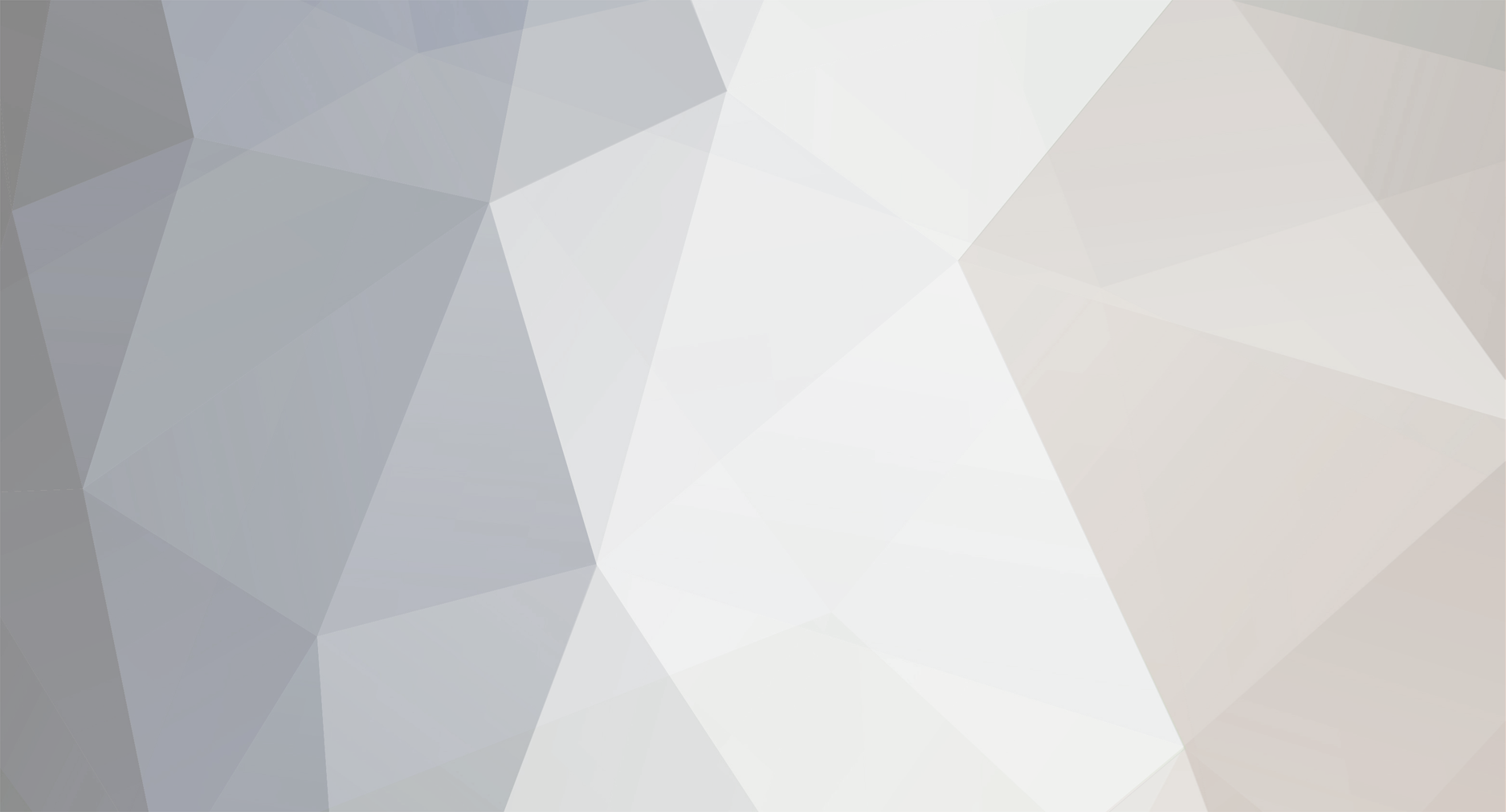
QuickOldCar
-
Posts
2,972 -
Joined
-
Last visited
-
Days Won
28
Posts posted by QuickOldCar
-
-
Would be easier for us to see the code you are using now.
You can use the email filter
<?php $email = "john@test.com, peter@test.com"; if(filter_var($email, FILTER_VALIDATE_EMAIL)){ echo $email; }else{ echo $email." Failed"; } ?>
-
Look at your last php block, you had an else where should be an echo
You can work out the if else issues if there is any
A suggestion would be to do all php processing above and the html display below in your code.
<?php include_once("init.php"); // Use session variable on this page. This function must put on the top of page. if (!isset($_SESSION['username']) || $_SESSION['usertype'] != 'admin') { // if session variable "username" does not exist. header("location: index.php?msg=Please%20login%20to%20access%20admin%20area%20!"); // Re-direct to index.php exit; } else { error_reporting(0); if (isset($_GET['sid'])) { echo $_GET['sid']; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1"/> <title>Simple invoice in PHP</title> <style type="text/css"> body { font-family: Verdana;; } div.invoice { border:1px solid #ccc; padding:10px; height:740pt; width:570pt; } div.company-address { border:1px solid #ccc; float:left; width:200pt; } div.invoice-details { border:1px solid #ccc; float:right; width:200pt; } div.customer-address { border:1px solid #ccc; float:right; margin-bottom:50px; margin-top:100px; width:200pt; } div.clear-fix { clear:both; float:none; } table { width:100%; } th { text-align: left; } td { } .text-left { text-align:left; } .text-center { text-align:center; } .text-right { text-align:right; } </style> </head> <body> <div class="invoice"> <div class="company-address"> <?php $sid = $_GET['sid']; $line = $db->queryUniqueObject("SELECT * FROM stock_sales WHERE transactionid='$sid' "); $mysqldate = $line->date; $phpdate = strtotime($mysqldate); $phpdate = date("d/m/Y", $phpdate); echo $phpdate; ?><?php echo $sid; ?> <?php $line4 = $db->queryUniqueObject("SELECT * FROM store_details "); ?> <?php echo $line4->name; ?> <br /> <?php echo $line4->address; ?>,<?php echo $line4->place; ?> <br /> LPhone <strong>:<?php echo $line4->phone; ?></strong> <br /> </div> <div class="invoice-details"> Invoice no : <?php echo $sid; ?> <br /> Date: <?php $sid = $_GET['sid']; $line = $db->queryUniqueObject("SELECT * FROM stock_sales WHERE transactionid='$sid' "); $mysqldate = $line->date; $phpdate = strtotime($mysqldate); $phpdate = date("d/m/Y", $phpdate); echo $phpdate; ?> </div> <div class="customer-address"> To: <br /> <?php echo $line->customer_id; $cname = $line->customer_id; $line2 = $db->queryUniqueObject("SELECT * FROM customer_details WHERE customer_name='$cname' "); echo $line2->customer_address; ?> <br /> 123 Long Street <br /> London, DC3P F3Z <br /> </div> <div class="clear-fix"></div> <table border='1' cellspacing='0'> <tr> <th width=250>Description</th> <th width=80>Quantity</th> <th width=100>Unit price</th> <th width=100>Total price</th> </tr> <?php $db->query("SELECT * FROM stock_sales where transactionid='$sid'"); while ($line3 = $db->fetchNextObject()) { ?> echo("<tr>"); echo("<td><?php echo $line3->stock_name; ?></td>"); echo("<td class='text-center'><?php echo $line3->quantity; ?></td>"); echo("<td class='text-right'><?php echo $line3->selling_price; ?></td>"); echo("<td class='text-right'><?php echo $line3->amount; ?></td>"); echo("</tr>"); } ?> echo("<tr>"); echo("<td colspan='3' class='text-right'>Sub total</td>"); echo("<td class='text-right'><?php $subtotal = $line3->subtotal; ?></td>"); echo("</tr>"); echo("<tr>"); echo("<td colspan='3' class='text-right'>VAT</td>"); echo("<td class='text-right'><?php $discount = $line3->discount; ?></td>"); echo("</tr>"); echo("<tr>"); echo("<td colspan='3' class='text-right'><b>TOTAL</b></td>"); echo("<td class='text-right'><b><?php $payment = $line3->payment; ?></b></td>"); echo("</tr>"); </table> </div> </body> </html> <?php echo "Error in processing printing the sales receipt"; } } ?>
-
How to create virtual host?
-
If using a local environment can use http://localhost
Can edit your hosts file located in C:\Windows\System32\drivers\etc , show all hidden files
192.168.1.194 assetsvr
-
mysqli_ has now been removed from PHP.
mysql_ has now been removed from PHP.
-
If this was written all the processing code up top and display output all below could have used header() prior to any output.
You can add a meta refresh in the place you have header() commented out.
echo "<meta http-equiv='refresh' content='5;URL='members_roster.php' />";
-
Actually when comes to chat is good to have your own chat server and based on sockets if server is adequate. http://socket.io/
There are a pile of IRC frontends out there can use. https://kiwiirc.com/
Can use some embedded chats like chatango , xat , cbox , shoutmix and piles others.
The cms I mentioned have a few chats can install as plugins/modules.
-
If you were not the one that posted will remove the warning, at least we know was not you and apologies.
-
To help troubleshoot you can turn on and display error reporting, also echo your queries or variables to make sure is what you expect.
-
-
-
-
This forum is designed to help people with problems their existing code, not make it.
First you would have to detect it's file type.
Depending what type it is handle in different ways.
For pdf need something that can read,extract,convert the pages into plain text
Once you can extract these document types into a similar format you can save them line by line.
As for getting specific data, you can try regex and matching data, seems to me would be quite difficult to do different patterns in files because nothing would be similar named or positions. Also have to deal with languages.
An online resume form and hiring a human as data entry is most likely the best solution to this.
I'm not saying it can't be done, but surely is not going to be fast and easy.
I would bet not one CV/Resume Parser is accurate.
-
You can get the html by using curl or file_get_contents
User can insert a url into a form, your script fetches information server side and save it.
-
1
-
-
Is already a plugin made for wordpress, although is older and not been updated. Most likely someone made a new version.
-
Had this for someone else, can use in any way need to with tracking clicks or views, Iframe,include,ajax. With a js onclick event or simple href link.
Could change it around to add views or clicks each users name or user id, matter of editing it.
http://forums.phpfreaks.com/topic/296442-unique-click-tracking-using-php/?p=1512504
-
No reason to drag up 6 year old posts
-
The op is calling the content being shared as shares.
see this area?
if ($lang->getTag() == 'it-IT') { $pageurl = 'http://'.$_SERVER['HTTP_HOST'].$urls; $spageurl = 'https://'.$_SERVER['HTTP_HOST'].$urls; } else { $pageurl = 'http://'.$_SERVER['HTTP_HOST'].$urls; $spageurl = 'https://'.$_SERVER['HTTP_HOST'].$urls; }
remove the links that have https, I'll comment them out
if ($lang->getTag() == 'it-IT') { $pageurl = 'http://'.$_SERVER['HTTP_HOST'].$urls; //$spageurl = 'https://'.$_SERVER['HTTP_HOST'].$urls; } else { $pageurl = 'http://'.$_SERVER['HTTP_HOST'].$urls; //$spageurl = 'https://'.$_SERVER['HTTP_HOST'].$urls; }
-
I don't have the rest of the class but that won't matter.
They changed the code since that script was made, is now all js and there is no class="mnllinklist dotted" within a td in the html code.
They don't want others scraping their links.
-
I suppose that will depend the rest of your code and what doing with it.
-
If want to match or more accurately compare something you can loop through an array with a foreach or for loop using a comparison operator , can also use some regex with preg_match or preg_match_all for multiples
Check if a specific value exists in the array using in_array true/false
array_search can return the key of the array
-
Still down 2 months later
-
A few ways can go about it
Do additional LIKE for each additional column want to look within
Make the column name a dynamic variable
Add a form and use if/else or a switch to perform different queries
Can use fulltext search in boolean mode and simplify the queries a lot
-
It's better to use password_hash and password_verify
Not too good to redirect back to the same script, could find yourself in an endless loop, redirect to the main site or somewhere else
if($_SESSION['user']==''){ header("Location:affiliate-login.php"); }else{
Php restrict emails
in PHP Coding Help
Posted · Edited by QuickOldCar
If you want to use just the first one can do something like this.