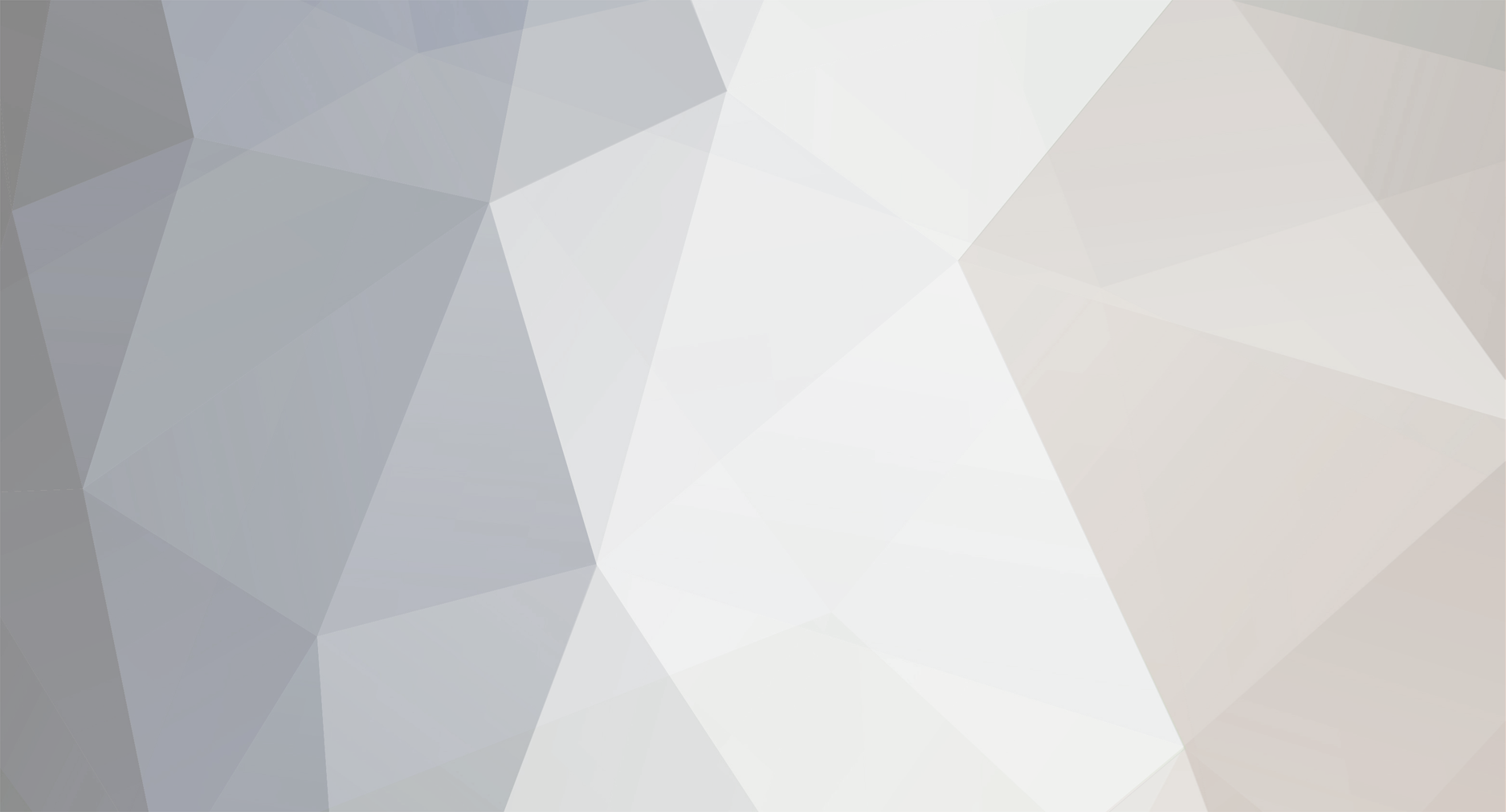
beegro
-
Posts
69 -
Joined
-
Last visited
Never
Posts posted by beegro
-
-
The function ini_set() is an "on the fly" modification to the php.ini file. The php.ini file is the PHP configuration file which controls how PHP will operate, manage memory, allow/disallow functionality, etc. So, by calling ini_set("display_errors", "1") you are overriding the php.ini setting of "display_errors" to now equal the value "1" for the duration of this script's execution.
-
Just to be clear, have you added session_start() to the top of EVERY page where you will be using session data before testing?
-
I see you're using the DOM object from PHP. Take a look at this URL for some examples of removing child elements form your XML document: http://us.php.net/manual/en/domnode.removechild.php
-
You can just import the file and then parse it. I suggest using XML because it's human and machine readable. So if you have an XML file with the numbers you can do the following:
$filename = "/some/file/location/file.xml"; $contents = file_get_contents($filename); $results = SimpleXML($contents); // take a look at your data print_r($results);
-
The easiest way is probably to add a parameter to the javascript function call with identifying information.
i.e.
$javacode = "javascript:popup_show('popup', 'popup_drag', 'popup_exit', 'screen-top-left', 1200, 20, $player1)";
Then you'll have to adjust the javascript function to use that new parameter
-
Are you getting any javascript errors in the browser when you click one of the links?
-
I just tried to get my script to run against that soap service and had an issue with connection. If there are connection credentials you may need to change the constructor arguments to include authentication information. See: http://us3.php.net/manual/en/soapclient.soapclient.php
-
From what I see there is a 'getKey' operation which uses a 'getKeyRequest' input message. The 'getKeyRequest' message has the format of:
<user>
<password>
So, your request would look something like.
$params = array('user' => 'my_username', 'password' => 'my_password'); $client = new SoapClient('http://www.maxbounty.com/api/api.cfc?wsdl'); $result = $client->__soapCall('getKey',$params);
$result will then be of type 'getKeyResponse' which will look like an array
$result['getKeyReturn']
The function print_r() is definitely your friend when working with SOAP and so are try catch blocks.
-
What does the WSDL look like? Can you post it?
-
Assuming it's a fully qualified URL
echo 'Website: <a href="'.$value['website'].'">' . $value['website'] . '</a><br />';
-
In terms of security you're doing 2 less-than-secure things here.
[*]storing a users password in plain text in the database
[*]storing the users password in the session
Is there a reason you'd need the password again later in the session and not just for comparison at login? Also, if someone, including other developers, get into the database and can see all the users individual passwords then their accounts are compromised. It's simply bad practice to store passwords in plain text.
-
You'll have to make the query string assignment as a single quote.
ex.
$query = 'INSERT mytable SET myfield=\'I would like to have this field contain this information with a $matches[1] showing in the field value as well\'';
That tells PHP not to look for PHP variables inside the string. It is a literal assignment.
-
All that code does is create a handle for a new PDF document. Is that all you're wanting to do?
-
I'm surprised that Dreamweaver gave you problems. I used it for years without issue. Guess I'll have to look more closely at the link Pikachu2000 sent regarding UTF encoding. That being said, I have moved on to NetBeans as my editor of choice because of its ability to expose and navigate through OO code as well as snap into source control tools like CVS, SVN and Git.
-
You should be fine in Dreamweaver. It doesn't add anything to the code as long as you're in the "code" pane which you should be in anyway if you're coding. Any luck on finding the white space?
-
What is in the variable $content? It looks fine to me but you'll probably need to apply urlencode() to the $content variable if it has any special characters in it.
-
There are a few lines of code that can echo stuff out to the browser:
echo "<p class='Body-error-P'><span class='Body-text-T'>You forgot to enter your username.</span></p>";
and
echo "<p class='Body-error2-P'><span class='Body-text-T'>You forgot to enter your password.</span></p>";
assuming they aren't actually being executed because you properly passed _POST['username'] and _POST['pass'], is there any other place that sends session, cookie, header or output information to the browsers? The error message is saying something else was outputted to the browser already and cookies have to be first. Make sure that your opening php tag "<?php" is on the first line of the script too.
-
This means that somewhere on your script something outputted data to the browser. What's on line 34?
-
Hmmm, you should get an error from php if the cookie is unable to be set. Is error reporting turned on? I'm going to load these on my machine and check it out.
-
There was a syntax error in my last post it should have a single quote to start the ini_set variable.
Updated:
error_reporting(E_ALL); ini_set('display_errors', 1);
Sorry :-\
-
Great. That means the cookies are being set properly or you should get error messages. As a matter of fact, you should be able to see your cookies in Firefox by right clicking somewhere on the page and selecting "View Page Info" from the menu. Then click the "Security" menu option on the top folowed by the "View Cookies" button. This will show you the cookies that are available to scripts on the site. You should see your domain on the left and "firstname" and "lastname" on the right. Click on those elements and you can see all the information about the cookie.
Can you see your cookies in Firefox?
-
Databases can be configured not to allow certain values and types as well as to require certain values to be of specific sizes, uniqueness, ... well, almost any sort of requirement you can think of a database table can have on its values. I think if there's an issue where sometimes people can submit data and it gets stored and other times it does not then we're looking at a problem with the data. It would be very beneficial to turn on error reporting by putting this at the top of your handling script for some testing to see if you get a message back from MySQL about possible errors with the query when different values are passed through it. This will also let you know if you're having undefined value assignments etc. so only use this for testing.
error_reporting(E_ALL); ini_set("display_errors', 1);
-
Are you getting a successful redirect? I guess I should say, is this line getting echoed to the screen?
print "<meta HTTP-EQUIV='REFRESH' content='5 url=./index.php'>";
-
On the "wall"
The syntax looks good so it may be a database constraint that is disallowing your INSERT. Are you working with error reporting turned on? If so, are you getting any error messages?
PHP rename image to input field
in PHP Coding Help
Posted
Excuse me if I missed it but I don't see any file upload processing in the PHP. Are you not sure how to upload the image?