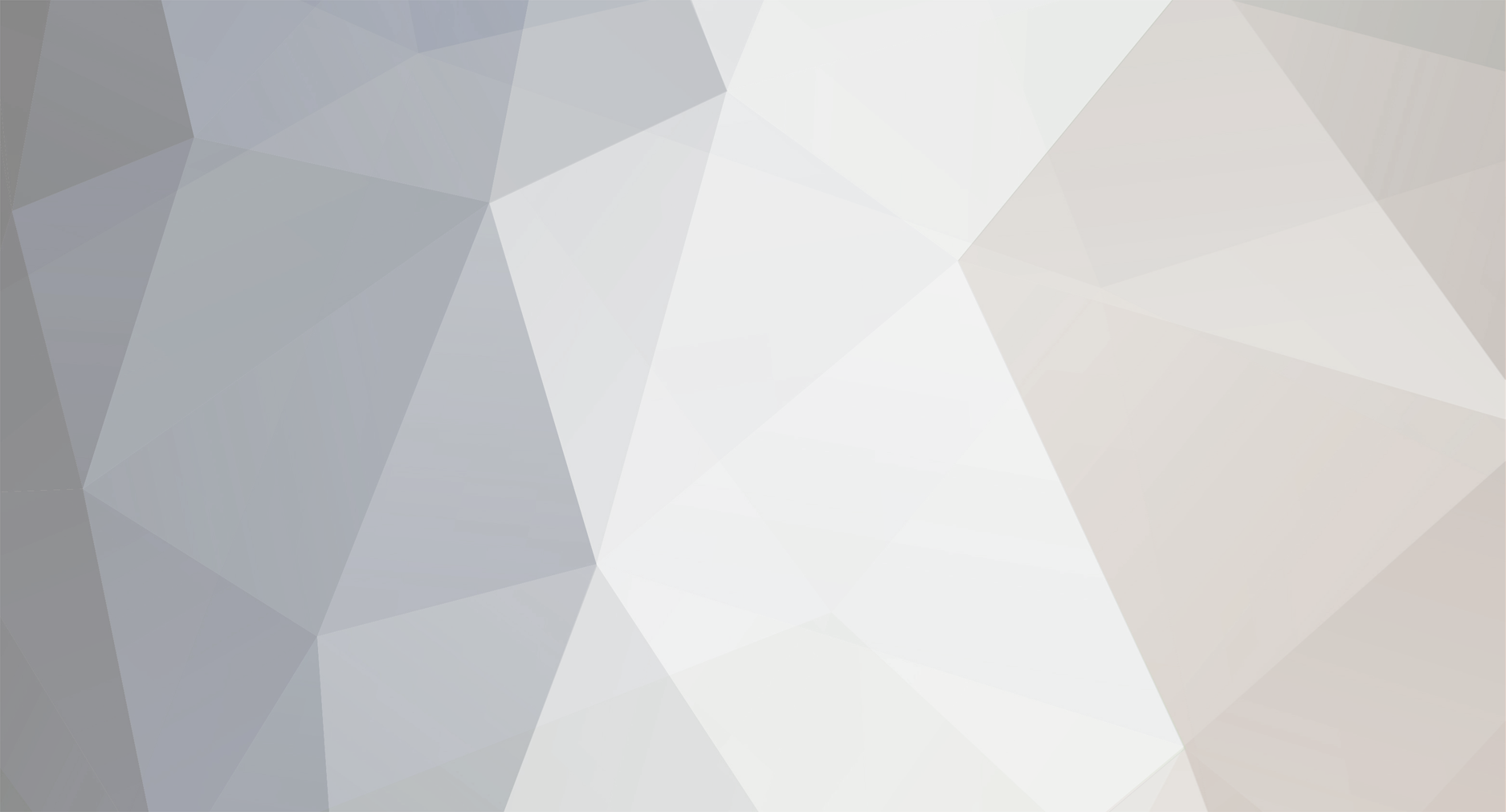
INeedAGig
-
Posts
112 -
Joined
-
Last visited
Never
Posts posted by INeedAGig
-
-
Still getting the incorrect echo....
-
Hmmmm...still echoing incorrect login credentials...
-
Chris, thank you for the reply! I went ahead and made the suggested changes but now it is echoing that I entered incorrect login information, even though it is correct....
-
*bump*
-
Still getting a blank page...I'm not sure what's going on...
-
Any ideas?
-
I'm still learning
What would you recommend? The remaing code on the main_interface.php is coding to load and display data for a different table in the same database. Any idea why I am getting a blank page after logging in? Thanks!
-
Okay, I went ahead and completely changed the coding for the login, but it is still returning a blank page. I have attached code for reference.
Login Page
<?php session_start(); // dBase file include "dbConfig.php"; if ($_GET["op"] == "login") { if (!$_POST["username"] || !$_POST["password"]) { die("Incorrect Username or Password, please check your credentials!"); } // Create query $q = "SELECT * FROM `access` " ."WHERE `username`='".$_POST["username"]."' " ."AND `password`=PASSWORD('".$_POST["password"]."') " ."LIMIT 1"; // Run query $r = mysql_query($q); if ( $obj = @mysql_fetch_object($r) ) { // Login successful, create session variables $_SESSION["valid_id"] = $obj->id; $_SESSION["valid_user"] = $_POST["username"]; $_SESSION["valid_time"] = time(); // Redirect to main database page Header("Location: main_interface.php"); } else { // Login not successful die("Unable to login, please verify your information."); } } else { //If all went right the web form appears and users can log in echo "<form action=\"?op=login\" method=\"POST\">"; echo "Username: <input name=\"username\" size=\"15\"><br />"; echo "Password: <input type=\"password\" name=\"password\" size=\"8\"><br />"; echo "<input type=\"submit\" value=\"Login\">"; echo "</form>"; } ?>
This is the first code on 'main_interface.php'
session_start(); if (!$_SESSION["valid_user"]) { // User not logged in, redirect to login page header("Location: interface_login.php"); }
-
Hey there, have a small issue at hand. My login script keeps returning a blank page. It does a proper echo if you enter an incorrect username or password, but if you enter correct information is returns only a blank page. Here is the code from the php file that checks and processes the username and password the user enters in the form on the login page.
<?php $host="removed_for_this_post"; // Host name $username="removed_for_this_post"; // Database Username $password="removed_for_this_post"; // Database password $db_name="removed_for_this_post"; // Database Name $tbl_name="access"; // Table name // Connect to server and select databse. mysql_connect("$host", "$username", "$password")or die("ALERT! Unable to connect to database!"); mysql_select_db("$db_name")or die("ALERT! Unable to select database!"); // Username and password sent from form $username=$_POST['username']; $pass=$_POST['pass']; // To protect against MySQL injection $username = stripslashes($username); $pass = stripslashes($pass); $username = mysql_real_escape_string($username); $pass = mysql_real_escape_string($pass); $query="SELECT * FROM $tbl_name WHERE username='$username' and password='$pass'"; $result=mysql_query($query); // Mysql_num_row is counting table row $count=mysql_num_rows($result); if($count==1){ // Register $username, $pass and redirect to main database page session_register("username"); session_register("pass"); header("location: main_interface.php"); } else { echo "Wrong Username or Password, please check check your credentials."; } ?>
Thank you in advance for your assistance!
-
Hey hey, coming off a little harsh there!
I was reviewing a tutorial, on this site actually, I just wanted to make sure that this would be the correct page for me to code it and if there might be an easier way.
-
Hey there, I have never really done pagination before and I was wondering if anyone can take a look at my code and help me with coding pagination? This database will be storing a lot of information and I am going to have the need to break it into multiple pages.
The following code is the code from my results page, which is where I assume I will need to code the pagination. This is the page that displays all of the results after everything is processed and entered into the database in a different php file.
<?php $username="removed_for_this_post"; //Database Username $password="removed_for_this_post"; //Database Password $database="removed_for_this_post"; //Database Name mysql_connect("localhost",$username,$password); //Connection to database @mysql_select_db($database) or die("ALERT! Database not found!"); //Selection of database $query="SELECT * FROM leads ORDER by id DESC"; //Database table to query $result=mysql_query($query); $num=mysql_numrows($result); mysql_close(); ?> <html> <head> <link href="db_style.css" rel="stylesheet" type="text/css" media="screen" /> </head> <body> <div id="wrap"> <div id="header"> <div id="hdr_content"> </div> </div> <br><br><br><br> <center> <table border="1" bordercolor="#000000" cellspacing="2" cellpadding="10"> <tr> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Name</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">E-Mail</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Age</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Gender</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Location</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Home Phone</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Other Phone</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Best Time to Reach</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Referrer</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF">Options</font></th> </tr> </div> <div id="footer"> <div class="footer_content"> <font class="footer_header"> </div> </div> <?php $i=0; while ($i < $num) { $id=mysql_result($result,$i,"id"); //Unique ID Field $name=mysql_result($result,$i,"name"); //Name $email=mysql_result($result,$i,"email"); //EMail Address $age=mysql_result($result,$i,"age"); //Age $gender=mysql_result($result,$i,"gender"); //Gender $location=mysql_result($result,$i,"location"); //City of Residence $homephone=mysql_result($result,$i,"homephone"); //Home Phone Number $otherphone=mysql_result($result,$i,"otherphone"); //Secondary Phone Number $besttime=mysql_result($result,$i,"besttime"); //Best Time to Reach $referrer=mysql_result($result,$i,"referrer"); //Referrer ?> <tr> <td align="center" bgcolor="#CCCCCC"><font class="lead_txt"><? echo $name; ?></font></td> <td align="center" bgcolor="#CCCCCC"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $email; ?></font></td> <td align="center" bgcolor="#CCCCCC"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $age; ?></font></td> <td align="center" bgcolor="#CCCCCC"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $gender; ?></font></td> <td align="center" bgcolor="#CCCCCC"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $location; ?></font></td> <td align="center" bgcolor="#CCCCCC"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $homephone; ?></font></td> <td align="center" bgcolor="#CCCCCC"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $otherphone; ?></font></td> <td align="center" bgcolor="#CCCCCC"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $besttime; ?></font></td> <td align="center" bgcolor="#CCCCCC"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $referrer; ?></font></td> <td bgcolor="#01337f""><a href="db_edit.php?id=<?php echo $id; ?>"><img src="edit.png" width="25" height="25" alt="Edit"></a> <a href="db_remove.php?id=<?php echo $id; ?>"><img src="delete.png" width="25" height="25" alt="Delete"></a> <a href="email_lead.php?id=<?php echo $name, $email; ?>"><img src="email.png" width="25" height="25" alt="E-Mail"></a></td> </tr> </center> </font> </body> </html> <?php $i++; } echo "</table>"; ?>
-
Hey guys, how is everyone today?
I am needing a little help with this one. I have a database that updates itself and the display page for it with every form submission on the website. I am wondering how I go about getting it to display across multiple pages? For example, once it hits 25 submissions, it would make a page 2, once page two hits 25 submissions, it creates page 3, etc. etc. Much like you see on something like a forum, it hits so many replies before it creates a new page, to limit one long page of results.
Thanks in advance!!
-
Well, I resolved the delete issue, its just the edit that isn't fully working, it doesn't display the updated information.
-
Okay, I have the edit and delete working, with confirmation with an echo statement. But, for some reason it is not showing up as edited or deleted on the main page, it keeps the same information that was originally there, what might the issue be with it not displaying the updated information or being deleted?
Thanks!
-
Problem solved. Just had to fetch the id from the database, which I failed to add to my code.
Changed it to:
<? $i=0; while ($i < $num) { $id=mysql_result($result,$i,"id"); $name=mysql_result($result,$i,"name"); $email=mysql_result($result,$i,"email"); $age=mysql_result($result,$i,"age"); $gender=mysql_result($result,$i,"gender"); $location=mysql_result($result,$i,"location"); $homephone=mysql_result($result,$i,"homephone"); $otherphone=mysql_result($result,$i,"otherphone"); $besttime=mysql_result($result,$i,"besttime"); $referrer=mysql_result($result,$i,"referrer"); ?>
The edit is now working
-
Okay, I reviewed the last few posts and made all suggested changes, but, I am still drawing a blank page. The only difference is in the url in the taskbar now, before it was displaying the ?id=$id but now it is displaying only ?id= and of course...still a blank page...thanks for the help so far!!
-
Wow, I didn't even notice that. I went ahead and took care of that, but I am still drawing a blank page.
-
This is my code in db_edit.php
<? $id=$_GET['id']; $username="myusernameishere"; $password="mypasswordishere"; $database="mydatabaseishere"; mysql_connect("localhost",$username,$password); $query="SELECT * FROM leads WHERE id='$id'"; $result=mysql_query($query); $num=mysql_numrows($result); mysql_close(); $i=0; while ($i < $num) { $name=mysql_result($result,$i,"name"); $email=mysql_result($result,$i,"email"); $age=mysql_result($result,$i,"age"); $gender=mysql_result($result,$i,"gender"); $location=mysql_result($result,$i,"location"); $homephone=mysql_result($result,$i,"homephone"); ?> <form action="db_updated.php" method="post"> <input type="hidden" name="ud_id" value="<? echo $id; ?>"> Name: <input type="text" name="ud_name" value="<? echo $name; ?>"><br> E-Mail: <input type="text" name="ud_email" value="<? echo $email; ?>"><br> Age: <input type="text" name="ud_company" value="<? echo $age; ?>"><br> Gender: <input type="text" name="ud_phone" value="<? echo $gender; ?>"><br> Location: <input type="text" name="ud_message" value="<? echo $location; ?>"><br> Home Phone: <input type="text" name="ud_referrer" value="<? echo $homephone; ?>"><br> <input type="Submit" value="Update"> </form> <? ++$i; } ?>
-
Any ideas??
-
Hi there, I have been following this tutorial: http://www.freewebmasterhelp.com/tutorials/phpmysql/6
I have everything working, but I am a little confused when it comes to the "Links for Single Records" part. The following code snippet is what I am using to display the data that is in the database on the page after it is inserted, inside of a table. It is working fine, but the "edit" link just brings up a blank page, that has my edit code on it.
<table border="1" bordercolor="#000000" cellspacing="2" cellpadding="10"> <tr> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Name</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">E-Mail</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Age</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Gender</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Location</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Home Phone</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Other Phone</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Best Time to Reach</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2">Referrer</font></th> <th bgcolor="#01337f"><font face="Arial, Helvetica, sans-serif" size="2" color="#FFFFFF">Options</font></th> </tr> <? $i=0; while ($i < $num) { $name=mysql_result($result,$i,"name"); $email=mysql_result($result,$i,"email"); $age=mysql_result($result,$i,"age"); $gender=mysql_result($result,$i,"gender"); $location=mysql_result($result,$i,"location"); $homephone=mysql_result($result,$i,"homephone"); $otherphone=mysql_result($result,$i,"otherphone"); $besttime=mysql_result($result,$i,"besttime"); $referrer=mysql_result($result,$i,"referrer"); ?> <tr> <td><font class="lead_txt"><? echo $name; ?></font></td> <td><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $email; ?></font></td> <td align="center"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $age; ?></font></td> <td align="center"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $gender; ?></font></td> <td align="center"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $location; ?></font></td> <td align="center"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $homephone; ?></font></td> <td align="center"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $otherphone; ?></font></td> <td align="center"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $besttime; ?></font></td> <td align="center"><font face="Arial, Helvetica, sans-serif" size="2" class="lead_txt"><? echo $referrer; ?></font></td> <td bgcolor="#01337f""><a href="db_edit.php?id=$id"><img src="edit.png" width="25" height="25" alt="Edit"></a> <a href=""><img src="delete.png" width="25" height="25" alt="Delete"></a> <a href=""><img src="email.png" width="25" height="25" alt="E-Mail"></a></td> </tr>
Any ideas? Thanks in advance!!
-
Any ideas??
-
Okay, I have been following this tutorial: http://www.freewebmasterhelp.com/tutorials/phpmysql/1 to achieve exactly what I wanted to get done. I also followed the way to have it formatted in tables and added extra columns that I needed, included an "Options" column which houses three links, including "edit" and "delete"
Now, I have everything working fine but I am stumped on how to get the "edit" and "delete" links to work for each individual entry that is listed. I have to have these features so the entries can be edited and deleted without having to physically go into the MySQL database to do it. The tutorial explains how to do it in Step 6, but I am confused. I'm not quite sure where to place the code for the links, which are generated automatically every time a new entry is inputted into the database.
Anybody available to help me out? Thanks!
-
Okay, I am going to try to explain this the best I can, I appreciate and thank you for your help in advance!
Okay, this is what I am wanting to do. I want to create a user area on my website. I don't need a common page that every user see's but each user to have there own unique page that they are brought to once they log-in, where they will have there own content that I will change and update often.
As for the usernames and passwords, I will be creating these when needed and giving them to the corresponding user, so I am not in need of a registration option. I am needing assistance on how to setup the MySQL database and the PHP coding for this. Remember, I am going to need to be able to add new users w/ passwords when needed.
Thanks for your help!
-
SOLVED.
It was simple actually, didn't realize I had the PHP file encoded in UTF-8 instead of ANSI, blah!
Login returning blank page
in PHP Coding Help
Posted
Whats the best way to fix this?