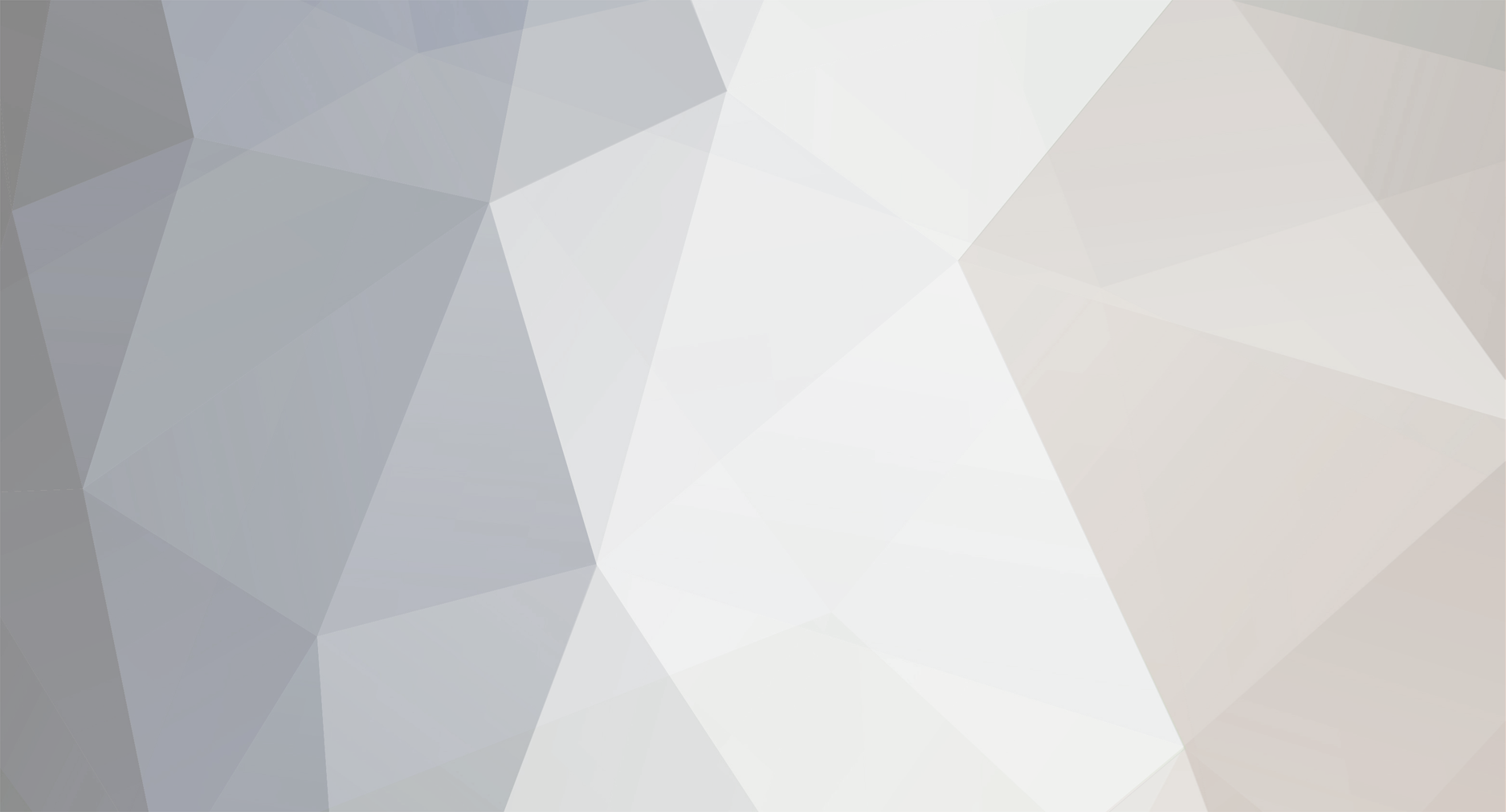
randal_138
Members-
Posts
20 -
Joined
-
Last visited
Never
Everything posted by randal_138
-
I modified it so that it would be easier to read with only what I need to submit. I did try this: <?php include('connection.php'); $query = "SELECT * FROM FORUM_MESSAGE ORDER BY MSG_DATE DESC"; $result = mysql_query($query); $num = mysql_num_rows($result); mysql_close(); $i = 0; while ($i < $num) { $subject = mysql_result ($result, $i, "SUBJECT"); $message_text = mysql_result ($result, $i, "MSG_TEXT"); echo '<div style="width: 400px;padding:20px;">'; echo '<table border=0 width="400px">'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo '</td>'; echo '<td style="vertical-align:top;width:320px;text-align:center;">'; echo '<form method="POST" name="subject">'; echo '<input type="hidden" name="update" value="' . $subject . '" />'; echo '<input type="submit" name="update" value="Update" />'; echo ' '; echo '<input type="hidden" name="delete" value="' . $subject . '" />'; echo '<input type="submit" value="Delete" />'; echo '</form>'; echo '</td>'; echo '</tr>'; echo '</table>'; echo '<br />'; echo '<hr />'; echo '</div>'; $i++; } if (isset($_POST['delete'])) { include ('connection.php'); mysql_query("DELETE FROM FORUM_MESSAGE WHERE SUBJECT = " . $subject); } ?> That is what I am working with right now. I don't think the $subject and the actual button are connecting, but I can't point what exactly is wrong. Ryan
-
My delete button will not work for some reason. I have created a loop, ordered my database entries with $i and have gotten closer, but not 100% of the way to making it work. I am trying to delete rows from my database individually. Not sure where I am going wrong with my syntax, but something is not working. The entries are not deleting nor am I receiving any errors. I am stumped. Here is the code. I shortened it for readability. <?php // database connection information include('connection.php'); $query = "SELECT * FROM FORUM_MESSAGE ORDER BY MSG_DATE DESC"; $result = mysql_query($query); $num = mysql_num_rows($result); $i = 0; while ($i < $num) { $subject = mysql_result ($result, $i, "SUBJECT"); $message_text = mysql_result ($result, $i, "MSG_TEXT"); echo '<form method="POST" name="subject">'; echo '<input type="hidden" name="update" value="<?php echo $subject; ?>" />'; echo '<input type="submit" name="update" value="Update" />'; echo ' '; echo '<input type="hidden" name="delete" value="' . $subject . '" />'; echo '<input type="submit" value="Delete" />'; echo '</form>'; $i++; } if (isset($_POST['delete'])) { include ('connection.php'); mysql_query("DELETE FROM FORUM_MESSAGE WHERE SUBJECT = " . $subject); } ?> </body> </html> Thanks again! Ryan
-
How would I have to word the query then? I need to make a connection between the delete button in that 'row' and pair it up with the primary key (SUBJECT) so that only that entry is deleted? Ryan
-
The only problem is that the INDEX column starts the increments at '1' and the $i increments start at '0'. I am trying to do a DELETE FROM table WHERE INDEX = $i + 1 (not exact syntax obviously), but that is not working out so well. Can I start the $i increments at '1'?
-
I thought that looked wrong... I still am stumped on the query: "DELETE FROM FORUM_MESSAGE WHERE ..." How do I get it to delete that numbered row? Maybe there is an easier way to do this, but I sure don't know it. Ryan
-
Pikachu, Too late. I was toying around with the script the other day and ended up with this: DELETE FROM FORUM_MESSAGE WHERE $i Of course, as soon as I clicked one of the buttons, the entire table was wiped. Good thing nothing important was on it... Will try the 'value' and reply back on whether or not it works. Oh, and I am trying to order my database results with the $i and then have the option to edit and delete the entries. Ryan
-
Here is the code for $num: $query = "SELECT * FROM FORUM_MESSAGE"; $result = mysql_query($query); $num = mysql_numrows($result); the entire code is here: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>My Profile</title> <link href="loginmodule.css" rel="stylesheet" type="text/css" /> </head> <body> <h1>My Profile </h1> <a href="member-index.php">Home</a> | <a href="member-profile.php">My Profile</a> | Update Posts | <a href="logout.php">Logout</a> <br /><br /> <?php // connection to DB $query = "SELECT * FROM FORUM_MESSAGE"; $result = mysql_query($query); $num = mysql_numrows($result); mysql_close(); $i = 0; while ($i < $num) { $subject = mysql_result ($result, $i, "SUBJECT"); $message_text = mysql_result ($result, $i, "MSG_TEXT"); echo '<div style="width: 400px;padding:20px;">'; echo '<table border=0 width="400px">'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo 'DB#: '; echo '</td>'; echo '<td style="vertical-align:top;width:320px;">'; echo '<div>'; echo $i; echo '</div>'; echo '</td>'; echo '</tr>'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo 'Subject: '; echo '</td>'; echo '<td style="vertical-align:top;width:320px;">'; echo '<div>'; echo $subject; echo '</div>'; echo '</td>'; echo '</tr>'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo 'Message: '; echo '</td>'; echo '<td style="vertical-align:top;width:320px;">'; echo '<div>'; echo '<textarea id="message_text" name="message_text" cols="50" rows="10">'; echo $message_text; echo '</textarea>'; echo '</div>'; echo '</td>'; echo '</tr>'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo '</td>'; echo '<td style="vertical-align:top;width:320px;text-align:center;">'; echo '<form method="post">'; echo '<input type="hidden" name="update" value="true" />'; echo '<input type="submit" value="Update" />'; echo ' '; echo '<input type="hidden" name="delete" value="true" />'; echo '<input type="submit" value="Delete" />'; echo '</form>'; echo '</td>'; echo '</tr>'; echo '</table>'; echo '<br />'; echo '<hr />'; echo '</div>'; $i++; } if($_POST['delete']) // from button name="delete" { for($i=0;$i<$num;$i++) { $sql = "DELETE FROM FORUM_MESSAGE WHERE $i='$num'"; $result = mysql_query($sql) or die (mysql_error()); } if($result) { echo 'Message deleted.'; } } ?> </body> </html> Hope this helps... Thanks again! Ryan
-
I feel dumb, but I am still new to all this. Here is the coding, but I still have incomplete syntax. <?php if($_POST['delete']) // from button name="delete" { for($i=0;$i<$num;$i++) { $sql = "DELETE FROM FORUM_MESSAGE WHERE '$num'='$i'"; $result = mysql_query($sql) or die (mysql_error()); } if($result) { header('Location: message-deleted.php'); } } ?> My problem is here: $sql = "DELETE FROM FORUM_MESSAGE WHERE '$num'='$i'"; What exact syntax to I need to use after "WHERE" ? Thanks again, Ryan
-
Having issues with a small piece of script. Trying to delete entries from a database. First, the essentials: the button being clicked is named 'delete' the columns in the actual table are 'INDEX' 'SUBJECT' 'MSG_TEXT' and 'MSG_DATE' Here is the code: <?php if($_POST['delete']) // from button name="delete" { for($i=0;$i<$count;$i++) { $del_id = $i; $sql = "DELETE FROM FORUM_MESSAGE WHERE id='$del_id'"; $result = mysql_query($sql) or die (mysql_error()); } if($result) { header('Location: message-deleted.php'); } } ?> Thanks again! Ryan
-
There is progess. I had some nice syntax errors that I had to fix in order for the results to display. The $i function is working, but now I am having some more coding errors. Easier stuff this time. I can't delete the row from the table. The code is very short and is as follows: <?php if($_POST['delete']) // from button name="delete" { for($i=0;$i<$count;$i++) { $del_id = $i; $sql = "DELETE FROM FORUM_MESSAGE WHERE id='$del_id'"; $result = mysql_query($sql) or die (mysql_error()); } if($result) { header('Location: message-deleted.php'); } } ?> Thanks again! Ryan
-
You guys are so smart. Will give that a whirl and post a reply if I am still having issues. Ryan
-
Here is my basic table set-up: table name: FORUM_MESSAGE SUBJECT, varchar(100), input box MSG_TEXT, varchar(500), textfield MSG_DATE, timestamp Ryan
-
If what you say is true, which I believe, then how exactly would I make the string look? Outside of that, I should be good to go on finishing the script and testing it. Ryan
-
So what your saying is that I can only do one? The problem is that I want to pull up the message_text associated with the subject and modify that. Can't I just assign numerical values to rows with rows containing the subject, message_text, delete button and edit button? That way I can delete a post with one button and query the DB with the other post so that I can use the UPDATE script? Hope I explained this so that you understand. Thanks again. Ryan
-
It shows my html coding, but the PHP is gone and not querying the database. Here is the code that works, but does not incorporate the $i listing element: <?php $subject = $_POST['subject']; $message_text = $_POST['message_text']; //Connect to mysql server $link = mysql_connect('localhost', 'ryan_ryan', 'nealon'); if(!$link) { die('Failed to connect to server: ' . mysql_error()); } //Select database $db = mysql_select_db('ryan_iframe'); if(!$db) { die("Unable to select database"); } // validate incoming values $subject = (isset($_GET['subject'])) ? (int)$_GET['subject'] : 0; $message_text = (isset($_GET['message_text'])) ? (int)$_GET['message_text'] : 0; ob_start(); $query = sprintf(' SELECT SUBJECT, MSG_TEXT, UNIX_TIMESTAMP(MSG_DATE) AS MSG_DATE FROM FORUM_MESSAGE WHERE SUBJECT = %d OR MSG_TEXT = %d ORDER BY MSG_DATE DESC', DB_DATABASE, DB_DATABASE, $subject, $subject); $result = mysql_query($query) or die(mysql_error()); $num=mysql_numrows($result); while ($row = mysql_fetch_assoc($result)) { echo '<div style="width: 400px;padding:20px;">'; echo '<table border=0 width="400px">'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo 'Date: '; echo '</td>'; echo '<td style="vertical-align:top;width:320px;">'; echo date('F d, Y', $row['MSG_DATE']) . '</td>'; echo '</tr>'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo 'Subject: '; echo '</td>'; echo '<td style="vertical-align:top;width:320px;">'; echo '<div>' . htmlspecialchars($row['SUBJECT']) . '</div>'; echo '</td>'; echo '</tr>'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo 'Message: '; echo '</td>'; echo '<td style="vertical-align:top;width:320px;">'; echo '<div>' . htmlspecialchars($row['MSG_TEXT']) . '</div>'; echo '</td>'; echo '</tr>'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo '</td>'; echo '<td style="vertical-align:top;width:320px;text-align:center;">'; echo '<form method="post">'; echo '<input type="hidden" name="update" value="true" />'; echo '<input type="submit" value="Update" />'; echo ' '; echo '<input type="hidden" name="delete" value="true" />'; echo '<input type="submit" value="Delete" />'; echo '</form>'; echo '</td>'; echo '</tr>'; echo '</table>'; echo '<br />'; echo '<hr />'; echo '</div>'; } mysql_free_result($result); ?> When I incorporate the $i = 0 and other script to create rows/lists, it no longer queries the database. Check my initial post for the scripting that does not work. Thanks again! Ryan
-
I assume I just do: <?php ... ?> Didn't know about this, my bad...
-
Hello all, Trying to figure out how to display database results so that they are numbered (for editing and deletion purposes). I want to be able to edit the message text and update the database information with the UPDATE command, but have not gotten that far yet. Current problem is that I am returning no results. Here is my script: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>My Profile</title> <link href="loginmodule.css" rel="stylesheet" type="text/css" /> </head> <body> <h1>My Profile </h1> <a href="member-index.php">Home</a> | <a href="member-profile.php">My Profile</a> | Update Posts | <a href="logout.php">Logout</a> <br /><br /> <?php $subject = $_POST['subject']; $message_text = $_POST['message_text']; //Connect to mysql server $link = mysql_connect('XXXXXX', 'XXXXXX', 'XXXXXX'); if(!$link) { die('Failed to connect to server: ' . mysql_error()); } //Select database $db = mysql_select_db('ryan_iframe'); if(!$db) { die("Unable to select database"); } // validate incoming values $subject = (isset($_GET['subject'])) ? (int)$_GET['subject'] : 0; $message_text = (isset($_GET['message_text'])) ? (int)$_GET['message_text'] : 0; ob_start(); $id = $_GET['SUBJECT']; $query = sprintf( " SELECT SUBJECT, MSG_TEXT, UNIX_TIMESTAMP(MSG_DATE) AS MSG_DATE FROM FORUM_MESSAGE WHERE SUBJECT = '$id' ORDER BY MSG_DATE DESC", DB_DATABASE, DB_DATABASE, $subject, $subject); $result = mysql_query($query) or die(mysql_error()); $num = mysql_numrows($result); mysql_close(); $i = 0; while ($i < $num) { $subject = mysql_result($result, $i, "SUBJECT"); $message_text = mysql_result($result, $i, "MSG_TEXT"); echo '<div style="width: 400px;padding:20px;">'; echo '<table border=0 width="400px">'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo 'Date: '; echo '</td>'; echo '<td style="vertical-align:top;width:320px;">'; echo date('F d, Y', $row['MSG_DATE']) . '</td>'; echo '</tr>'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo 'Subject: '; echo '</td>'; echo '<td style="vertical-align:top;width:320px;">'; echo '<div>' . htmlspecialchars($row['SUBJECT']) . '</div>'; echo '</td>'; echo '</tr>'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo 'Message: '; echo '</td>'; echo '<td style="vertical-align:top;width:320px;">'; echo '<div>' . htmlspecialchars($row['MSG_TEXT']) . '</div>'; echo '</td>'; echo '</tr>'; echo '<tr>'; echo '<td style="vertical-align:top;width:auto;">'; echo '</td>'; echo '<td style="vertical-align:top;width:320px;text-align:center;">'; echo '<form method="post">'; echo '<input type="hidden" name="update" value="true" />'; echo '<input type="submit" value="Update" />'; echo ' '; echo '<input type="hidden" name="delete" value="true" />'; echo '<input type="submit" value="Delete" />'; echo '</form>'; echo '</td>'; echo '</tr>'; echo '</table>'; echo '<br />'; echo '<hr />'; echo '</div>'; ++$i; } ?> </body> </html> Thanks in advance. Ryan
-
The mysql_error() did wonders for me! Not only was my syntax messed up in the $query string and the associated lines, but thanks to my organizational skill, the script was not actually connecting to the database. mysql_connect() and mysql_select_db() did the trick and it now works. You guys are awesome! Ryan
-
Will give these a whirl and reply with the results.
-
Hello PHP freaks. Having a few issues with my PHP scripts, specifically the $query = sprintf and mysql_real_escape_string functions. Kind of new to this, so if you do reply, explain it to me like I am a complete moron... Oh, before I forget. My specific problem is that I can click the "Post" button and follow the header to "Location: view.php", but the actual text in the Subject and Message fields is not being sent to the database. Finally managed to get rid of all the error messages I was getting, and now I get this... Thanks in advance! Here is my script for the entire page: _____________________________________________________________ <?php require_once('auth.php'); ?> <?php mysql_connect('xxxxxx', 'xxxxxx', 'xxxxxx'); $subject = $_POST['subject']; $message_text = $_POST['message_text']; // add entry to the databse if the form was submitted and // the necessary information was supplied in the form if (isset($_POST['submitted']) && $subject && $message_text) { $query = sprintf('INSERT INTO FORUM_MESSAGE (SUBJECT, MSG_TEXT) VALUES ($subject, $message_text)', Ryan_iframe, mysql_real_escape_string($subject), mysql_real_escape_string($message_text)); mysql_query($query); // redirect user to list of forums after new record has been stored header('Location: view.php'); } // form was submitted but not all the information was correctly filled in else if (isset($_POST['submitted'])) { $message = '<p>Not all information was provided. Please correct ' . 'and resubmit.</p>'; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Member Index</title> <link href="loginmodule.css" rel="stylesheet" type="text/css" /> </head> <body> <h1>Welcome <?php echo $_SESSION['SESS_FIRST_NAME'];?></h1> Home | <a href="member-profile.php">My Profile</a> | <a href="logout.php">Logout</a> <br /><br /> <form method="post"> <div> <label for="subject">Subject: </label> <input type="text" id="subject" name="subject" value="<?php echo htmlspecialchars($subject); ?>" /><br /> <label for="message_text">Message: </label> <input type="text" id="message_text" name="message_text" value="<?php echo htmlspecialchars($message_text); ?>" /><br /> <input type="hidden" name="submitted" value="true" /> <input type="submit" value="Post" /> </div> </form> </body> </html>