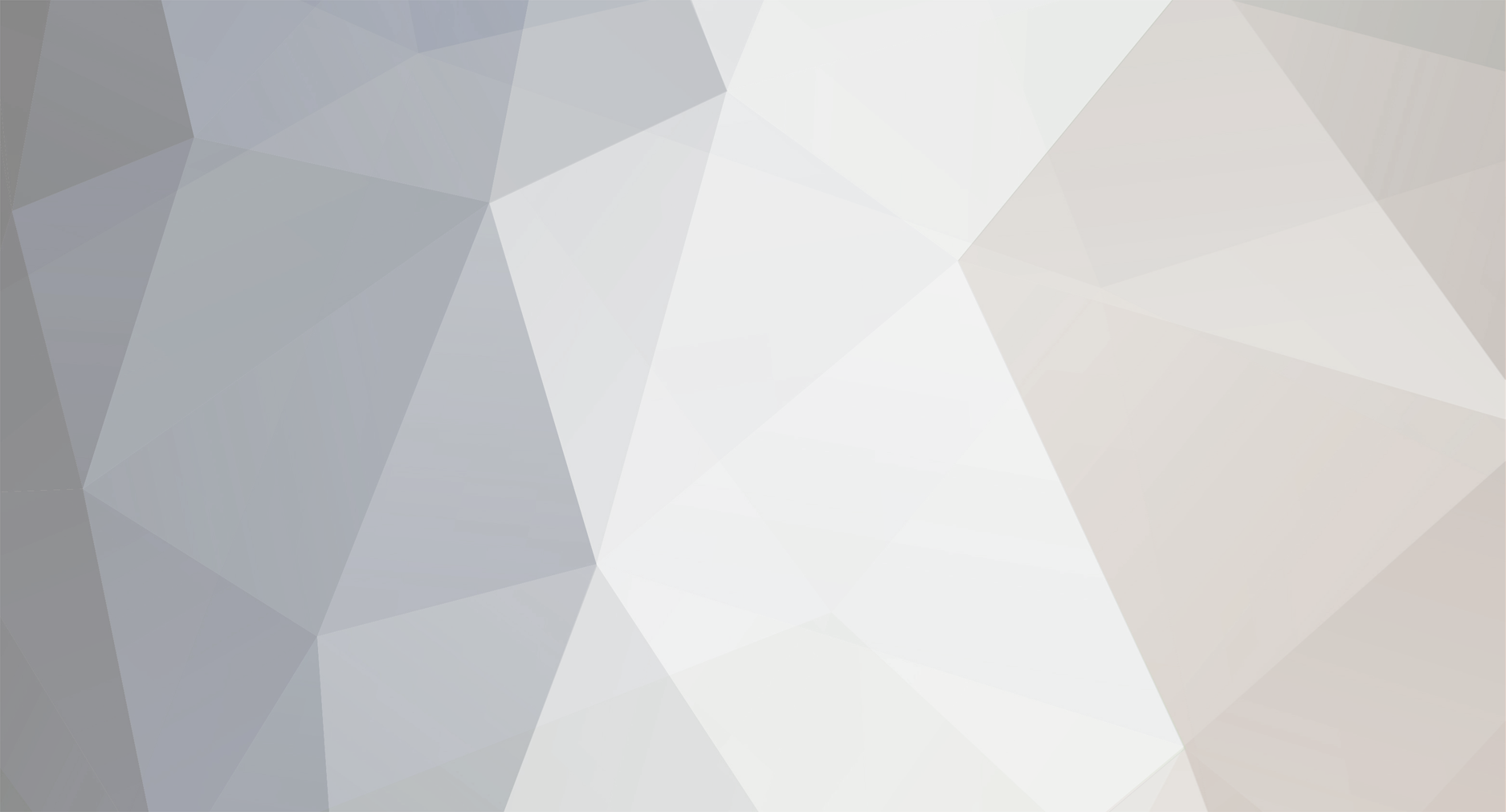
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
How about adding all the curly brackets to your functions and if/else statements. myFunction($string,$functions) { $funcs = explode(",",$functions); foreach ($funcs as $func) { if (function_exists($func)) { $string = $func($string); } else { $string = trim($string); } return $string; } Then carefully look through them to make sure in fact that rules reside within the specified amount of brackets under certain conditions. Place an echo in each of your statements and see what it's actually doing, it seems the else statements are executing as well as the if's under certain conditions of your function.
-
Here's what I do. ID is set to auto-increment in mysql I do a select query to see if the value exists, if value exists I get the current id and make a variable of $check. $sql_check = mysql_query("SELECT * FROM table WHERE value = '".$value."'"); $check = mysql_num_rows($sql_check); $row = mysql_fetch_array($sql_check); $the_ID = $row['ID']; when doing the first check to see if the name is there you now have the row id, now run this if/else query if($check > 0) { mysql_query("UPDATE table SET value_one='$value_one', value_two='$value_two', value_three='$value_three' WHERE ID='$the_ID'"); echo "Updated id $the_ID"; } else { //for inserts don't use id and let mysql auto-increment the id mysql_query("INSERT INTO table (value_one,value_two,value_three) VALUES ('$value_one', '$value_two', '$value_three')"); printf("Inserted id %d\n", mysql_insert_id()); }
-
I'd have to say it may have to do with the way you did your form itself. Could you paste that as well? But to see if your get is passing anything just do like this top your code. $seshid = $_GET['finish']; echo $seshid; Then we can go from there to see what the problem might be.
-
You can follow this tutorial here. http://www.phpfreaks.com/tutorial/simple-sql-search
-
What do you expect to look into or reference a search term without some sort of database?
-
Search MySQL with several words in once?
QuickOldCar replied to magnusalex's topic in PHP Coding Help
OK, you can look here a bit. http://dev.mysql.com/doc/refman/5.5/en/fulltext-boolean.html So keep your code as it is, but get rid the % in your query. Again.....the one that dreamwest posted. $sql_res = mysql_query("SELECT * FROM test_user_data WHERE MATCH streng AGAINST ('$q' IN BOOLEAN MODE) ORDER BY streng LIMIT 5" ); If you do the above query....any word they insert you then added the + to the front of the word by using $q2 .= "+{$word}* ";..., so that means in any order it should find the same results no matter which order of the words they use. All these would return the same exact results Duracell Procell AAA Procell Duracell AAA AAA Procell Duracell AAA Duracell Procell as for AAA not there, most likely has to do with mysql minimum word length default of 4 minimum characters. http://dev.mysql.com/doc/refman/5.5/en/server-system-variables.html#sysvar_ft_min_word_len -
That's the way I do it and prefer.
-
For what it's worth I redid this into selects that get the cities names from the same data.txt file. Same link for demo http://get.blogdns.com/dynaindex/citysize/ <?php //data.txt contains all city information. On each line is similar to new york,large or smallville,medium $city = mysql_real_escape_string($_GET['city']); $city = trim($city); $my_file = "data.txt"; if (file_exists($my_file)) { $data = file($my_file); ?> <form name="input" action="" method="get"> <select name="city"> <?php foreach($data as $lines){ $info = explode("|",$lines); $cities = ucwords(strtolower($info[0])); echo "<option value='$cities'>$cities</option>"; } ?> </select> <input type="submit" value="Get Size" /> </form> <?php if (!isset($_GET['city']) || $city == ""){ echo "Insert a city name"; EXIT; } function get_size($string) { $pos = strrpos($string, '|'); if($pos===false) { return false; } else { return substr($string, $pos+1); } } foreach ($data as $line) { if (preg_match("/$city/i", $line)) { $check = "Yes"; } } if ($check != "Yes"){ echo "$city not found"; } else { $line = trim($line); $line = get_size($line); echo "$city is $line size.<br />"; } } else { echo "No file to display"; } ?>
-
oops, pasted that wrong somehow <?php //data.txt contains all city information. On each line is new york,large $city = mysql_real_escape_string($_GET['city']); $city = trim(strtolower($city)); ?> <form name="input" action="" method="get"> City <input type="text" name="city" value="<?php echo $city;?>" /> <input type="submit" value="See City Size" /> </form> <?php if (!isset($_GET['city']) || $city == ""){ echo "Insert a city name"; EXIT; } function get_size($string) { $pos = strrpos($string, '|'); if($pos===false) { return false; } else { return substr($string, $pos+1); } } $my_file = "data.txt"; if (file_exists($my_file)) { $data = file($my_file); $check = "No"; foreach ($data as $line) { if (preg_match("/$city/i", $line)) { $check = "Yes"; } } if ($check != "Yes"){ echo "$city not found"; } else { $line = trim($line); $line = get_size($line); echo "$city is $line size.<br />"; } } else { echo "No file to display"; } ?>
-
I wrote up a code specific for you. It uses a text file for the array using a pipe to separate the city to size demo here if would like to try it http://get.blogdns.com/dynaindex/citysize/?city=altoona <?php //data.txt contains all city information. On each line is new york,large $city = mysql_real_escape_string($_GET['city']); $city = trim(strtolower($city)); ?> <form name="input" action="" method="get"> City <input type="text" name="city" value="<?php echo $city;?>" /> <input type="submit" value="See City Size" /> </form> <?php <?php //data.txt contains all city information. On each line is new york,large $city = mysql_real_escape_string($_GET['city']); $city = trim(ucfirst(strtolower($city))); ?> <form name="input" action="" method="get"> City <input type="text" name="city" value="<?php echo $city;?>" /> <input type="submit" value="See City Size" /> </form> <?php if (!isset($_GET['city']) || $city == ""){ echo "Insert a city name"; EXIT; } function get_size($string) { $pos = strrpos($string, '|'); if($pos===false) { return false; } else { return substr($string, $pos+1); } } $my_file = "data.txt"; if (file_exists($my_file)) { $data = file($my_file); $check = "No"; foreach ($data as $line) { if (preg_match("/$city/i", $line)) { $check = "Yes"; } } if ($check != "Yes"){ echo "$city not found"; } else { $line = trim($line); $line = get_size($line); echo "$city is $line size.<br />"; } } else { echo "No file to display"; } ?> data.txt example used (of course is not all cities or accurate data) Abbeville|small Adamsville|large Addison|medium Akron|large Alabaster|small Albertville|small Alexander City|small Aliceville|small Allgood|medium Altoona|large Anderson|medium
-
Search MySQL with several words in once?
QuickOldCar replied to magnusalex's topic in PHP Coding Help
Here's an example of the "at least one word" search query I use which also can do the +,- and the or if no + or - is used between the words. If you don't explode the search word by + and just use the POST value as is, you can then use the add/include/or with +,-,space Typical searches would be +battery +procell +battery +procell -aaa battery +a +c -d battery procell $q= mysql_real_escape_string($_POST['searchword']); $q = trim($q); $sql_res = mysql_query("SELECT * FROM test_user_data WHERE MATCH streng AGAINST ('$q' IN BOOLEAN MODE) ORDER BY streng LIMIT 5" ); Dreamwest posted the same query. -
http://www.kitco.com/market/ http://www.kitcometals.com/ http://www.kitcosilver.com/charts.html
-
All laptops get too hot and usually puke sooner or later, is inevitable and are pretty much making them a disposable item versus repairing them. It could be a number of issues, but none of the solutions to fix it are cost effective, unless you take it apart, clean it and suddenly works. Otherwise could be: Any cooling fans stopped working. Motherboard capacitors leaking or swelled. Bad video chipset on board. Weak power supply. Broken cdrom or dvdrom (yes I've seen it a few times, I assume it shorts and draws too much power) Harddrive failure as in shorting. Cpu failure. It's not very often for just a black screen, but could even be faulty memory or the memory slot/controller itself. Since it's a laptop and the screen is on a hinge...the wires could have also broken leading to the screen. Those would be the most common issues.
-
You could do the switch statement or even an if/else. I think the best way would be to make 3 arrays of small, medium, large, ..with your appropriate cities in each array. Then do a check if in_array is ........ <?php $small = array("city1", "city2", "city3", "city4"); $medium = array("city1", "city2", "city3", "city4"); $large = array("city1", "city2", "city3", "city4"); if (in_array($the_city, $small)) { echo "$the_city is small"; } elseif (in_array($the_city, $medium)) { echo "$the_city is medium"; } elseif (in_array($the_city, $large)) { echo "$the_city is large"; } else { echo "$the_city size is unknown"; } ?> Something like that should work with whatever results you may want to do versus the echo.
-
Search MySQL with several words in once?
QuickOldCar replied to magnusalex's topic in PHP Coding Help
You have that backwards, innodb does not support full text search. As for the change of database structure, you make an index on anywhere in the query is a where or and -
The error was on the front page in the footer area at Top jobs to work at near you:. I haven't seen the error the last few times I looked at your site.
-
I added a few more things to this. Did a check on file extension first, if matches then continues with the rest. A check to see if is no redirection and that curl responds to the same link as was inserted. Lowercase the protocol and parsed domain section, for checking to see if they are the same. <form name="input" action="" method="get"> Check if image url exists: <input type="text" name="url" style="width:480px" /> <input type="submit" value="Go" /> </form> <?php $url = mysql_real_escape_string($_GET['url']); if (isset($_GET['url']) OR $url != ""){ function checkRemote($check_url) { function parsedHost($new_parse_url) { $parsedUrl = parse_url(trim($new_parse_url)); return trim($parsedUrl[host] ? $parsedUrl[host] : array_shift(explode('/', $parsedUrl[path], 2))); } function trimProtocol($the_url) { $the_url = trim($the_url); $lowered_url = substr_replace(strtolower(parsedHost($the_url)),$the_url,0); $trimmed_url = ltrim($lowered_url, array('http://','http://www.','https://','https://www.','www.')); return $trimmed_url; } $check_url = trim($check_url); $allowedExtensions = array("gif","png","bmp", "jpg","jpeg","ico"); if (in_array(end(explode(".", strtolower($check_url))), $allowedExtensions)) { //echo"allowed type -"; $ch = curl_init(); curl_setopt($ch, CURLOPT_URL,$check_url); curl_setopt($ch, CURLOPT_NOBODY, 1); curl_setopt($ch, CURLOPT_FAILONERROR, 1); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); $lastUrl = curl_getinfo($ch, CURLINFO_EFFECTIVE_URL); /* echo trimProtocol($check_url) ." compares ". trimProtocol($lastUrl); if (trimProtocol($check_url) != trimProtocol($lastUrl)) { echo "$check_url Different location than $lastUrl"; } */ if(curl_exec($ch)!==FALSE && $check_url == $lastUrl) { echo"<h3>Image Exists</h3>"; return true; } else { echo"image does not exist -"; return false; } } else { echo" not allowed type -"; return false; } } //usage $check_result = checkRemote($url); if ($check_result == "true") { if (substr($url, 0, 4) != "http") { $url = "http://$url"; } echo "<a href='$url'><img src='$url' border='0'></a>"; } else { echo "$url doesn't exist or not an accepted image type."; } } else { echo"<h3>Please insert an image location.</h3>"; } echo '<br />'; ?> I do see some flaws with this method, may be better to get the image info actually. queries after the image, possible to eliminate the query after the extension http://mm04.nasaimages.org/MediaManager/srvr?mediafile=/Size1/nasaNAS-12-NA/66989/sig07-009_mac.jpg&userid=1&username=admin&resolution=1&servertype=JVA&cid=12&iid=nasaNAS&vcid=NA&usergroup=spitzer_-_nasa-12-Admin&profileid=56 but images displayed through a script would be impossible to determine without seeing the actual image info http://get.blogdns.com/url-thumb.php?size=400&text=Dynaindex.com&textsize=12&textcolor=aqua&url=http://kindergoth.com/ I came across this that gets the image size without using getimagesize() http://mtekk.us/archives/guides/check-image-dimensions-without-getimagesize/ But it has to do it in different ways per image type, that still wouldn't help if was a script. It seems there is no extremely fast 100% correct way to do this.
-
Just had a thought to be even faster. I would probably do a check to see if the image extension is acceptable first ..then do a curl check. No sense checking it with curl if isn't an image and also the right extension in the first place.
-
Ha, I couldn't stand it, I was compelled to make this into a single function. <form name="input" action="" method="get"> Check Files Remote Url: <input type="text" name="url" /> <input type="submit" value="Go" /> </form> <?php $url = mysql_real_escape_string($_GET['url']); function checkRemote($check_url) { $ch = curl_init(); curl_setopt($ch, CURLOPT_URL,$check_url); curl_setopt($ch, CURLOPT_NOBODY, 1); curl_setopt($ch, CURLOPT_FAILONERROR, 1); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); if(curl_exec($ch)!==FALSE) { $allowedExtensions = array("gif","png","bmp", "jpg","jpeg","ico"); if (in_array(end(explode(".", strtolower($check_url))), $allowedExtensions)) { return true; } else { return false; } } else { return false; } } //usage $check_result = checkRemote($url); if ($check_result == "true") { echo "$url good image."; } else { echo "$url doesn't exist or not an accepted image type."; } ?>
-
I added the image check, I'm sure can shift things around or even make it all one function. <form name="input" action="" method="get"> Check Files Remote Url: <input type="text" name="url" /> <input type="submit" value="Go" /> </form> <?php $url = mysql_real_escape_string($_GET['url']); function checkRemote($check_url) { $ch = curl_init(); curl_setopt($ch, CURLOPT_URL,$check_url); curl_setopt($ch, CURLOPT_NOBODY, 1); curl_setopt($ch, CURLOPT_FAILONERROR, 1); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); if(curl_exec($ch)!==FALSE) { return true; } else { return false; } } $check_result = checkRemote($url); if ($check_result == "true") { echo "$url exists.<br />"; $allowedExtensions = array("gif","png","bmp","jpg","jpeg","gif","ico"); if (!in_array(end(explode(".", strtolower($url))), $allowedExtensions)) { echo "not allowed image type"; } else { echo "$url allowed and good image type.<br />"; $check_ok = true; } } else { echo "$url doesn't exist.<br />"; } if ($check_ok){ echo "Do something with this good image at $url"; } ?>
-
Could this help at all? <form name="input" action="" method="get"> Check Files Remote Url: <input type="text" name="url" /> <input type="submit" value="Go" /> </form> <?php $url = mysql_real_escape_string($_GET['url']); function checkRemote($check_url) { $ch = curl_init(); curl_setopt($ch, CURLOPT_URL,$check_url); curl_setopt($ch, CURLOPT_NOBODY, 1); curl_setopt($ch, CURLOPT_FAILONERROR, 1); curl_setopt($ch, CURLOPT_RETURNTRANSFER, 1); if(curl_exec($ch)!==FALSE) { return true; } else { return false; } } $check_result = checkRemote($url); if ($check_result == "true") { echo "$url exists."; } else { echo "$url doesn't exist."; } ?> Now that you know if actually exists or not, you can then just check the end of the url to see if it's an acceptable image format as .png .jpg .jpeg .gif .bmp or so on.
-
I also made a bad words filter to do single words or entire posts. The demo is there, able to download, can also see the code below the demo. http://get.blogdns.com/dynaindex/Safe-Filter/ One day I'll have to make this a function, but was never at the top of my list.
-
Well here's a breakdown of how I approached this. I have a search/navigation form using get. I do multiple gets in the same form with a combination of text fields and dropdowns with all my values, that also includes a page number text input. On my display page i first discover the url and if there are any queries, if no queries i display a default basic query going by last id's and show 10...for speed purposes. For display page if no page number is set it defaults to page=1 With a few checks for isset or empty I set default values for the other GET requests For the pagination I do similar to this, I actually use similar to the second for my dynaindex.com http://dynainternet.com/paginate/ This one can do any amount of posts per page http://dynaindex.com/paginate.php This one I hard set it to 10 posts per page but works differently Now that I have GET values for everything I do checks with a multi if else statement that contains different mysql queries, each of those queries are variables for what type of search it is and what to look in mysql for. I also use full text indexing and boolean mode, a few likes and even use match against. As per http://dev.mysql.com/doc/refman/5.5/en/fulltext-boolean.html In implementing this feature, MySQL uses what is sometimes referred to as implied Boolean logic, in which * + stands for AND * - stands for NOT * [no operator] implies OR With that said here would be an example of the multi query I actually use a multi post status for logged in or admin, we'll just set this to publish $post_status = "WHERE post_status='publish'"; $search I use as type of search doing $search_words is the search terms or words $display = "post_date"; as default, but I have get values of name,id and so on $order is for ASC or DESC look in my pagination scripts $startrow is the mysql starting row $posts_per_page is amount of posts per page //search get variables from search-navigation if ($search == "Date") { // $result = mysql_query("SELECT * FROM posts $post_status AND post_date LIKE //'".$search_words."%' ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); //$total_count = mysql_query("SELECT * FROM posts $post_status AND post_date LIKE //'".$search_words."%'"); $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_date) AGAINST ('\"$search_words\"' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_date) AGAINST ('\"$search_words\"' IN BOOLEAN MODE)"); } elseif ($search == "ID") { $result = mysql_query("SELECT * FROM posts $post_status AND ID LIKE '".$search_words."%' ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND ID LIKE '".$search_words."%'"); } elseif ($search == "url_begins_characters") { $result = mysql_query("SELECT * FROM posts $post_status AND post_title LIKE '".$search_words."%' ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND post_title LIKE '".$search_words."%'"); } elseif ($search == "url_contains_characters") { $result = mysql_query("SELECT * FROM posts $post_status AND post_title LIKE '%"."$search_words"."%' ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND post_title LIKE '%"."$search_words"."%'"); } elseif ($search == "feed_single_word") { $result = mysql_query("SELECT * FROM posts $post_status AND link_rss LIKE '%"."$search_words"."%' ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND link_rss LIKE '%"."$search_words"."%'"); } elseif ($search == "one_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_title,post_content) AGAINST ('$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_title,post_content) AGAINST ('$search_words' IN BOOLEAN MODE)"); } elseif ($search == "exact_words") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_title,post_content) AGAINST ('\"$search_words\"' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_title,post_content) AGAINST ('\"$search_words\"' IN BOOLEAN MODE)"); } elseif ($search == "least_one_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_title,post_content) AGAINST ('$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_title,post_content) AGAINST ('$search_words' IN BOOLEAN MODE)"); } elseif ($search == "exclude_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_title,post_content) AGAINST ('+$search_words -$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_title,post_content) AGAINST ('+$search_words -$search_words' IN BOOLEAN MODE)"); } elseif ($search == "title_one_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (title_2) AGAINST ('$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (title_2) AGAINST ('$search_words' IN BOOLEAN MODE)"); } elseif ($search == "title_exact_words") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (title_2) AGAINST ('\"$search_words\"' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (title_2) AGAINST ('\"$search_words\"' IN BOOLEAN MODE)"); } elseif ($search == "title_least_one_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (title_2) AGAINST ('$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (title_2) AGAINST ('$search_words' IN BOOLEAN MODE)"); } elseif ($search == "title_exclude_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (title_2) AGAINST ('+$search_words -$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (title_2) AGAINST ('+$search_words -$search_words' IN BOOLEAN MODE)"); } elseif ($search == "description_one_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_description) AGAINST ('$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_description) AGAINST ('$search_words' IN BOOLEAN MODE)"); } elseif ($search == "description_exact_words") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_description) AGAINST ('\"$search_words\"' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_description) AGAINST ('\"$search_words\"' IN BOOLEAN MODE)"); } elseif ($search == "description_least_one_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_description) AGAINST ('$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_description) AGAINST ('$search_words' IN BOOLEAN MODE)"); } elseif ($search == "description_exclude_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_description) AGAINST ('+$search_words -$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_description) AGAINST ('+$search_words -$search_words' IN BOOLEAN MODE)"); } elseif ($search == "keyword_one_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_keywords) AGAINST ('$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_keywords) AGAINST ('$search_words' IN BOOLEAN MODE)"); } elseif ($search == "keyword_exact_words") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_keywords) AGAINST ('\"$search_words\"' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_keywords) AGAINST ('\"$search_words\"' IN BOOLEAN MODE)"); } elseif ($search == "keyword_least_one_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_keywords) AGAINST ('$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_keywords) AGAINST ('$search_words' IN BOOLEAN MODE)"); } elseif ($search == "keyword_exclude_word") { $result = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_keywords) AGAINST ('+$search_words -$search_words' IN BOOLEAN MODE) ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND MATCH (post_keywords) AGAINST ('+$search_words -$search_words' IN BOOLEAN MODE)"); } else { //if anything goes wrong above or nothing selected, this will be used as the default query instead /* all posts //$result = mysql_query("SELECT * FROM posts $post_status ORDER BY $display $order //LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status"); */ //just show last 10 by id main page if ($url == "http://get.blogdns.com/dynaindex/index.php") { $result = mysql_query("SELECT * FROM posts ORDER BY ID DESC LIMIT 0,10"); $total_count = $result; } else { //todays results new and updated $result = mysql_query("SELECT * FROM posts $post_status AND post_date LIKE '".$today_date."%' ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM posts $post_status AND post_date LIKE '".$today_date."%'"); } I believe my method is a bit different than most pagination you will see, most define a page number and do a plus or minus 1 by using hyperlinks, I go by the total count and the current page and insert it into a query. The results of the total count determine how many there are, then through calculations determines how many pages there are. Then clicking or inserting the page number would then insert the correct startrow and posts per page. I created full text indexes in mysql on anything that would use a where or and clause. You must be using MyISAM to use full text indexing, also is a min word length and stop words which you may change. change your my.ini like this if want it 3, can use 1 if really wanted to, add any excluded words into your stop.txt: [mysqld] ft_min_word_len=3 ft_stopword_file="C:\\MySQL\\stop.txt" restart your mysqld at services.msc reindex your table using REPAIR TABLE tbl_name QUICK; If using Innodb is entirely different, I would then recommend trying sphinxsearch.com or lucene.apache.org lemme tell you, I use myisam and full text, but if were to do it again I'd opt for innodb, I really hate how it locks the tables every insert or update. I put up with it for now but one day I think I'm gonna switch over to cassandra.apache.org as the database and use python as the search method.
-
I would be able to help with this, not an easy task by any means. You need multiple items to be done to accomplish your goal, like an entire script, indexes in the database, custom queries and forms. Plus pagination for it all. I ended up doing what you are looking for, took me a long time and lots of trial and error. You can browse my profile posts for some suggestions and ideas, or can try my dynaindex.com out while being logged in and see how the search/navigation actually works. You can post your current code here just to see what you are currently doing.
-
if remote url string is a file or directory?
QuickOldCar replied to XpertWorlock's topic in PHP Coding Help
Run the url through this function http://php.net/manual/en/function.filetype.php