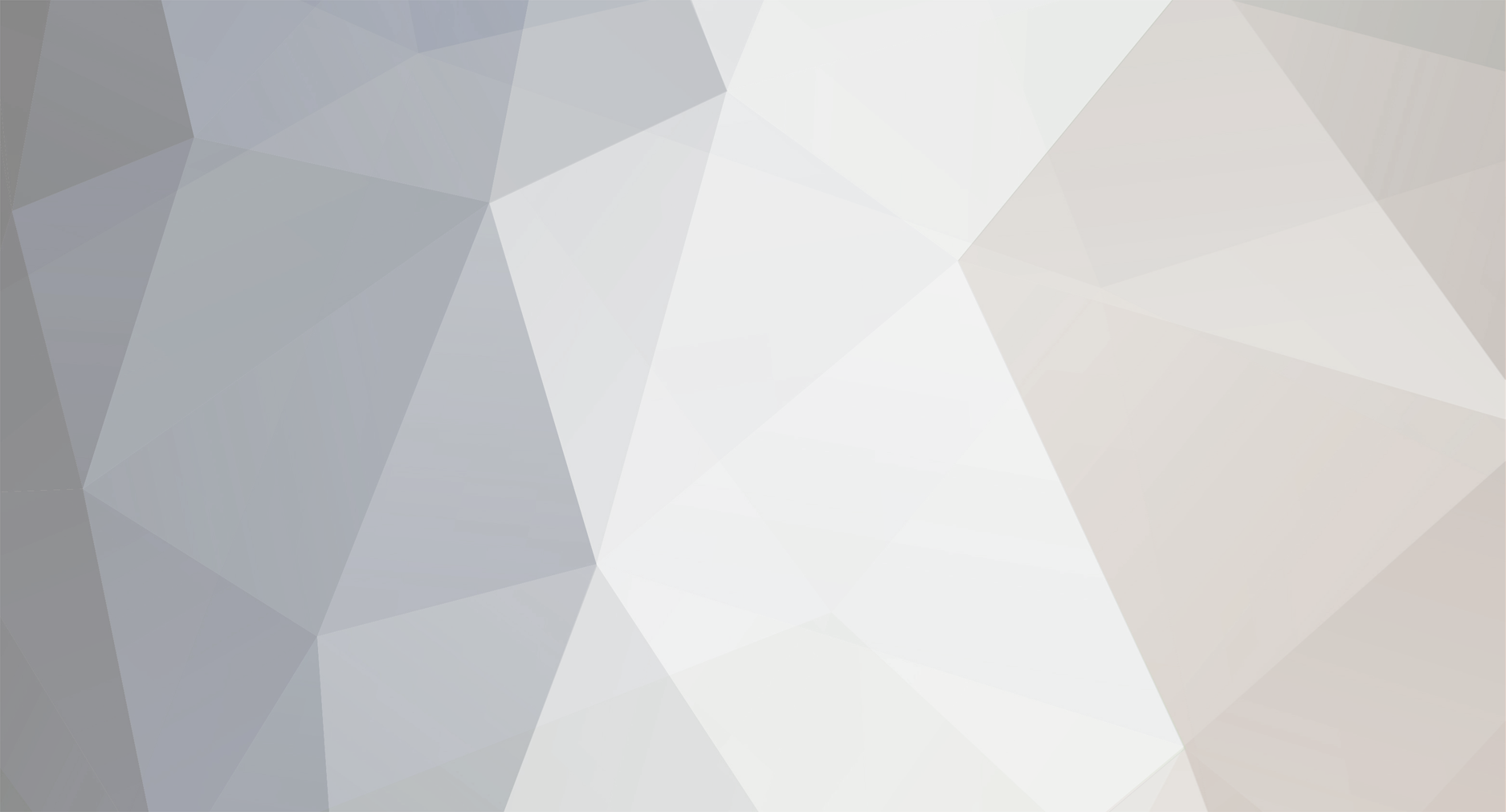
QuickOldCar
Staff Alumni-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Everything posted by QuickOldCar
-
Pagination on search database do not work help !!!!
QuickOldCar replied to jhondoe144's topic in PHP Coding Help
I fail to see where the mysql select is limiting the results. I'm in a real big hurry here and on way out the door, look how I made it get values for start and stop for the limit of mysql select, in mysql the first value startrow is actually zero not 1, so I made a rule for that, this needs more work with the hyperlinks and navigation, I don't have the time right now. <?php // Get the search variable from URL $var = @$_GET['q'] ; $trimmed = trim($var); //trim whitespace from the stored variable // rows to return $limit=10; $calc_startrow = $limit-$limit; if ($calc_startrow < 0){ $calc_startrow = 0; } $startrow =$calc_startrow; $stoprow = $startrow+$limit; // check for an empty string and display a message. if ($trimmed == "") { echo "<p>Please enter a search...</p>"; exit; } // check for a search parameter if (!isset($var)) { echo "<p>We dont seem to have a search parameter!</p>"; exit; } //connect to your database ** EDIT REQUIRED HERE ** mysql_connect("localhost","username","password"); //(host, username, password) //specify database ** EDIT REQUIRED HERE ** mysql_select_db("database") or die("Unable to select database"); //select which database we're using // Build SQL Query $query = "select * from the_table where 1st_field like \"%$trimmed%\" order by 1st_field LIMIT $startrow,$stoprow"; // EDIT HERE and specify your table and field names for the SQL query $numresults=mysql_query($query); $numrows=mysql_num_rows($numresults); // If we have no results, offer a google search as an alternative if ($numrows == 0) { echo "<h4>Results</h4>"; echo "<p>Sorry, your search: "" . $trimmed . "" returned zero results</p>"; // google echo "<p><a href=\"http://www.google.com/search?q=" . $trimmed . "\" target=\"_blank\" title=\"Look up " . $trimmed . " on Google\">Click here</a> to try the search on google</p>"; } // next determine if s has been passed to script, if not use 0 if (empty($s)) { $s=0; } // get results $query .= " limit $s,$limit"; $result = mysql_query($query) or die("Couldn't execute query"); // display what the person searched for echo "<p>You searched for: "" . $var . ""</p>"; // begin to show results set echo "Results"; $count = 1 + $s ; // now you can display the results returned while ($row= mysql_fetch_array($result)) { $title = $row["1st_field"]; echo "$count.) $title" ; $count++ ; } $currPage = (($s/$limit) + 1); //break before paging echo "<br />"; // next we need to do the links to other results if ($s>=1) { // bypass PREV link if s is 0 $prevs=($s-$limit); print " <a href=\"$PHP_SELF?s=$prevs&q=$var\"><< Prev 10</a>  "; } // calculate number of pages needing links $pages=intval($numrows/$limit); // $pages now contains int of pages needed unless there is a remainder from division if ($numrows%$limit) { // has remainder so add one page $pages++; } // check to see if last page if (!((($s+$limit)/$limit)==$pages) && $pages!=1) { // not last page so give NEXT link $news=$s+$limit; echo " <a href=\"$PHP_SELF?s=$news&q=$var\">Next 10 >></a>"; } $a = $s + ($limit) ; if ($a > $numrows) { $a = $numrows ; } $b = $s + 1 ; echo "<p>Showing results $b to $a of $numrows</p>"; ?> -
Need Help with a very simple Employee Time Clock
QuickOldCar replied to madjack87's topic in PHP Coding Help
Ever consider using http://timeclock.sourceforge.net/index.php The hard work is already done for you. -
By default mysql is supposed to be case insensitive, not sure why yours would not be. I'm using MySQL 5.0.51b I use a multiple select query like below and for all it finds everything. if ($search == "user_begins_characters") { $result = mysql_query("SELECT * FROM users WHERE username LIKE '".$search_words."%' ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM users WHERE username LIKE '".$search_words."%'"); } elseif ($search == "user_contains_characters") { $result = mysql_query("SELECT * FROM users WHERE username LIKE '%"."$search_words"."%' ORDER BY $display $order LIMIT $startrow,$posts_per_page" ); $total_count = mysql_query("SELECT * FROM users WHERE username LIKE '%"."$search_words"."%'");
-
I didn't notice you said within a circle also, so here is for that. <?php $text = "QuickOldCar"; $image = imagecreatetruecolor(400,400); $white = imagecolorallocate($image,255,255,255); imagefill($image,0,0,$white); $red = imagecolorallocate($image,255,0,0); $degrees = (180/strlen($text)); $black = imagecolorallocate($image, 0, 0, 0); imagearc($image, 200, 200, 390, 390, 0, 360, $black); for ($i=0;$i<strlen($text);$i++) { $a = ($degrees*$i)+95; $cos = cos(deg2rad($a)); $sin = sin(deg2rad($a)); $x = 0; $y = 180; $xt = round($cos*($x) - $sin*($y)); $yt = round($sin*($x) + $cos*($y)); imagettftext($image,12,180-($a),200+$xt,200+$yt,$red,"font.TTF",$text[$i]); } header("Content-type: image/jpeg"); imagejpeg($image,"",80); imagedestroy($image); ?>
-
If you want just a name with arc on top change the $degrees and then the $a values for length of your text to look right. <?php $text = "QuickOldCar"; $image = imagecreatetruecolor(400,400); $white = imagecolorallocate($image,255,255,255); imagefill($image,0,0,$white); $red = imagecolorallocate($image,255,0,0); $degrees = (180/strlen($text)); for ($i=0;$i<strlen($text);$i++) { $a = ($degrees*$i)+95; $cos = cos(deg2rad($a)); $sin = sin(deg2rad($a)); $x = 0; $y = 180; $xt = round($cos*($x) - $sin*($y)); $yt = round($sin*($x) + $cos*($y)); imagettftext($image,20,180-($a),200+$xt,200+$yt,$red,"font.TTF",$text[$i]); } header("Content-type: image/jpeg"); imagejpeg($image,"",100); imagedestroy($image); ?>
-
Those codes don't work, try them. But the one below does, replace the font with your own font and location. <?php $text = "- Phpfreaks - QuickOldCar"; $image = imagecreatetruecolor(400,400); $white = imagecolorallocate($image,255,255,255); imagefill($image,0,0,$white); $red = imagecolorallocate($image,255,0,0); $degrees = (360/strlen($text)); for ($i=0;$i<strlen($text);$i++) { $a = ($degrees*$i)+180; $cos = cos(deg2rad($a)); $sin = sin(deg2rad($a)); $x = 0; $y = 180; $xt = round($cos*($x) - $sin*($y)); $yt = round($sin*($x) + $cos*($y)); imagettftext($image,20,180-($a),200+$xt,200+$yt,$red,"font.TTF",$text[$i]); } header("Content-type: image/jpeg"); imagejpeg($image,"",100); imagedestroy($image); ?>
-
upload a few files and then have them rename with rand()?
QuickOldCar replied to SnakZ's topic in PHP Coding Help
Without me writing your entire code for you, I'll tell some hints that maybe will help you along. you have for sql "title , upload_1, upload_2, upload_3, upload_4, upload_5" if added a text input alongside your file upload in the form and label it description, add this line in your while loop and after value check $description = $_POST["description"][$key]; $escaped_description = htmlentities($description, ENT_NOQUOTES); then use this in your form File: <input type="file" name="file[]"/> Description:<input size="80"type="text" name="description[]" style="color: #FFFFFF; font-family: Verdana; font-weight: bold; font-size: 12px; background-color: #000000;" size="15" value=""><br/> So can make a sql insert something like this: <?php //these are just some random type examples of renaming to prevent bad syntax errors into mysql $sql_title = $info['title']; $sql_upload = $_FILE['name']['key']; $final_file_location = "$destpath$filename"; /*mysql connection login info change these values to your own*/ $con_upload = mysql_connect("localhost","username","password"); if (!$con_upload) { die('Could not connect: ' . mysql_error()); } /*connect to database - change databasename to own*/ mysql_select_db("databasename", $con_upload); mysql_query('SET NAMES utf8'); //this line fir whatever values your database has mysql_query("INSERT INTO files_data (title, description, file_location) //this line can be any variable and follows same positions as above VALUES ('$sql_title', '$escaped_description', '$final_file_location')"); //always close the connection mysql_close($con_upload); ?> Here is a sql statement to create the table and fields CREATE TABLE `files_data` ( `id` int(11) NOT NULL auto_increment, `title` varchar(255) default NULL, `description` text, `file_location` varchar(255) default NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=utf8 AUTO_INCREMENT=3 ; -- -- Dumping data for table `files_data` -- INSERT INTO `files_data` VALUES (1, 'Title', 'First Description.', 'files/filenumber1'); INSERT INTO `files_data` VALUES (2, 'Title2', 'Second Description', 'files/filenumber2'); -
upload a few files and then have them rename with rand()?
QuickOldCar replied to SnakZ's topic in PHP Coding Help
What exactly are you trying to make here. I understand would like to save the values, but for what reason. This gonna be a large thumbnail gallery, individual posts per image, will there be users areas or folders, categories. It's some things should consider and have a plan for this. Sure can do some sql insertions going by auto increment and id numbers, title,description,image location -
I believe you would have to change the POST values to GET values for SelectedID
-
upload a few files and then have them rename with rand()?
QuickOldCar replied to SnakZ's topic in PHP Coding Help
Are you placing the sql line within the while loop? It should do for each one if did that, otherwise if would like to save all as a group can make it an array $filenames[] = $filename; -
upload a few files and then have them rename with rand()?
QuickOldCar replied to SnakZ's topic in PHP Coding Help
Well actually I rewrote this into a more usable thing. I added the image source display, but can remove it. I must advise you should be having checks for max filesize and types. <?php $timestamp = time();; $destpath = "images/"; $target = "$target$the_file"; while(list($key,$value) = @each($_FILES["file"]["name"])) { if(!empty($value)){ if ($_FILES["file"]["error"][$key] > 0) { echo "Error: " . $_FILES["file"]["error"][$key] . "<br/>" ; } else { $file_name = $timestamp.$_FILES['file']['name']; $source = $_FILES["file"]["tmp_name"][$key] ; $filename = $_FILES["file"]["name"][$key]; $filename = strtolower($filename); $filename = "$timestamp$filename"; move_uploaded_file($source, $destpath . $filename) ; $final_file_location = "$destpath$filename"; echo "Uploaded to: $final_file_location<br/>"; //just remove the image source code below if not for images ?> <h2><a href="<?php echo $final_file_location; ?>" target="_blank"><img src="<?php echo $final_file_location; ?>" border="0"></a></h2> <?php } } } if(move_uploaded_file($_FILES['uploaded']['tmp_name'], $target)) { echo "The file has been uploaded as $filename"; } else { echo "Upload your file."; } ?> <form action="upload.php" method="post" enctype="multipart/form-data"> File: <input type="file" name="file[]"/><br/> File: <input type="file" name="file[]"/><br/> File: <input type="file" name="file[]"/><br/> <input type="submit" name="submit" value="Upload"/> </form> -
upload a few files and then have them rename with rand()?
QuickOldCar replied to SnakZ's topic in PHP Coding Help
I thought more about this. Just make it timestamp $timestamp = "date('Y-m-d H:i:s')"; $image_value = "$timestamp.$ext"; Please choose a file: <input name="uploaded" type="file" /><br /> <input type="submit" value="Upload" /> </form> //This function separates the extension from the rest of the file name and returns it function findexts ($filename) { $filename = strtolower($filename) ; $exts = split("[/\\.]", $filename) ; $n = count($exts)-1; $exts = $exts[$n]; return $exts; } //This applies the function to our file $ext = findexts ($_FILES['uploaded']['name']) ; //This line assigns a timestamp. $timestamp = "date('Y-m-d H:i:s')"; //This takes the timestamp you generated and adds a . on the end, so it is ready of the file extension to be appended. $timestamp = $timestamp"."; //This assigns the subdirectory you want to save into... make sure it exists! $target = "images/"; //This combines the directory, the random file name, and the extension $target = "$target$timestamp$ext"; if(move_uploaded_file($_FILES['uploaded']['tmp_name'], $target)) { echo "The file has been uploaded as "$timestamp$ext; } else { echo "Sorry, there was a problem uploading your file."; } -
Why not just do this as a fix if(!$show_poll) { $results = webPoll::getData($this->md5); $votes = array_sum($results); } else { $results = "No Data"; $votes = 0; }
-
upload a few files and then have them rename with rand()?
QuickOldCar replied to SnakZ's topic in PHP Coding Help
Is a few ways to go about this. Giving them a random number, well.....eventually it will give the file the same number,but here is the code for that. $random_number = rand(1,50000)-1;//change 50000 to max number you would like If you truly want unique, rename the file with a md5 of the name of the file. $md5_image = md5($image_name.jpg); I suggest saving these values as you can't reverse the md5, so set a database and save the md5 image value if need to reference it in some way, if it's just displaying the images what I do for my thumbserver is on the order of this. I go by the url of the website, convert url to md5 value,then save it as a md5 with png extension. http://www.computech.net/ becomes aa5f43bb38e109925f4cb23ea7046d8c So the image would then be aa5f43bb38e109925f4cb23ea7046d8c.png Now here's a bit of code to show how i find it, I really suggest saving these values, in my case I do not, I let it search for multiple values and give me a match, otherwise get the image through firefox, but lets not get into that process. $image_value = "http://www.computech.net/";//this could be a variable versus this website $image_md5 = md5($image_value);//convert to md5 $image_location = "./thumb/$image_md5.png";//folder images are saved andadd the md5 plus extension //see if file exists , if does use it, if not do something else if (file_exists($image_location)) { $source_image = $image_location; } else { $source_image = "Some alternate image or message"; } -
Ha ha, Sorry I missed about what you said about the url. The below code will do as you need. I had $url = mysql_real_escape_string($_GET['url']); That was so you would be able to do any url with a get request , The links would have looked something like http://mysite.com/simple-parse.php?url=http://somesite.com So the below code all you have to do is visit the page or do an include with the site specified. <?php include('simple_html_dom.php'); function getHost($url) { $parseUrl = parse_url(trim($url)); return trim($parseUrl[host] ? $parseUrl[host] : array_shift(explode('/', $parseUrl[path], 2))); } $url = "http://schulnetz.nibis.de/db/schulen/schule.php?schulnr=35877&lschb="; //simple way to add the http:// that dom requires, using curl is a better option if (substr($url, 0, 4) != "http") { $url = "http://$url"; } $parsed_url = getHost($url); $http_parsed_host = "http://$parsed_url/"; $html = file_get_html($url); foreach($html->find('a') as $element) $dom = new DOMDocument(); @$dom->loadHTML($html); $xpath = new DOMXPath($dom); $hrefs = $xpath->evaluate("/html/body//a"); for ($i = 0; $i < $hrefs->length; $i++) { $href = $hrefs->item($i); $href_link = $href->getAttribute('href'); $parse_count = count("$http_parsed_host"); $substr_count = +7; if (substr($href_link, 0, $substr_count) == "mailto:") { $mail_link = $href_link; $href_link = trim($mail_link,$href_link); } if (substr($href_link, 0, 1) == "/") { $href_link = trim($href_link,"/"); } if (substr($href_link, 0, 2) == "//") { $href_link = trim($href_link,"//"); } if (substr($href_link, 0, 3) == "///") { $href_link = trim($href_link,"///"); } if ((substr($href_link, 0, == "https://") OR (substr($href_link, 0, 12) == "https://www.") OR (substr($href_link, 0, 7) == "http://") OR (substr($href_link, 0, 11) == "http://www.") OR (substr($href_link, 0, 6) == "ftp://") OR (substr($href_link, 0, 11) == "feed://www.") OR (substr($href_link, 0, 7) == "feed://")) { $final_href_link[] = $href_link; } else { if (substr($href_link, 0, 1) != "/") { $final_href_link[] = "$http_parsed_host$href_link"; } } } $links_array = array_unique($final_href_link); sort($links_array); foreach ($links_array as $links) { //echo "$links<br />"; echo "<a href='$links'>$links</a><br />"; } echo "<a href='$mail_link'>$mail_link</a><br />"; ?>
-
You need to add your meta data such as title,description,keywords.
-
Browser game - Site design and game mechanics
QuickOldCar replied to intellix's topic in Website Critique
Hard to read whats in the footer. -
Seems nice to me and works well. But why would you use a tiny url and not http://www.philmarkham.com/
-
As for the first code I did. This line: $final_href_link[] = $href_link; { Should be this: $final_href_link[] = $href_link; But the last code works much better. Thanks for the kind words, and I was just letting you know within the code I wrote that I had it that simple_dom file was in the same folder. You can place it anywhere that can be accessed. I just find it easier if was maybe all in an include folder in root, or in the same folder as what you are doing.
-
Parse Errors: Hours of Head Banging Fun
QuickOldCar replied to Nathan54AB's topic in PHP Coding Help
<!DOCTYPE html PUBLIC "-//W3C// DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/shtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="content-type" content="text/html; charset=iso-8859-1" /> <title>Registration Form</title> </head> <body><?php ini_set('display_errors', 1); // Defines variables from registration.html. $firstName = $_POST['firstName']; $lastName = $_POST['lastName']; $email = $_POST['email']; $password = $_POST['passowrd']; $confirm = $_POST['confirm']; $birthday = $_POST['birthday']; $favGameGenre = $_POST['favGameGenre']; ?> <p>Registration results:</p> <?php if(empty($firstName)) { echo " <p>You must enter your first name.</p> "; } if(empty($lastName)) { echo " <p>You must enter your last name.</p> "; } if(empty($email)) { echo " <p>You must enter your email</p> "; } if(empty($password)) { echo " <p>You must enter a password</p> "; } ?> </body> </html> -
You are welome. I used this method in creating a paginated full search/navigation system with multiple selects for mysql. Converting the count to page numbers and a whole lot of math and determining mysqls start row for the selects. Then set max pages to those values. I'd post the code but is so complex would not be usable unless under real circumstances will all the correct parameters set.
-
<?php $newvar = mysql_real_escape_string($_GET['newvar']); $home_url = "http://".$_SERVER['HTTP_HOST']; $url = "http://".$_SERVER['HTTP_HOST'].$_SERVER['SCRIPT_NAME']; if (!empty($_SERVER["QUERY_STRING"])){ $url .= "?".$_SERVER['QUERY_STRING']; } if ($url == "$home_url") { $url = "$url?$newvar"; } echo "<a href='$home_url'>Home</a><br />"; echo "<a href='$url'>$url</a><br />"; ?> <form name="input" action="<?php echo $url; ?>" method="get"> New Variable:<input size="30"type="text" name="newvar" style="color: #FFFFFF; font-family: Verdana; font-weight: bold; font-size: 12px; background-color: #000000;" size="15" value="<?php echo $_GET['newvar']; ?>"> <input type="submit" style="color: #FFFFFF; font-family: Verdana; font-weight: bold; font-size: 12px; background-color: #000000;" size="15" value="Make New Variable" /> </form> <?php echo "Compliments of Quick"; ?> And to see in action http://dynaindex.com/test-new-query
-
Here is the image and code <?php //<img src="quick-image.php" alt="you are so sweet " width="200" height="100"> $start_left_line = 30; $end_right_line = 160; $left_tilt = 45; $right_tilt = 45; $quick_img = imagecreate( 200, 100 ); $background = imagecolorallocate( $quick_img, 192, 255, 255 ); $text_color = imagecolorallocate( $quick_img, 238, 44, 44 ); $line_color = imagecolorallocate( $quick_img, 255, 246, 143 ); imagestring( $quick_img, 4, 30, 25, "you are so sweet", $text_color ); imagesetthickness ( $quick_img, 5 ); imageline( $quick_img, $start_left_line, $left_tilt, $end_right_line, $right_tilt, $line_color ); header( "Content-type: image/png" ); imagepng( $quick_img ); imagecolordeallocate( $line_color ); imagecolordeallocate( $text_color ); imagecolordeallocate( $background ); imagedestroy( $quick_img ); ?> If would like to see the result look here. http://dynaindex.com/quick-image
-
Change this line in your my.cnf file under the etc folder [mysqld] ft_min_word_len = 2 You can also use in boolean mode to be more accurate like exact phrase, beginning,ending or with a word, and so on. It's the advanced search mode. The way I do it is a multiple select to mysql using get values from dropdowns to change the modes. and a search for ronnie's would actually become a search for ronnie\'s because mysql escapes the quote
-
My best advice would be to tell you use the function imageline(). And then link you to the manual. http://nl3.php.net/imageline